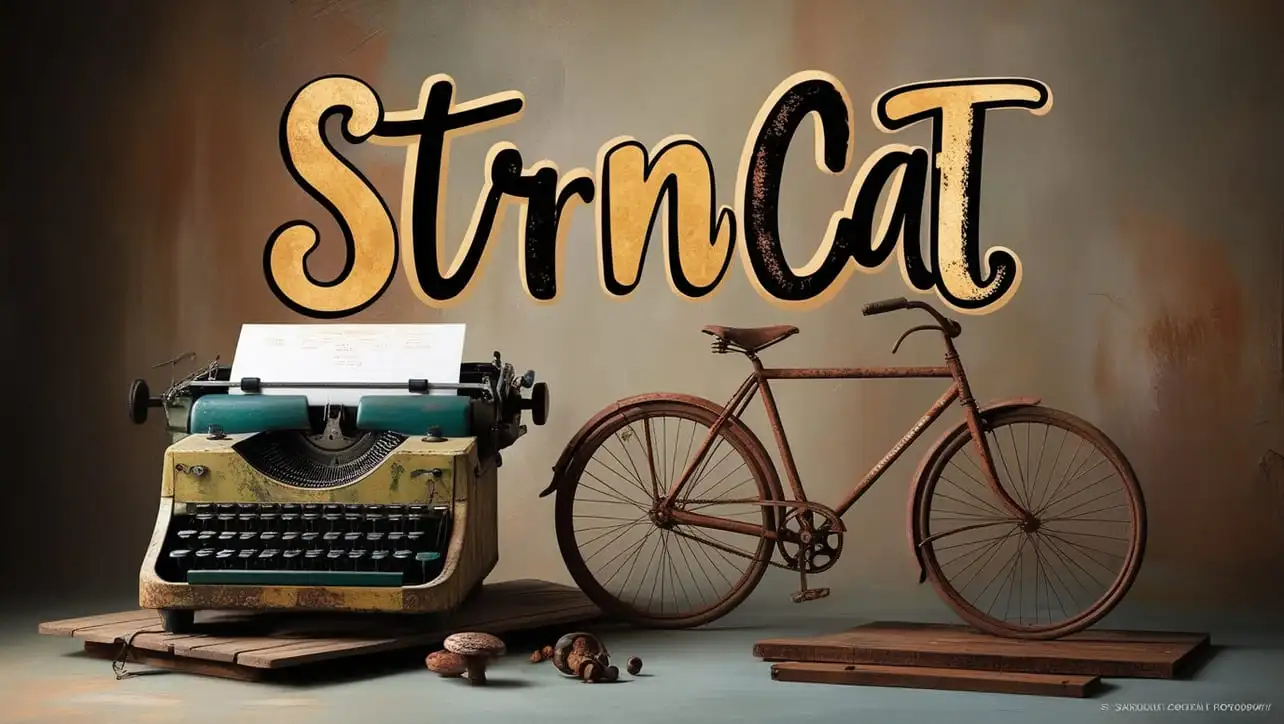
C++ Topics
- C++ Intro
- C++ String Functions
- C++ Interview Programs
- Abundant Number
- Amicable Number
- Armstrong Number
- Average of N Numbers
- Automorphic Number
- Biggest of three numbers
- Binary to Decimal
- Common Divisors
- Composite Number
- Condense a Number
- Cube Number
- Decimal to Binary
- Decimal to Octal
- Disarium Number
- Even Number
- Evil Number
- Factorial of a Number
- Fibonacci Series
- GCD
- Happy Number
- Harshad Number
- LCM
- Leap Year
- Magic Number
- Matrix Addition
- Matrix Division
- Matrix Multiplication
- Matrix Subtraction
- Matrix Transpose
- Maximum Value of an Array
- Minimum Value of an Array
- Multiplication Table
- Natural Number
- Number Combination
- Odd Number
- Palindrome Number
- Pascalβs Triangle
- Perfect Number
- Perfect Square
- Power of 2
- Power of 3
- Pronic Number
- Prime Factor
- Prime Number
- Smith Number
- Strong Number
- Sum of Array
- Sum of Digits
- Swap Two Numbers
- Triangular Number
- C++ Star Pattern
- C++ Number Pattern
- C++ Alphabet Pattern
C++ Program to Check Power of 3
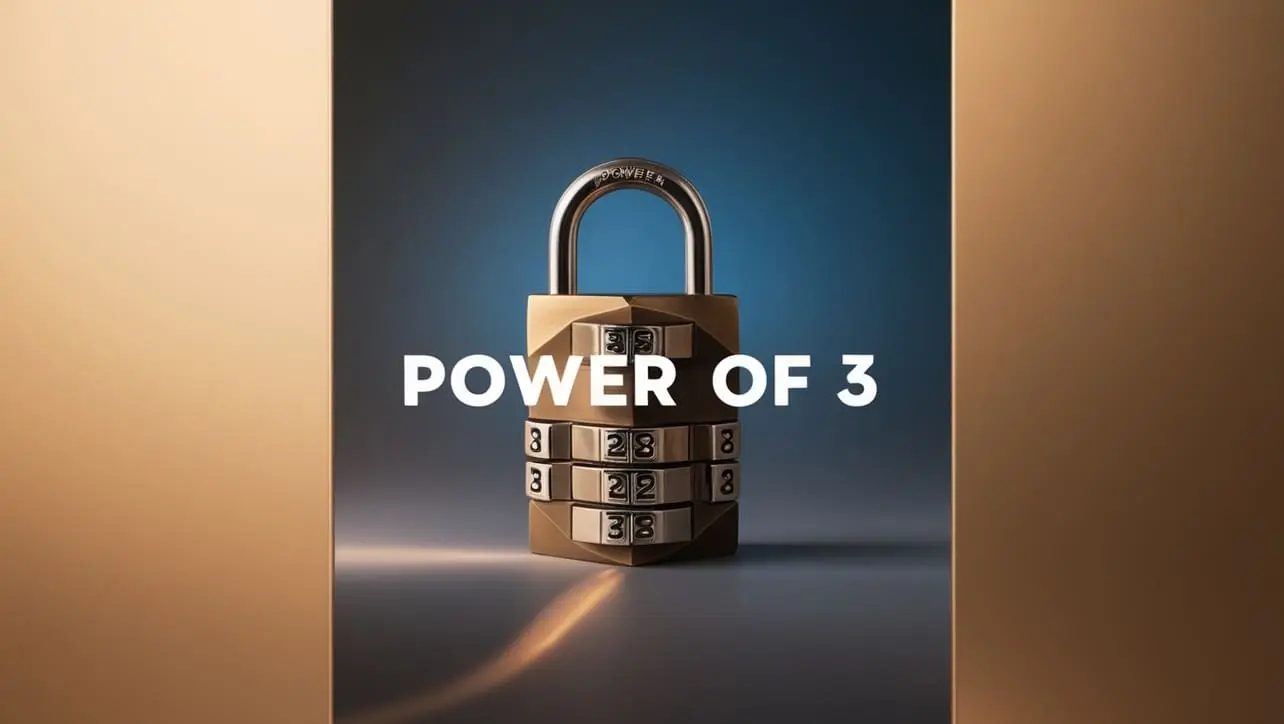
Photo Credit to CodeToFun
π Introduction
In the realm of programming, one often encounters the need to check if a given number is a power of another.
In this case, we'll explore a C++ program specifically designed to check if a number is a power of 3.
The logic behind this program involves repeatedly dividing the number by 3 until it becomes 1, indicating that it is a power of 3.
π Example
Let's delve into the C++ code that achieves this functionality.
#include <iostream>
// Function to check if a number is a power of 3
bool isPowerOf3(int n) {
// Keep dividing the number by 3 until it is greater than 1
while (n % 3 == 0 && n > 1) {
n /= 3;
}
// If the final value is 1, the original number is a power of 3
return n == 1;
}
// Driver program
int main() {
// Replace this value with your desired number
int number = 27;
// Check if the number is a power of 3
if (isPowerOf3(number)) {
std::cout << number << " is a power of 3" << std::endl;
} else {
std::cout << number << " is not a power of 3" << std::endl;
}
return 0;
}
π» Testing the Program
To test the program with different numbers, simply replace the value of number in the main function.
27 is a power of 3
Compile and run the program to see if the given number is a power of 3.
π§ How the Program Works
- The program defines a function isPowerOf3 that takes an integer as input and checks if it is a power of 3.
- Inside the function, it repeatedly divides the number by 3 until it becomes greater than 1.
- If the final value is 1, the original number is a power of 3, and the function returns true; otherwise, it returns false.
- The driver program in the main function tests a specific number and prints whether it is a power of 3 or not.
π Between the Given Range
Let's take a look at the C++ code that checks for powers of 3 in the given range.
#include <iostream>
#include <cmath>
// Function to check if a number is a power of 3
bool isPowerOfThree(int num) {
if (num <= 0) {
return false;
}
// Using logarithmic property to check if num is a power of 3
double logResult = log10(num) / log10(3);
return (logResult - int(logResult)) == 0;
}
// Driver program
int main() {
// Output header
std::cout << "Power of 3 in the range 1 to 20:" << std::endl;
// Loop through numbers 1 to 20 and check if they are powers of 3
for (int i = 1; i <= 20; ++i) {
if (isPowerOfThree(i)) {
std::cout << i << " ";
}
}
std::cout << std::endl;
return 0;
}
π» Testing the Program
Power of 3 in the range 1 to 20: 1 3 9
Compile and run the program to see the numbers in the range 1 to 20 that are powers of 3.
π§ How the Program Works
- The program defines a function isPowerOfThree that checks if a given number is a power of 3.
- Inside the function, it uses logarithmic properties to determine if the logarithm of the number with base 3 is an integer.
- The main function tests this by iterating through numbers from 1 to 20 and printing those that are powers of 3.
π§ Understanding the Concept of Power of 3
Before diving into the code, let's understand the concept of a power of 3.
A number is considered a power of 3 if it can be expressed as 3^n, where n is an integer.
For example, 1, 3, 9, 27, and so on are powers of 3.
π’ Optimizing the Program
While the provided program is straightforward, there are alternative approaches to check if a number is a power of 3. Consider exploring other mathematical techniques for optimization.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
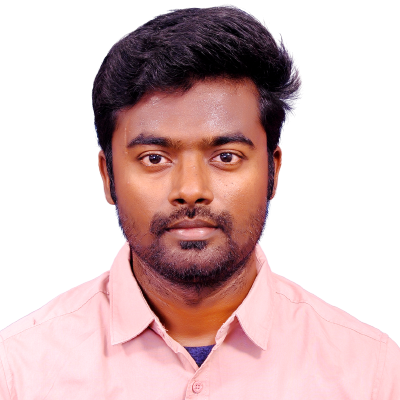
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C++ Program to Check Power of 3), please comment here. I will help you immediately.