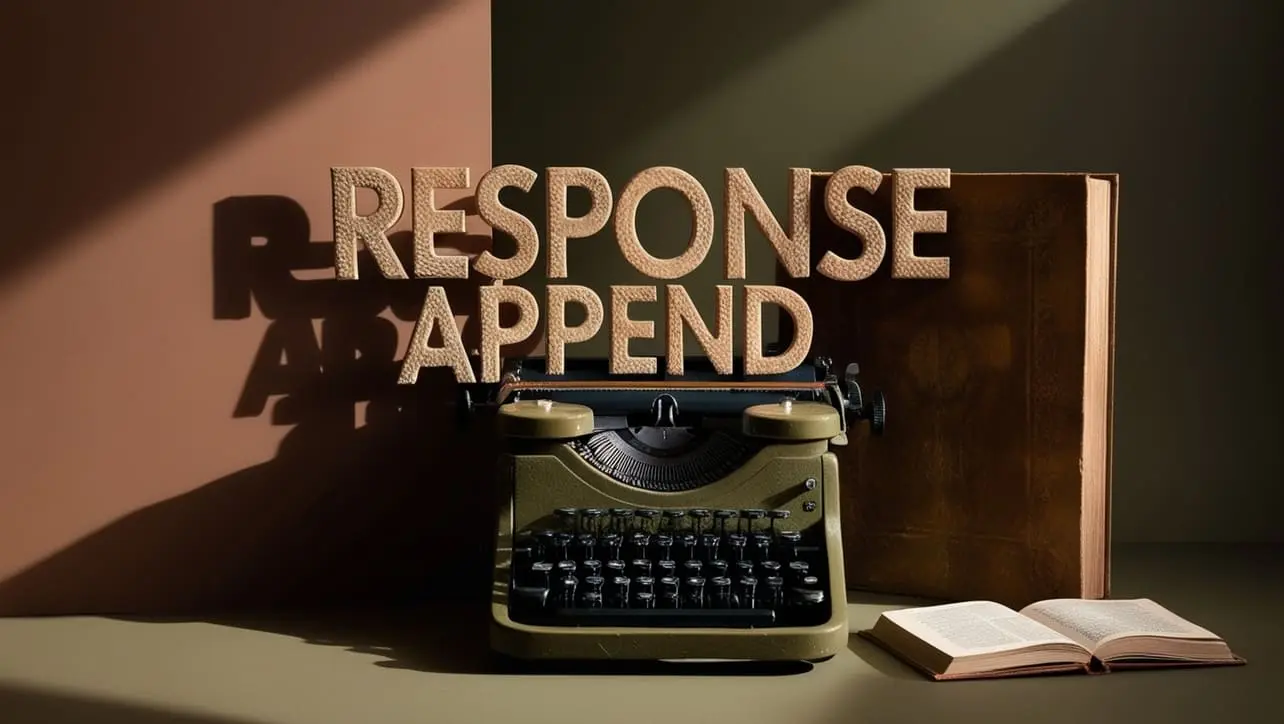
Express express.static() Method
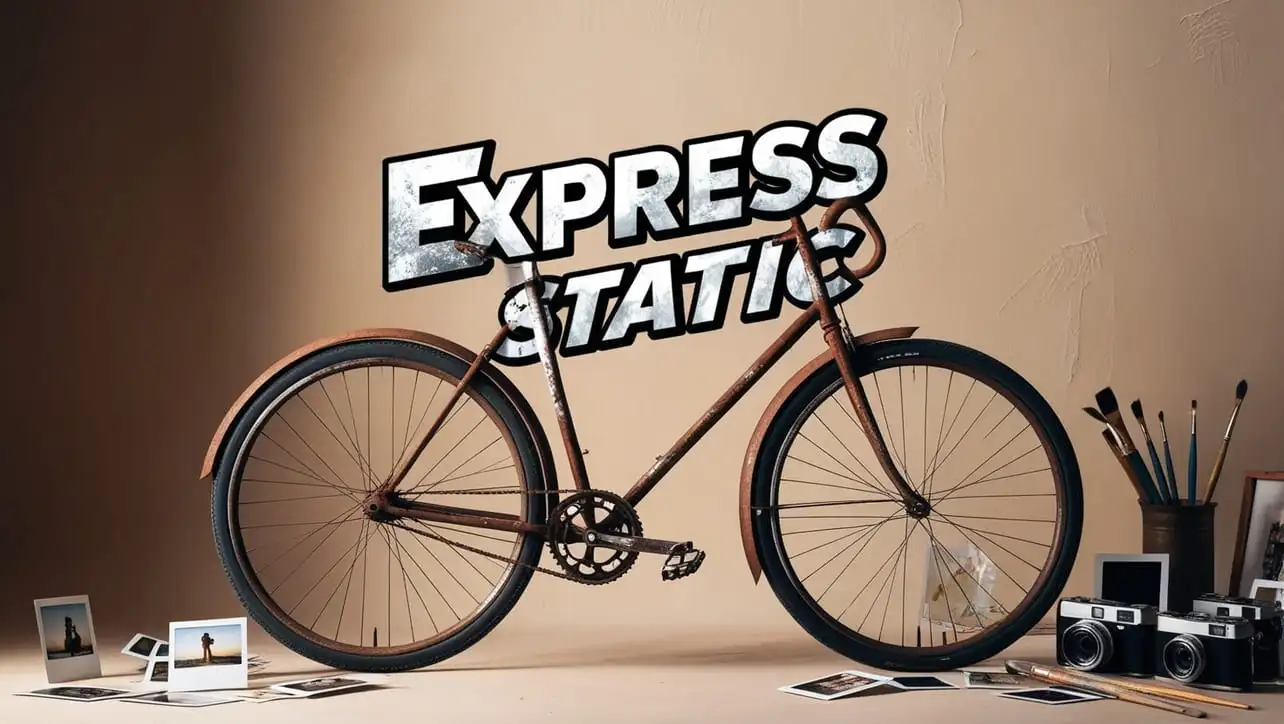
Photo Credit to CodeToFun
🙋 Introduction
In web development, serving static files like images, stylesheets, and scripts is a common requirement.
Express.js simplifies this process through the express.static()
method, making it efficient to serve static assets.
Join us as we explore the syntax, use cases, and best practices of the express.static()
method in Express.js.
💡 Syntax
The syntax for the express.static()
method is straightforward:
express.static(root, [options])
- root: A string specifying the root directory from which to serve static assets.
- options: An optional object providing additional configuration, such as cache settings.
❓ How express.static() Works
express.static()
is Express's built-in middleware function specifically designed for serving static files. When configured with a root directory, it intercepts incoming requests and attempts to match them with files in the specified directory. If a match is found, it sends the file back as the response.
const express = require('express');
const app = express();
// Serve static files from the 'public' directory
app.use(express.static('public'));
// Your route handlers go here
const PORT = 3000;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
In this example, the express.static('public') middleware is added to the application, allowing files in the 'public' directory to be served.
📚 Use Cases
Serving CSS, JavaScript, and Images:
This middleware allows you to serve CSS, JavaScript, and image files stored in the 'public' directory of your project.
example.jsCopiedapp.use(express.static('public'));
Handling Client-Side Libraries:
You can use
express.static()
to serve client-side libraries, making it easy to include them in your HTML files.example.jsCopiedapp.use('/libs', express.static('node_modules'));
🏆 Best Practices
Organize Static Assets:
Organize your static assets logically within a dedicated directory (e.g., 'public'). This makes it easier to manage and maintain your project.
Leverage Subdirectories:
Take advantage of subdirectories within the static directory to organize assets further. For example, place images in public/images and stylesheets in 'public/styles'.
Use a Content Delivery Network (CDN):
Consider using a CDN for hosting static assets in a production environment. This improves performance by serving files from geographically distributed servers.
🎉 Conclusion
The express.static()
method in Express.js simplifies the process of serving static files, enhancing the performance and organization of your web applications. By understanding its syntax, use cases, and best practices, you can effectively integrate static file serving into your Express.js projects.
Now, go ahead and optimize the delivery of static assets in your web applications with the express.static()
method!
👨💻 Join our Community:
Author
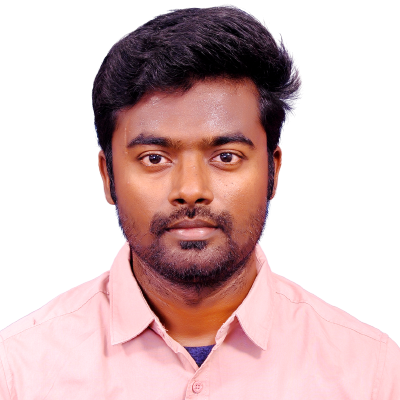
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express express.static() Method), please comment here. I will help you immediately.