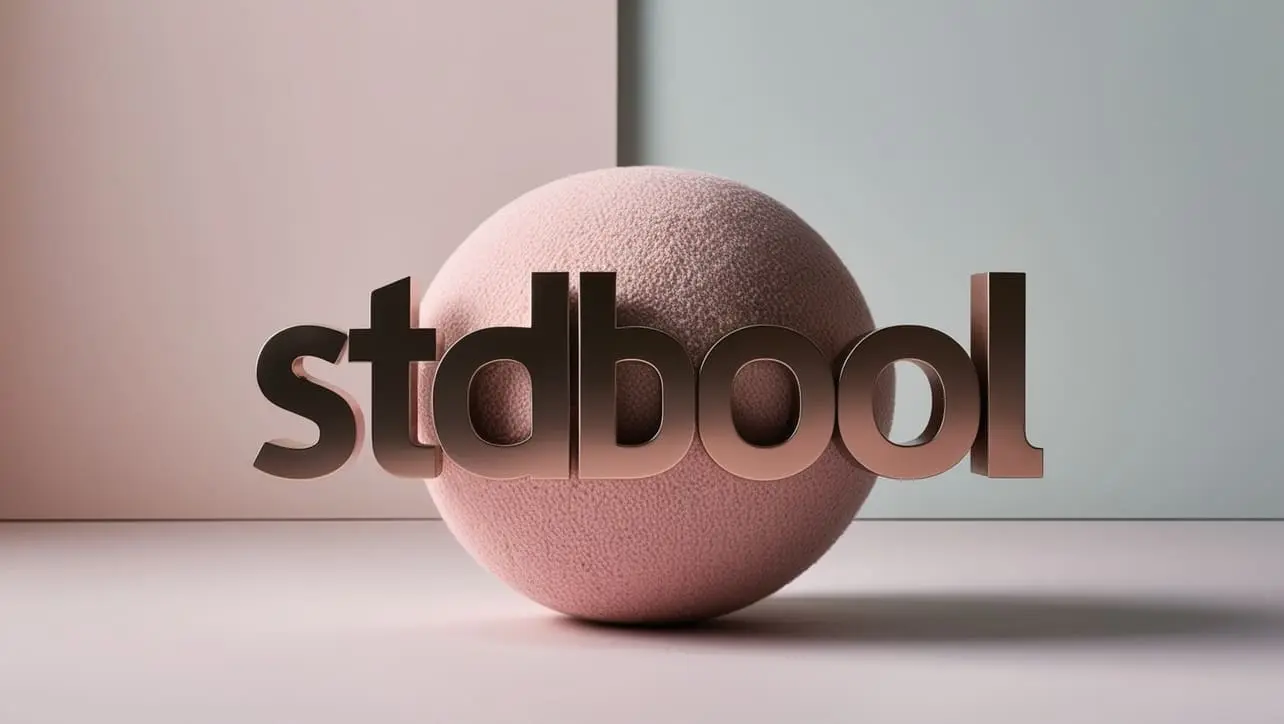
C Basic
C String Functions
- C String Functions
- C strcasecmp()
- C strcat()
- C strncat()
- C strcpy()
- C strncpy()
- C strlen()
- C strcmp()
- C strncmp()
- C stricmp()
- C strchr()
- C strrchr()
- C strstr()
- C strdup()
- C strlwr()
- C strupr()
- C strrev()
- C strset()
- C strnset()
- C strtok()
- C strerror()
- C strpbrk()
- C strcoll()
- C strspn()
- C strcspn()
- C strxfrm()
- C memchr()
- C memmove()
- C memcpy()
- C memcmp()
- C memset()
C memchr() Function
.webp)
Photo Credit to CodeToFun
đ Introduction
In C programming, the memchr()
function is part of the C Standard Library and is used for searching a byte sequence within a given memory block.
This function is particularly useful when working with strings and arrays of characters.
In this tutorial, we'll explore the usage and functionality of the memchr()
function in C.
đĄ Syntax
The signature of the memchr()
function is as follows:
void *memchr(const void *str, int c, size_t n);
- str: Pointer to the memory block to be examined.
- c: Value to be searched, cast to an unsigned char.
- n: Number of bytes to be examined.
đ Example
Let's delve into an example to illustrate how the memchr()
function works.
#include <stdio.h>
#include <string.h>
int main() {
const char * str = "Hello, C Programming!";
char searchChar = 'C';
// Search for 'C' in the string
void * result = memchr(str, searchChar, strlen(str));
if (result != NULL) {
printf("'%c' found at position %ld.\n", searchChar, (char * ) result - str + 1);
} else {
printf("'%c' not found in the string.\n", searchChar);
}
return 0;
}
đģ Output
'C' found at position 8.
đ§ How the Program Works
In this example, the memchr()
function is used to search for the character 'C' within the string "Hello, C Programming!".
âŠī¸ Return Value
The memchr()
function returns a pointer to the first occurrence of the specified value within the given memory block. If the value is not found, it returns NULL.
đ Common Use Cases
The memchr()
function is particularly useful when you need to search for a specific byte value within a block of memory. It can be employed for tasks such as parsing strings or validating the presence of certain characters.
đ Notes
- The
memchr()
function is not limited to strings; it can be applied to any block of memory. - For strings, consider using the strchr() function, which is specifically designed for null-terminated strings.
đĸ Optimization
The memchr()
function is optimized for performance and is part of the standard C library. No specific optimizations are typically needed.
đ Conclusion
The memchr()
function in C provides a reliable means of searching for a byte value within a specified memory block. Whether used for strings or general memory operations, this function is a valuable tool in C programming.
Feel free to experiment with different strings, characters, and memory blocks to deepen your understanding of the memchr()
function. Happy coding!
đ¨âđģ Join our Community:
Author
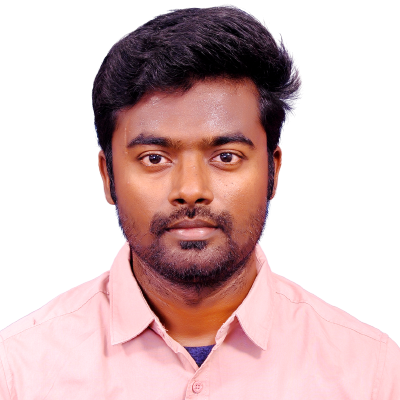
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C memchr() Function) please comment here. I will help you immediately.