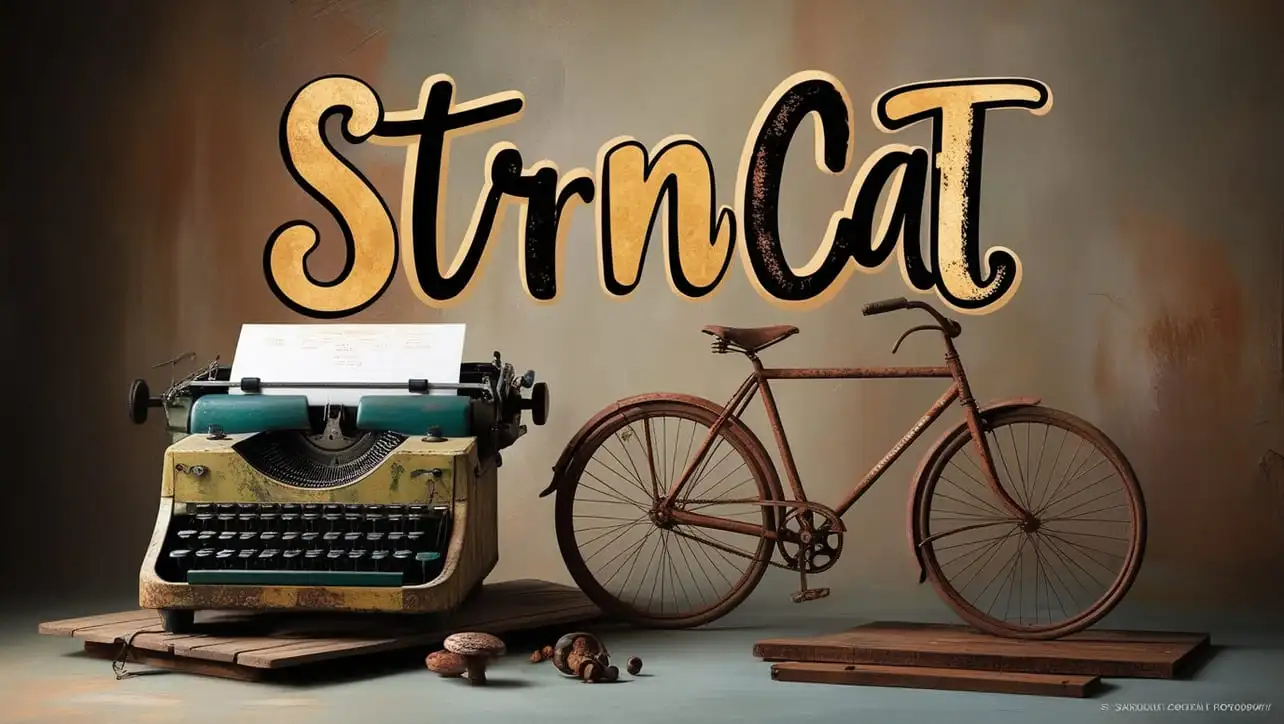
C++ Topics
- C++ Intro
- C++ String Functions
- C++ Interview Programs
- Abundant Number
- Amicable Number
- Armstrong Number
- Average of N Numbers
- Automorphic Number
- Biggest of three numbers
- Binary to Decimal
- Common Divisors
- Composite Number
- Condense a Number
- Cube Number
- Decimal to Binary
- Decimal to Octal
- Disarium Number
- Even Number
- Evil Number
- Factorial of a Number
- Fibonacci Series
- GCD
- Happy Number
- Harshad Number
- LCM
- Leap Year
- Magic Number
- Matrix Addition
- Matrix Division
- Matrix Multiplication
- Matrix Subtraction
- Matrix Transpose
- Maximum Value of an Array
- Minimum Value of an Array
- Multiplication Table
- Natural Number
- Number Combination
- Odd Number
- Palindrome Number
- Pascalβs Triangle
- Perfect Number
- Perfect Square
- Power of 2
- Power of 3
- Pronic Number
- Prime Factor
- Prime Number
- Smith Number
- Strong Number
- Sum of Array
- Sum of Digits
- Swap Two Numbers
- Triangular Number
- C++ Star Pattern
- C++ Number Pattern
- C++ Alphabet Pattern
C++ Program to find Factorial of a Number
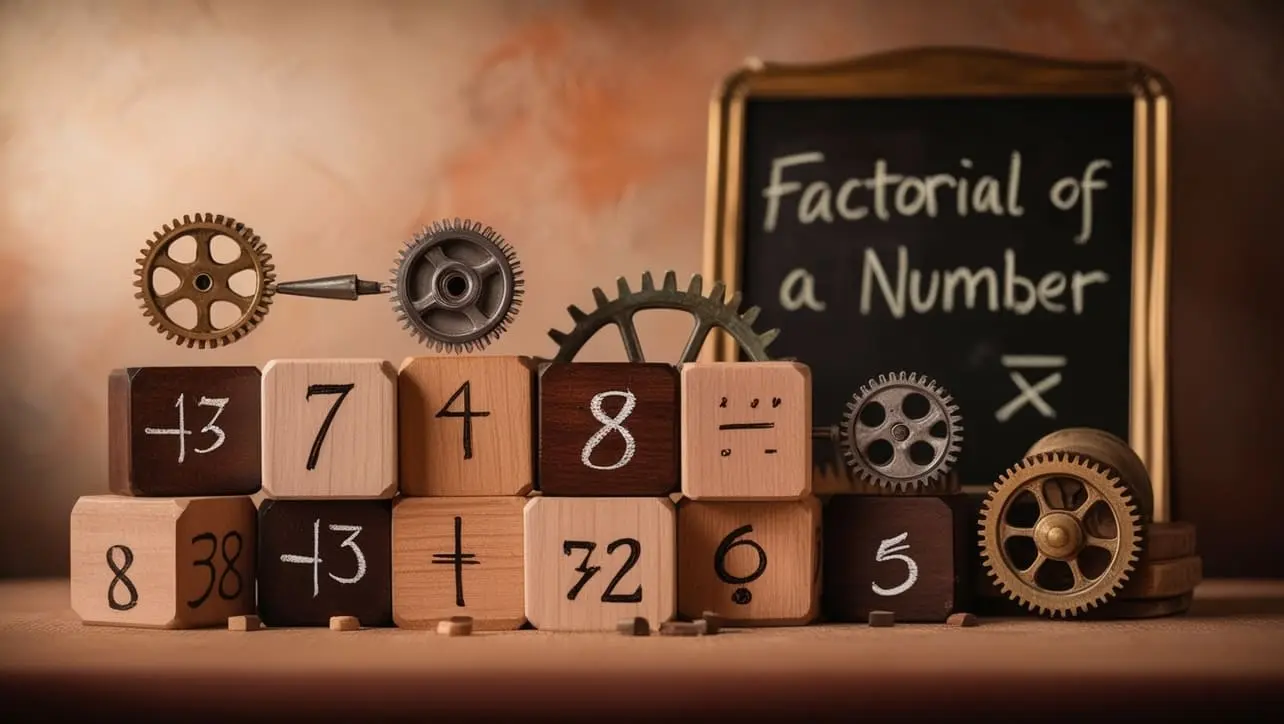
Photo Credit to CodeToFun
π Introduction
In the world of programming, solving mathematical problems is a fundamental skill. One such mathematical operation is finding the factorial of a number.
The factorial of a non-negative integer is the product of all positive integers less than or equal to that number. It's denoted by the symbol !.
In this tutorial, we'll explore a C++ program that efficiently calculates the factorial of a given number.
π Example
Let's dive into the C++ code to achieve this functionality.
#include <iostream>
// Function to calculate factorial
unsigned long long calculateFactorial(int num) {
if (num == 0 || num == 1) {
return 1;
} else {
return num * calculateFactorial(num - 1);
}
}
// Driver program
int main() {
// Replace this value with your desired number
int number = 5;
// Call the function to calculate factorial
unsigned long long result = calculateFactorial(number);
// Display the result
std::cout << "Factorial of " << number << " is: " << result << std::endl;
return 0;
}
π» Testing the Program
To test the program with a different number, replace the value of number in the main function.
Factorial of 5 is: 120
Compile and run the program to see the factorial in action.
π§ How the Program Works
- The program defines a function calculateFactorial that recursively calculates the factorial of a given number.
- In the main function, replace the value of number with the desired input.
- The program then calls the calculateFactorial function to compute the factorial.
- The result is displayed using std::cout.
π§ Understanding the Concept of Factorial
Before delving into the code, let's take a moment to understand the concept of factorial.
The factorial of a non-negative integer n, denoted as n!, is the product of all positive integers less than or equal to n.
For example, the factorial of 5 (5!) is calculated as 5 × 4 × 3 × 2 × 1 = 120.
π’ Optimizing the Program
While the provided program is effective, it uses recursion to calculate the factorial. Depending on the input value, this approach may lead to a stack overflow for large numbers. Consider exploring iterative solutions for larger inputs or optimizing the recursive approach.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
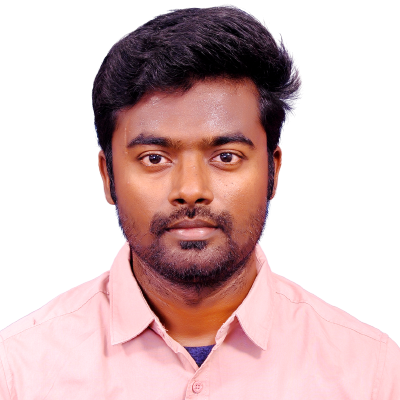
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C++ Program to find Factorial of a Number), please comment here. I will help you immediately.