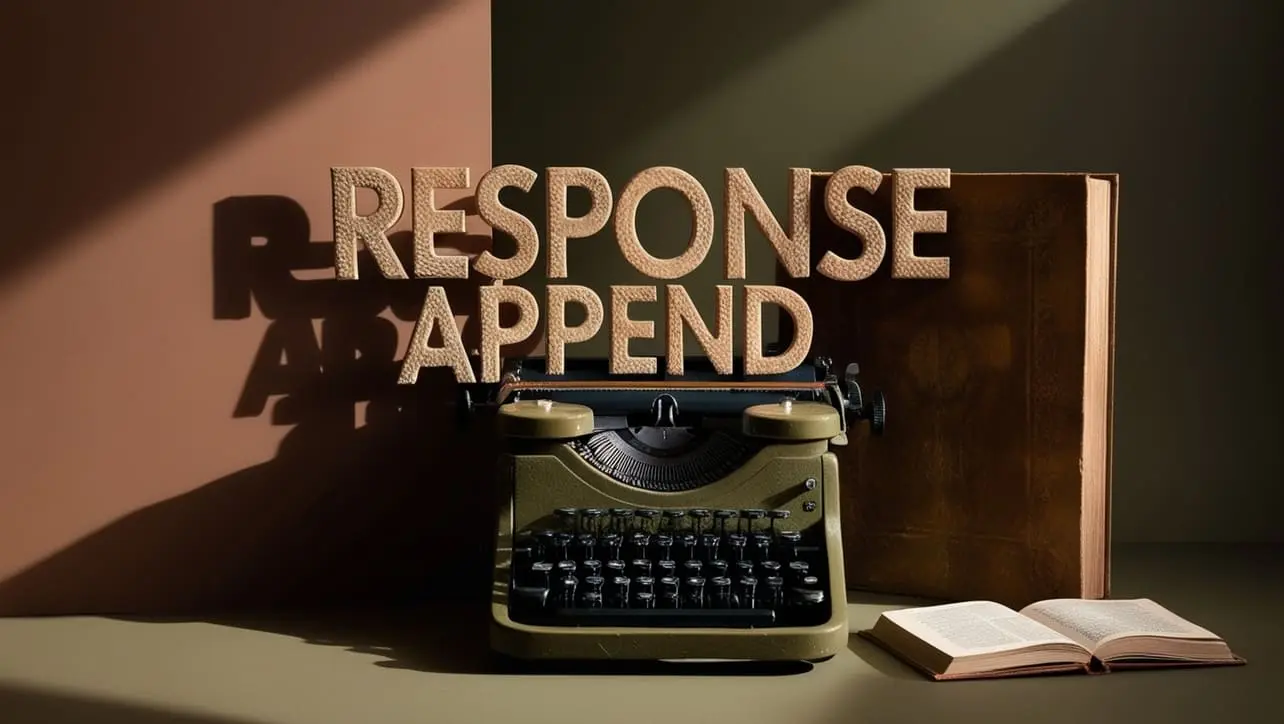
Express app.router Property
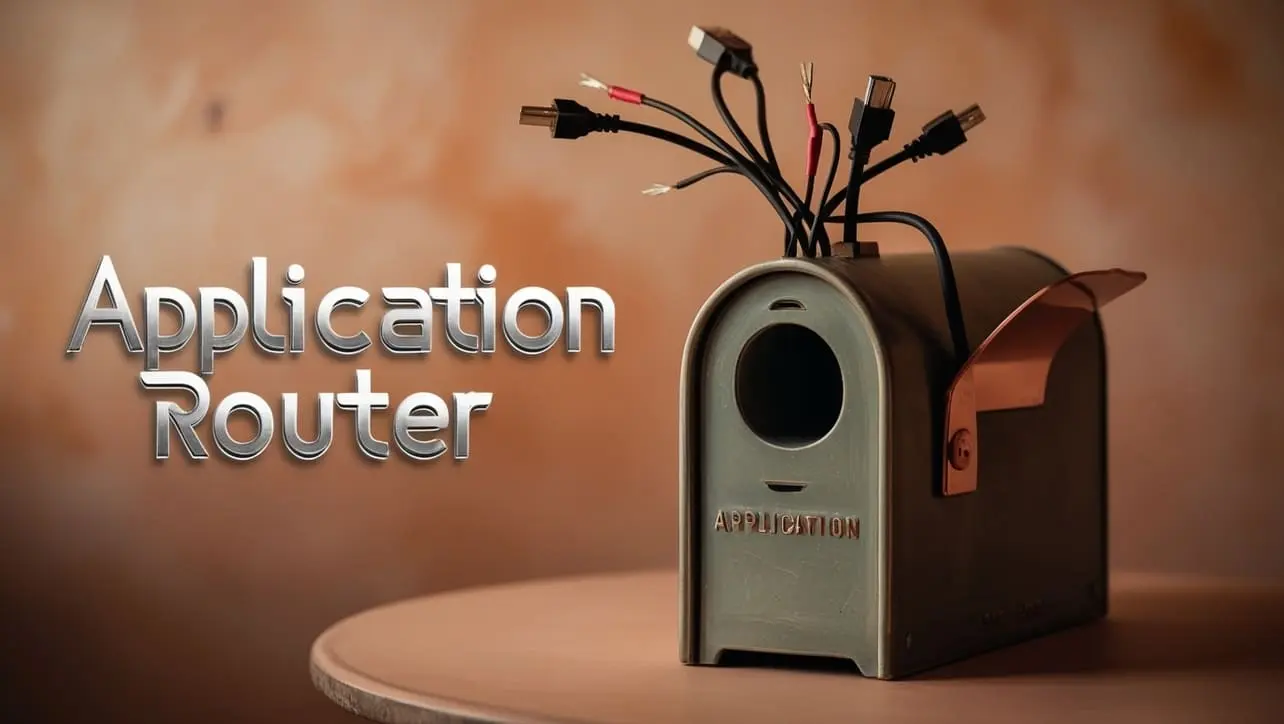
Photo Credit to CodeToFun
🙋 Introduction
Express.js, a robust Node.js web application framework, introduces the app.router
property, an in-built instance of the router. This dynamic feature is created lazily on first access, providing developers with a versatile tool for defining middleware and HTTP method routes.
In this guide, we'll explore the syntax, capabilities, and practical examples of utilizing the app.router
property in your Express.js applications.
💡 Syntax
The app.router
property is accessed as follows:
const express = require('express');
const app = express();
const router = app.router;
Once accessed, the router instance can be enhanced with middleware and HTTP method routes, just like the main application.
❓ How app.router Works
The app.router
property represents the application's in-built router. It is created lazily, which means it is instantiated on first access. This router instance allows developers to modularize route handling and middleware, promoting a cleaner and more organized code structure.
const express = require('express');
const app = express();
const router = app.router;
router.get('/', (req, res) => {
res.send('Hello, World!');
});
app.use(router); // Mount the router on the main application
app.listen(3000);
In this example, the router instance is created lazily and handles the GET request for the root route.
📚 Use Cases
Modularizing Routes:
Use
app.router
to modularize routes, keeping your codebase organized and maintainable.modularizing-routes.jsCopiedconst express = require('express'); const app = express(); const router = app.router; router.get('/home', (req, res) => { res.send('Welcome to the home page!'); }); router.get('/about', (req, res) => { res.send('Learn more about us on the about page.'); }); app.use(router); // Mount the router on the main application app.listen(3000);
Applying Middleware:
Leverage
app.router
to apply middleware selectively to specific routes within your application.applying-middleware.jsCopiedconst express = require('express'); const app = express(); const router = app.router; router.use((req, res, next) => { console.log('Middleware applied to router'); next(); }); router.get('/protected', (req, res) => { res.send('Protected route, middleware executed.'); }); app.use(router); // Mount the router on the main application app.listen(3000);
🏆 Best Practices
Lazy Initialization:
Take advantage of the lazy initialization of
app.router
. Access it when needed to ensure efficient resource utilization.lazy-initialization.jsCopiedconst express = require('express'); const app = express(); // Access app.router lazily const router = app.router; router.get('/', (req, res) => { res.send('Lazy initialization at its best!'); }); app.use(router); app.listen(3000);
🎉 Conclusion
The app.router
property in Express.js provides a powerful means to organize and modularize your route handling. By taking advantage of this in-built router, you can create cleaner and more maintainable Express.js applications.
Now, armed with knowledge about app.router
, go ahead and elevate your Express.js projects with efficient route management!
👨💻 Join our Community:
Author
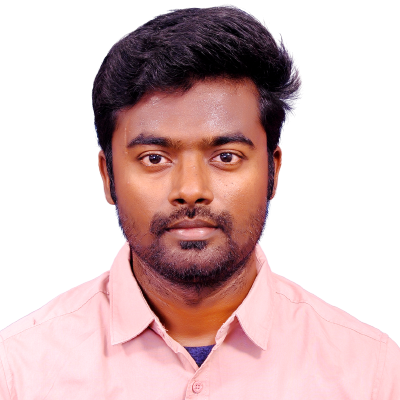
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express app.router Property), please comment here. I will help you immediately.