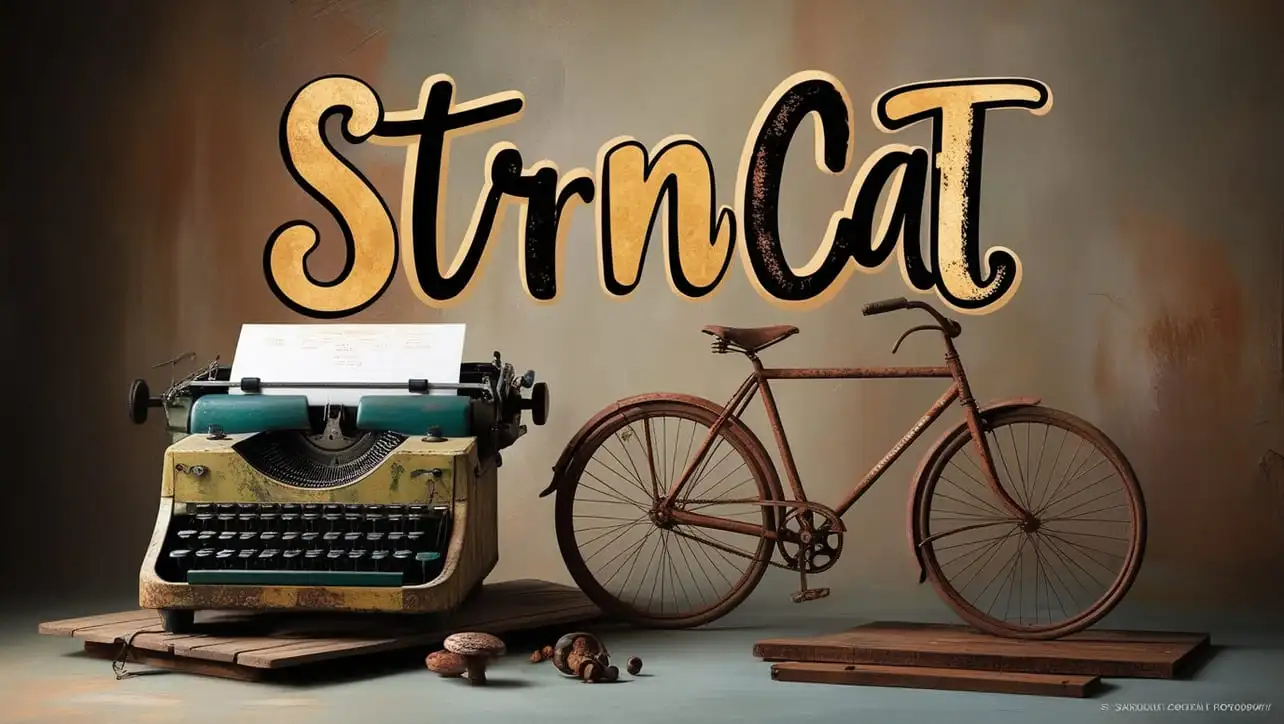
C++ String strlen() Function
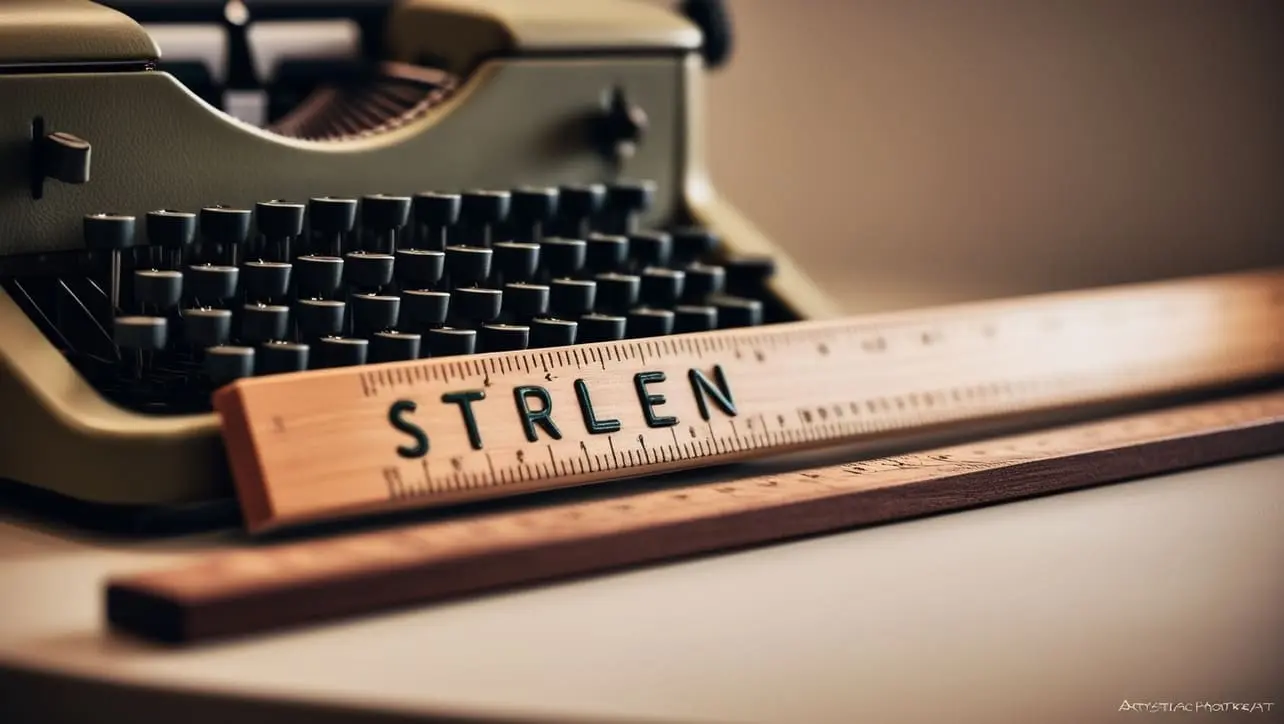
Photo Credit to CodeToFun
đ Introduction
In C++ programming, strings are commonly used for storing and manipulating text.
The strlen()
function is a fundamental part of the C++ Standard Library, and it allows you to determine the length of a C-style string.
In this tutorial, we'll explore the usage and functionality of the strlen()
function.
đĄ Syntax
The signature of the strlen()
function is as follows:
size_t strlen(const char *str);
This function takes a pointer to a null-terminated C-style string (const char* str) and returns the length of the string.
đ Example
Let's delve into an example to illustrate how the strlen()
function works.
#include <iostream>
#include <cstring>
int main() {
const char * str = "Hello, C++!";
// Get the length of the string
size_t length = strlen(str);
// Output the result
std::cout << "Length of the string: " << length << std::endl;
return 0;
}
đģ Output
Length of the string: 11
đ§ How the Program Works
In this example, the strlen()
function is used to determine the length of the C-style string "Hello, C++!" and prints the result.
âŠī¸ Return Value
The strlen()
function returns the number of characters in the C-style string str before the null terminator.
đ Common Use Cases
The strlen()
function is useful when you need to obtain the length of a C-style string dynamically during runtime. It's commonly used in scenarios where you want to iterate through the characters of a string or allocate memory based on the string's length.
đ Notes
- Ensure that the C-style string passed to
strlen()
is null-terminated. Not doing so can result in undefined behavior. - For C++ std::string objects, you can use the size() member function or length() member function to get the length directly.
đĸ Optimization
The strlen()
function is optimized for efficiency, but for performance-critical applications, consider caching the length of a string if it is used multiple times, rather than repeatedly calling strlen()
.
đ Conclusion
The strlen()
function in C++ is a straightforward yet powerful tool for obtaining the length of C-style strings. It plays a crucial role in string manipulation and memory allocation tasks.
Feel free to experiment with different strings and explore the behavior of the strlen()
function in various scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
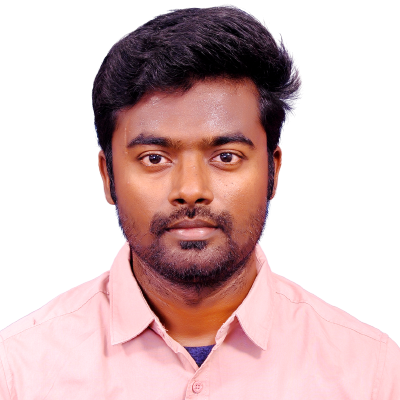
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C++ String strlen() Function), please comment here. I will help you immediately.