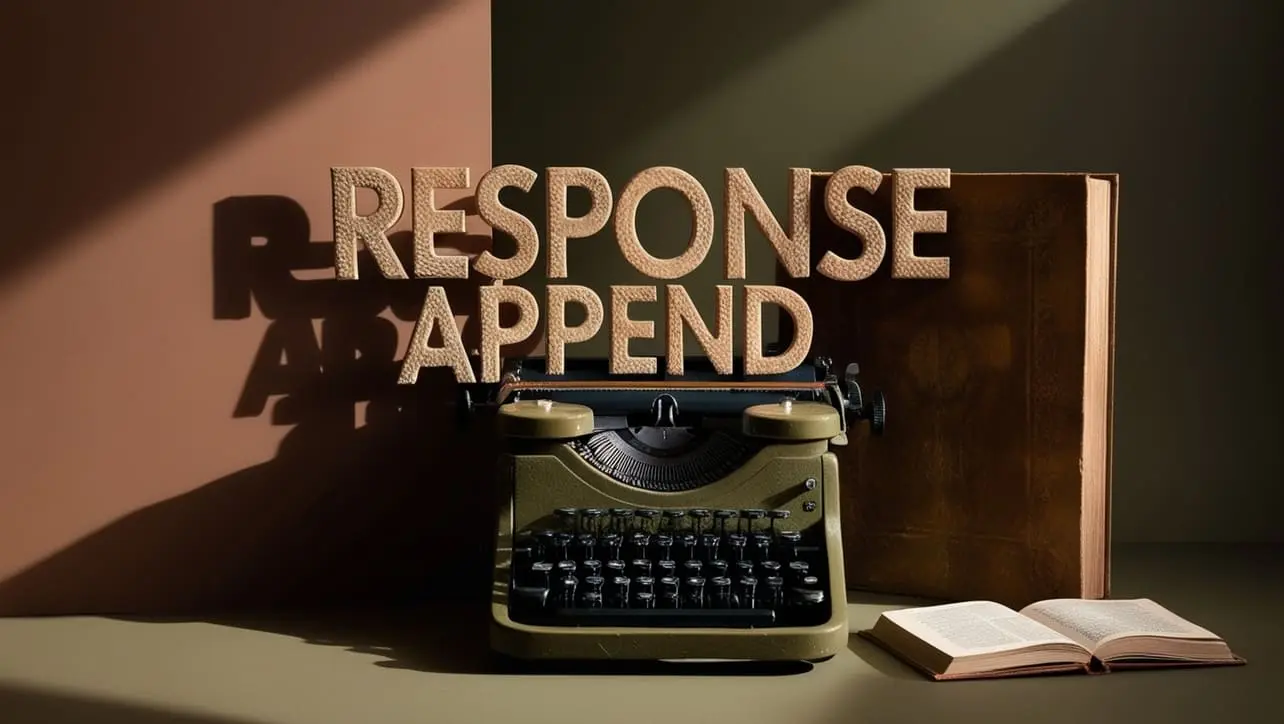
Express express.json() Method
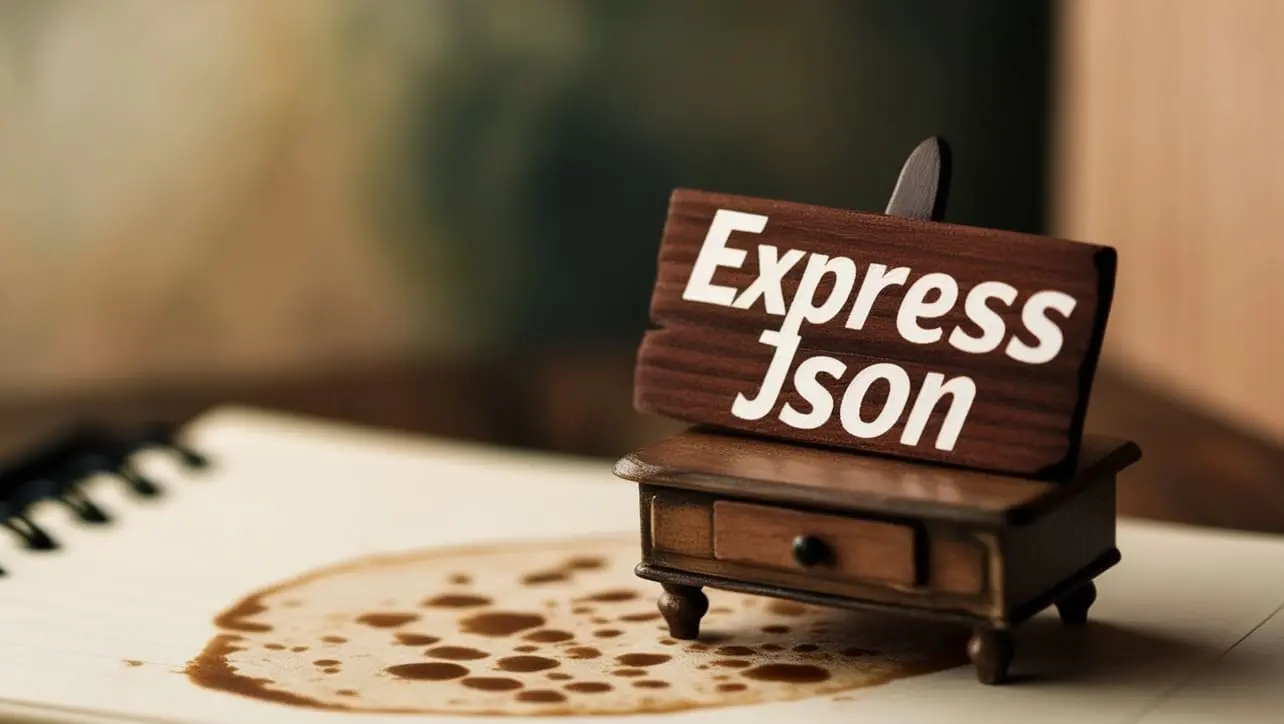
Photo Credit to CodeToFun
🙋 Introduction
Handling JSON data is a common task in modern web development, especially when building APIs. Express.js, a popular Node.js web application framework, simplifies the process with its express.json()
method.
In this guide, we'll explore the syntax, use cases, and best practices of the express.json()
method for effectively parsing JSON in your Express.js applications.
💡 Syntax
The syntax for using express.json()
is straightforward. It is typically used as middleware in your Express application:
const express = require('express');
const app = express();
// Use express.json() middleware
app.use(express.json());
This middleware parses incoming JSON requests and makes the parsed data available in req.body.
❓ How express.json() Works
The express.json()
middleware parses incoming requests with a Content-Type of application/json. It then populates the req.body object with the parsed JSON data, allowing you to access it in your route handlers.
app.post('/api/users', (req, res) => {
// Access JSON data from the request body
const newUser = req.body;
// Logic to add the new user to the database
// ...
res.status(201).json({ message: 'User created successfully', user: newUser });
});
In this example, the express.json()
middleware enables the route to handle JSON data sent in a POST request to create a new user.
📚 Use Cases
Handling JSON in POST Requests:
Use
express.json()
when handling JSON data in POST requests to create resources.example.jsCopiedapp.post('/api/posts', (req, res) => { const newPost = req.body; // Logic to add the new post to the database // ... res.status(201).json({ message: 'Post created successfully', post: newPost }); });
Updating Resources with JSON:
Leverage
express.json()
for updating resources with JSON data in PUT requests.example.jsCopiedapp.put('/api/posts/:postId', (req, res) => { const postId = req.params.postId; const updatedPostData = req.body; // Logic to update the post with updatedPostData // ... res.json({ message: `Post with ID ${postId} updated successfully` }); });
🏆 Best Practices
Validate JSON Data:
Always validate incoming JSON data to ensure it adheres to the expected structure and properties.
example.jsCopiedapp.post('/api/posts', (req, res) => { const newPost = req.body; // Validate required properties in the new post if (!newPost.title || !newPost.content) { return res.status(400).json({ error: 'Title and content are required' }); } // Logic to add the new post to the database // ... res.status(201).json({ message: 'Post created successfully', post: newPost }); });
Use Try-Catch for Error Handling:
Wrap your route handler logic in a try-catch block when working with JSON data to handle parsing or processing errors gracefully.
example.jsCopiedapp.post('/api/posts', (req, res) => { try { const newPost = req.body; // Logic to add the new post to the database // ... res.status(201).json({ message: 'Post created successfully', post: newPost }); } catch (error) { res.status(500).json({ error: 'Internal Server Error' }); } });
🎉 Conclusion
The express.json()
method in Express.js simplifies the handling of JSON data in your applications. Whether you're building RESTful APIs or processing JSON in other scenarios, incorporating express.json()
as middleware ensures seamless parsing and access to JSON data in your Express.js routes.
Now equipped with knowledge about the express.json()
method, enhance your Express.js projects with efficient JSON parsing capabilities!
👨💻 Join our Community:
Author
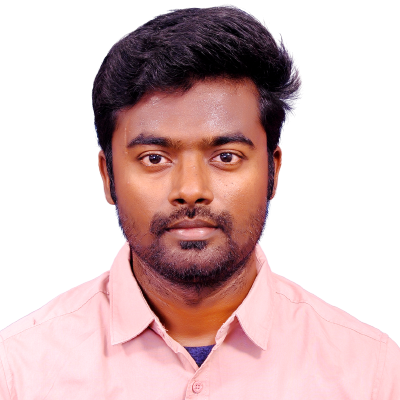
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express express.json() Method), please comment here. I will help you immediately.