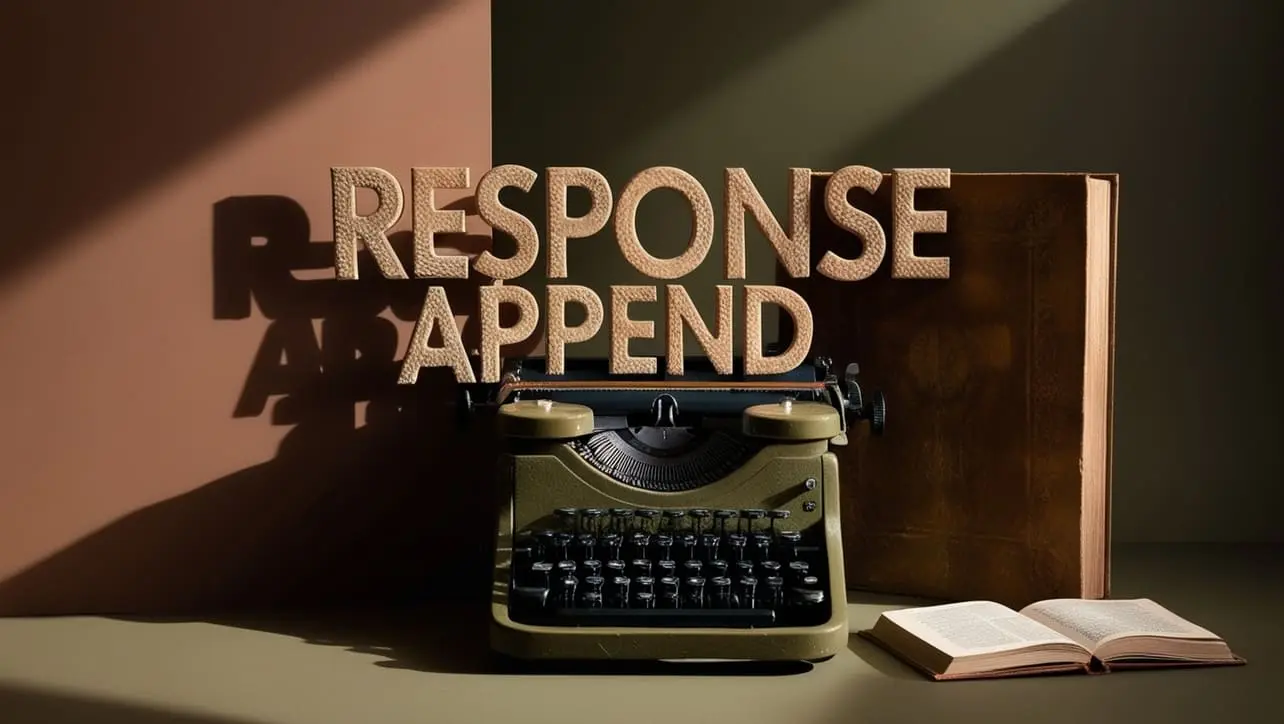
Express req.acceptsEncodings() Method
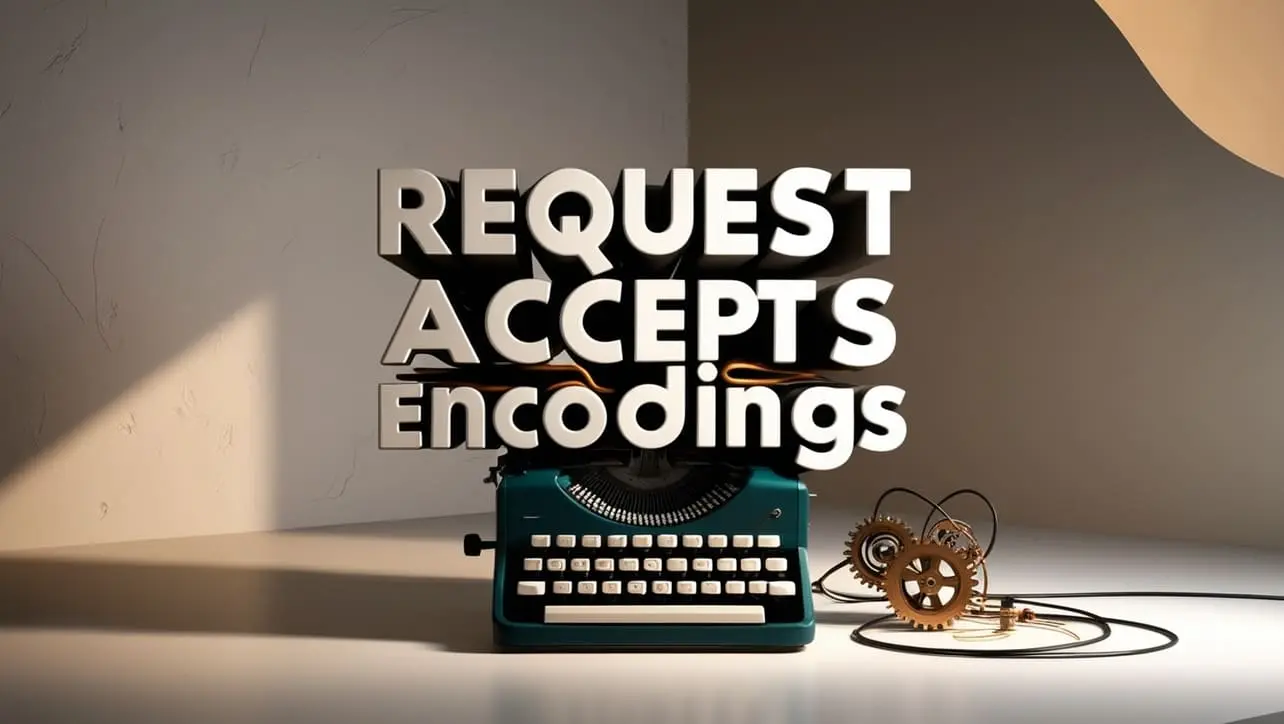
Photo Credit to CodeToFun
🙋 Introduction
Handling content encoding is crucial in web development to optimize data transfer between clients and servers.
Express.js provides various methods to work with HTTP headers, including req.acceptsEncodings()
.
In this guide, we will explore the syntax, usage, and examples of the req.acceptsEncodings()
method, empowering you to efficiently handle content encoding preferences in your Express.js applications.
💡 Syntax
The syntax for the req.acceptsEncodings()
method is straightforward:
req.acceptsEncodings([encoding1, encoding2, ...])
- encoding1, encoding2, ...: An array of encoding types that the client supports.
The method returns the best matching encoding or false if none of the provided encodings are accepted.
❓ How req.acceptsEncodings() Works
The req.acceptsEncodings()
method allows you to determine the preferred content encoding based on the client's preferences. It considers the Accept-Encoding header sent by the client and matches it with the provided encoding types.
app.get('/data', (req, res) => {
const acceptedEncoding = req.acceptsEncodings(['gzip', 'deflate', 'identity']);
if (acceptedEncoding) {
// Respond with the preferred encoding
res.set('Content-Encoding', acceptedEncoding);
// Your logic to send compressed data
res.send(compressedData);
} else {
// If no accepted encoding, send data without compression
res.send(data);
}
});
In this example, the server checks the client's preferred encodings and responds accordingly with the best-matching encoding or uncompressed data.
📚 Use Cases
Efficient Data Transfer:
Leverage
req.acceptsEncodings()
to efficiently transfer large data by sending compressed content when supported by the client.example.jsCopiedapp.get('/large-data', (req, res) => { const acceptedEncoding = req.acceptsEncodings(['gzip', 'deflate', 'identity']); if (acceptedEncoding) { // Respond with the preferred encoding res.set('Content-Encoding', acceptedEncoding); // Your logic to send compressed large data res.send(compressedLargeData); } else { // If no accepted encoding, send large data without compression res.send(largeData); } });
Content Negotiation:
Implement content negotiation using
req.acceptsEncodings()
to serve content in the preferred encoding supported by the client.example.jsCopiedapp.get('/content', (req, res) => { const acceptedEncoding = req.acceptsEncodings(['gzip', 'deflate', 'identity']); if (acceptedEncoding) { // Respond with the preferred encoding res.set('Content-Encoding', acceptedEncoding); // Your logic to send content with negotiated encoding res.send(negotiatedContent); } else { // If no accepted encoding, send content without compression res.send(content); } });
🏆 Best Practices
Provide a Default Encoding:
Always provide a default encoding, such as 'identity', to ensure that the client receives uncompressed data if none of the specified encodings are accepted.
example.jsCopiedconst acceptedEncoding = req.acceptsEncodings(['gzip', 'deflate', 'identity']) || 'identity';
Handle Unsupported Encodings:
Check if the returned encoding is false and handle the case where none of the specified encodings are accepted by the client.
example.jsCopiedconst acceptedEncoding = req.acceptsEncodings(['gzip', 'deflate', 'identity']); if (!acceptedEncoding) { // Handle unsupported encoding case res.status(406).send('Not Acceptable'); }
🎉 Conclusion
The req.acceptsEncodings()
method in Express.js is a powerful tool for managing content encoding preferences between clients and servers. By understanding its usage and integrating it into your routes, you can optimize data transfer and enhance the efficiency of your Express.js applications.
Now, armed with knowledge about req.acceptsEncodings()
, you can fine-tune your content delivery strategies based on client preferences!
👨💻 Join our Community:
Author
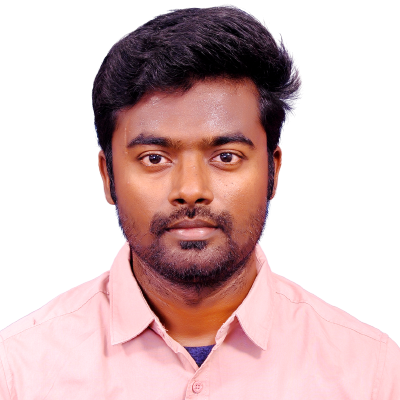
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express req.acceptsEncodings() Method), please comment here. I will help you immediately.