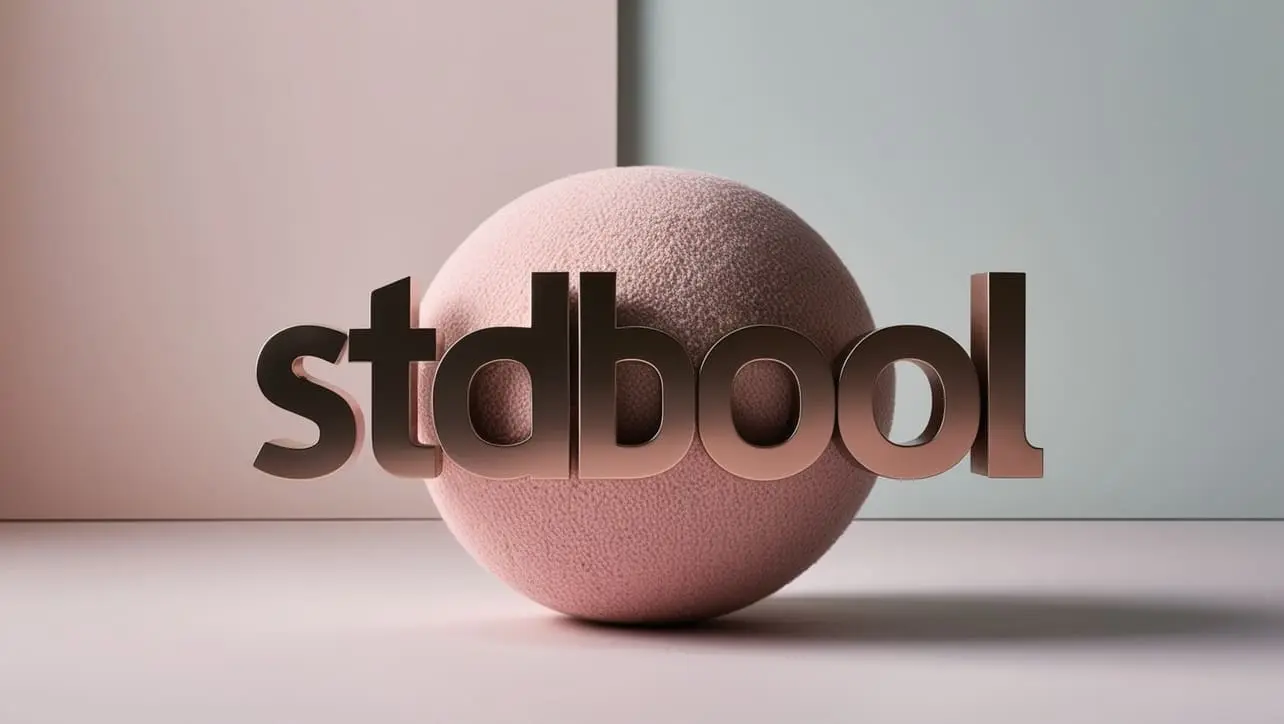
C Basic
C String Functions
- C String Functions
- C strcasecmp()
- C strcat()
- C strncat()
- C strcpy()
- C strncpy()
- C strlen()
- C strcmp()
- C strncmp()
- C stricmp()
- C strchr()
- C strrchr()
- C strstr()
- C strdup()
- C strlwr()
- C strupr()
- C strrev()
- C strset()
- C strnset()
- C strtok()
- C strerror()
- C strpbrk()
- C strcoll()
- C strspn()
- C strcspn()
- C strxfrm()
- C memchr()
- C memmove()
- C memcpy()
- C memcmp()
- C memset()
C strlwr() Function
.webp)
Photo Credit to CodeToFun
đ Introduction
In C programming, manipulating strings is a common and essential task.
The strlwr()
function is a library function provided by <string.h> that converts each uppercase letter in a string to its corresponding lowercase letter.
In this tutorial, we'll explore the usage and functionality of the strlwr()
function in C.
đĄ Syntax
The syntax for the strlwr()
function is as follows:
char *strlwr(char *str);
- str: The string to be converted to lowercase.
đ Example 1
Let's dive into an example to illustrate how the strlwr()
function works.
Warning: The strlwr()
function is not part of the standard C library and is not supported by all compilers.
#include <stdio.h>
#include <string.h>
int main() {
char original[] = "Hello, C Programming!";
char * lowercase;
// Copy the original string to avoid modifying it directly
char buffer[50];
strcpy(buffer, original);
// Convert the string to lowercase
lowercase = strlwr(buffer);
// Output the result
printf("Original String: %s\n", original);
printf("Lowercase String: %s\n", lowercase);
return 0;
}
đģ Output
Original String: Hello, C Programming! Lowercase String: hello, c programming!
đ§ How the Program Works
In this example, the strlwr()
function is used to convert the string "Hello, C Programming!" to lowercase, and the result is then printed.
đ Example 2
In this example, we will consider using an alternative approach or implementing your own function to convert a string to lowercase. Here's an example using a simple function:
#include <stdio.h>
#include <ctype.h>
#include <string.h>
void strToLower(char * str) {
for (int i = 0; str[i]; i++) {
str[i] = tolower(str[i]);
}
}
int main() {
char original[] = "Hello, C Programming!";
char lowercase[50];
// Copy the original string to avoid modifying it directly
strcpy(lowercase, original);
// Convert the string to lowercase
strToLower(lowercase);
// Output the result
printf("Original String: %s\n", original);
printf("Lowercase String: %s\n", lowercase);
return 0;
}
đģ Output
Original String: Hello, C Programming! Lowercase String: hello, c programming!
đ§ How the Program Works
In this example, the strToLower() function is defined to convert each character of the string to lowercase using the tolower() function.
This should work on most C compilers. If you specifically need the strlwr()
function and your compiler supports it, you might need to enable certain features or include specific headers depending on your compiler and platform.
âŠī¸ Return Value
The strlwr()
function returns a pointer to the modified string. It directly modifies the input string and returns the same pointer.
đ Common Use Cases
The strlwr()
function is particularly useful when you need to standardize the case of characters in a string. It's commonly employed in scenarios where case-insensitivity is required for comparison operations.
đ Notes
- The
strlwr()
function is not a standard C library function and might not be available on all platforms. Consider using platform-independent alternatives if portability is a concern. - This function modifies the original string, so be cautious if you need to preserve the original content.
đĸ Optimization
The strlwr()
function itself is a simple operation and doesn't require optimization. However, for larger strings or frequent use, consider optimizing the overall string manipulation code.
đ Conclusion
The strlwr()
function in C is a practical tool for converting a string to lowercase. It provides a convenient way to handle case-insensitive string comparisons and is a valuable asset in string manipulation tasks.
Feel free to experiment with different strings and explore the behavior of the strlwr()
function in various scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
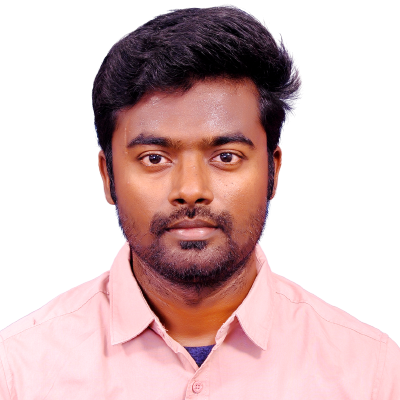
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C strlwr() Function) please comment here. I will help you immediately.