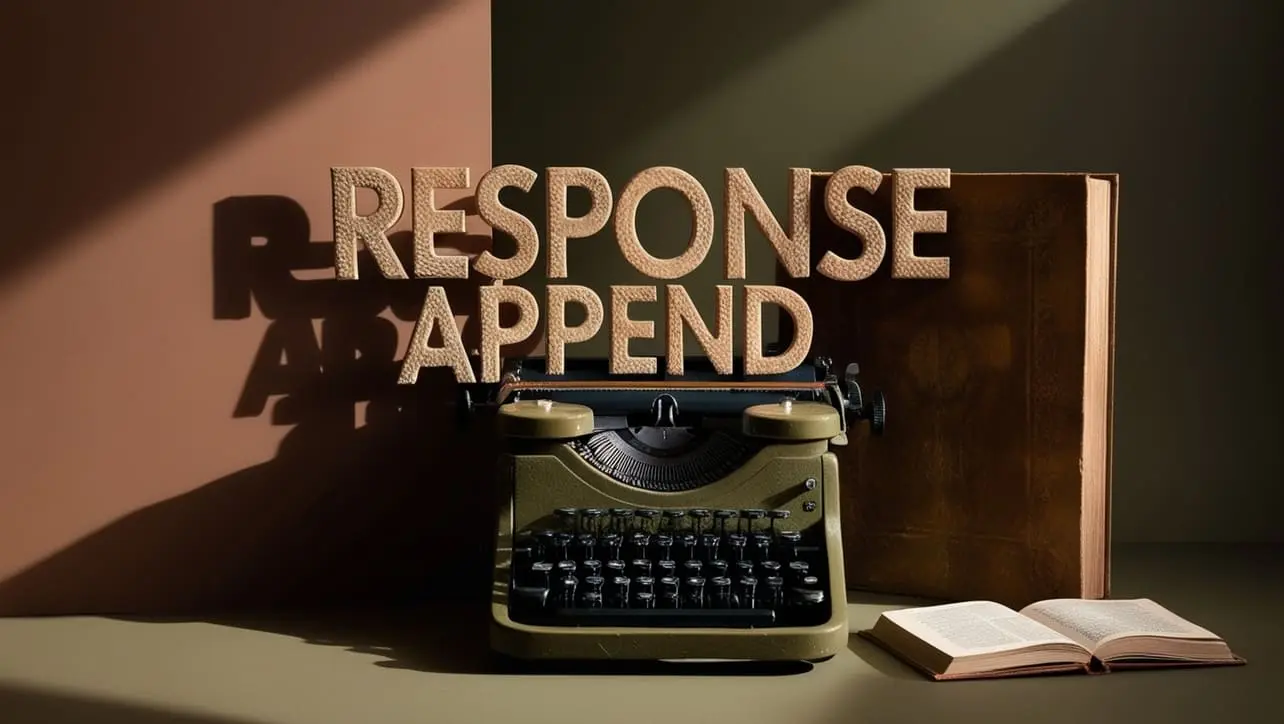
Express req.body Property
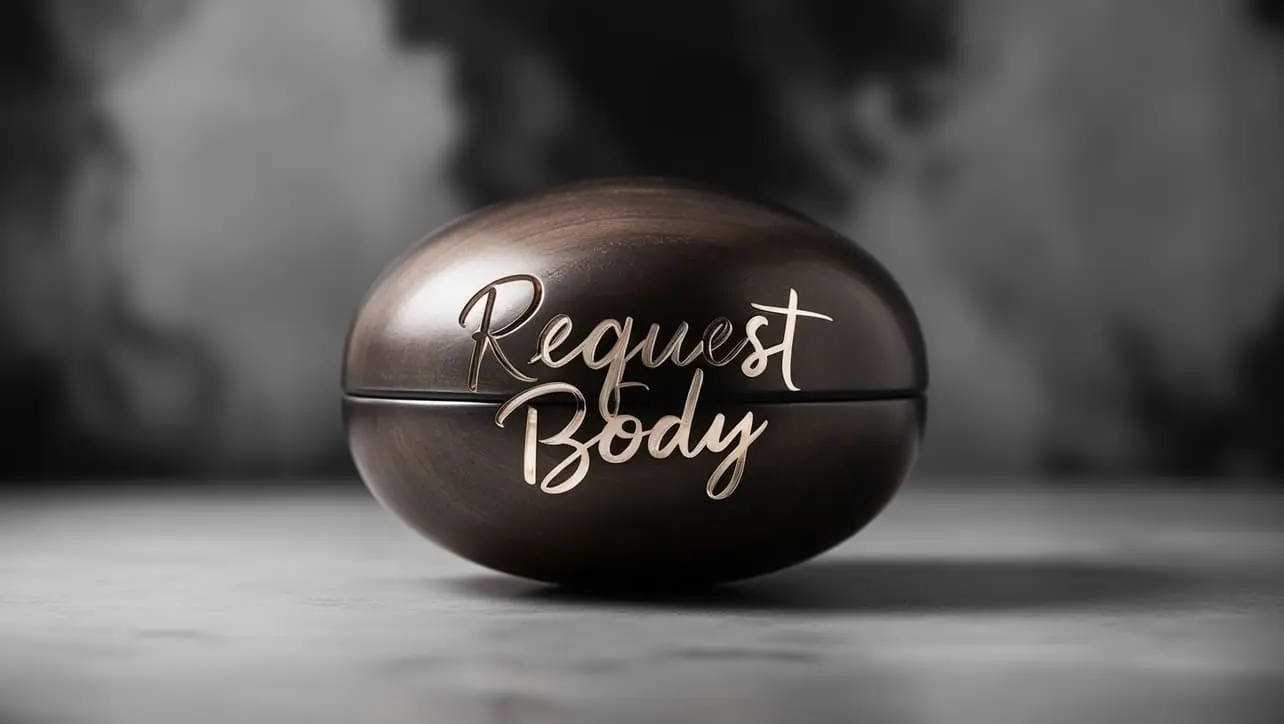
Photo Credit to CodeToFun
🙋 Introduction
Handling data from incoming requests is a crucial aspect of web development, and Express.js simplifies this process through the req.body
property. This property provides access to the data sent in the body of a POST or PUT request.
In this guide, we'll explore the syntax, use cases, and best practices for working with req.body
in Express.js.
💡 Syntax
The req.body
property is automatically populated by Express.js when the express.json() or express.urlencoded() middleware is used. Here's a basic overview:
app.use(express.json()); // for parsing application/json
app.use(express.urlencoded({ extended: true })); // for parsing application/x-www-form-urlencoded
app.post('/example', (req, res) => {
const requestBodyData = req.body;
// Handle the data as needed
});
❓ How req.body Works
When a POST or PUT request is made with data in the request body, the req.body
property is populated with that data. This allows you to access and process the information submitted by the client.
app.post('/submit', (req, res) => {
const formData = req.body;
// Process the form data
res.send(`Received data: ${JSON.stringify(formData)}`);
});
In this example, the req.body
property is used to retrieve form data submitted via a POST request.
📚 Use Cases
Form Submissions:
Handle form submissions by accessing the form data through
req.body
in your Express route.form-submissions.jsCopied<!-- Example HTML form --> <form action="/submit" method="post"> <label for="username">Username:</label> <input type="text" id="username" name="username" required> <button type="submit">Submit</button> </form>
API Endpoints:
For API endpoints, use
req.body
to handle JSON data sent from client applications.api-endpoints.jsCopied// Handling JSON data from a client-side API request app.post('/api/data', (req, res) => { const jsonData = req.body; // Process the JSON data res.json({ message: 'Data received successfully', data: jsonData }); });
🏆 Best Practices
Middleware Order Matters:
Ensure that the body-parsing middleware (express.json() and express.urlencoded()) is applied before your route handlers. The order of middleware matters in Express.js.
middleware-order-matters.jsCopied// Correct order: Apply body-parsing middleware before routes app.use(express.json()); app.use(express.urlencoded({ extended: true })); // Your routes go here
Error Handling:
Implement error handling for cases where the request body might not contain the expected data.
error-handling.jsCopiedapp.post('/submit', (req, res) => { if (!req.body || Object.keys(req.body).length === 0) { return res.status(400).send('Bad Request: Missing or empty request body'); } // Continue processing the form data res.send('Form data received successfully'); });
🎉 Conclusion
Understanding and effectively using req.body
in Express.js is crucial for handling data from client requests. Whether you're dealing with form submissions or processing API data, mastering req.body
empowers you to build dynamic and interactive web applications with ease.
Now, equipped with the knowledge of req.body
properties, go ahead and enhance your Express.js projects by seamlessly handling incoming data!
👨💻 Join our Community:
Author
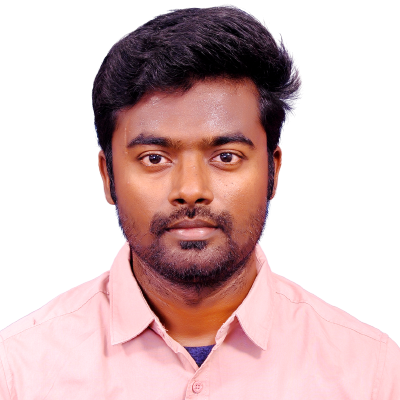
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express req.body Property), please comment here. I will help you immediately.