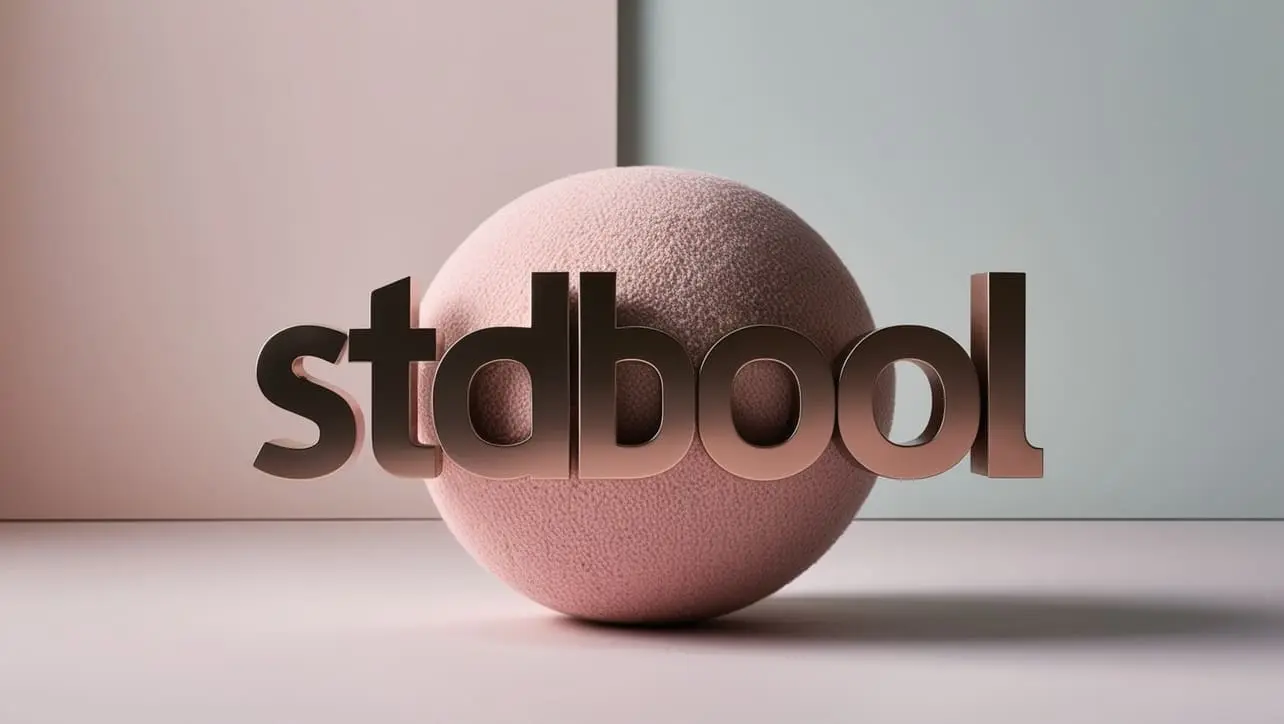
C Basic
C String Functions
- C String Functions
- C strcasecmp()
- C strcat()
- C strncat()
- C strcpy()
- C strncpy()
- C strlen()
- C strcmp()
- C strncmp()
- C stricmp()
- C strchr()
- C strrchr()
- C strstr()
- C strdup()
- C strlwr()
- C strupr()
- C strrev()
- C strset()
- C strnset()
- C strtok()
- C strerror()
- C strpbrk()
- C strcoll()
- C strspn()
- C strcspn()
- C strxfrm()
- C memchr()
- C memmove()
- C memcpy()
- C memcmp()
- C memset()
C strset() Function
.webp)
Photo Credit to CodeToFun
đ Introduction
In the C programming language, string manipulation is a common and important aspect of coding.
The strset()
function is one of the string manipulation functions provided by the C Standard Library. It is used to set every character of a string to a specified character.
In this tutorial, we'll explore the usage and functionality of the strset()
function in C.
đĄ Syntax
The syntax for the strset()
function is as follows:
char *strset(char *str, int character);
- str: A pointer to the null-terminated string to be modified.
- character: The character to set each element of the string to.
đ Example 1
Let's dive into an example to illustrate how the strset()
function works.
Warning: The strset()
function is not a standard C library function, and its availability might vary across different compilers.
#include <stdio.h>
#include <string.h>
int main() {
char myString[] = "Hello, C Programming!";
// Set every character in the string to '*'
strset(myString, '*');
// Output the modified string
printf("Modified String: %s\n", myString);
return 0;
}
đģ Output
Modified String: *********************
đ§ How the Program Works
In this example, the strset()
function is used to set every character in the string "Hello, C Programming!" to '*'.
đ Example 2
Here's an alternative example using a loop through each character in the string to the specified character.
#include <stdio.h>
// Custom strset-like function
char * custom_strset(char * str, int character) {
if (str == NULL) {
// Handle null pointer case
return NULL;
}
char * ptr = str; // Save the original pointer
while ( * str != '\0') {
* str = (char) character; // Set each character to the specified value
str++;
}
return ptr; // Return the original pointer
}
int main() {
char myString[] = "Hello, C Programming!";
// Use the custom_strset function to set every character in the string to '*'
custom_strset(myString, '*');
// Output the modified string
printf("Modified String: %s\n", myString);
return 0;
}
đģ Output
Modified String: *********************
đ§ How the Program Works
In this program, custom_strset() is a custom function that behaves similarly to strset()
. It takes a string and a character as parameters and sets each character in the string to the specified character. The program then uses this custom function to modify a string and prints the result.
âŠī¸ Return Value
The strset()
function returns a pointer to the modified string. The returned pointer is the same as the pointer passed as the str argument.
đ Common Use Cases
The strset()
function is useful when you need to replace or initialize every character in a string with a specific character. It provides a quick and efficient way to perform bulk character assignments within a string.
đ Notes
- The
strset()
function operates on null-terminated strings. Ensure that the input string is properly null-terminated. - It is important to note that the
strset()
function does not change the size of the string; it only modifies the existing characters.
đĸ Optimization
The strset()
function is optimized for setting multiple characters within a string efficiently. However, keep in mind that it operates on individual characters, so its efficiency is linear with the length of the string.
đ Conclusion
The strset()
function in C is a valuable tool for manipulating strings by setting each character to a specified value. It is particularly handy for tasks that involve initializing or resetting string contents.
Feel free to experiment with different strings and characters to deepen your understanding of the strset()
function. Happy coding!
đ¨âđģ Join our Community:
Author
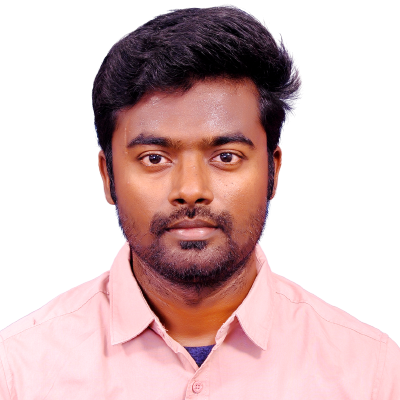
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C strset() Function) please comment here. I will help you immediately.