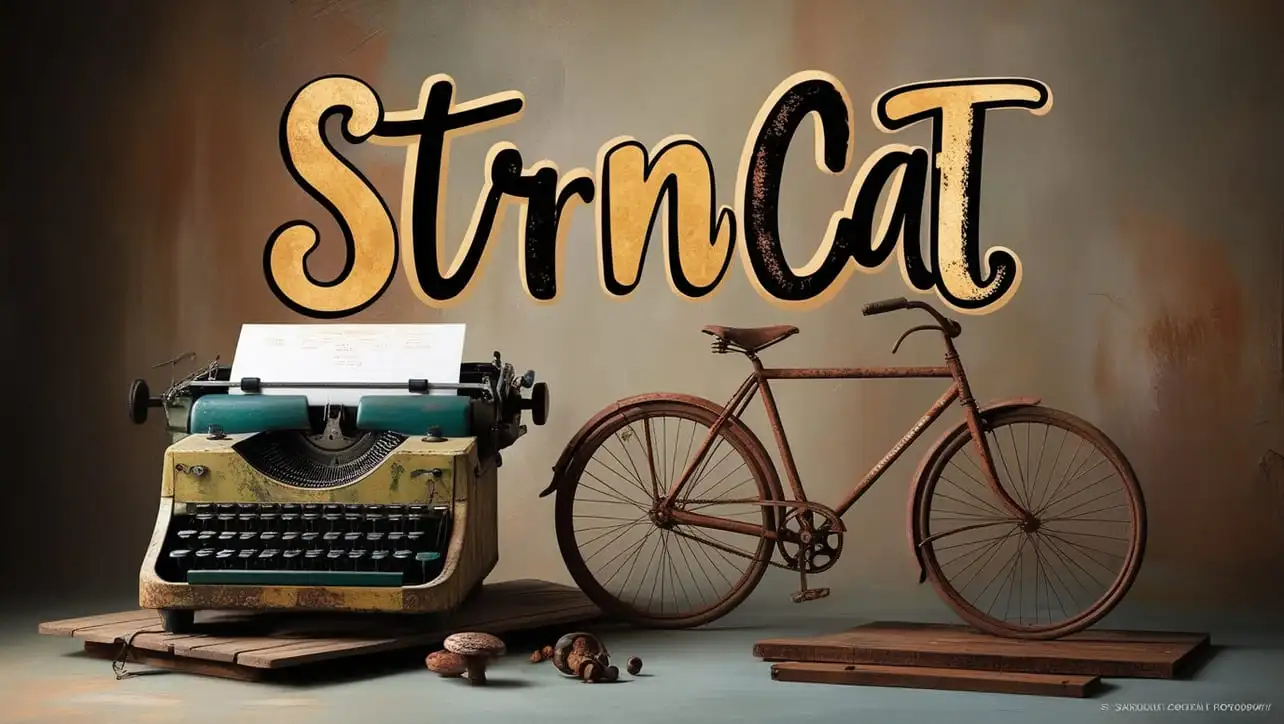
C++ String strerror() Function
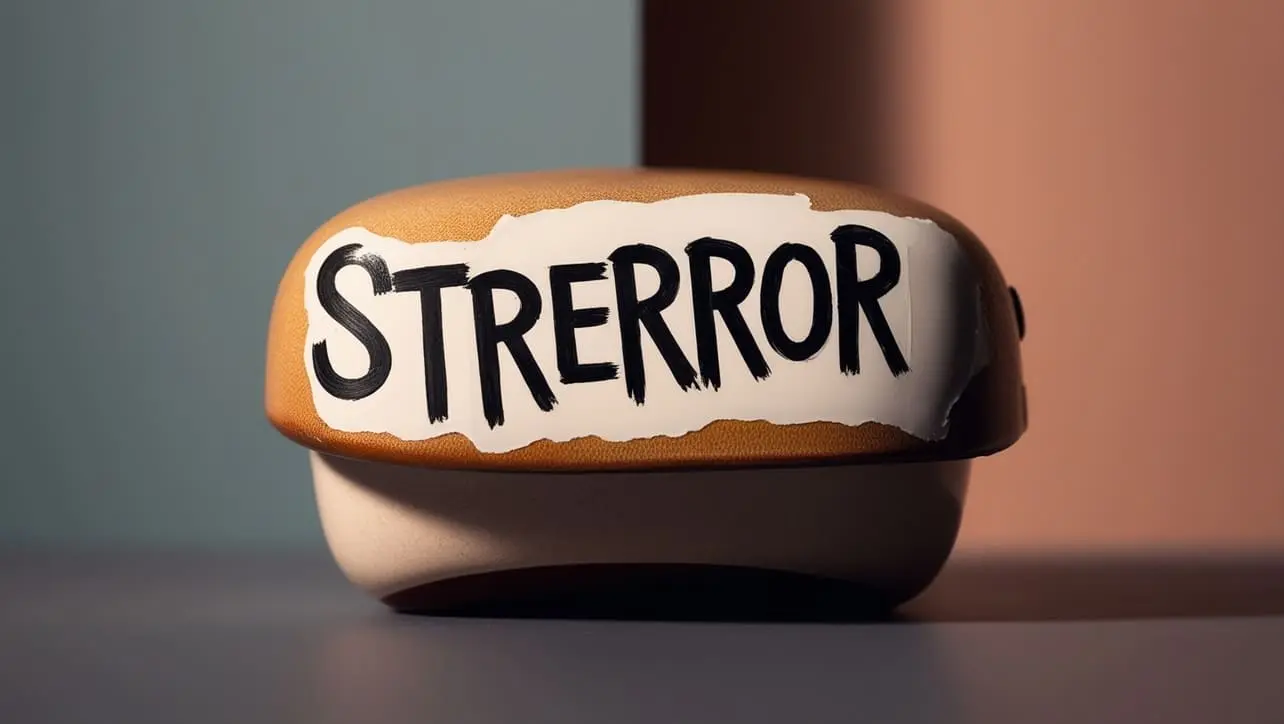
Photo Credit to CodeToFun
Introduction
In C++ programming, error handling is an essential aspect of robust software development.
The strerror()
function is a part of the C Standard Library, and it is used to retrieve a textual representation of the current errno value.
In this tutorial, we'll explore the usage and functionality of the strerror()
function in C++.
Syntax
The signature of the strerror()
function is as follows:
char* strerror(int errnum);
This function takes an error number errnum as an argument and returns a pointer to the textual representation of the corresponding error.
Example
Let's delve into an example to illustrate how the strerror()
function works.
#include <iostream>
#include <cstring>
int main() {
// Simulate an error by setting errno
errno = ENOENT; // No such file or directory
// Get the error message using strerror()
const char * errorMessage = strerror(errno);
// Output the error message
std::cout << "Error: " << errorMessage << std::endl;
return 0;
}
Output
Error: No such file or directory
How the Program Works
In this example, the strerror()
function is used to obtain a textual representation of the error associated with errno, which is set to ENOENT (No such file or directory). The error message is then printed to the console.
Return Value
The strerror()
function returns a pointer to a null-terminated string containing the textual representation of the error.
Common Use Cases
The strerror()
function is particularly useful in scenarios where you want to convert an error number into a human-readable error message. This can be crucial for providing meaningful information about errors in your program.
Notes
- Ensure that you include the necessary header file for
strerror()
, which is <cstring> in C++. - The errno variable is a part of the C Standard Library and is used to store information about errors.
Optimization
The strerror()
function itself is optimized for efficiency. However, for performance-critical applications, consider handling errors in a way that minimizes the need for frequent calls to strerror()
.
Conclusion
The strerror()
function in C++ is a valuable tool for error handling. It allows developers to obtain human-readable error messages associated with error numbers, facilitating effective debugging and troubleshooting.
Feel free to experiment with different error numbers and explore the behavior of the strerror()
function in various error-handling scenarios. Happy coding!
Join our Community:
Author
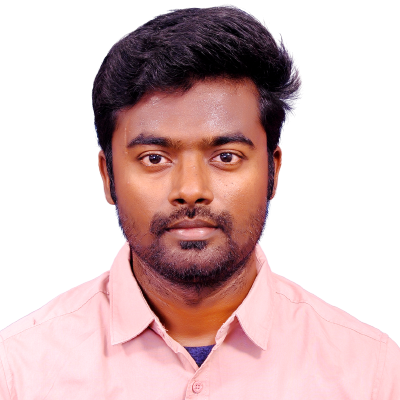
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C++ String strerror() Function), please comment here. I will help you immediately.