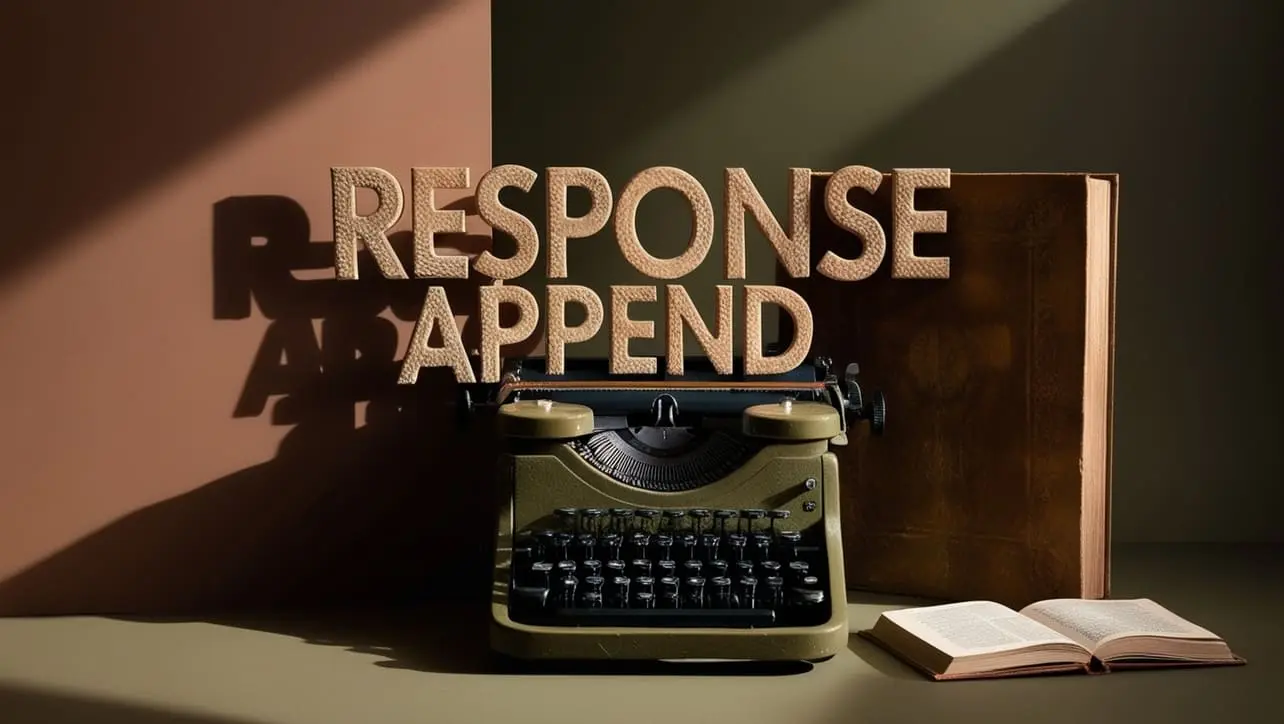
Express.js Request
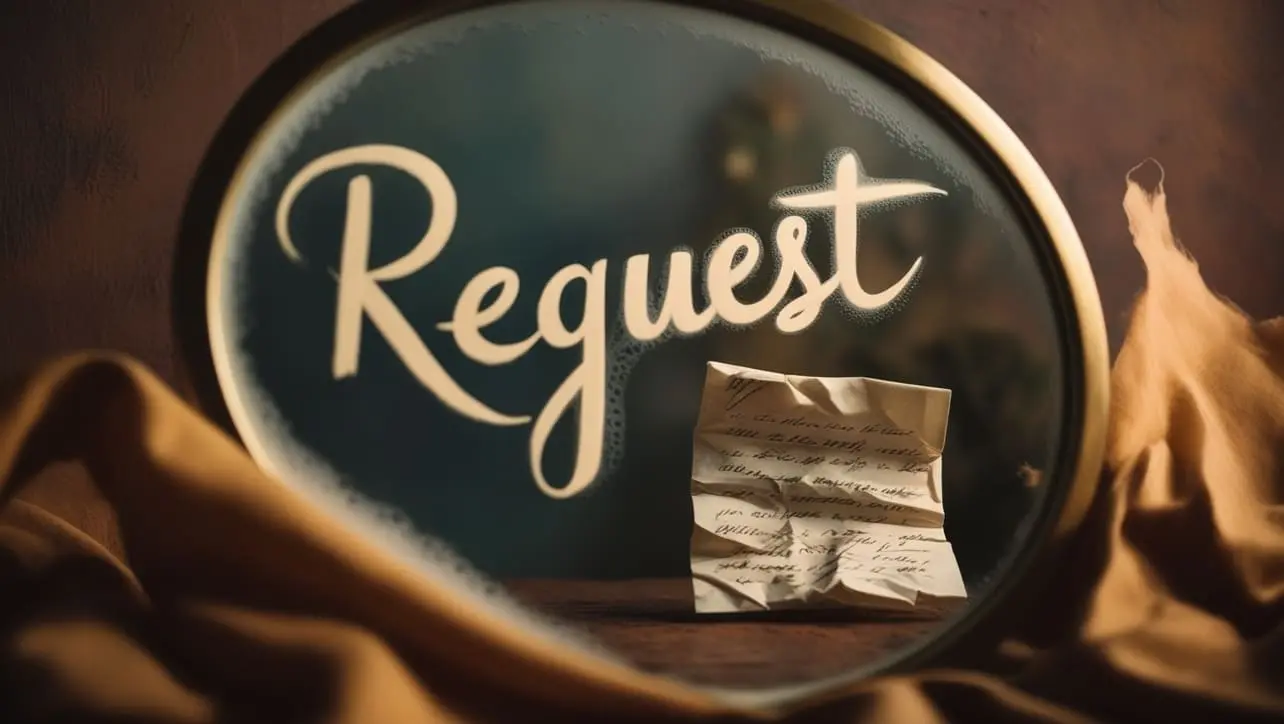
Photo Credit to CodeToFun
🙋 Introduction
In the realm of Express.js, the req
object is a fundamental component, representing the HTTP request made by a client.
Understanding the structure and capabilities of the req
object is essential for building robust and responsive web applications.
This page serves as a comprehensive guide to the req
object, providing insights into its properties, methods, and best practices.
🧐 What is the req Object?
The req
object in Express.js is a crucial component that encapsulates all information related to the incoming HTTP request made by a client. It is automatically created and populated by Express.js when a client interacts with your server.
The req
object holds essential details such as URL parameters, query parameters, request headers, and the request body. This enables developers to extract, process, and respond to client input effectively.
🏁 Initializing the req Object
When a client sends an HTTP request to your Express.js server, the req
object is automatically created. It holds vital information about the request, including the URL, HTTP method, headers, and any data sent by the client. Let's explore the key aspects of the req
object.
🧠 Anatomy of the req Object
Basic Structure:
The
req
object encapsulates various properties and methods that enable developers to access details about the incoming request. From extracting parameters to handling query strings, thereq
object is your gateway to seamlessly interacting with client requests.
🔑 Key Features and Methods
req.params:
The req.params property allows you to access route parameters specified in the URL. Learn how to leverage this property to capture dynamic values from the URL and tailor your server's response accordingly.
req-params.jsCopied// Define a route with a parameter app.get('/users/:userId', (req, res) => { const userId = req.params.userId; res.send(`User ID: ${userId}`); });
req.query:
Query parameters play a crucial role in client-server communication. The req.query property simplifies the extraction of query parameters from the URL, providing a convenient way to handle client input.
req-query.jsCopied// Handle a request with query parameters app.get('/search', (req, res) => { const query = req.query.q; res.send(`Search query: ${query}`); });
req.body:
When clients send data in the request body, the req.body property becomes essential for accessing that information. Discover how to parse and handle data sent via POST requests.
req-body.jsCopied// Middleware to parse JSON requests app.use(express.json()); // Handle a POST request with JSON data app.post('/api/data', (req, res) => { const jsonData = req.body; res.json({ message: 'Data received successfully', data: jsonData }); });
🚀 Advanced Features
File Uploads:
Explore how to handle file uploads using the req.file property and the multer middleware. Learn to manage and process uploaded files seamlessly in your Express.js application.
file-uploads.jsCopiedconst multer = require('multer'); const upload = multer({ dest: 'uploads/' }); // Handle file upload app.post('/upload', upload.single('file'), (req, res) => { const uploadedFile = req.file; res.json({ message: 'File uploaded successfully', file: uploadedFile }); });
Request Headers:
Gain insights into accessing and utilizing request headers using properties like req.headers. Learn how to enhance security and customization by inspecting and manipulating headers in your Express.js application.
request-headers.jsCopied// Access and utilize request headers app.get('/headers', (req, res) => { const userAgent = req.headers['user-agent']; res.send(`User-Agent: ${userAgent}`); });
📝 Example
Let's illustrate the usage of the req
object with a comprehensive example:
const express = require('express');
const app = express();
// Define a route with parameters and query
app.get('/profile/:username', (req, res) => {
const username = req.params.username;
const age = req.query.age || 'Not specified';
res.send(`Profile: ${username}, Age: ${age}`);
});
// Handle POST request with JSON data
app.post('/api/user', express.json(), (req, res) => {
const userData = req.body;
res.json({ message: 'User data received successfully', data: userData });
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
In this example, we define routes with parameters, handle query parameters, and process a POST request with JSON data, showcasing the versatile use of the req
object.
🎉 Conclusion
In conclusion, the req
object is a powerful tool in Express.js, providing developers with the means to extract and handle client input effectively. This overview has covered key properties and methods of the req
object, empowering you to navigate and utilize incoming requests in your Express.js applications.
👨💻 Join our Community:
Author
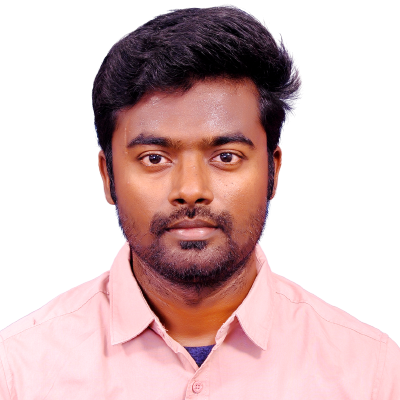
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express.js Request), please comment here. I will help you immediately.