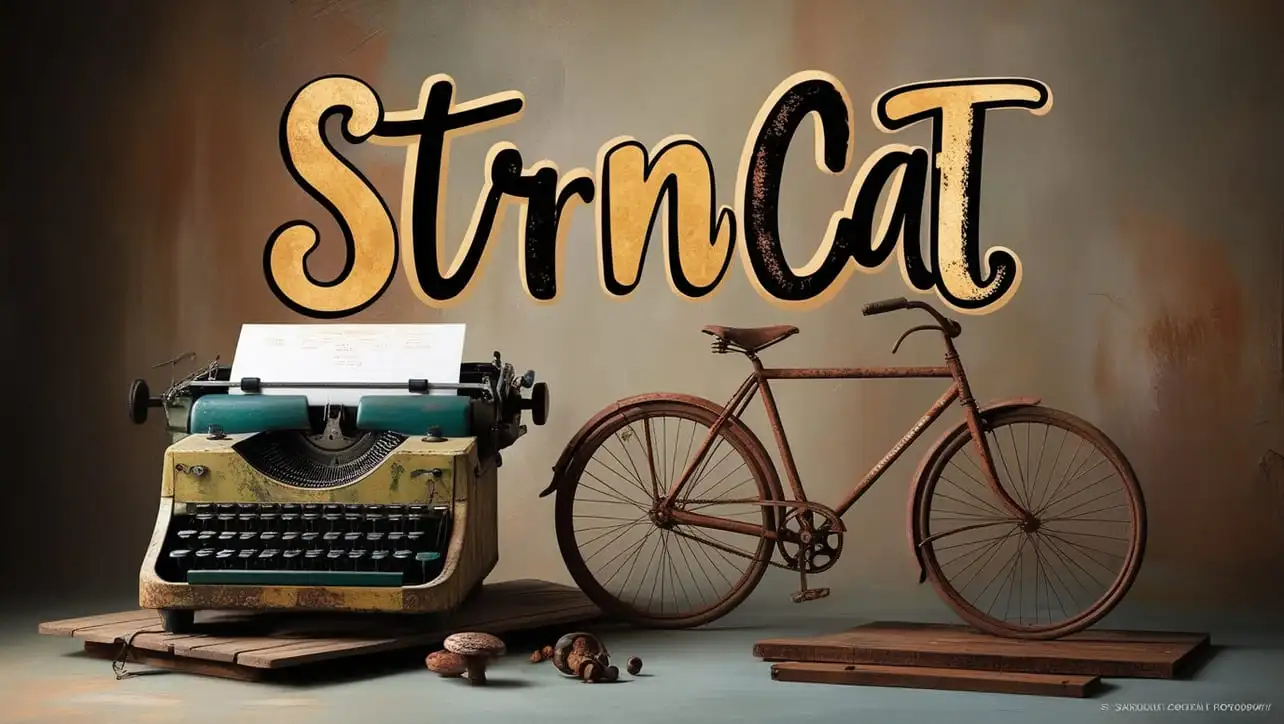
C++ String strrchr() Function
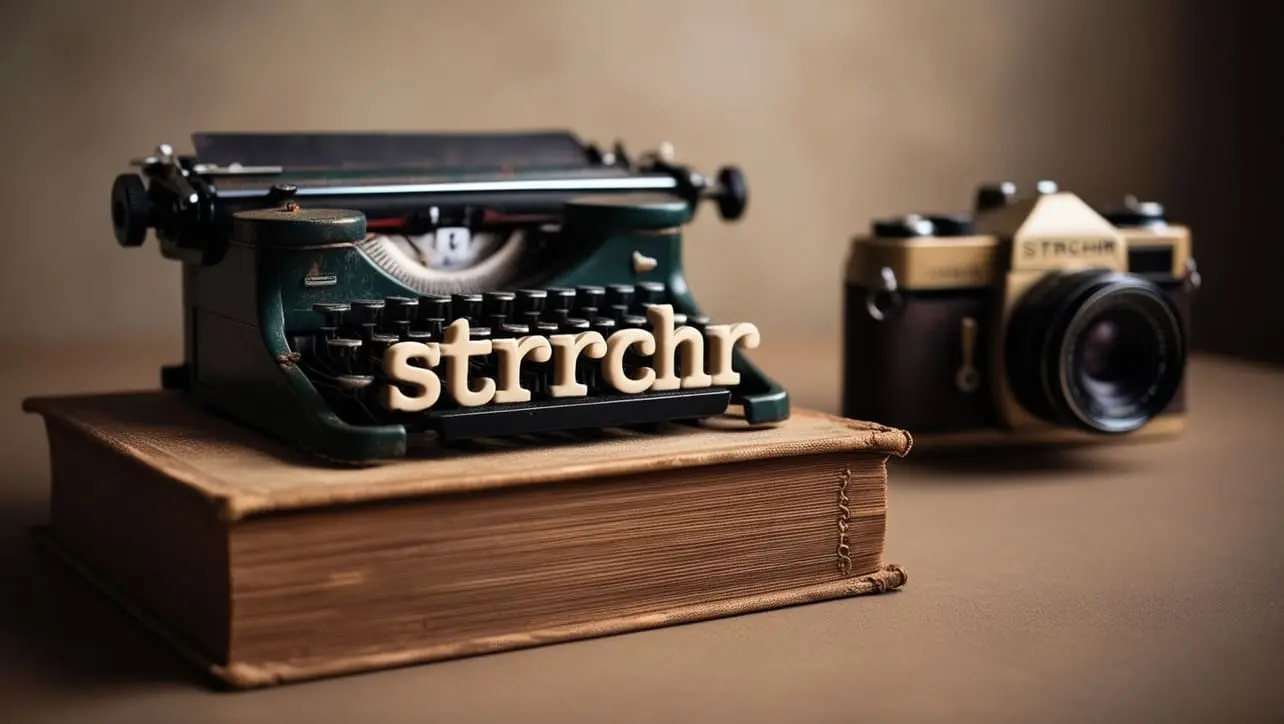
Photo Credit to CodeToFun
đ Introduction
In C++ programming, manipulating strings is a common and fundamental task.
The strrchr()
function is a part of the C library and is used for searching a character in a string. Unlike other string functions, strrchr()
searches for the last occurrence of a character in a given string.
In this tutorial, we'll explore the usage and functionality of the strrchr()
function in C++.
đĄ Syntax
The signature of the strrchr()
function is as follows:
char* strrchr(const char* str, int character);
This function takes a C-style string str and a character character and returns a pointer to the last occurrence of the character in the string. If the character is not found, it returns a null pointer.
đ Example
Let's delve into an example to illustrate how the strrchr()
function works.
#include <iostream>
#include <cstring>
int main() {
const char * sentence = "Hello, C++ programming!";
// Find the last occurrence of 'o'
const char * lastO = strrchr(sentence, 'o');
if (lastO != nullptr) {
std::cout << "Last occurrence of 'o' found at position: " << lastO - sentence << std::endl;
} else {
std::cout << "'o' not found in the string." << std::endl;
}
return 0;
}
đģ Output
Last occurrence of 'o' found at position: 13
đ§ How the Program Works
In this example, the strrchr()
function is used to find the last occurrence of the character 'o' in the string "Hello, C++ programming!" and prints the position.
âŠī¸ Return Value
The strrchr()
function returns a pointer to the last occurrence of the specified character in the string. If the character is not found, it returns a null pointer (nullptr).
đ Common Use Cases
The strrchr()
function is useful when you need to find the last occurrence of a specific character in a string. It can be applied in scenarios such as parsing file paths, extracting file extensions, or manipulating text data.
đ Notes
- The
strrchr()
function operates on C-style strings, and the character argument is specified as an integer. Ensure that the character is cast to an integer if it is specified as a character literal.
đĸ Optimization
The strrchr()
function is optimized for searching the last occurrence of a character in a string. However, for performance-critical applications, you may want to consider alternative approaches or additional checks based on specific requirements.
đ Conclusion
The strrchr()
function in C++ is a valuable tool for searching the last occurrence of a character in a string. It provides a standardized and efficient way to perform this task, contributing to the versatility of string manipulation in C++.
Feel free to experiment with different strings and characters to explore the behavior of the strrchr()
function in various scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
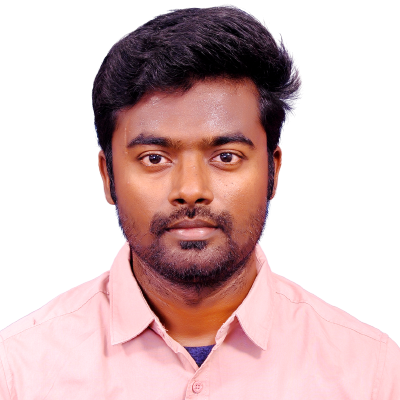
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C++ String strrchr() Function), please comment here. I will help you immediately.