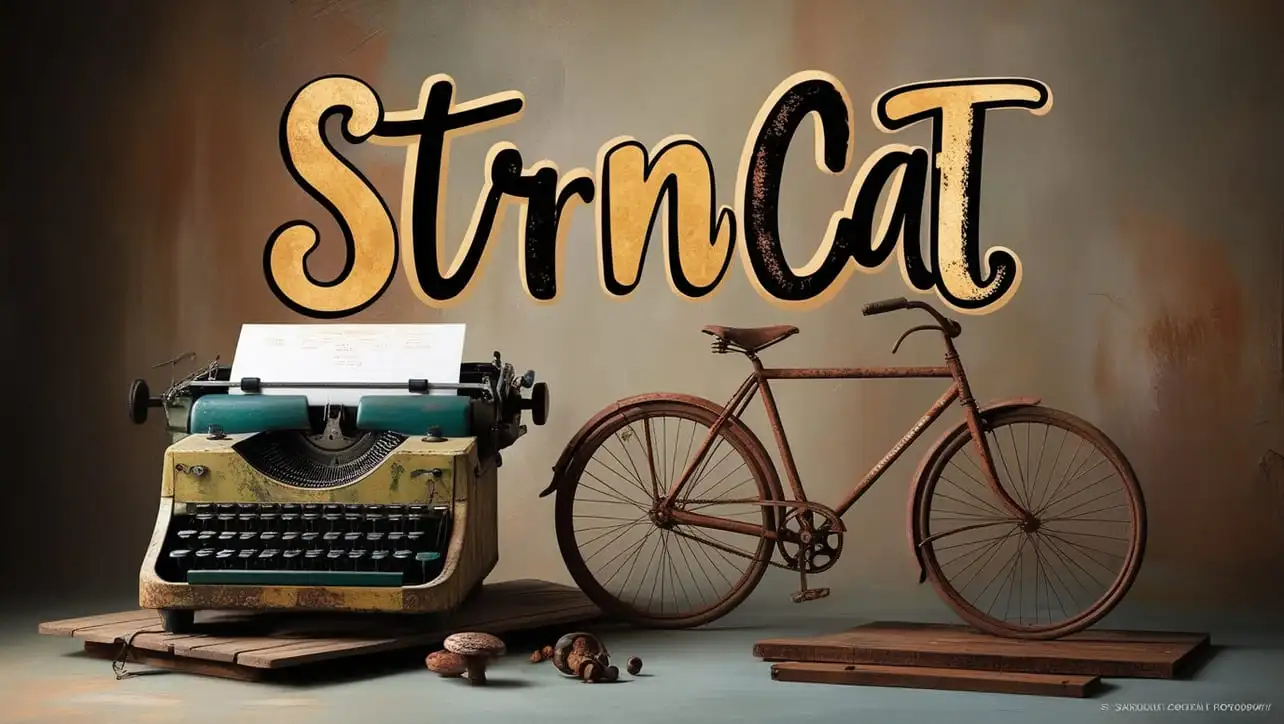
C++ Topics
- C++ Intro
- C++ String Functions
- C++ Interview Programs
- Abundant Number
- Amicable Number
- Armstrong Number
- Average of N Numbers
- Automorphic Number
- Biggest of three numbers
- Binary to Decimal
- Common Divisors
- Composite Number
- Condense a Number
- Cube Number
- Decimal to Binary
- Decimal to Octal
- Disarium Number
- Even Number
- Evil Number
- Factorial of a Number
- Fibonacci Series
- GCD
- Happy Number
- Harshad Number
- LCM
- Leap Year
- Magic Number
- Matrix Addition
- Matrix Division
- Matrix Multiplication
- Matrix Subtraction
- Matrix Transpose
- Maximum Value of an Array
- Minimum Value of an Array
- Multiplication Table
- Natural Number
- Number Combination
- Odd Number
- Palindrome Number
- Pascal’s Triangle
- Perfect Number
- Perfect Square
- Power of 2
- Power of 3
- Pronic Number
- Prime Factor
- Prime Number
- Smith Number
- Strong Number
- Sum of Array
- Sum of Digits
- Swap Two Numbers
- Triangular Number
- C++ Star Pattern
- C++ Number Pattern
- C++ Alphabet Pattern
C++ Program to Generate Pascal’s Triangle
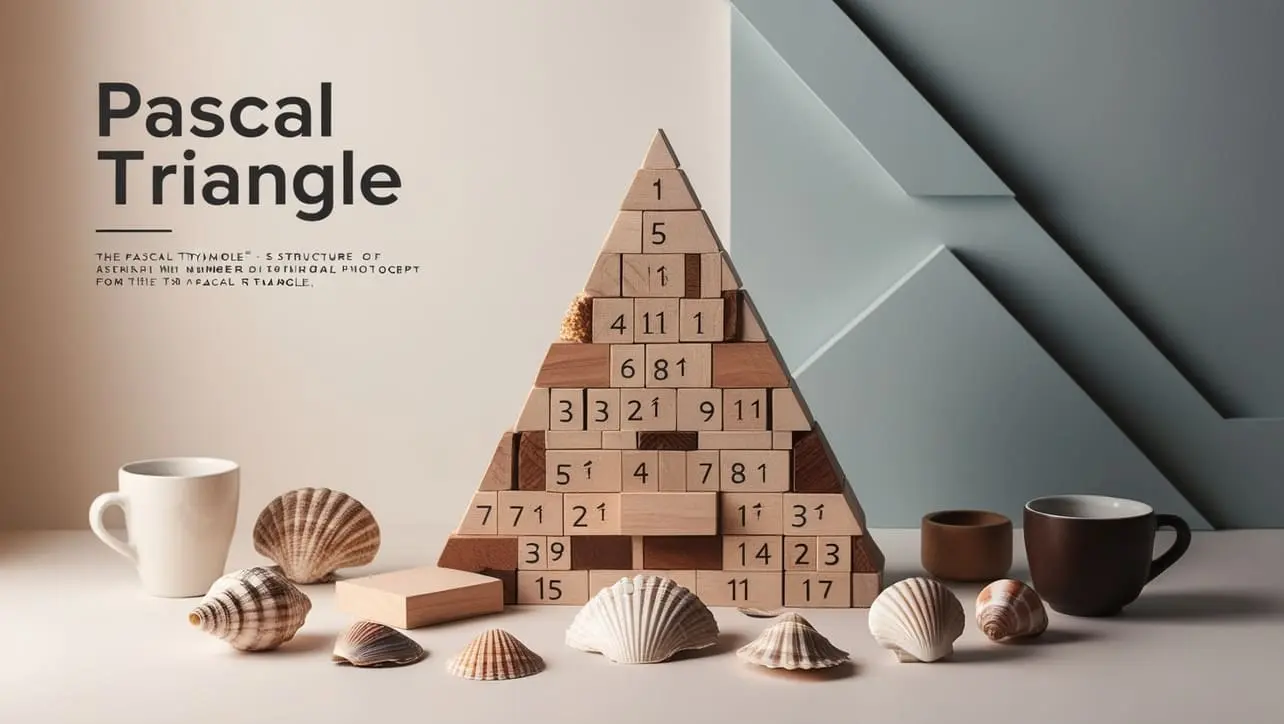
Photo Credit to CodeToFun
🙋 Introduction
Pascal's Triangle is a mathematical construct named after the French mathematician Blaise Pascal.
It is an arrangement of numbers in a triangular shape where each number is the sum of the two numbers directly above it. This triangle has many interesting properties and applications in combinatorics and algebra.
In this tutorial, we'll explore a C++ program that generates Pascal's Triangle and prints it to the console.
📄 Example
Let's delve into the C++ code that generates Pascal's Triangle.
#include <iostream>
// Function to generate and print Pascal's Triangle
void generatePascalsTriangle(int rows) {
for (int i = 0; i < rows; i++) {
// Print leading spaces for alignment
for (int space = 0; space < rows - i - 1; space++) {
std::cout << " ";
}
int coefficient = 1;
for (int j = 0; j <= i; j++) {
// Print the current coefficient
std::cout << coefficient << " ";
// Update the coefficient for the next iteration
coefficient = coefficient * (i - j) / (j + 1);
}
std::cout << std::endl;
}
}
int main() {
// Replace this value with the desired number of rows
int numRows = 5;
// Call the function to generate Pascal's Triangle
generatePascalsTriangle(numRows);
return 0;
}
💻 Testing the Program
To test the program with a different number of rows, simply replace the value of numRows in the main function.
1 1 1 1 2 1 1 3 3 1 1 4 6 4 1
🧠 How the Program Works
- The program defines a function generatePascalsTriangle that takes the number of rows as input and prints Pascal's Triangle up to the specified number of rows.
- Inside the function, two nested loops are used. The outer loop iterates through each row, and the inner loop calculates and prints the coefficients of each row.
- The coefficients are calculated using the formula C(n, k) = n! / (k! * (n-k)!), where n is the row number, and k is the position in the row.
🧐 Understanding the Concept of Pascal's Triangle
Pascal's Triangle is a triangular array of numbers in which each number is the sum of the two numbers directly above it.The first few rows of Pascal's Triangle look like this:
1 1 1 1 2 1 1 3 3 1 1 4 6 4 1
Each number in the triangle represents a binomial coefficient, also known as "n choose k," denoted as C(n, k). These coefficients have many applications in probability, algebra, and combinatorics.
🎢 Optimizing the Program
While the provided program is straightforward, consider exploring ways to optimize it for larger triangle sizes or implementing additional features, such as formatting the triangle for better readability.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
👨💻 Join our Community:
Author
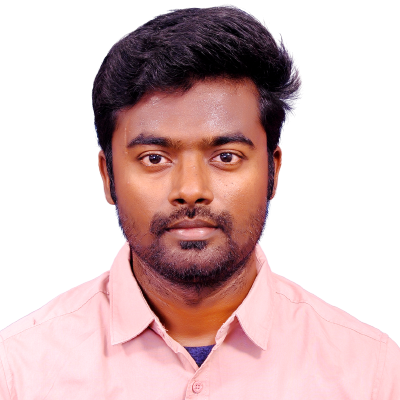
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C++ Program to Generate Pascal’s Triangle), please comment here. I will help you immediately.