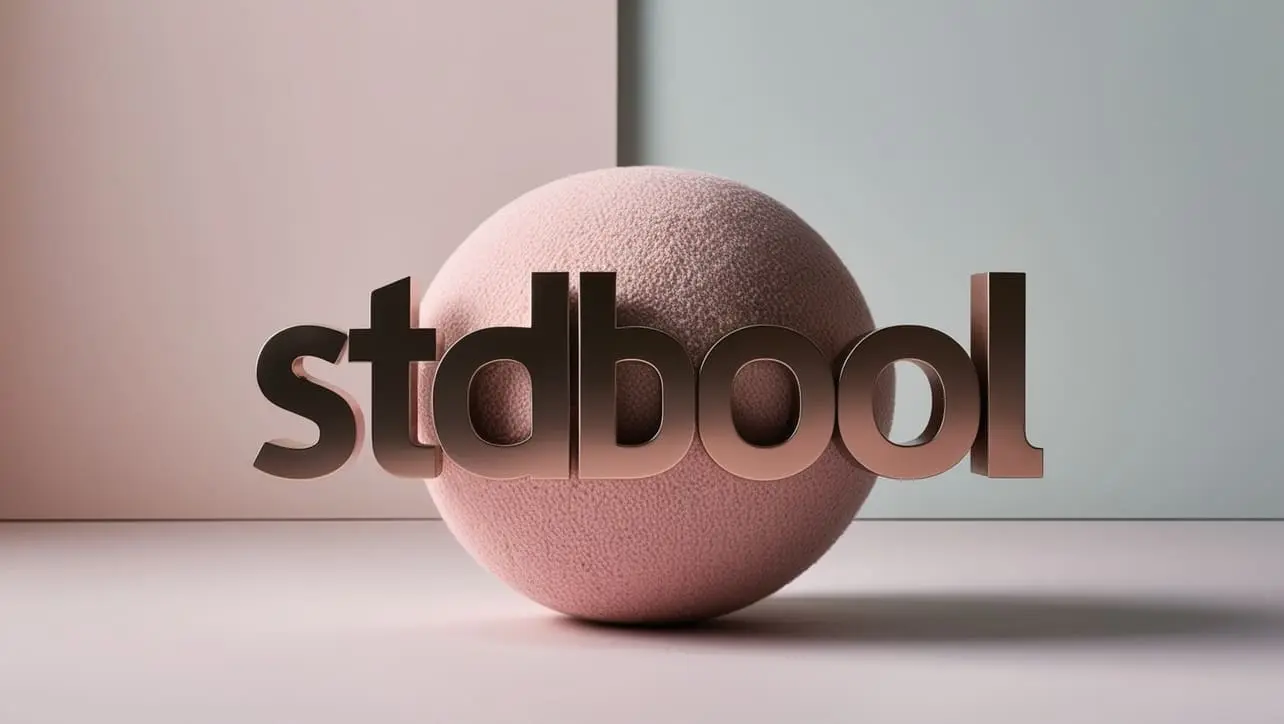
C switch Statement
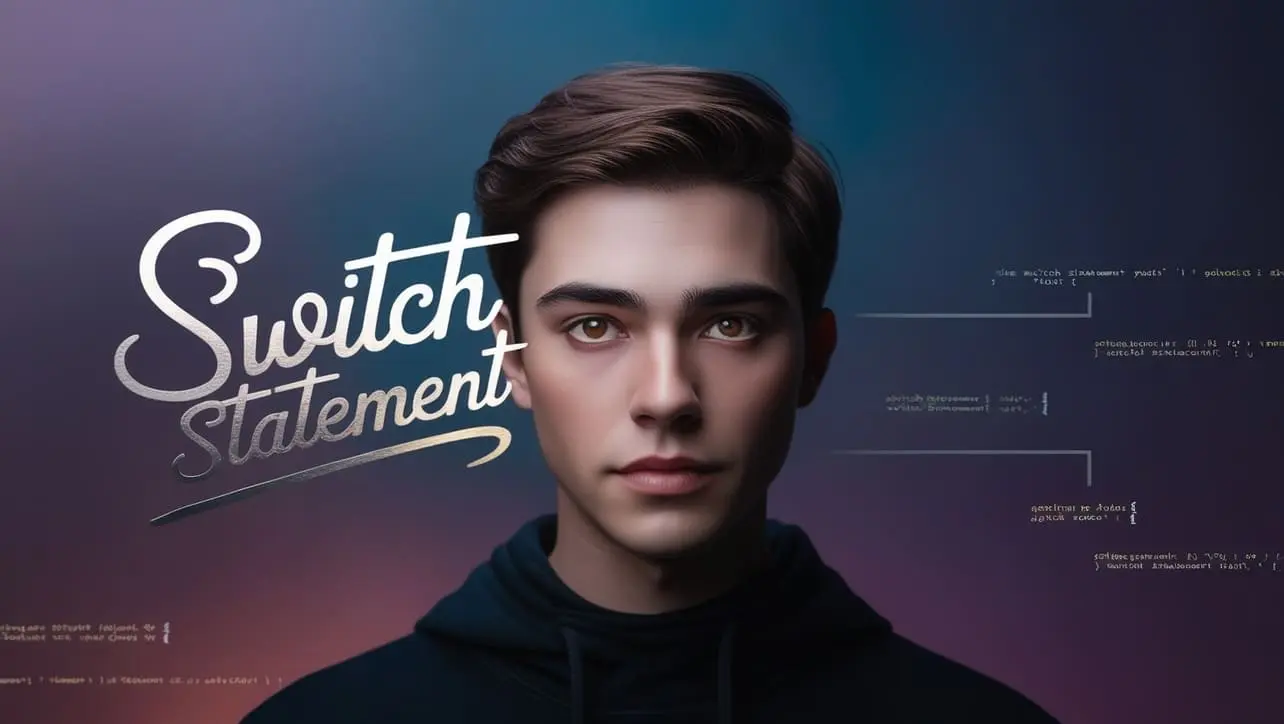
Photo Credit to CodeToFun
Introduction
The switch
statement in C is a powerful control statement that allows you to execute different parts of code based on the value of an expression. It is an alternative to using multiple if-else statements and is particularly useful when you need to compare a variable to multiple possible values.
What is the switch Statement?
In C programming, the switch
statement evaluates an expression and executes the corresponding case block based on the value of the expression. If no matching case is found, the default block is executed if it is present.
Key Features
- Multi-way Branching: Simplifies the process of choosing between multiple actions.
- Readability: Makes code easier to read compared to a long sequence of if-else statements.
- Efficiency: Can be more efficient than if-else chains in terms of execution time.
Syntax
The syntax for the switch
statement is as follows:
switch (expression) {
case value1:
// Statements
break;
case value2:
// Statements
break;
// Additional cases...
default:
// Default statements
}
Explanation:
- switch (expression): The expression is evaluated once and compared against the values of each case.
- case value:: If the expression matches this value, the corresponding statements are executed.
- break;: Exits the switch block. Without break, execution will fall through to the next case.
- default: Optional: Executes if no case matches the expression.
Using the switch Statement:
Hereβs a basic example of a switch
statement:
#include <stdio.h>
int main() {
int day = 3;
switch (day) {
case 1:
printf("Monday\n");
break;
case 2:
printf("Tuesday\n");
break;
case 3:
printf("Wednesday\n");
break;
case 4:
printf("Thursday\n");
break;
case 5:
printf("Friday\n");
break;
case 6:
printf("Saturday\n");
break;
case 7:
printf("Sunday\n");
break;
default:
printf("Invalid day\n");
}
return 0;
}
Output
In this example, if day is 3, the output will be:
Wednesday
The default Case
The default case in a switch
statement is executed if none of the case values match the expression. It is optional but recommended for handling unexpected values.
Example with default Case
#include <stdio.h>
int main() {
int grade = 'B';
switch (grade) {
case 'A':
printf("Excellent!\n");
break;
case 'B':
printf("Well done\n");
break;
case 'C':
printf("Good\n");
break;
case 'D':
printf("You passed\n");
break;
case 'F':
printf("Better try again\n");
break;
default:
printf("Invalid grade\n");
}
return 0;
}
Output
If grade is 'B', the output will be:
Well done
Fall-through Behavior
In C, if you omit the break statement, the program will continue executing the next case statements until it encounters a break or the end of the switch block. This is known as fall-through.
Example of Fall-through
#include <stdio.h>
int main() {
int number = 2;
switch (number) {
case 1:
printf("One\n");
case 2:
printf("Two\n");
case 3:
printf("Three\n");
default:
printf("Default\n");
}
return 0;
}
Output
The output will be:
Two Three Default
Common Pitfalls and Best Practices
Avoid Unintentional Fall-through:
Always use break unless intentional fall-through is required. Unintentional fall-through can lead to logical errors and unexpected behavior.
Use default Case:
Always include a default case to handle unexpected values, making your code more robust and easier to debug.
Limit Complex Logic:
Keep the logic within case blocks simple. If complex operations are required, consider calling functions instead to keep the
switch
statement readable.
Conclusion
The switch
statement is a valuable tool in C programming for handling multiple conditions more elegantly than multiple if-else statements.
By understanding its syntax, usage, and common pitfalls, you can write clearer and more efficient code.
Join our Community:
Author
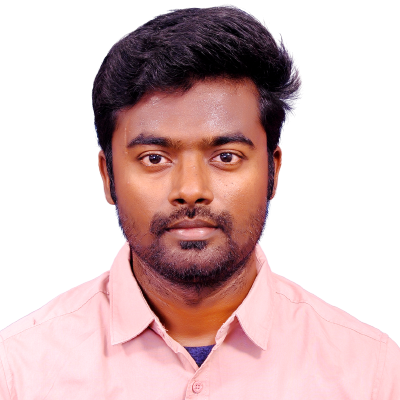
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C switch Statement), please comment here. I will help you immediately.