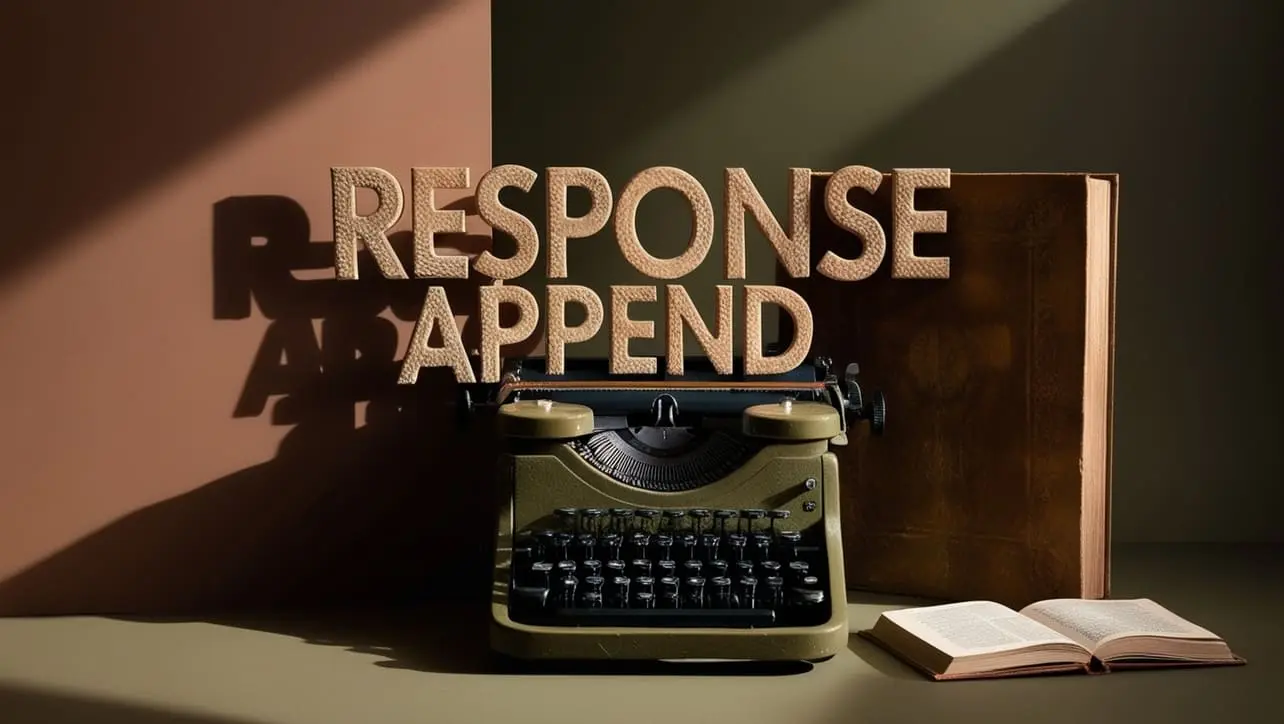
Express app.locals Property
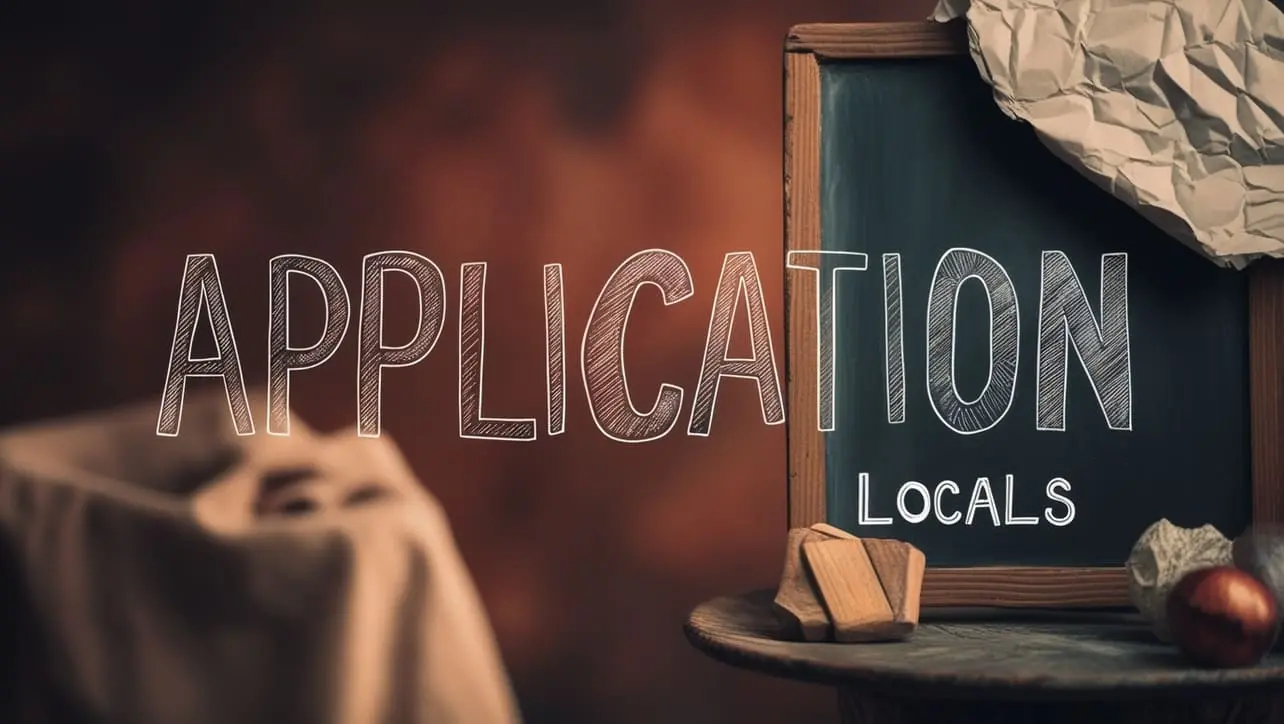
Photo Credit to CodeToFun
🙋 Introduction
Express.js, a popular Node.js web application framework, provides developers with powerful tools to build robust and scalable web applications. One such feature is the app.locals
property, which allows you to define variables accessible throughout your application's lifecycle.
In this guide, we'll explore how to use app.locals
effectively, covering its syntax, usage, and best practices.
💡 Syntax
The syntax for using app.locals
is straightforward:
app.locals.name = value;
- name: The name of the variable you want to define.
- value: The value assigned to the variable.
❓ How app.locals Works
The app.locals
property in Express.js provides a way to define application-level variables that are accessible in all middleware and route handler functions. These variables are available throughout the entire lifecycle of the application, making them ideal for storing data that needs to be shared across different parts of your application.
// Define a global variable
app.locals.siteName = 'My Express App';
// Access the global variable in a route handler
app.get('/', (req, res) => {
res.send(`Welcome to ${app.locals.siteName}`);
});
In this example, the siteName variable is defined using app.locals
and is accessible within the route handler function.
📚 Use Cases
Configuration Settings:
Use
app.locals
to store configuration settings that are used throughout your application, such as port numbers or database URLs.configuration-settings.jsCopied// Define global configuration settings app.locals.port = 3000; app.locals.databaseUrl = 'mongodb://localhost/my_database';
Shared Data:
Store shared data, such as user information or application-wide constants, using
app.locals
.shared-data.jsCopied// Define global data app.locals.users = [ { id: 1, name: 'John' }, { id: 2, name: 'Jane' }, // more users... ];
🏆 Best Practices
Limit Global Variables:
Avoid cluttering
app.locals
with too many variables. Only store data that needs to be accessed globally across your application.limit-global-variables.jsCopied// Good practice: Limit global variables to essential data app.locals.siteName = 'My Express App'; app.locals.port = 3000;
Immutable Variables:
Prefer immutable variables in
app.locals
to prevent unintended modifications.immutable-variables.jsCopied// Immutable variable app.locals.siteName = 'My Express App';
🎉 Conclusion
The app.locals
property in Express.js provides a convenient way to define and access global variables in your application. By following best practices and using app.locals
effectively, you can streamline your development process and build more maintainable Express.js applications.
Now, armed with knowledge about app.locals
, go ahead and leverage this powerful feature in your Express.js projects!
👨💻 Join our Community:
Author
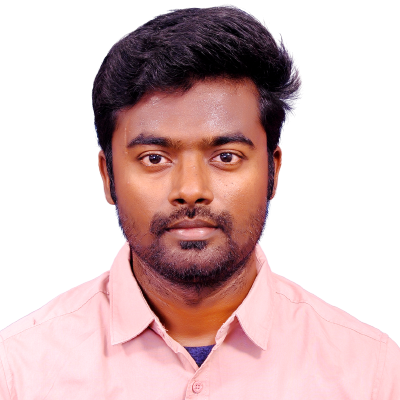
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express app.locals Property), please comment here. I will help you immediately.