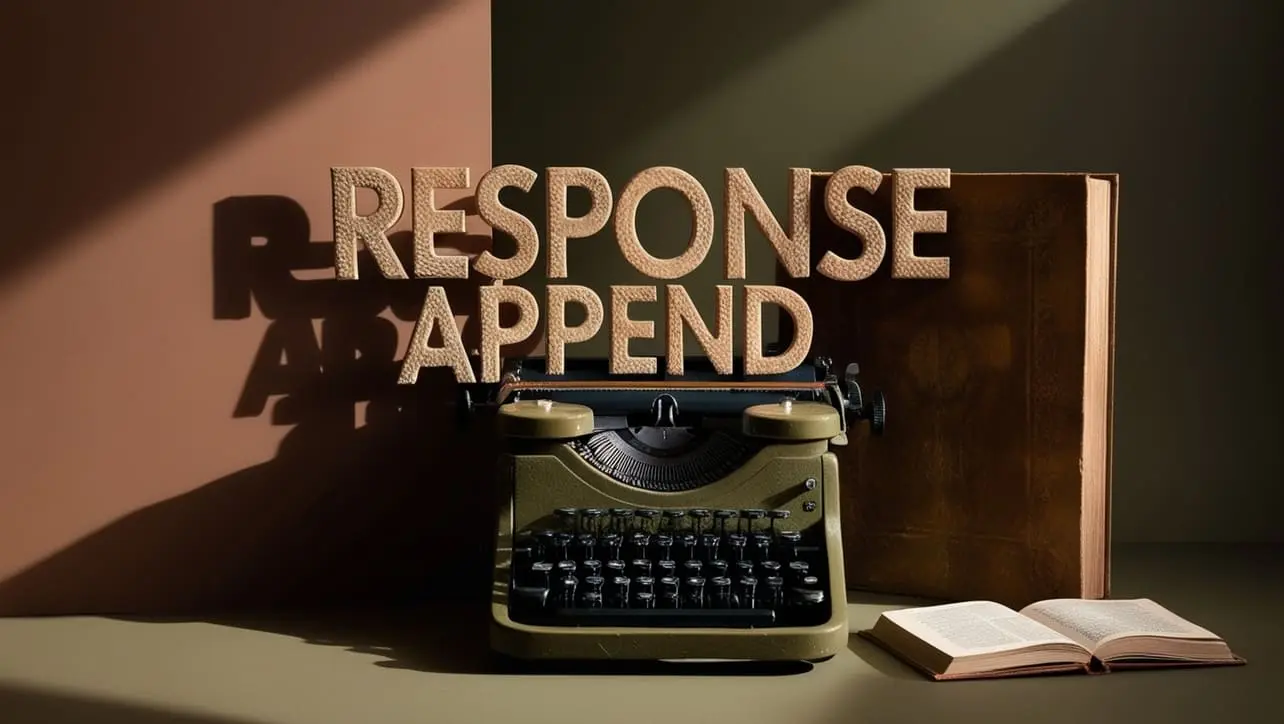
Express app.mount Event
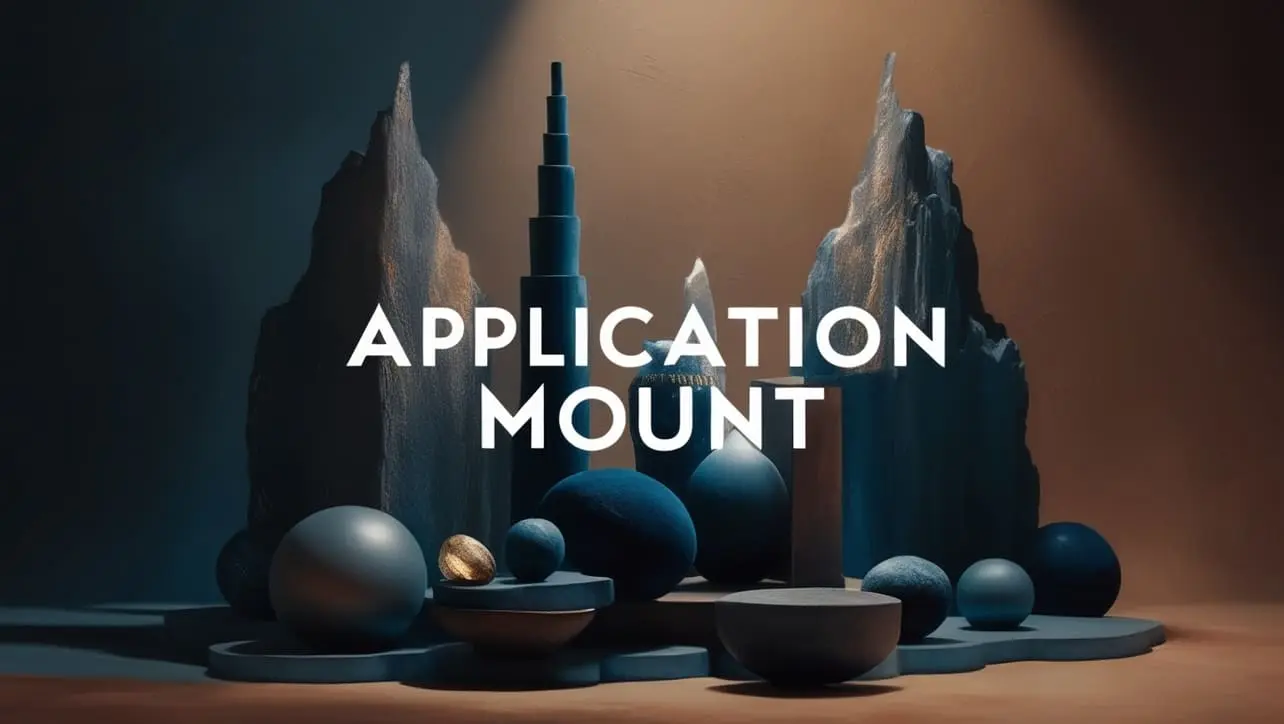
Photo Credit to CodeToFun
🙋 Introduction
Express.js, a versatile Node.js web application framework, offers a powerful event-driven architecture. One key event that plays a crucial role in Express.js is the mount
event.
In this guide, we'll delve into the mount
event, exploring its significance, syntax, and how it facilitates the mounting of middleware in your Express application.
🌐 Overview
The mount
event is emitted when a middleware is mounted on a route using the app.use() method. This event provides a way to execute logic or perform actions when a middleware is successfully mounted.
💡 Syntax
To listen for the mount
event, you can use the on() method on your Express application instance:
app.on('mount', (parent) => {
// Logic to execute when middleware is mounted
console.log('Middleware is mounted on', parent.name);
});
- parent: The parent application on which the middleware is mounted.
❓ How mount Event Works
When a middleware is mounted using app.use(), the mount
event is emitted on the parent application. This event allows you to perform actions or execute logic specific to the mounting process.
const express = require('express');
const app = express();
// Custom middleware
const myMiddleware = (req, res, next) => {
console.log('Custom middleware executed');
next();
};
// Mounting middleware with the use method
app.use('/api', myMiddleware);
// Listen for the mount event
app.on('mount', (parent) => {
console.log('Middleware is mounted on', parent.name);
});
// Your route handlers go here
const PORT = 3000;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
In this example, when the myMiddleware is mounted on the /api route, the mount
event is triggered, and the associated logic is executed.
📚 Use Cases
Logging:
Use the
mount
event to log information about the route on which a middleware is mounted.example.jsCopiedapp.on('mount', (parent) => { console.log(`Middleware mounted on route: ${parent.mountpath}`); });
Dynamic Configuration:
Dynamically configure settings based on the parent application when a middleware is mounted.
example.jsCopiedapp.on('mount', (parent) => { if (parent.name === 'subApp') { // Configure sub-app specific settings parent.set('subAppSetting', true); } });
🏆 Best Practices
Centralized Logging:
Consider using a centralized logging middleware to avoid duplicating on('mount') logic across your application.
example.jsCopied// Centralized logging middleware const logMiddleware = (parent) => { parent.on('mount', (mountedApp) => { console.log(`Middleware mounted on route: ${mountedApp.mountpath}`); }); }; // Use centralized logging middleware logMiddleware(app);
Order Matters:
Ensure that event listeners for the
mount
event are registered before the actual mounting of middleware to capture the event effectively.
🎉 Conclusion
Understanding the mount
event in Express.js enhances your ability to handle middleware mounting events efficiently. By leveraging the mount
event, you can implement dynamic configuration, centralized logging, and more, ultimately leading to more maintainable and modular Express.js applications.
Now, armed with knowledge about the mount
event, go ahead and enhance your middleware management in Express.js!
👨💻 Join our Community:
Author
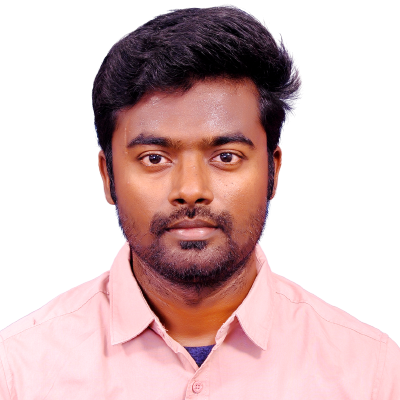
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express app.mount Event), please comment here. I will help you immediately.