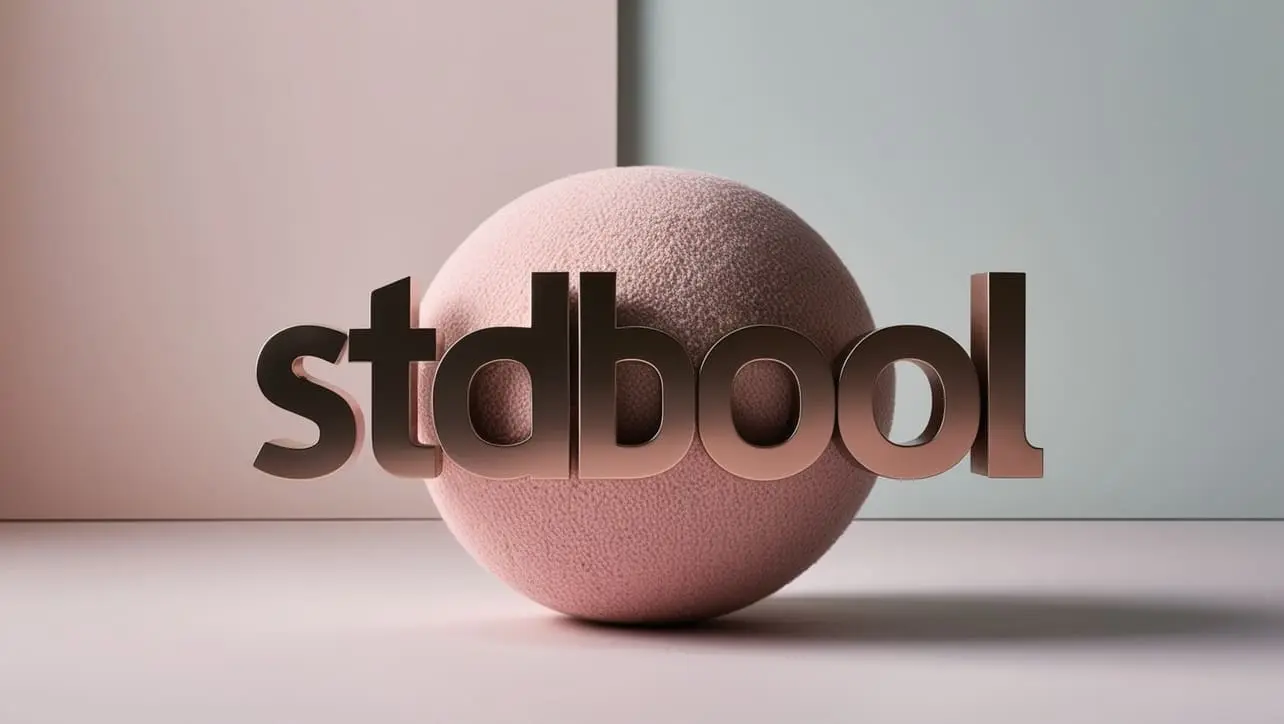
C memset() Function
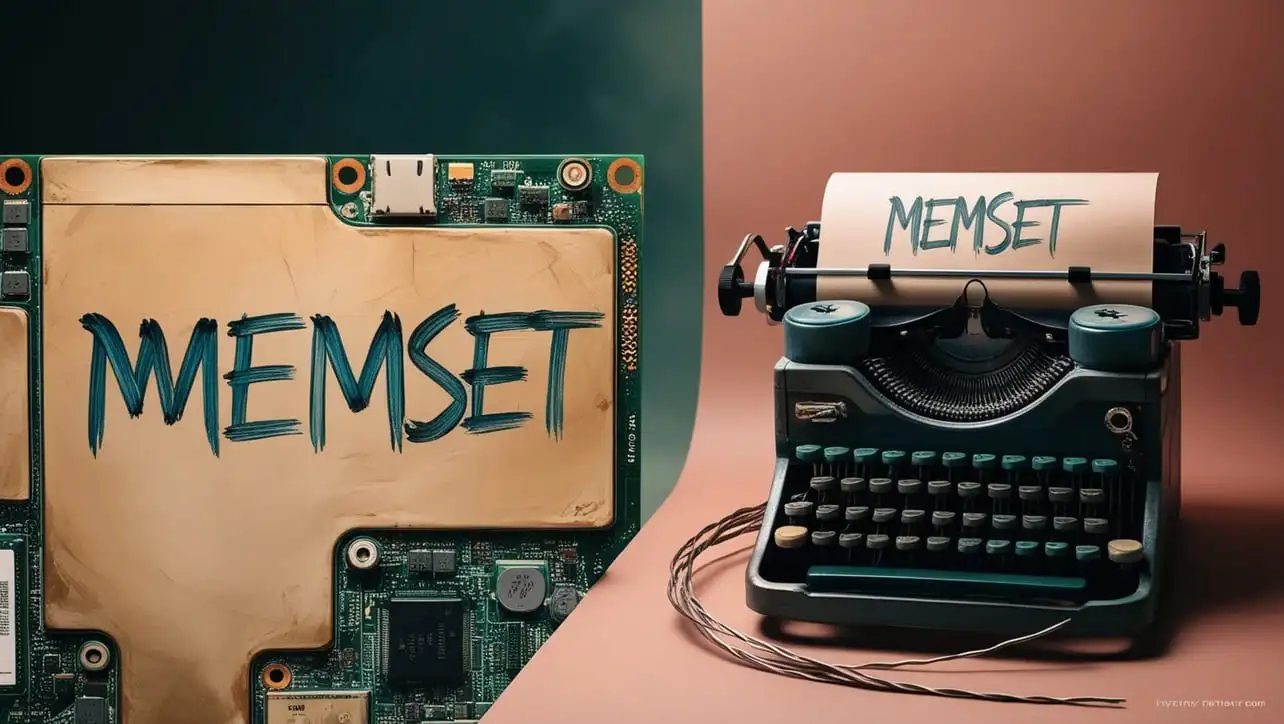
Photo Credit to CodeToFun
đ Introduction
In C programming, the memset()
function is a powerful tool for manipulating memory.
It is commonly used to set a block of memory to a particular value. When applied to strings, memset()
is often employed to initialize or reset the contents of character arrays.
In this tutorial, we'll explore the usage and functionality of the memset()
function in C with a focus on strings.
đĄ Syntax
The syntax for the memset()
function is as follows:
void *memset(void *ptr, int value, size_t num);
- ptr: A pointer to the memory block to be set.
- value: The value to be set. It is typically an unsigned char value, which can represent any byte value.
- num: The number of bytes to be set to the value.
đ Example
Let's dive into an example to illustrate how the memset()
function works with strings.
#include <stdio.h>
#include <string.h>
int main() {
char str[20];
// Using memset to initialize the string
memset(str, 'A', sizeof(str));
// Output the initialized string
printf("Initialized String: %s\n", str);
return 0;
}
đģ Output
Initialized String: AAAAAAAAAAAAAAAAAAAA
đ§ How the Program Works
In this example, the memset()
function is used to set all elements in the character array str to the value 'A'. The result is an initialized string.
âŠī¸ Return Value
The memset()
function returns a pointer to the memory block it was used to fill (ptr). This return value is often not used in practice.
đ Common Use Cases
The memset()
function is particularly useful when you need to initialize arrays, especially character arrays that represent strings. It provides a concise way to set a block of memory to a specific value.
đ Notes
- It's important to note that
memset()
works with bytes, so when initializing strings, make sure to use the ASCII values of the characters you want to set. - Be cautious when using
memset()
with non-character arrays, as setting memory blocks to arbitrary values may lead to unexpected behavior.
đĸ Optimization
The memset()
function is optimized for performance and is a standard library function. There is usually no need for manual optimization. However, for very large memory blocks, consider the efficiency of the algorithm used by the standard library on your specific platform.
đ Conclusion
The memset()
function in C is a versatile tool for setting memory blocks to a specific value. When working with strings, it offers a concise way to initialize character arrays. Understanding how to use memset()
is valuable for efficient memory manipulation in C programming.
Feel free to experiment with different values and sizes to deepen your understanding of the memset()
function. Happy coding!
đ¨âđģ Join our Community:
Author
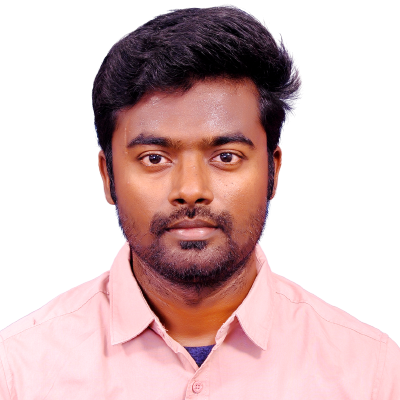
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C memset() Function) please comment here. I will help you immediately.