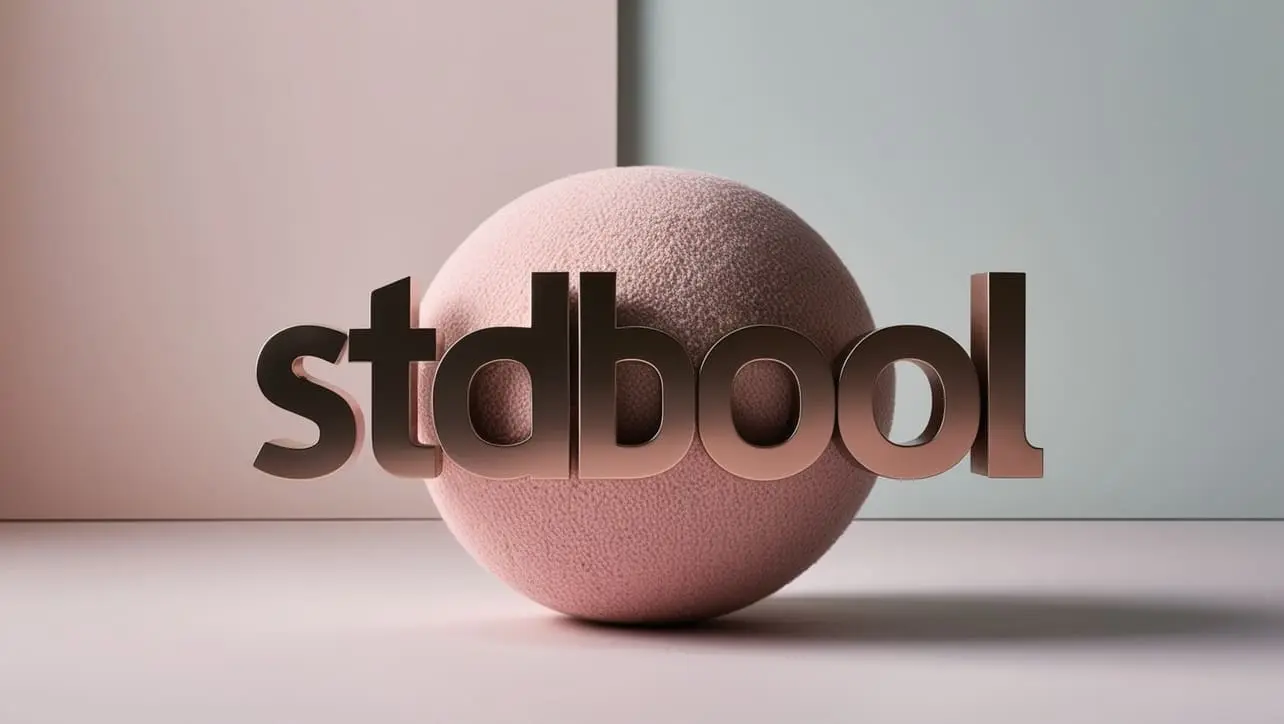
C Basic
C String Functions
- C String Functions
- C strcasecmp()
- C strcat()
- C strncat()
- C strcpy()
- C strncpy()
- C strlen()
- C strcmp()
- C strncmp()
- C stricmp()
- C strchr()
- C strrchr()
- C strstr()
- C strdup()
- C strlwr()
- C strupr()
- C strrev()
- C strset()
- C strnset()
- C strtok()
- C strerror()
- C strpbrk()
- C strcoll()
- C strspn()
- C strcspn()
- C strxfrm()
- C memchr()
- C memmove()
- C memcpy()
- C memcmp()
- C memset()
C strcspn() Function
.webp)
Photo Credit to CodeToFun
đ Introduction
In C programming, strings are manipulated using various functions provided by the standard library.
The strcspn()
function is one such function that calculates the length of the initial segment of a string that consists of characters not in a specified set.
In this tutorial, we'll explore the usage and functionality of the strcspn()
function in C.
đĄ Syntax
The syntax for the strcspn()
function is as follows:
size_t strcspn(const char *str1, const char *str2);
- str1: The string to be searched.
- str2: The set of characters to search for in str1.
đ Example
Let's dive into an example to illustrate how the strcspn()
function works.
#include <stdio.h>
#include <string.h>
int main() {
const char * string = "Hello, World!";
const char * charset = "aeiou";
// Calculate the length of the initial segment without vowels
size_t length = strcspn(string, charset);
// Output the result
printf("Length of initial segment without vowels: %zu\n", length);
return 0;
}
đģ Output
Length of initial segment without vowels: 1
đ§ How the Program Works
In this example, the strcspn()
function is used to calculate the length of the initial segment of the string "Hello, World!" that does not contain any vowels ('a', 'e', 'i', 'o', 'u').
âŠī¸ Return Value
The strcspn()
function returns the length of the initial segment of the string that does not contain any characters from the specified set.
đ Common Use Cases
The strcspn()
function is particularly useful when you need to find the length of a prefix substring that does not contain certain characters. It is commonly used for input validation and parsing tasks.
đ Notes
- If the set of characters is an empty string, the function returns the length of the entire string.
- The time complexity of
strcspn()
is linear with respect to the length of the string.
đĸ Optimization
The strcspn()
function is already optimized for efficiency. However, consider optimizing your algorithm if you are repeatedly calling this function in performance-critical sections of your code.
đ Conclusion
The strcspn()
function in C is a valuable tool for determining the length of the initial segment of a string that does not contain certain characters. It provides a simple and efficient way to perform such substring analysis.
Feel free to experiment with different strings and character sets to explore the behavior of the strcspn()
function in various scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
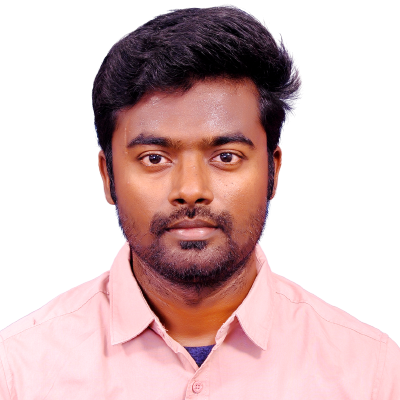
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C strcspn() Function) please comment here. I will help you immediately.