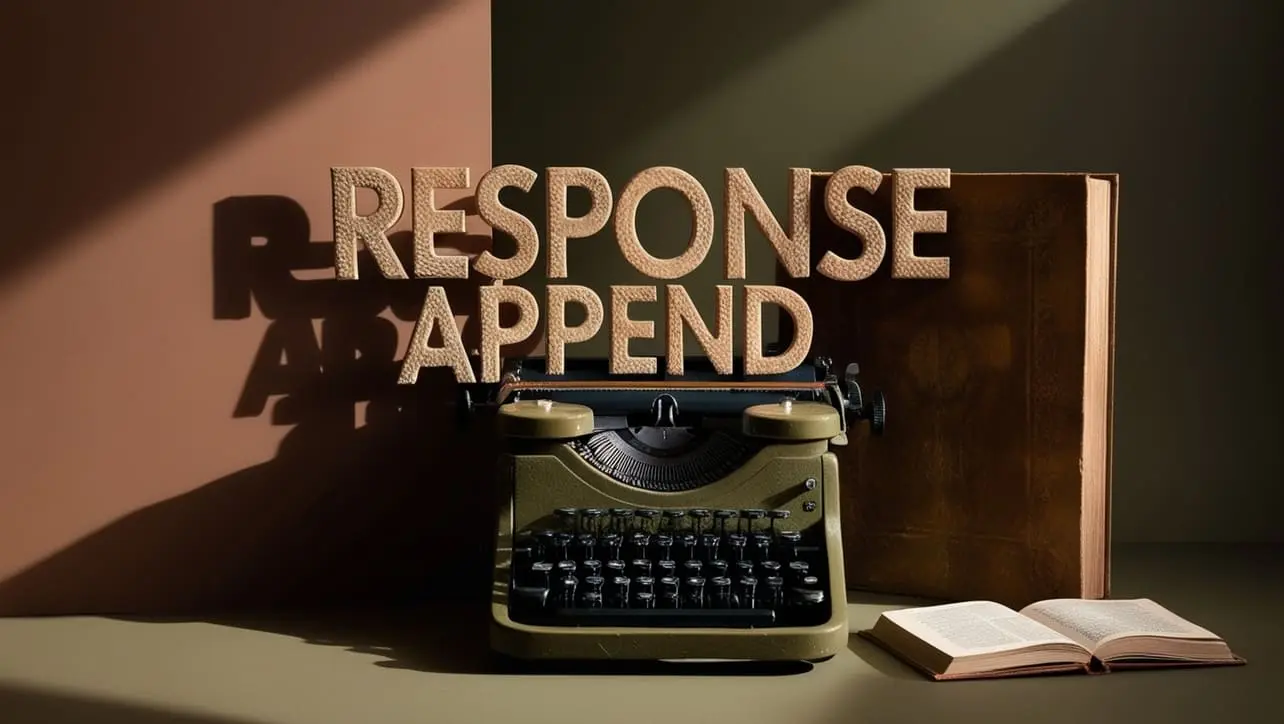
Express.js express()
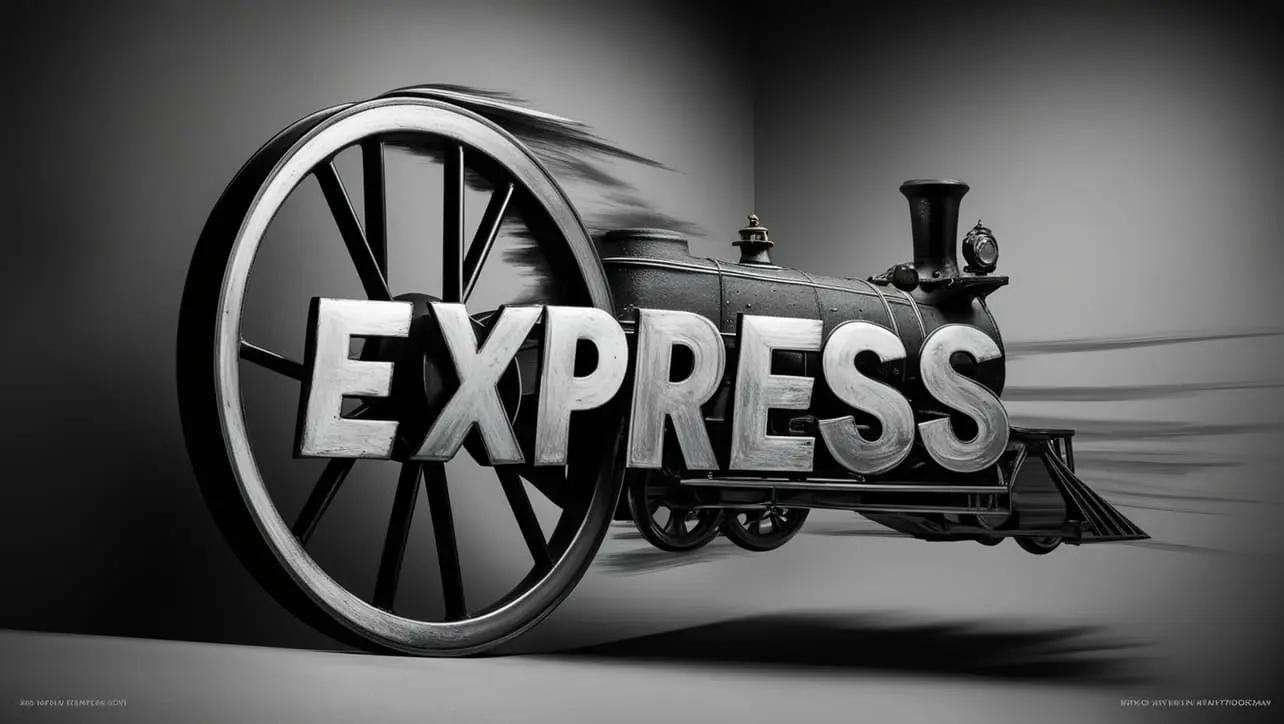
Photo Credit to CodeToFun
🙋 Introduction
The express()
function is at the core of Express.js, representing the starting point for creating an instance of the Express application. This page delves into the specifics of the express()
function, offering insights into its usage, features, and key practices.
🧐 What is the express() Function?
The express()
function is a fundamental element in Express.js that initializes and configures an Express application. It returns an instance of the application, providing a robust framework for building web servers and APIs.
The entire functionality of Express.js is built around the configuration and utilization of this central express()
function.
✨ Using express() to Create an Application
To begin an Express.js project, the journey starts with a simple call to the express()
function, creating an instance of the Express application:
const express = require('express');
const app = express();
This straightforward step sets the stage for defining routes, middleware, and handling HTTP requests within the application.
🔑 Key Features and Methods
Routing:
The
express()
function underpins the robust routing capabilities of Express.js. Explore how to utilize routing methods such as app.get(), app.post(), and others to handle various HTTP methods and establish RESTful APIs.routing.jsCopied// Define a simple route app.get('/', (req, res) => { res.send('Hello, Express!'); }); // Handling POST request app.post('/api/data', (req, res) => { // Process and handle the POST request res.json({ message: 'Data received successfully' }); });
Middleware:
Middleware functions, crucial for processing incoming requests, are seamlessly integrated using the app.use() method. This function is a key feature provided by the
express()
function, enabling the incorporation of middleware for tasks like authentication, logging, and error handling.middleware.jsCopied// Logger middleware const logger = (req, res, next) => { console.log(`[${new Date().toISOString()}] ${req.method} ${req.url}`); next(); }; // Apply logger middleware to all routes app.use(logger); // Handling requests after middleware app.get('/example', (req, res) => { res.send('Example route'); });
Configuration:
The
express()
function facilitates the configuration of various aspects within the application. Learn to use app.set() and app.get() to control the behavior and appearance of your Express.js app.configuration.jsCopied// Set the view engine to EJS app.set('view engine', 'ejs'); // Set the views directory app.set('views', path.join(__dirname, 'views'));
🚀 Advanced Features
express.Router():
Explore modular routing with express.Router(). This function allows the creation of modular, mountable route handlers, enhancing the organization and scalability of your Express.js application.
express-router.jsCopied// Create a router instance const router = express.Router(); // Define routes within the router router.get('/', (req, res) => { res.send('Router Home'); }); // Mount the router at a specific path app.use('/api', router);
express.static():
Serve static files efficiently using express.static(). This middleware function simplifies the delivery of static assets such as images, stylesheets, and scripts to clients.
express-static.jsCopied// Serve static files from the 'public' directory app.use(express.static('public'));
🎉 Conclusion
In conclusion, the express()
function serves as the bedrock of Express.js, initiating and configuring the web framework. This overview has focused on its usage, features, and key functions, providing you with a foundational understanding to harness the power of the express()
function in your Express.js applications.
👨💻 Join our Community:
Author
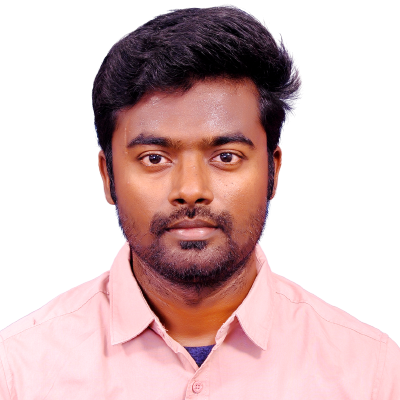
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express.js express()), please comment here. I will help you immediately.