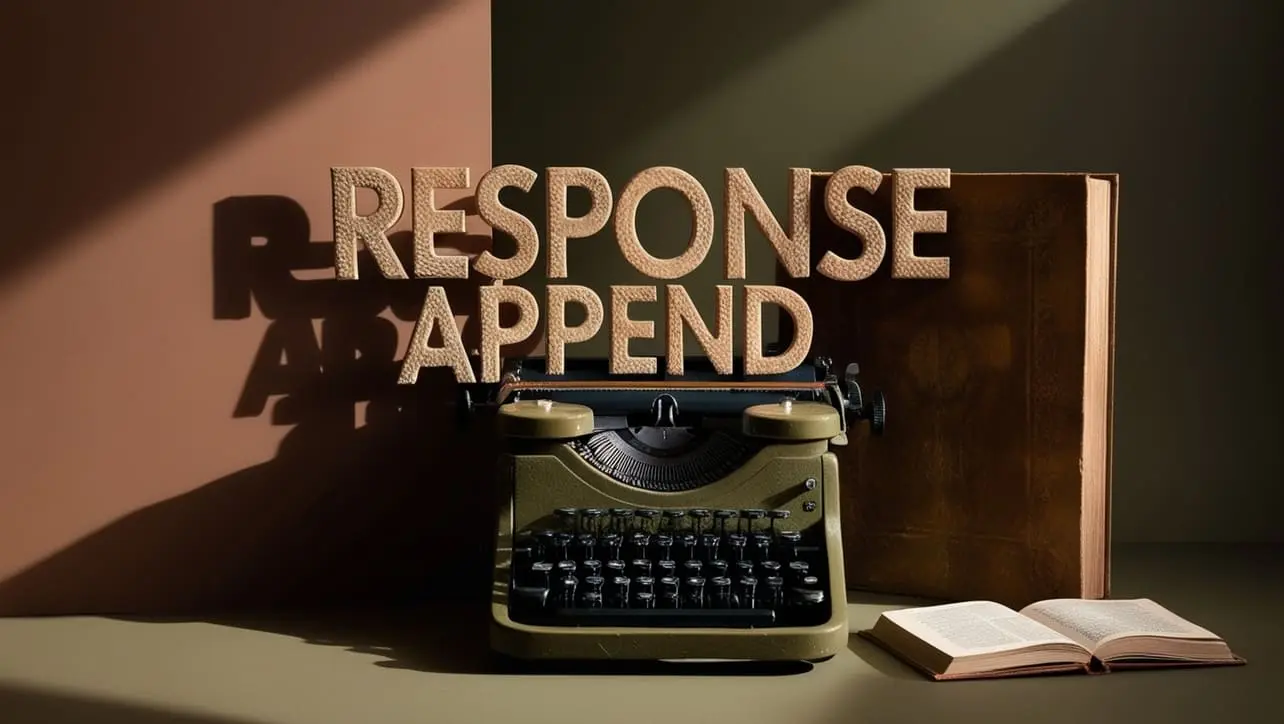
Express app.delete() Method
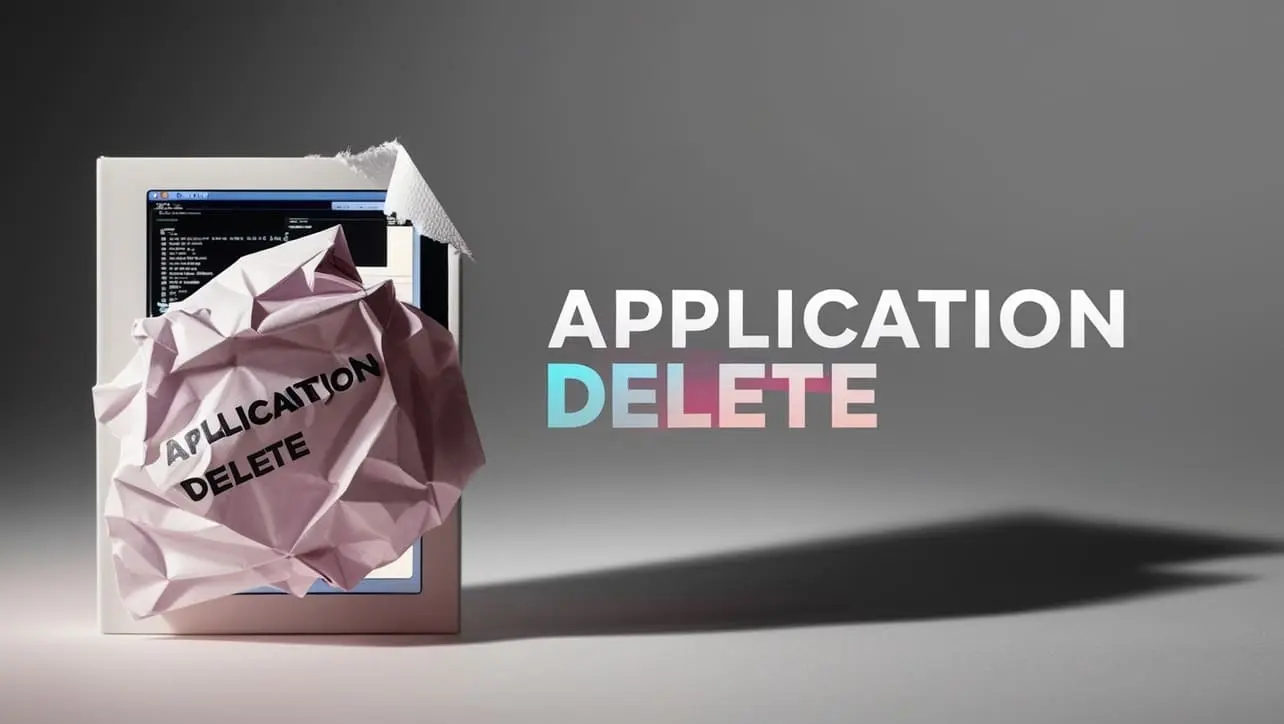
Photo Credit to CodeToFun
🙋 Introduction
In the realm of web development, handling different HTTP methods is essential for building robust applications.
Express.js, a popular Node.js web application framework, provides specific methods for various HTTP verbs.
This guide focuses on the app.delete()
method, which is tailored for handling HTTP DELETE requests.
💡 Syntax
The syntax for the app.delete()
method is simple and mirrors other route methods in Express.js:
app.delete(path, callback)
- path: A string representing the route or path for which the DELETE request is being handled.
- callback: The route handler function that processes the DELETE request.
❓ How app.delete() Works
With app.delete()
, you can define route-specific logic to handle HTTP DELETE requests. This method is useful when you need to perform actions such as deleting resources or data on the server.
app.delete('/users/:id', (req, res) => {
const userId = req.params.id;
// Logic to delete user with userId
res.send(`Deleted user with ID ${userId}`);
});
In this example, the route '/users/:id' captures DELETE requests and executes logic to delete a user with the specified ID.
📚 Use Cases
Resource Deletion:
Use
app.delete()
to handle requests for deleting specific resources, such as posts or users.example.jsCopiedapp.delete('/posts/:postId', (req, res) => { const postId = req.params.postId; // Logic to delete post with postId res.send(`Deleted post with ID ${postId}`); });
Data Cleanup:
Leverage
app.delete()
for routes dedicated to data cleanup tasks, ensuring a clean and organized server environment.example.jsCopiedapp.delete('/cleanup', (req, res) => { // Logic to perform data cleanup tasks res.send('Data cleanup successful'); });
🏆 Best Practices
Use RESTful Routes:
Follow RESTful principles when designing your routes. Utilize
app.delete()
for routes that involve resource deletion.example.jsCopied// RESTful route for deleting a user app.delete('/users/:id', (req, res) => { const userId = req.params.id; // Logic to delete user with userId res.send(`Deleted user with ID ${userId}`); });
Implement Robust Error Handling:
Implement robust error handling within your
app.delete()
route handlers to handle potential issues gracefully.example.jsCopiedapp.delete('/posts/:postId', (req, res) => { const postId = req.params.postId; // Simulate an error during deletion if (Math.random() < 0.5) { res.status(500).send('Internal Server Error'); } else { // Logic to delete post with postId res.send(`Deleted post with ID ${postId}`); } });
Middleware Integration:
Combine
app.delete()
with middleware functions for tasks like authentication or authorization before processing the delete operation.example.jsCopied// Middleware for user authentication const authenticateUser = (req, res, next) => { // Implement your authentication logic here if (req.isAuthenticated()) { return next(); } else { res.status(401).send('Unauthorized'); } }; // Apply authentication middleware to the delete route app.delete('/users/:id', authenticateUser, (req, res) => { const userId = req.params.id; // Logic to delete user with userId res.send(`Deleted user with ID ${userId}`); });
🎉 Conclusion
The app.delete()
method in Express.js empowers you to handle HTTP DELETE requests effectively. Whether you're building RESTful APIs or handling resource deletions, understanding how to leverage app.delete()
is crucial for developing scalable and maintainable applications.
Now, armed with knowledge about the app.delete()
method, go ahead and enhance your Express.js projects with robust handling of DELETE requests!
👨💻 Join our Community:
Author
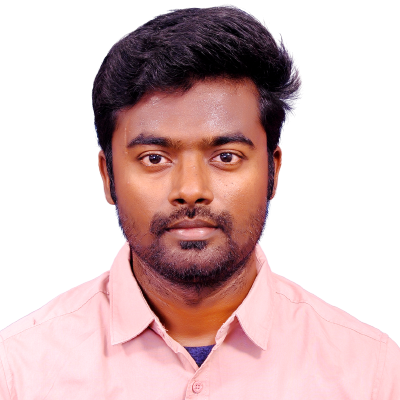
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express app.delete() Method), please comment here. I will help you immediately.