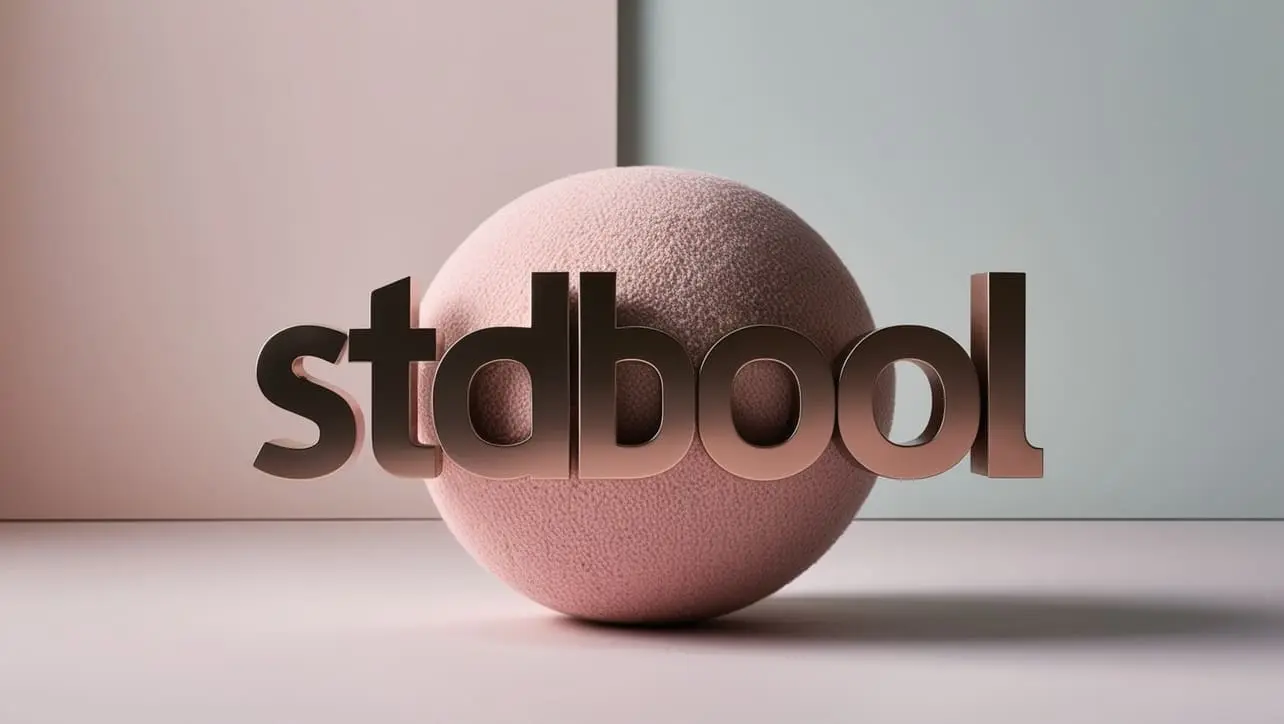
C Basic
C String Functions
- C String Functions
- C strcasecmp()
- C strcat()
- C strncat()
- C strcpy()
- C strncpy()
- C strlen()
- C strcmp()
- C strncmp()
- C stricmp()
- C strchr()
- C strrchr()
- C strstr()
- C strdup()
- C strlwr()
- C strupr()
- C strrev()
- C strset()
- C strnset()
- C strtok()
- C strerror()
- C strpbrk()
- C strcoll()
- C strspn()
- C strcspn()
- C strxfrm()
- C memchr()
- C memmove()
- C memcpy()
- C memcmp()
- C memset()
C strerror() Function
.webp)
Photo Credit to CodeToFun
đ Introduction
In C programming, error handling is a critical aspect of writing robust and reliable code.
The strerror()
function is a part of the C Standard Library (header file: <errno.h>) and plays a crucial role in error reporting.
It takes an error number as an argument and returns a pointer to the textual representation of the corresponding error message.
In this tutorial, we'll explore the usage and functionality of the strerror()
function in C.
đĄ Syntax
The syntax for the strerror()
function is as follows:
char *strerror(int errnum);
- errnum: An integer representing the error number for which you want the corresponding error message.
đ Example
Let's dive into an example to illustrate how the strerror()
function works.
#include <stdio.h>
#include <string.h>
#include <errno.h>
int main() {
// Simulating an error condition (e.g., opening a non-existent file)
FILE * file = fopen("nonexistent.txt", "r");
// Check for error
if (file == NULL) {
// Get the error number
int errorNumber = errno;
// Get the error message using strerror()
const char * errorMessage = strerror(errorNumber);
// Output the error message
printf("Error opening file: %s\n", errorMessage);
}
return 0;
}
đģ Output
Error opening file: No such file or directory
đ§ How the Program Works
In this example, the strerror()
function is used to obtain the textual representation of the error message corresponding to the error number obtained from errno. This is a common usage scenario for error handling in C.
âŠī¸ Return Value
The strerror()
function returns a pointer to a string containing the textual representation of the error message. The string is part of the internal memory of the C library and should not be modified.
đ Common Use Cases
The strerror()
function is particularly useful in scenarios where you need to provide meaningful error messages to users or log detailed error information during debugging. It enhances the interpretability of error conditions in your C programs.
đ Notes
- The
strerror()
function is thread-safe in most implementations. - Ensure that you include the <errno.h> header to use the
strerror()
function. - Common error numbers include EINVAL (Invalid argument), ENOMEM (Out of memory), and EIO (Input/output error), among others.
đĸ Optimization
The strerror()
function itself is optimized for efficiency. However, for performance-critical applications, ensure that error handling is used judiciously to avoid unnecessary overhead.
đ Conclusion
The strerror()
function in C is a valuable tool for converting error numbers into human-readable error messages. It is an essential component of robust error handling in C programs.
Feel free to experiment with different error scenarios and leverage strerror()
to enhance the error reporting capabilities of your C applications. Happy coding!
đ¨âđģ Join our Community:
Author
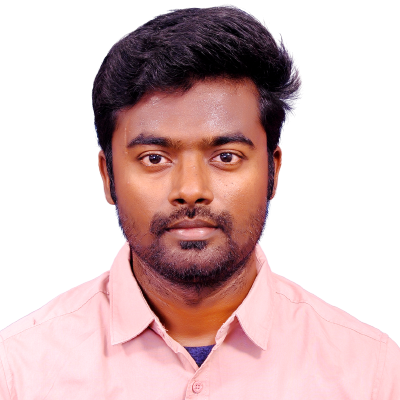
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C strerror() Function) please comment here. I will help you immediately.