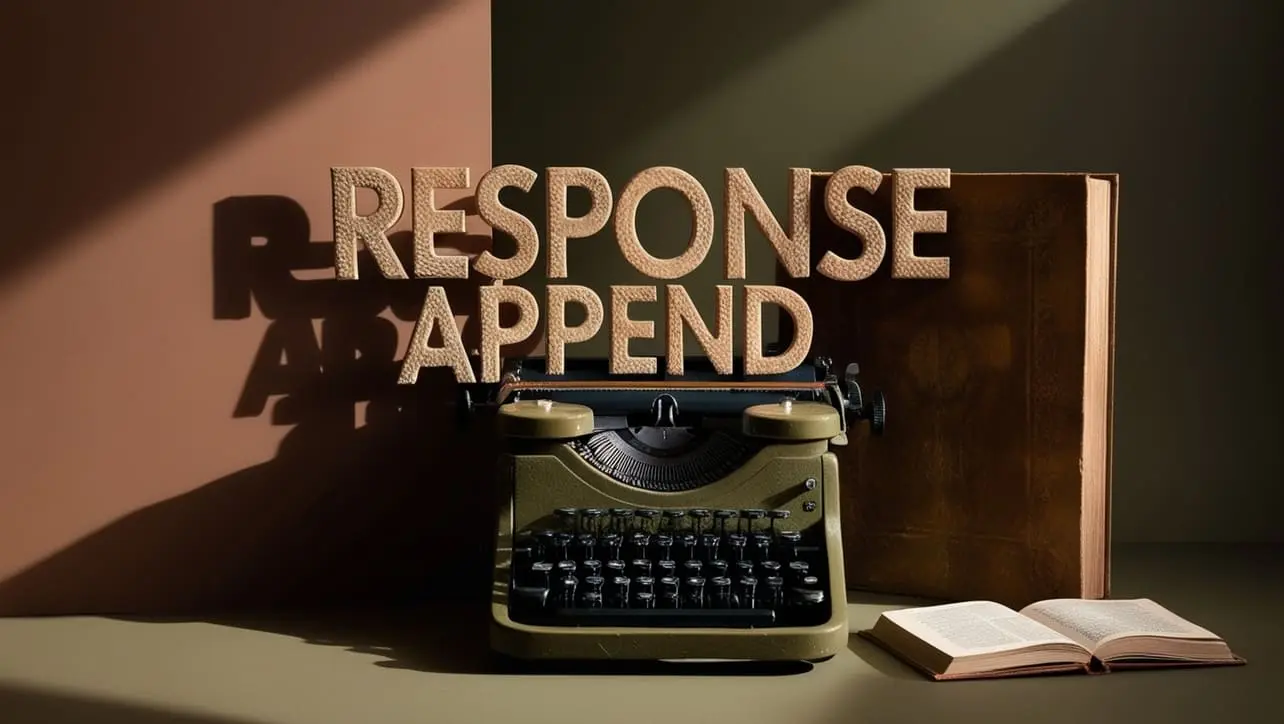
Express.js Basic
- express Intro
- express express()
- express Application
Properties
Events
Methods
- express Request
- express Response
- express Router
Express.js Application
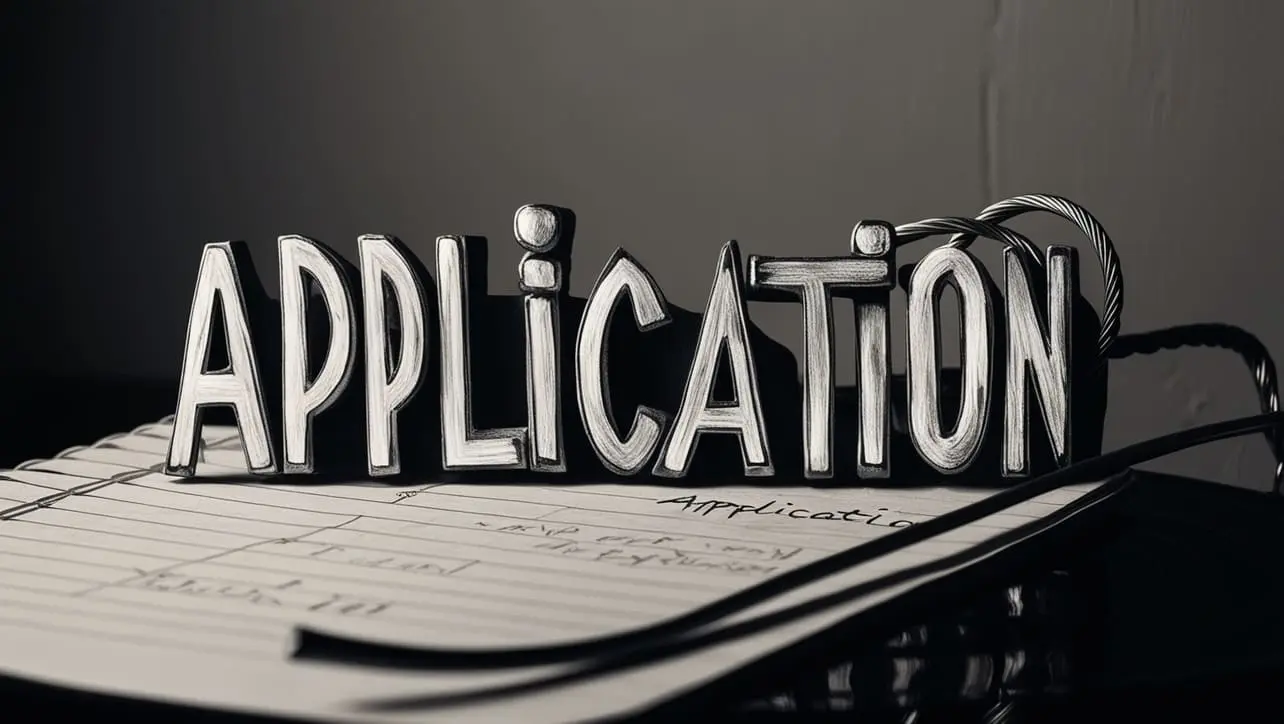
Photo Credit to CodeToFun
🙋 Introduction
In the world of Node.js web frameworks, Express.js stands out as a powerful and flexible choice for building web applications.
The core of any Express.js project is the app
object, which is central to defining routes, middleware, and configuring various aspects of your web application.
This page serves as a comprehensive guide to the app
object, providing insights into its functionalities and best practices.
🧐 What is the App Object?
The app
object in Express.js is a crucial component that represents your web application. It is created by calling the top-level express() function exported by the Express module. The app
object becomes the hub for managing routes, middleware, and configurations, allowing you to structure and control the behavior of your web application.
🏁 Initializing the app Object
To kickstart your Express.js journey, you begin by creating the app
object:
const express = require('express');
const app = express();
This single line of code initializes your Express application, setting the stage for defining routes, middleware, and handling HTTP requests.
🧠 Anatomy of the app Object
Routing:
At the heart of Express.js is its robust routing system facilitated by the
app
object. Routes define how your application responds to client requests. Learn how to leverage app.get(), app.post(), and other routing methods to handle various HTTP methods and create RESTful APIs.routing.jsCopied// Define a simple route app.get('/', (req, res) => { res.send('Hello, Express!'); }); // Handling POST request app.post('/api/data', (req, res) => { // Process and handle the POST request res.json({ message: 'Data received successfully' }); });
Middleware:
Express.js middleware functions play a crucial role in processing incoming requests before they reach your routes. Explore how to use app.use() to integrate middleware, enabling features such as authentication, logging, and error handling seamlessly.
middleware.jsCopied// Logger middleware const logger = (req, res, next) => { console.log(`[${new Date().toISOString()}] ${req.method} ${req.url}`); next(); }; // Apply logger middleware to all routes app.use(logger); // Handling requests after middleware app.get('/example', (req, res) => { res.send('Example route'); });
Configuration:
The
app
object allows you to configure various aspects of your application, from setting the port to managing environment-specific settings. Delve into the world of app.set() and app.get() to control the behavior and appearance of your Express.js app.configuration.jsCopied// Set the port for the Express app app.set('port', process.env.PORT || 3000); // Get the configured port const port = app.get('port'); // Start the server on the configured port app.listen(port, () => { console.log(`Server is running on port ${port}`); });
🔑 Key Features and Methods
app.listen():
The app.listen() method is your gateway to starting the Express.js server, binding it to a specific port. Learn how to utilize this function to make your application accessible over the web.
app-listen.jsCopied// Set the port for the Express app const port = 3000; // Start the server on the specified port app.listen(port, () => { console.log(`Server is running on port ${port}`); });
app.use():
Discover the power of app.use() in integrating middleware functions. This method is instrumental in applying middleware across your entire application or specific routes, ensuring consistent behavior and enhanced functionality.
app-use.jsCopied// Middleware to parse JSON requests app.use(express.json()); // Middleware to log request details app.use((req, res, next) => { console.log(`Received ${req.method} request at ${req.url}`); next(); });
app.set():
Configuration is key to tailoring your Express.js
app
to specific needs. Explore the app.set() method to define global variables, influencing the behavior of your application across different environments.app-set.jsCopied// Set the view engine to EJS app.set('view engine', 'ejs'); // Set the views directory app.set('views', path.join(__dirname, 'views'));
🚀 Advanced Features
View Engines:
Enhance the presentation layer of your Express.js
app
with view engines like EJS or Handlebars. Learn how to configure and utilize these engines to render dynamic content and create a seamless user experience.view-engines.jsCopied// Set the view engine to EJS app.set('view engine', 'ejs'); // Render a dynamic view app.get('/dynamic', (req, res) => { const data = { message: 'Hello, Express!' }; res.render('dynamic-view', { data }); });
Error Handling:
Effectively handling errors is a critical aspect of web development. Discover how to implement error-handling middleware using the app.use() function, ensuring your application gracefully manages unexpected issues.
error-handling.jsCopied// Error-handling middleware app.use((err, req, res, next) => { console.error(err.stack); res.status(500).send('Something went wrong!'); });
📝 Example
Let's illustrate the basic structure of an Express.js app:
const express = require('express');
const app = express();
const port = 3000;
// Define a simple route
app.get('/', (req, res) => {
res.send('Hello, Express!');
});
// Start the server
app.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
In this example, we create a minimal Express.js app, define a route, and start the server. This serves as a foundation for building more complex applications using the app
object.
🎉 Conclusion
In conclusion, the app
object is the backbone of your Express.js application, providing a structured and powerful framework for building robust web solutions. This overview has shed light on the various facets of the app
object, equipping you with the knowledge to architect and manage Express.js applications effectively.
👨💻 Join our Community:
Author
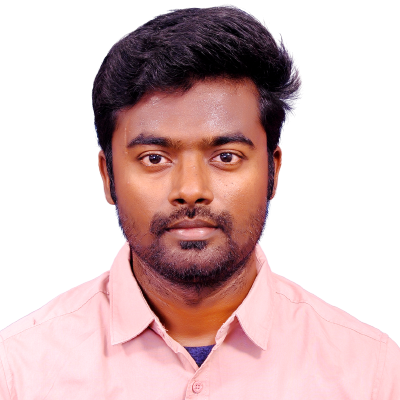
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express.js Application), please comment here. I will help you immediately.