.webp)
C++ Basic
C++ Interview Programs
- C++ Interview Programs
- C++ Abundant Number
- C++ Amicable Number
- C++ Armstrong Number
- C++ Average of N Numbers
- C++ Automorphic Number
- C++ Biggest of three numbers
- C++ Binary to Decimal
- C++ Common Divisors
- C++ Composite Number
- C++ Condense a Number
- C++ Cube Number
- C++ Decimal to Binary
- C++ Decimal to Octal
- C++ Disarium Number
- C++ Even Number
- C++ Evil Number
- C++ Factorial of a Number
- C++ Fibonacci Series
- C++ GCD
- C++ Happy Number
- C++ Harshad Number
- C++ LCM
- C++ Leap Year
- C++ Magic Number
- C++ Matrix Addition
- C++ Matrix Division
- C++ Matrix Multiplication
- C++ Matrix Subtraction
- C++ Matrix Transpose
- C++ Maximum Value of an Array
- C++ Minimum Value of an Array
- C++ Multiplication Table
- C++ Natural Number
- C++ Number Combination
- C++ Odd Number
- C++ Palindrome Number
- C++ Pascalβs Triangle
- C++ Perfect Number
- C++ Perfect Square
- C++ Power of 2
- C++ Power of 3
- C++ Pronic Number
- C++ Prime Factor
- C++ Prime Number
- C++ Smith Number
- C++ Strong Number
- C++ Sum of Array
- C++ Sum of Digits
- C++ Swap Two Numbers
- C++ Triangular Number
C++ Program to Check for a Cube Number
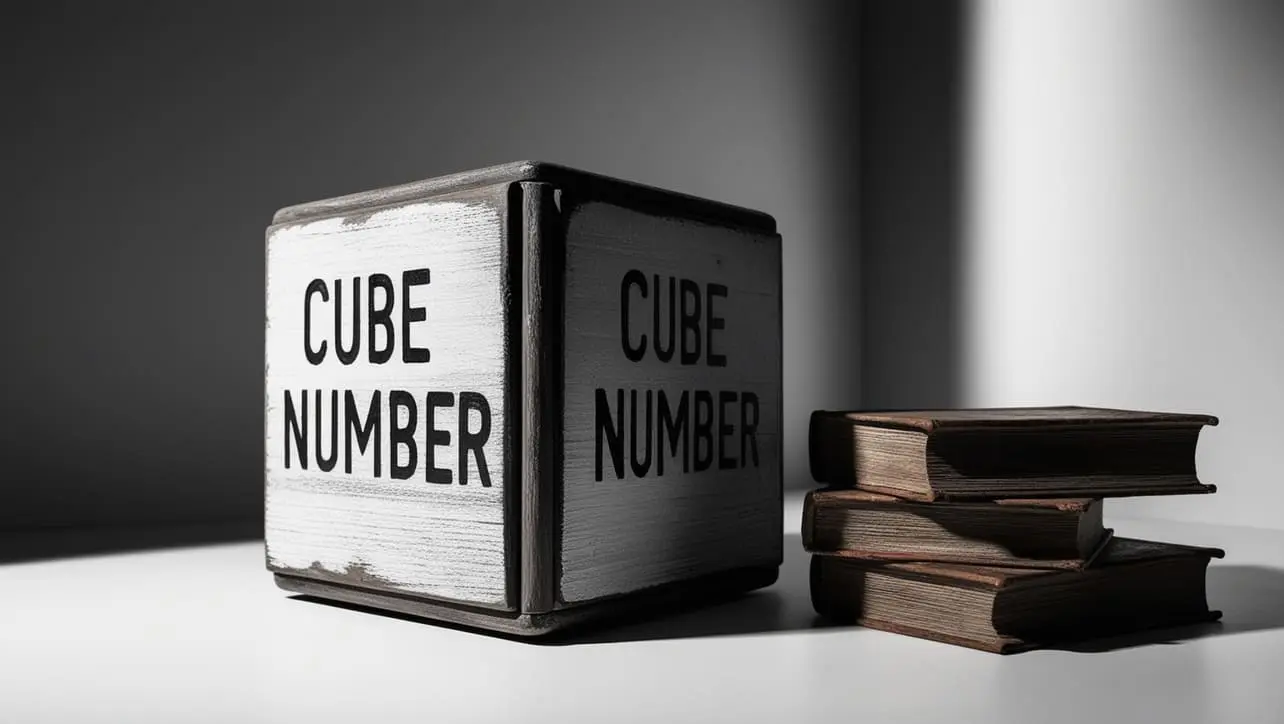
Photo Credit to CodeToFun
π Introduction
In the realm of programming, mathematical properties of numbers often lead to interesting and practical applications. One such property is being a cube number. A cube number is an integer that is the cube of an integer.
In this tutorial, we'll delve into a C++ program that efficiently checks whether a given number is a perfect cube or not.
π Example
Let's take a look at the C++ code that accomplishes this functionality.
#include <iostream>
#include <cmath>
// Function to check if a number is a perfect cube
bool isPerfectCube(int number) {
// Calculate the cube root of the number
double cubeRoot = cbrt(number);
// Check if the cube root is close to an integer
return std::abs(cubeRoot - round(cubeRoot)) < 1e-10;
}
// Driver program
int main() {
// Replace this value with your desired number
int number = 27;
// Check if the number is a perfect cube
if (isPerfectCube(number)) {
std::cout << number << " is a perfect cube.\n";
} else {
std::cout << number << " is not a perfect cube.\n";
}
return 0;
}
π» Testing the Program
To test the program with different numbers, simply replace the value of the number variable in the main function.
27 is a cube number.
Compile and run the program to check if the number is a perfect cube.
π§ How the Program Works
- The program defines a function isPerfectCube that takes an integer as input and returns true if it is a perfect cube, and false otherwise.
- Inside the function, it calculates the cube root of the number using the cbrt function from the <cmath> header.
- It then checks if the cube root is close to an integer using a tolerance value (1e-10 in this case).
- The main function initializes a variable with a number and calls the isPerfectCube function to determine if it's a perfect cube.
π Between the Given Range
Let's delve into the C++ code that checks and displays cube numbers in the specified range.
#include <iostream>
#include <bits/stdc++.h>
// Function to check if a number is a cube number
bool isCubeNumber(int number) {
int cubeRoot = cbrt(number); // cbrt() calculates the cube root
return (cubeRoot * cubeRoot * cubeRoot == number);
}
// Driver program
int main() {
std::cout << "Cube numbers in the range 1 to 50:" << std::endl;
for (int i = 1; i <= 50; ++i) {
if (isCubeNumber(i)) {
std::cout << i << " ";
}
}
std::cout << std::endl;
return 0;
}
π» Testing the Program
Cube numbers in the range 1 to 50: 1 8 27
Compile and run the program to see the cube numbers in the specified range.
π§ How the Program Works
- The program defines a function isCubeNumber that takes an integer as input and checks if it is a cube number using the cbrt function, which calculates the cube root.
- The main function iterates through numbers from 1 to 50 and prints those that are cube numbers.
π§ Understanding the Concept of Cube Number
A cube number is the result of raising an integer to the power of 3. For example, 2 * 2 * 2 = 8, so 8 is a cube number.
π’ Optimizing the Program
The provided program is straightforward, but you might consider optimizing it further. For instance, you can explore alternative methods for checking if a number is a cube number without using the cbrt function.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
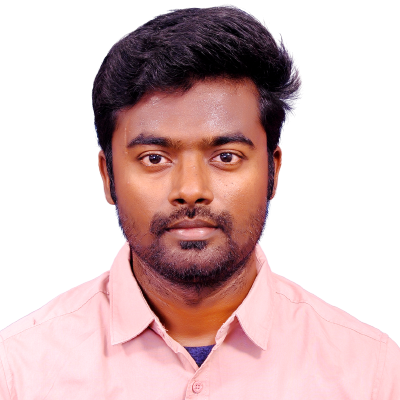
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C++ Program to Check for a Cube Number), please comment here. I will help you immediately.