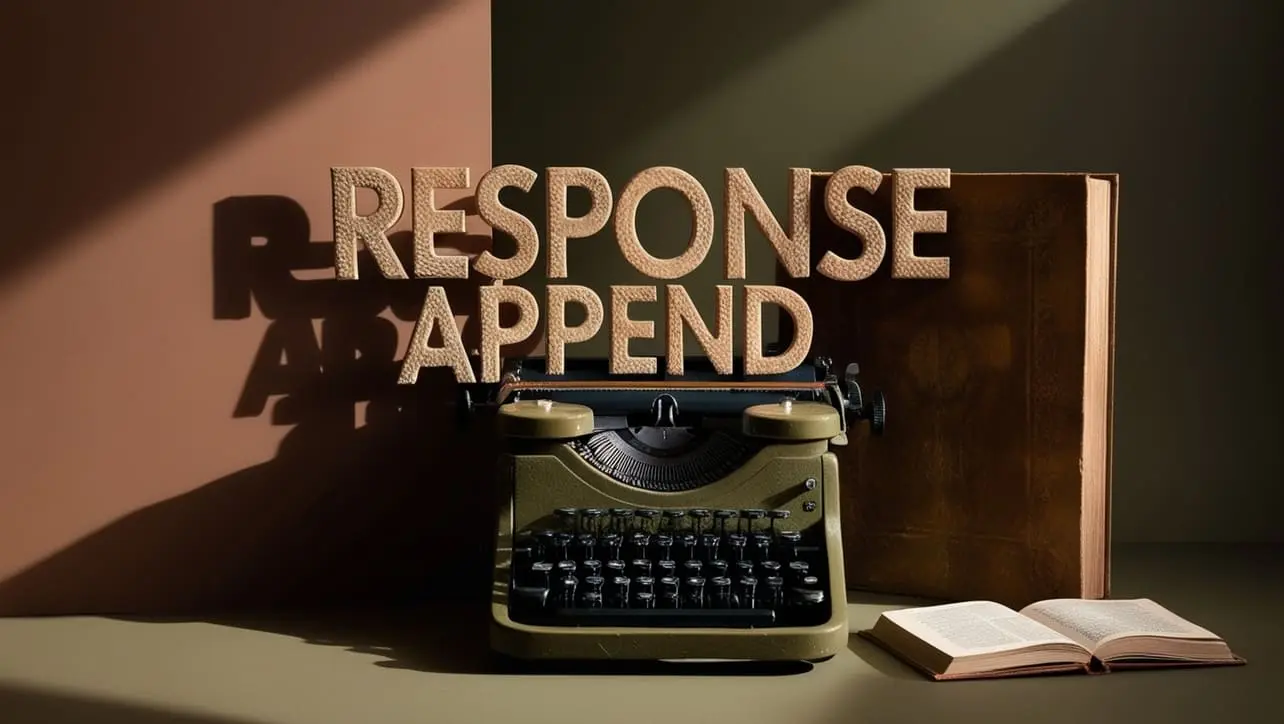
Express express.Router() Method
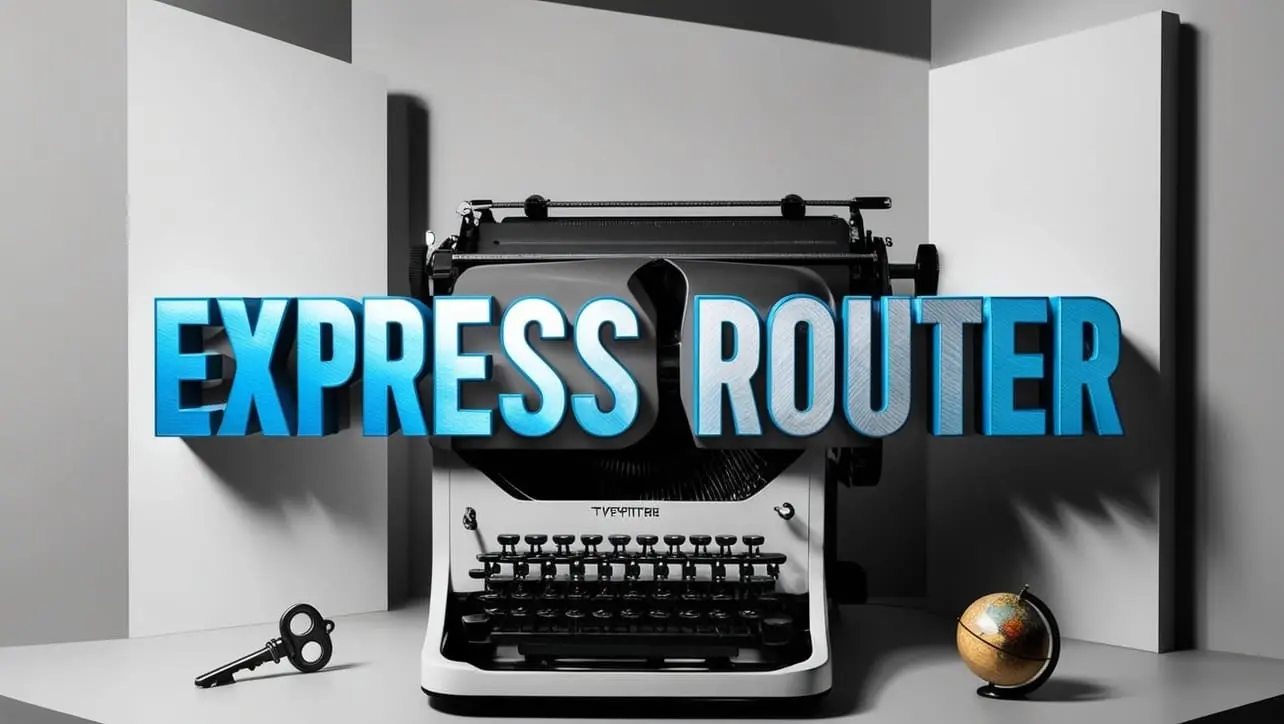
Photo Credit to CodeToFun
Introduction
Express.js provides a powerful feature called express.Router()
that allows you to create modular and mountable route handlers. This method is especially useful when you want to organize your routes into separate files or modules, making your Express application more maintainable and scalable.
In this guide, we'll explore the syntax, usage, and practical examples of the express.Router()
method.
Syntax
The syntax for creating a router using express.Router()
is straightforward:
const express = require('express');
const router = express.Router();
Once you have the router instance, you can define routes and middleware on it, similar to how you would with the main Express application object.
Creating Modular Routes
With express.Router()
, you can break down your application routes into smaller, manageable modules. This is particularly beneficial for larger applications where organizing code becomes crucial.
Create a file named userRoutes.js:
// userRoutes.js
const express = require('express');
const router = express.Router();
router.get('/', (req, res) => {
res.send('User home page');
});
router.get('/profile', (req, res) => {
res.send('User profile page');
});
module.exports = router;
In your main application file:
// app.js
const express = require('express');
const app = express();
// Import the userRoutes module
const userRoutes = require('./userRoutes');
// Mount the userRoutes module at the '/users' path
app.use('/users', userRoutes);
const PORT = 3000;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
With this setup, all routes defined in userRoutes.js are now accessible under the /users path.
Use Cases
Modular Route Organization:
Organize your routes into separate files or modules, making it easier to manage and understand your application structure.
example.jsCopied// userRoutes.js const express = require('express'); const router = express.Router(); router.get('/', (req, res) => { res.send('User home page'); }); router.get('/profile', (req, res) => { res.send('User profile page'); }); module.exports = router;
Route Prefixing:
Prefix routes for a specific module, creating a more organized and expressive API structure.
example.jsCopied// productsRoutes.js const express = require('express'); const router = express.Router(); router.get('/', (req, res) => { res.send('Products home page'); }); router.get('/details', (req, res) => { res.send('Product details page'); }); module.exports = router;
In your main application file:
example.jsCopied// app.js const express = require('express'); const app = express(); // Import the productsRoutes module and mount it at '/products' const productsRoutes = require('./productsRoutes'); app.use('/products', productsRoutes); const PORT = 3000; app.listen(PORT, () => { console.log(`Server is running on port ${PORT}`); });
Best Practices
Modularity:
Break down your routes into modular components to improve maintainability.
Example: Organize routes into separate files and modules, like userRoutes.js and productsRoutes.js.
Route Prefixing:
Use route prefixing to create a logical and organized API structure.
Example: Prefix routes for a specific module, such as /users or /products.
Middleware Integration:
Apply middleware to specific routers for focused functionality.
example.jsCopied// Middleware for logging const logMiddleware = (req, res, next) => { console.log(`Request received for path: ${req.path}`); next(); }; // Apply logging middleware to the userRoutes const userRoutes = require('./userRoutes'); userRoutes.use(logMiddleware); app.use('/users', userRoutes);
Conclusion
The express.Router()
method in Express.js provides a powerful way to create modular and organized route handlers in your applications. By breaking down routes into separate files and using route prefixing, you can significantly enhance the maintainability and scalability of your Express.js projects.
Now, armed with knowledge about the express.Router()
method, go ahead and structure your Express.js applications with modular and organized routes!
Join our Community:
Author
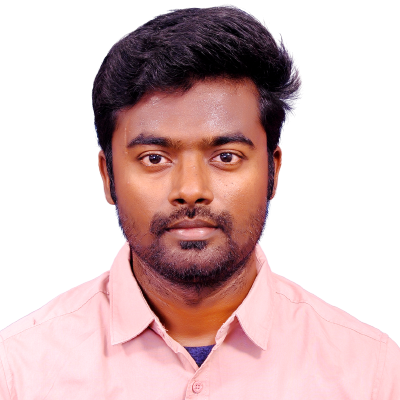
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express express.Router() Method), please comment here. I will help you immediately.