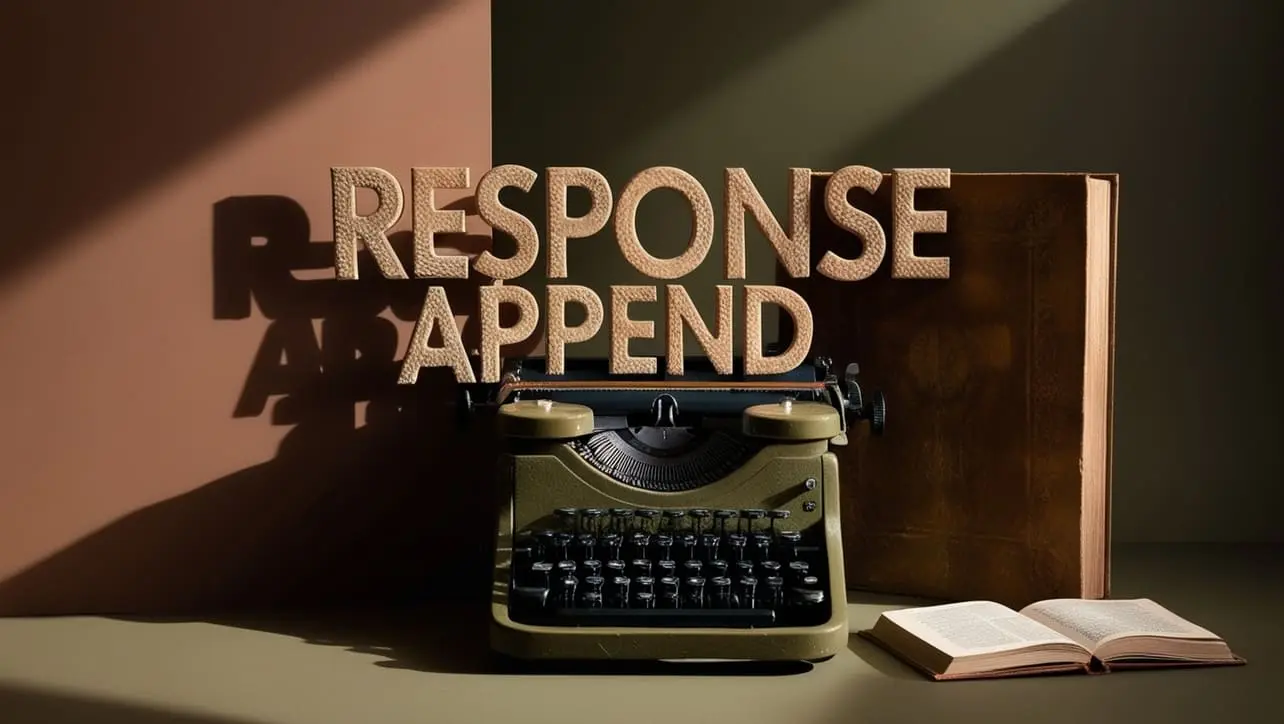
Express.js Introduction
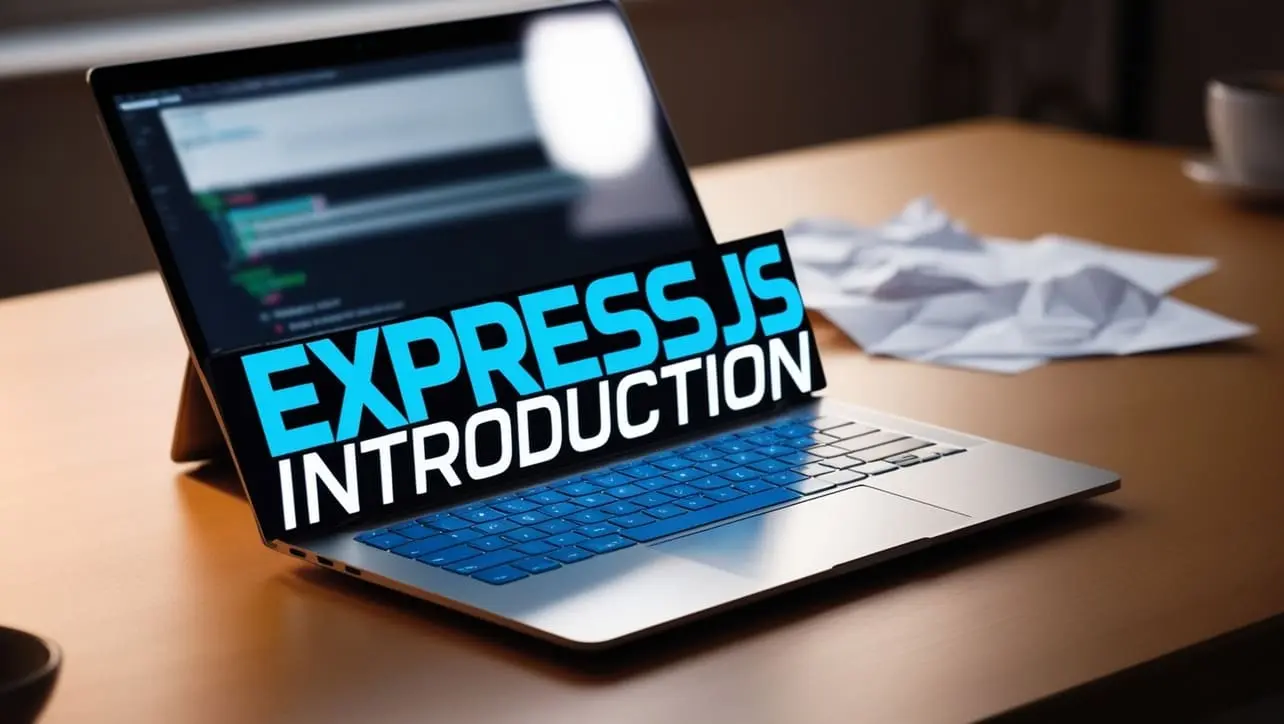
Photo Credit to CodeToFun
Introduction
Express.js, commonly known as Express, is a minimal and flexible Node.js web application framework that provides a robust set of features to develop web and mobile applications.
It simplifies the process of building scalable and maintainable web applications by providing a simple, yet powerful, set of tools for web development.
Key Features of Express.js
Middleware Support:
Express.js is known for its middleware support, which allows developers to extend the functionality of the application by using middleware functions. These functions have access to the request and response objects, enabling tasks such as authentication, logging, and error handling.
middleware-support.jsCopiedconst express = require('express'); const app = express(); // Custom middleware function const customMiddleware = (req, res, next) => { console.log('This middleware runs before the route handler.'); next(); // Call the next middleware or route handler }; // Using middleware in the application app.use(customMiddleware); app.get('/', (req, res) => { res.send('Hello, Express!'); }); const port = 3000; app.listen(port, () => { console.log(`Server is running on http://localhost:${port}`); });
Routing:
Express.js provides a simple and effective routing system that allows developers to define routes based on HTTP methods and URL patterns. This makes it easy to create clean and organized code for handling different parts of your application.
routing.jsCopiedconst express = require('express'); const app = express(); // Define a route for the root URL app.get('/', (req, res) => { res.send('Hello, Express!'); }); // Define a route for '/about' app.get('/about', (req, res) => { res.send('This is the About page.'); }); const port = 3000; app.listen(port, () => { console.log(`Server is running on http://localhost:${port}`); });
Template Engines:
Express.js supports various template engines, such as EJS and Pug, making it easy to render dynamic views on the server. This separation of concerns between the server and the view layer enhances the maintainability of the codebase.
template-engines.jsCopiedconst express = require('express'); const app = express(); // Set the view engine to EJS app.set('view engine', 'ejs'); // Define a route that renders a dynamic view app.get('/', (req, res) => { res.render('index', { title: 'Express.js Example', message: 'Hello, Express!' }); }); const port = 3000; app.listen(port, () => { console.log(`Server is running on http://localhost:${port}`); });
HTTP Utility Methods:
With Express.js, handling HTTP requests and responses becomes straightforward. It provides utility methods for common tasks, such as parsing incoming request bodies, setting response headers, and sending JSON responses.
http-utility-methods.jsCopiedconst express = require('express'); const app = express(); // Parse JSON in request body app.use(express.json()); // Handle POST request and send JSON response app.post('/api/user', (req, res) => { const { username, email } = req.body; res.json({ username, email }); }); const port = 3000; app.listen(port, () => { console.log(`Server is running on http://localhost:${port}`); });
Advantages of Express.js
Fast and Minimalistic:
Express.js is known for its minimalistic design and lightweight nature, making it fast and efficient. It focuses on the core features needed for web development, allowing developers to build applications without unnecessary overhead.
Active Community and Ecosystem:
Express.js has a vibrant and active community, contributing to an extensive ecosystem of middleware and plugins. This active support ensures that developers have access to a wealth of resources and solutions when building Express.js applications.
Flexibility and Customization:
Express.js provides a high level of flexibility, allowing developers to customize their applications according to specific project requirements. It doesn't impose a strict structure, giving developers the freedom to choose the tools and architecture that best fit their needs.
Disadvantages of Express.js
Lack of Built-in Features:
While Express.js provides a solid foundation for building web applications, it intentionally keeps its feature set minimal. Developers may need to rely on additional third-party libraries for certain functionalities, leading to a more modular but potentially fragmented development experience.
Learning Curve for Beginners:
For beginners in web development or Node.js, the learning curve for Express.js might be steeper compared to more opinionated frameworks. Understanding concepts like middleware and routing may require additional time and effort for those new to the framework.
Example
Create a simple Express.js application with the following code:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello, Express!');
});
const port = 3000;
app.listen(port, () => {
console.log(`Server is running on http://localhost:${port}`);
});
This example creates a basic server that listens on port 3000 and responds with "Hello, Express!" when you access the root URL.
Conclusion
Express.js is a powerful and widely used framework in the Node.js ecosystem. Its simplicity, flexibility, and rich set of features make it an excellent choice for building web applications and APIs.
Whether you are a beginner or an experienced developer, Express.js can streamline your development process and help you create scalable and efficient applications.
Join our Community:
Author
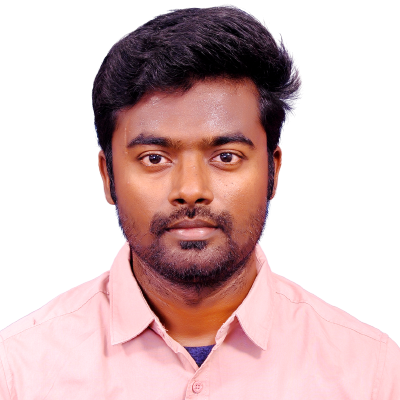
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express.js Introduction), please comment here. I will help you immediately.