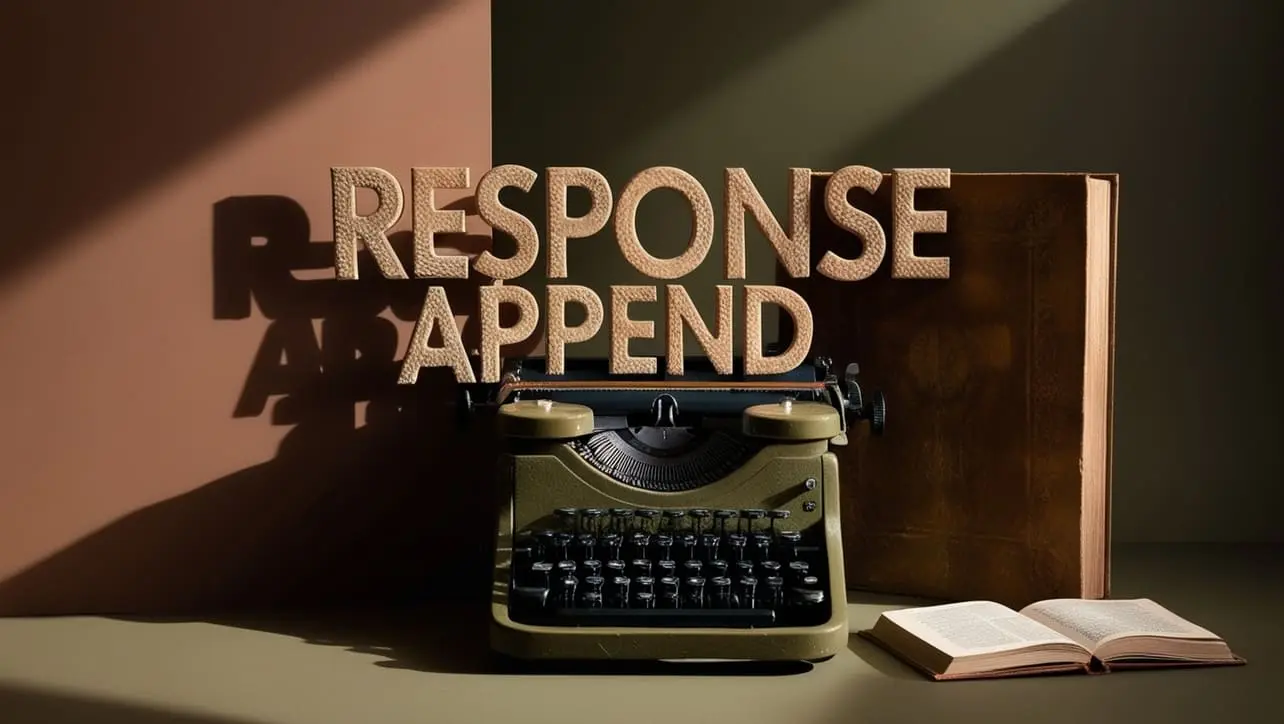
Express.js Basic
- express Intro
- express express()
- express Application
Properties
Events
Methods
- express Request
- express Response
- express Router
Express app.listen() Method
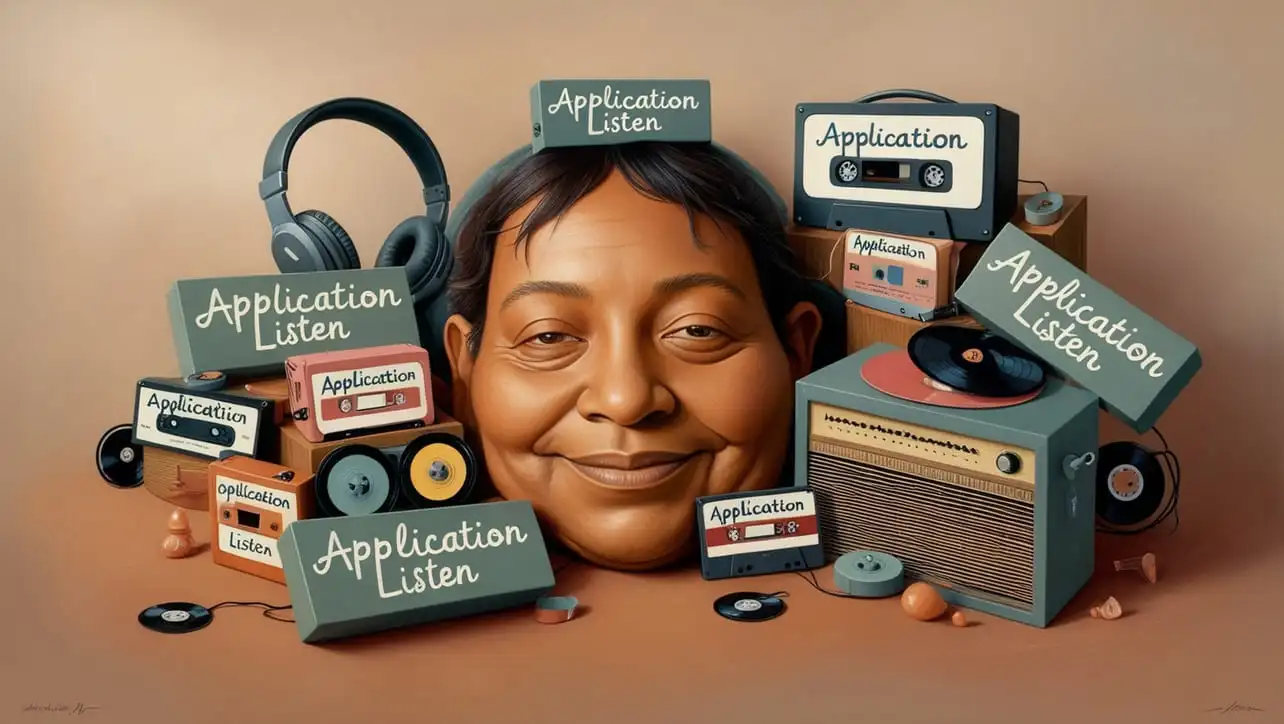
Photo Credit to CodeToFun
🙋 Introduction
Express.js provides a straightforward way to create web servers in Node.js, and the app.listen()
method is a crucial part of this process.
In this guide, we'll explore the app.listen()
method, its syntax, and best practices for starting and configuring your Express.js server.
💡 Syntax
The syntax for the app.listen()
method is simple:
const server = app.listen(port, [hostname], [backlog], [callback])
- port: The port number on which the server will listen for incoming requests.
- hostname (optional): The hostname or IP address on which the server will listen. Defaults to 'localhost'.
- backlog (optional): The maximum length of the queue of pending connections. Defaults to 511.
- callback (optional): A callback function that is executed once the server is listening.
❓ How app.listen() Works
The app.listen()
method binds and listens for connections on the specified host and port. When a client makes a request, the server processes it and sends back the appropriate response.
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('Hello, Express!');
});
const server = app.listen(port, () => {
console.log(`Server is running on http://localhost:${port}`);
});
In this example, the server is set up to listen on port 3000, and a callback logs a message once the server is running.
📚 Use Cases
Basic Server Setup:
Use
app.listen()
to start a basic server that responds to requests on a specified port.example.jsCopiedconst express = require('express'); const app = express(); const port = 3000; app.get('/', (req, res) => { res.send('Welcome to my Express server!'); }); const server = app.listen(port, () => { console.log(`Server is running on http://localhost:${port}`); });
Dynamic Port Assignment:
Dynamically assign the port based on the environment variable or use a default value.
example.jsCopiedconst express = require('express'); const app = express(); const port = process.env.PORT || 3000; app.get('/', (req, res) => { res.send('Dynamic Port Assignment'); }); const server = app.listen(port, () => { console.log(`Server is running on http://localhost:${port}`); });
🏆 Best Practices
Port Availability Check:
Check if the specified port is available before calling
app.listen()
to avoid conflicts.example.jsCopiedconst express = require('express'); const app = express(); const port = 3000; const server = app.listen(port, () => { console.log(`Server is running on http://localhost:${port}`); }); server.on('error', (error) => { if (error.code === 'EADDRINUSE') { console.error(`Port ${port} is already in use`); } });
Separate Server Setup:
Separate the server setup logic from the
app.listen()
call for better modularity.example.jsCopiedconst express = require('express'); const app = express(); const port = 3000; app.get('/', (req, res) => { res.send('Separate Server Setup'); }); const startServer = () => { const server = app.listen(port, () => { console.log(`Server is running on http://localhost:${port}`); }); }; startServer();
Graceful Shutdown:
Implement a graceful shutdown mechanism to handle server termination.
example.jsCopiedconst express = require('express'); const app = express(); const port = 3000; app.get('/', (req, res) => { res.send('Graceful Shutdown'); }); const server = app.listen(port, () => { console.log(`Server is running on http://localhost:${port}`); }); // Graceful shutdown example process.on('SIGTERM', () => { server.close(() => { console.log('Server gracefully terminated'); process.exit(0); }); });
🎉 Conclusion
The app.listen()
method in Express.js is fundamental for starting your server and listening for incoming requests. By understanding its syntax, examples, and best practices, you can ensure a robust and well-configured Express.js server for your web applications.
Now, go ahead and set up your Express.js server with confidence!
👨💻 Join our Community:
Author
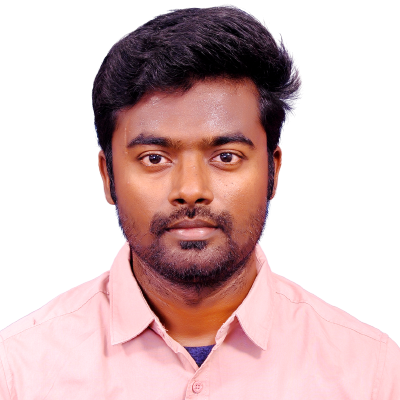
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express app.listen() Method), please comment here. I will help you immediately.