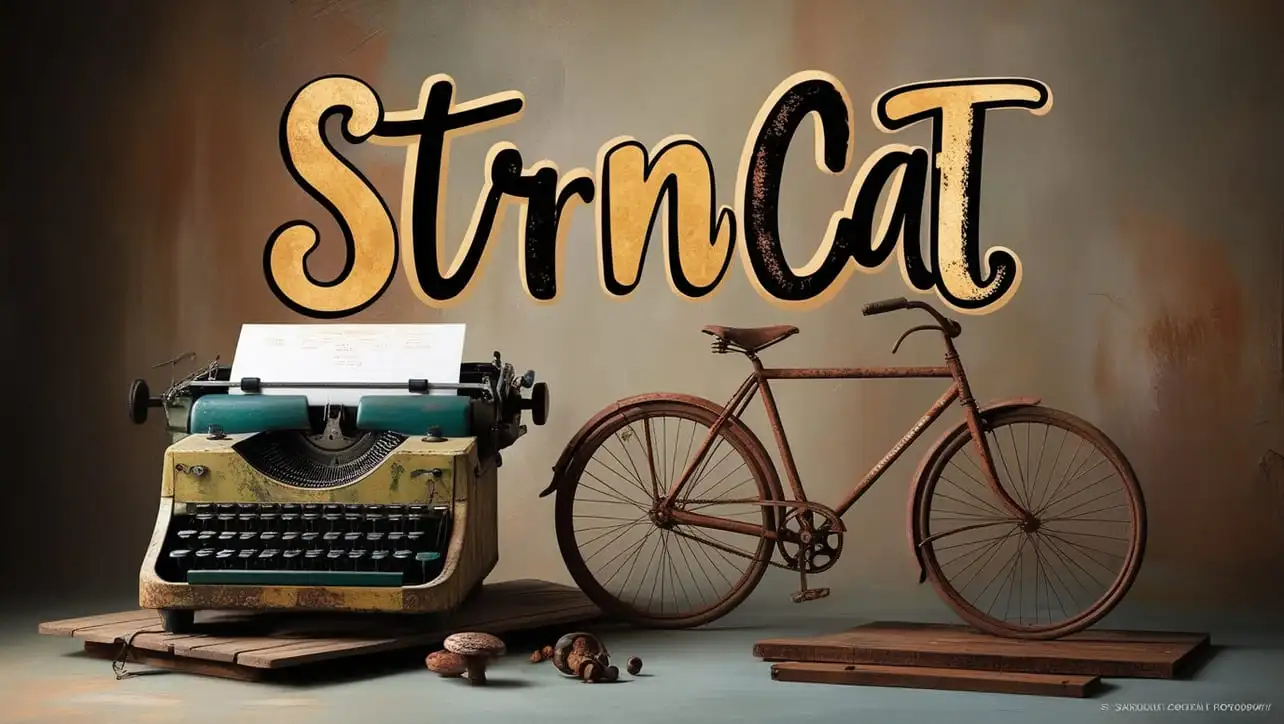
C++ Topics
- C++ Intro
- C++ String Functions
- C++ Interview Programs
- Abundant Number
- Amicable Number
- Armstrong Number
- Average of N Numbers
- Automorphic Number
- Biggest of three numbers
- Binary to Decimal
- Common Divisors
- Composite Number
- Condense a Number
- Cube Number
- Decimal to Binary
- Decimal to Octal
- Disarium Number
- Even Number
- Evil Number
- Factorial of a Number
- Fibonacci Series
- GCD
- Happy Number
- Harshad Number
- LCM
- Leap Year
- Magic Number
- Matrix Addition
- Matrix Division
- Matrix Multiplication
- Matrix Subtraction
- Matrix Transpose
- Maximum Value of an Array
- Minimum Value of an Array
- Multiplication Table
- Natural Number
- Number Combination
- Odd Number
- Palindrome Number
- Pascalβs Triangle
- Perfect Number
- Perfect Square
- Power of 2
- Power of 3
- Pronic Number
- Prime Factor
- Prime Number
- Smith Number
- Strong Number
- Sum of Array
- Sum of Digits
- Swap Two Numbers
- Triangular Number
- C++ Star Pattern
- C++ Number Pattern
- C++ Alphabet Pattern
C++ Program to find GCD
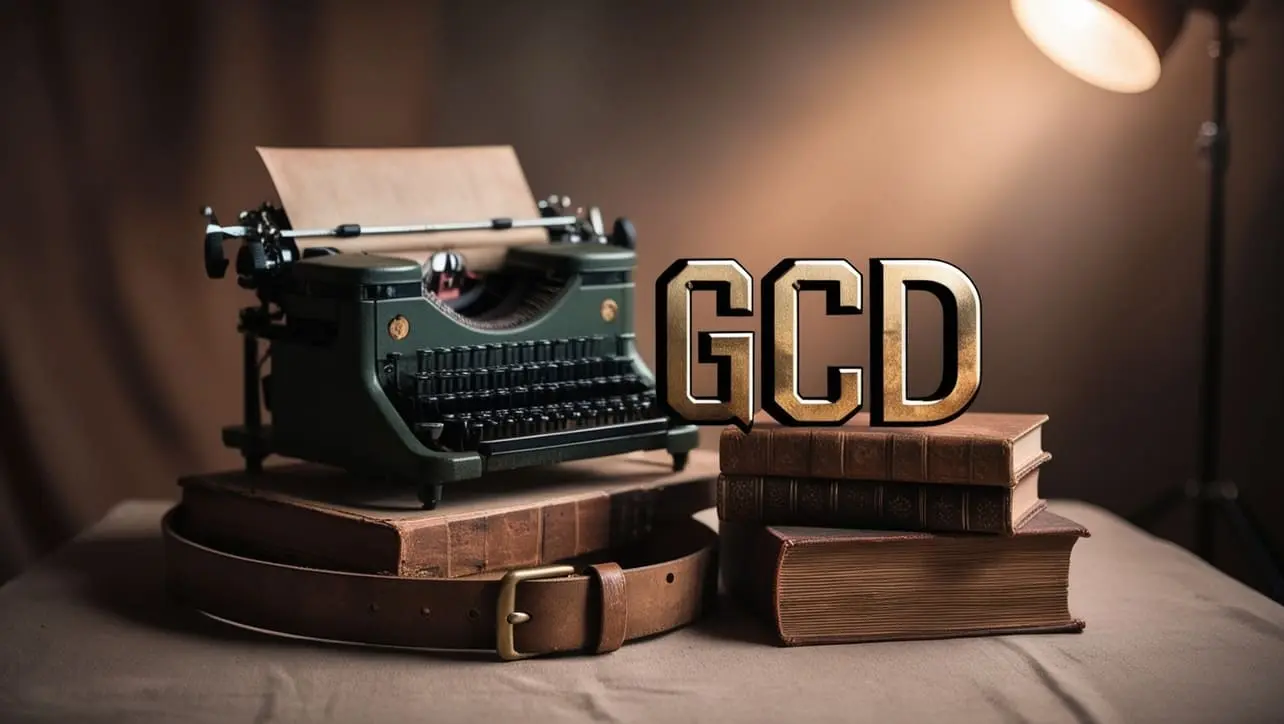
Photo Credit to CodeToFun
Introduction
In the realm of programming, solving mathematical problems is a common and essential task. One frequently encountered mathematical concept is the Greatest Common Divisor (GCD) of two numbers.
The GCD is the largest positive integer that divides both numbers without leaving a remainder.
In this tutorial, we'll explore a C++ program that efficiently calculates the GCD of two given numbers.
Example
Let's delve into the C++ code that achieves this functionality.
#include <iostream>
// Function to find GCD using Euclidean algorithm
int findGCD(int num1, int num2) {
while (num2 != 0) {
int temp = num2;
num2 = num1 % num2;
num1 = temp;
}
return num1;
}
// Driver program
int main() {
// Replace these values with your desired numbers
int number1 = 48;
int number2 = 18;
// Call the function to find GCD
int gcd = findGCD(number1, number2);
std::cout << "GCD of " << number1 << " and " << number2 << " is: " << gcd << std::endl;
return 0;
}
Testing the Program
To test the program with different numbers, simply replace the values of number1 and number2 in the main function.
GCD of 48 and 18 is: 6
Compile and run the program to see the GCD in action.
How the Program Works
- The program defines a function findGCD that takes two numbers as input and uses the Euclidean algorithm to calculate their GCD.
- Inside the main function, replace the values of number1 and number2 with the desired numbers.
- The program calls the findGCD function and prints the result.
Understanding the Euclidean Algorithm
The Euclidean algorithm is a widely used method for finding the GCD of two numbers. It iteratively replaces the larger number with the remainder of the division of the larger number by the smaller number until the remainder becomes zero. The GCD is then the non-zero remainder.
Real-World Applications
Understanding and calculating the GCD is essential in various fields, including cryptography, computer science, and number theory.
For instance, it plays a crucial role in algorithms for reducing fractions to their simplest form.
Optimizing the Program
While the provided program is effective, there are other algorithms, such as the Stein algorithm, that can be more efficient for large numbers. Consider exploring and implementing different algorithms based on your specific requirements.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
Join our Community:
Author
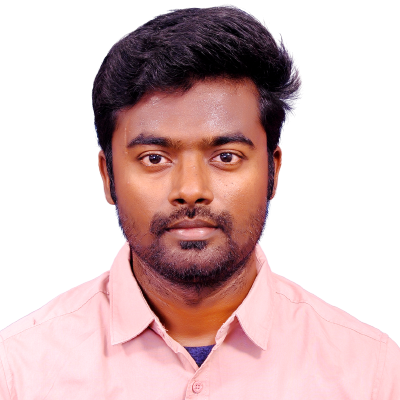
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C++ Program to find GCD), please comment here. I will help you immediately.