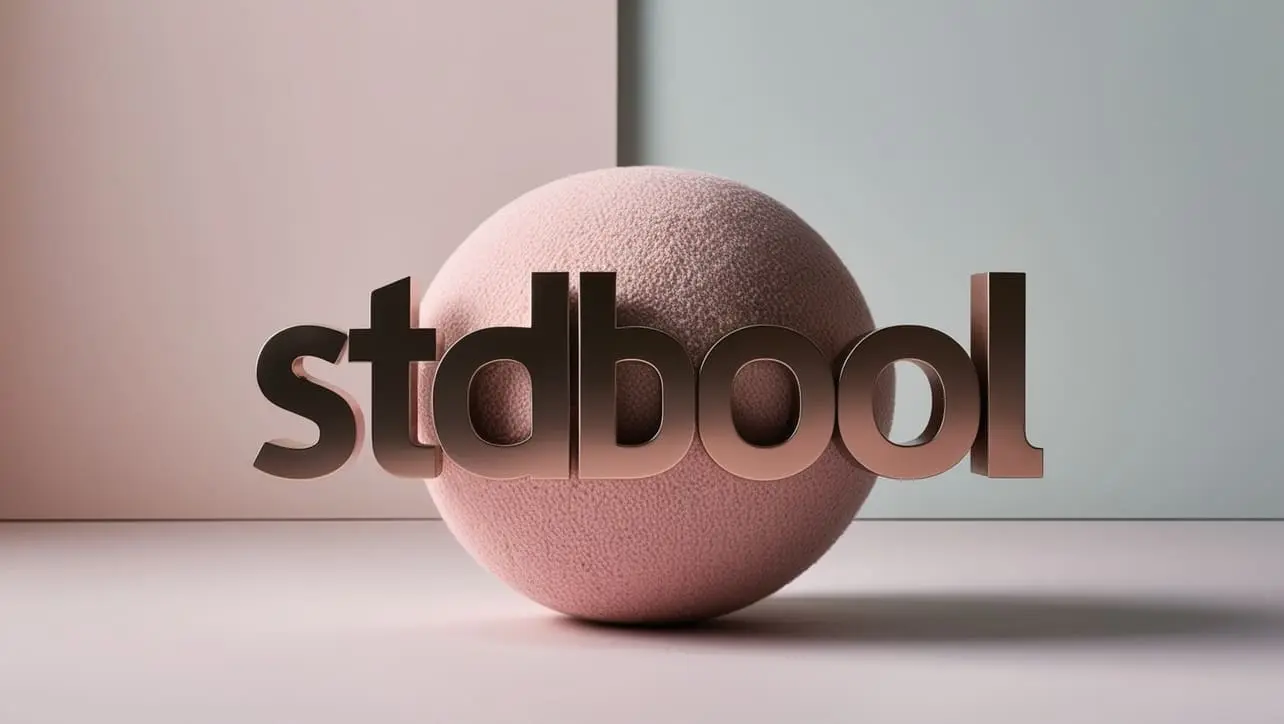
C while Loop
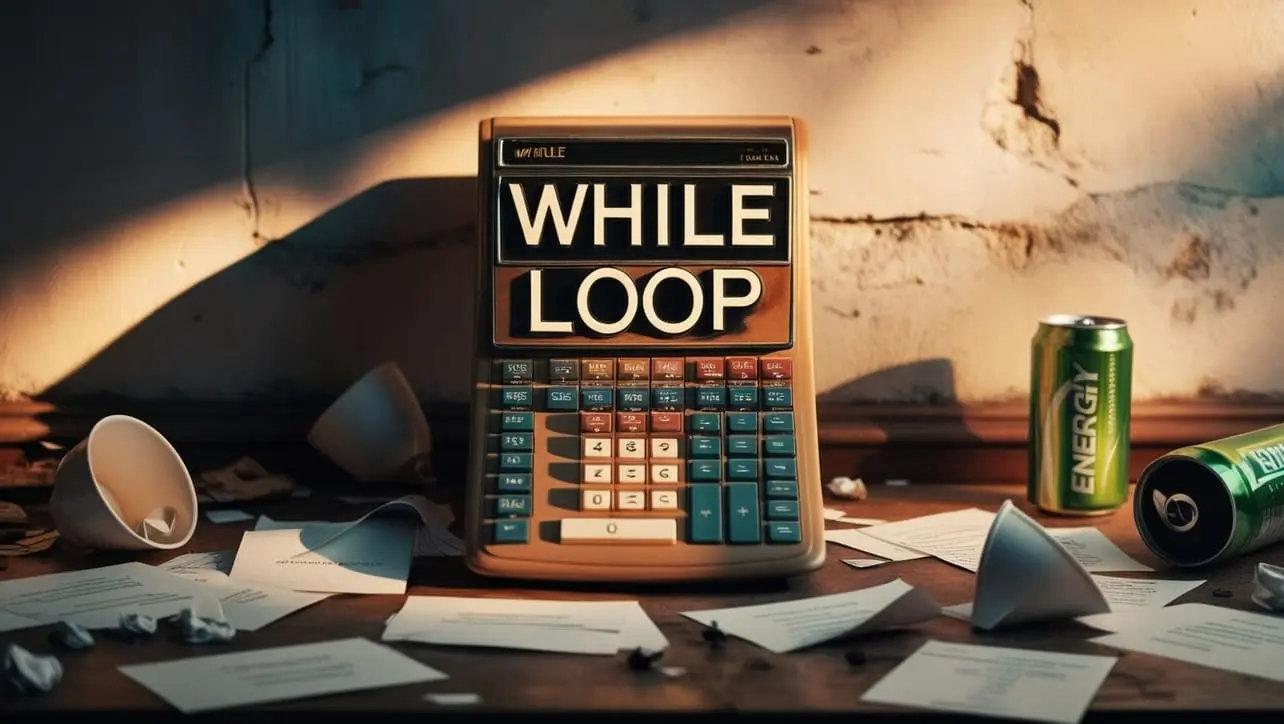
Photo Credit to CodeToFun
π Introduction
The while
loop in C is a fundamental control structure used to repeat a block of code as long as a specified condition remains true.
This guide will provide an in-depth look at how the while
loop works, its syntax, and practical examples of its usage.
π€ What is a while Loop?
In C programming, a while
loop is used to execute a block of code repeatedly as long as a given condition evaluates to true. The condition is evaluated before the execution of the loopβs body, making it a pre-test loop.
π Key Features
- Pre-test Loop: The condition is evaluated before executing the loop body.
- Flexible Control: Can handle complex conditions and dynamic ranges.
- Used for Unknown Iterations: Ideal when the number of iterations is not known beforehand.
π‘ Syntax
The syntax for the while
loop is straightforward:
while (condition) {
// Code to be executed
}
π§ Explanation:
- condition: A boolean expression that is evaluated before each iteration. If the condition is true, the loop body is executed. If false, the loop terminates.
- { // Code to be executed }: The block of code that runs repeatedly as long as the condition is true.
Basic Example
Here's a simple example of a while
loop that prints numbers from 1 to 5:
#include <stdio.h>
int main() {
int i = 1;
while (i <= 5) {
printf("%d\n", i);
i++;
}
return 0;
}
Explanation:
- The variable i is initialized to 1.
- The
while
loop checks if i is less than or equal to 5. - If true, it prints the value of i and then increments i by 1.
- This process repeats until i is greater than 5.
Infinite while Loop
An infinite loop is a loop that runs indefinitely because the condition never becomes false. Hereβs an example of an infinite while
loop:
#include <stdio.h>
int main() {
while (1) {
printf("This is an infinite loop\n");
}
return 0;
}
π§ Explanation
- The condition 1 (which is always true) causes the loop to run forever.
- Infinite loops are generally used for programs that need to run continuously, such as servers or embedded systems, but should be used with caution to avoid unintentional infinite loops.
while Loop with User Input
Here's an example of using a while
loop to repeatedly prompt the user for input until they enter a specific value:
#include <stdio.h>
int main() {
int number;
printf("Enter a number (0 to exit): ");
scanf("%d", &number);
while (number != 0) {
printf("You entered: %d\n", number);
printf("Enter a number (0 to exit): ");
scanf("%d", &number);
}
printf("Exited the loop.\n");
return 0;
}
π§ Explanation:
- The user is prompted to enter a number.
- The
while
loop continues to execute as long as the entered number is not 0. - If the user enters 0, the loop terminates.
Nested while Loops
You can nest while
loops within each other to handle more complex scenarios. Hereβs an example:
#include <stdio.h>
int main() {
int i = 1;
while (i <= 3) {
int j = 1;
while (j <= 2) {
printf("i = %d, j = %d\n", i, j);
j++;
}
i++;
}
return 0;
}
π§ Explanation:
- The outer loop runs while i is less than or equal to 3.
- The inner loop runs while j is less than or equal to 2.
- This results in a total of 6 iterations (3 outer * 2 inner).
π Common Pitfalls and Best Practices
Ensure Termination:
Always ensure that the condition will eventually become false to avoid infinite loops unless intentionally creating one.
Update Variables Inside the Loop:
Ensure that variables affecting the loop condition are updated within the loop to avoid infinite loops.
Clear and Maintainable Conditions:
Keep loop conditions simple and clear to improve readability and maintainability of your code.
π Conclusion
The while
loop is a versatile and powerful tool in C programming for executing code repetitively based on a condition.
By understanding its syntax and proper usage, you can efficiently control the flow of your programs.
π¨βπ» Join our Community:
Author
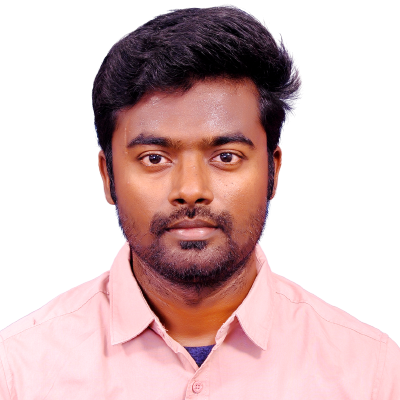
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C while Loop), please comment here. I will help you immediately.