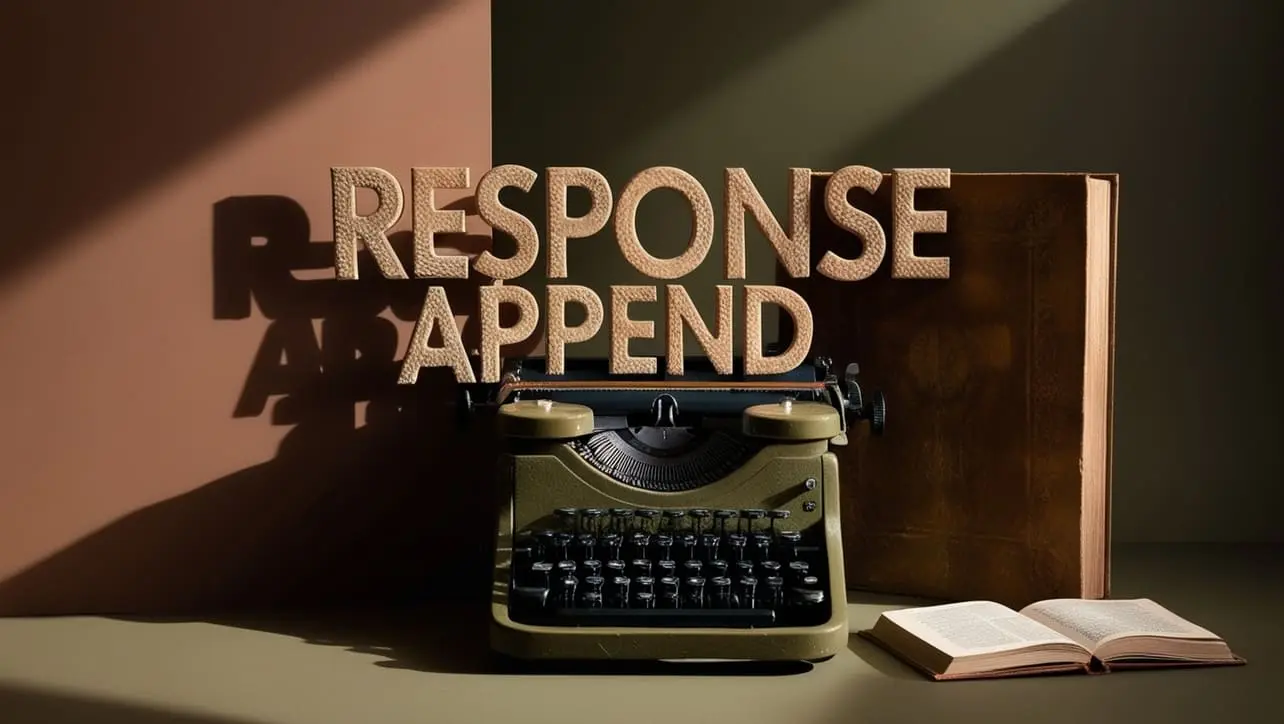
Express.js Basic
- express Intro
- express express()
- express Application
- express Request
Properties
- req.app
- req.baseUrl
- req.body
- req.cookies
- req.fresh
- req.host
- req.hostname
- req.ip
- req.ips
- req.method
- req.originalUrl
- req.params
- req.path
- req.protocol
- req.query
- req.res
- req.route
- req.secure
- req.signedCookies
- req.stale
- req.subdomains
- req.xhr
Methdos
- express Response
- express Router
Express req.host Property
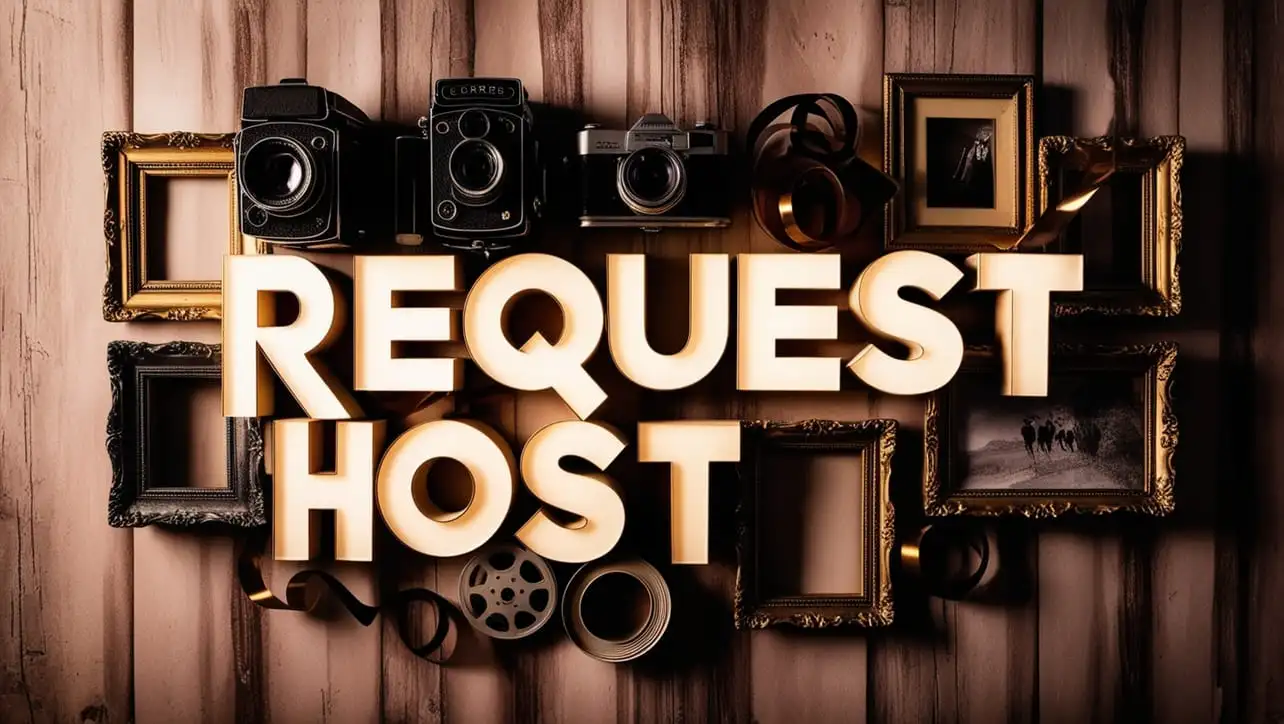
Photo Credit to CodeToFun
🙋 Introduction
In the world of web development, understanding and utilizing information about the host server is crucial.
Express.js, a popular Node.js web application framework, provides the req.host
property to retrieve details about the host from the incoming request.
In this guide, we'll explore the syntax, usage, and practical examples of the req.host
property in Express.js.
💡 Syntax
The syntax for accessing the req.host
property is simple:
const host = req.host;
- req: The request object in an Express route handler.
❓ How req.host Works
The req.host
property in Express.js allows you to retrieve the host name from the incoming request. This information can be valuable for various purposes, such as routing decisions or generating dynamic content based on the host.
app.get('/', (req, res) => {
const host = req.host;
res.send(`Hello from ${host}`);
});
In this example, the req.host
property is used to retrieve the host name from the incoming request and include it in the response.
📚 Use Cases
Dynamic Content Generation:
Utilize
req.host
to generate dynamic content based on the host name, allowing for personalized responses.dynamic-content-generation.jsCopiedapp.get('/', (req, res) => { const host = req.host; // Generate dynamic content based on the host const greeting = host === 'example.com' ? 'Welcome' : 'Hello'; res.send(`${greeting} from ${host}`); });
Host-Based Routing:
Implement host-based routing using
req.host
to direct requests to specific endpoints based on the host name.host-based-routing.jsCopiedapp.get('/', (req, res) => { const host = req.host; // Perform different actions based on the host if (host === 'api.example.com') { // Handle API requests res.send('API endpoint'); } else { // Handle regular requests res.send('Home page'); } });
🏆 Best Practices
Validate Host Information:
Ensure that host information is validated and used securely to prevent potential security vulnerabilities.
validate-host-information.jsCopiedapp.get('/', (req, res) => { const host = req.host; // Validate the host to prevent security issues if (isValidHost(host)) { // Proceed with the request res.send(`Hello from ${host}`); } else { // Respond with an error for invalid hosts res.status(400).send('Invalid host'); } });
Consistent Naming Conventions:
Maintain consistent naming conventions for hosts to simplify conditionals and routing logic.
naming-conventions.jsCopiedapp.get('/', (req, res) => { const host = req.host; // Use consistent naming conventions for hosts const greeting = host.startsWith('api.') ? 'Welcome to the API' : 'Hello'; res.send(`${greeting} from ${host}`); });
🎉 Conclusion
The req.host
property in Express.js is a valuable tool for extracting host information from incoming requests. Whether you're generating dynamic content, implementing host-based routing, or validating hosts securely, understanding and utilizing req.host
can enhance the functionality of your Express.js applications.
Now equipped with knowledge about req.host
, go ahead and leverage this property to tailor your Express.js projects based on host-specific information!
👨💻 Join our Community:
Author
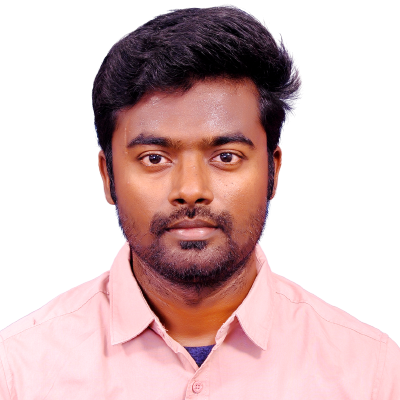
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express req.host Property), please comment here. I will help you immediately.