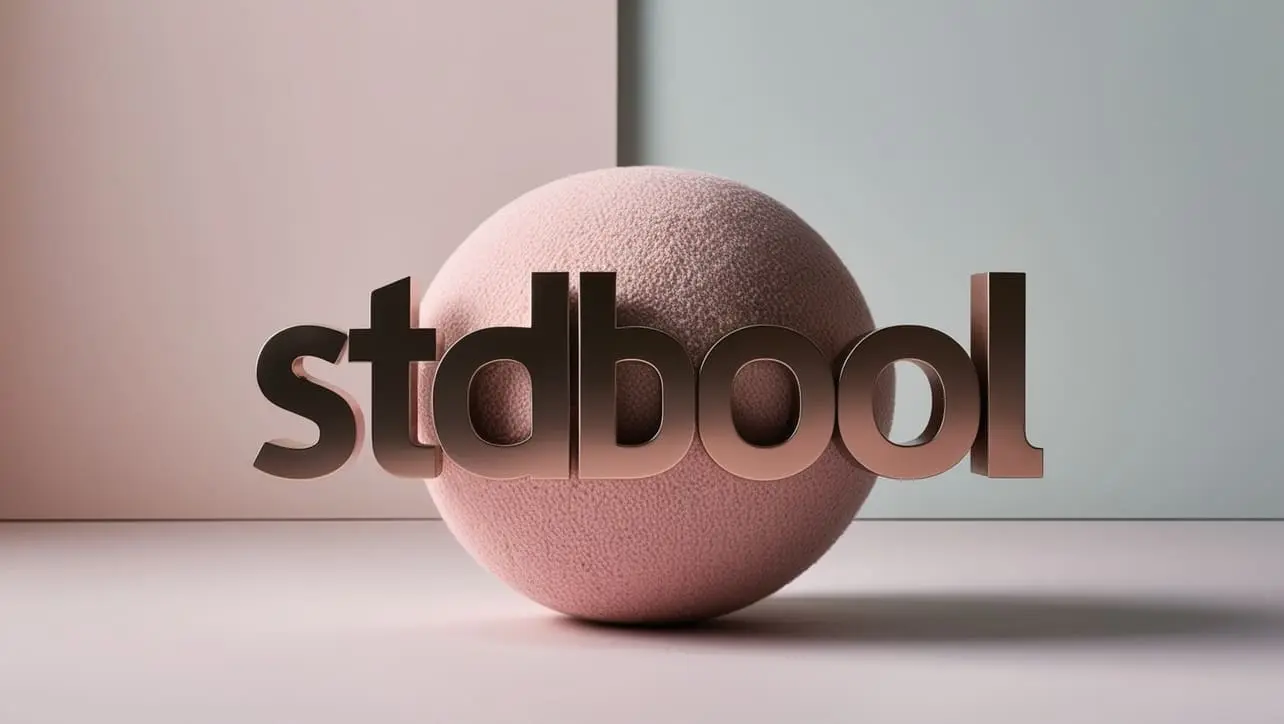
C Library – stdio.h
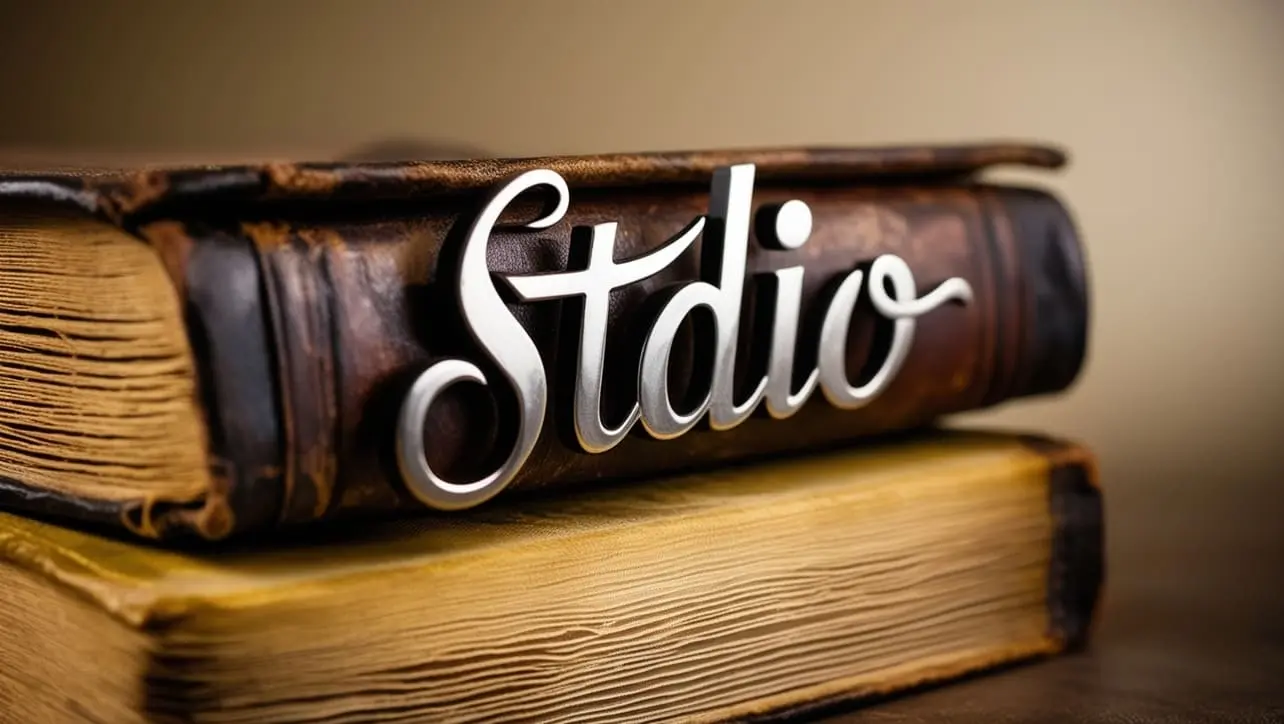
Photo Credit to CodeToFun
π Introduction
The <stdio.h>
header file in C is one of the most commonly used and essential libraries. It provides functionalities for input and output operations, making it a crucial part of any C programmer's toolkit.
This guide will give you an in-depth look at what <stdio.h>
offers, its key functions, and how to use them effectively.
π― Purpose of <stdio.h>
<stdio.h>
stands for Standard Input Output Header. It contains definitions of macros, constants, and declarations of functions and types used for performing input and output operations in C.
π Key Features
- Standard I/O Functions: Provides functions for reading and writing data.
- File Handling: Supports file operations such as opening, reading, writing, and closing files.
- Buffer Management: Includes functions for managing input and output buffers.
π Common Functions
Here are some of the most commonly used functions provided by <stdio.h>
:
- printf: Prints formatted output to the standard output (usually the screen).
- scanf: Reads formatted input from the standard input (usually the keyboard).
- fopen: Opens a file and returns a file pointer.
- fclose: Closes an opened file.
- fread: Reads data from a file.
- fwrite: Writes data to a file.
- fgets: Reads a string from a file.
- fputs: Writes a string to a file.
- fscanf: Reads formatted input from a file.
- fprintf: Prints formatted output to a file.
π Example Usage
printf and scanf
These functions are used for standard input and output operations.
#include <stdio.h>
int main() {
int num;
printf("Enter an integer: ");
scanf("%d", &num);
printf("You entered: %d\n", num);
return 0;
}
π» Output
Enter an integer: 36 You entered: 36
File Operations
The <stdio.h>
library provides robust functions for file handling, allowing you to read from and write to files.
Example: Reading from a File
#include <stdio.h>
int main() {
FILE *file;
char buffer[100];
file = fopen("example.txt", "r");
if (file == NULL) {
perror("Error opening file");
return -1;
}
while (fgets(buffer, 100, file) != NULL) {
printf("%s", buffer);
}
fclose(file);
return 0;
}
Example: Writing to a File
#include <stdio.h>
int main() {
FILE *file;
file = fopen("example.txt", "w");
if (file == NULL) {
perror("Error opening file");
return -1;
}
fprintf(file, "Hello, World!\n");
fclose(file);
return 0;
}
Buffer Management
The <stdio.h>
library also includes functions for managing input and output buffers, which can optimize performance by reducing the number of I/O operations.
Example: Flushing the Output Buffer
#include <stdio.h>
int main() {
printf("This will be printed immediately.\n");
fflush(stdout); // Flush the output buffer
return 0;
}
Constants and Macros
<stdio.h>
defines several constants and macros that are useful for file operations and error handling:
- EOF: Indicates the end of a file.
- NULL: Represents a null pointer.
- FOPEN_MAX: Maximum number of files that can be opened simultaneously.
- FILENAME_MAX: Maximum length of a filename.
Error Handling
When performing I/O operations, itβs crucial to handle errors gracefully. The <stdio.h>
library provides several functions to assist with this:
- perror: Prints a descriptive error message to stderr.
- feof: Checks if the end of a file has been reached.
- ferror: Checks for errors in a file stream.
- clearerr: Clears the error and end-of-file indicators for a file stream.
Example: Handling File Errors
#include <stdio.h>
int main() {
FILE *file;
file = fopen("nonexistent.txt", "r");
if (file == NULL) {
perror("Error opening file");
return -1;
}
// Perform file operations
fclose(file);
return 0;
}
π Conclusion
The <stdio.h>
header file is a cornerstone of C programming, providing essential functions for input and output operations.
Understanding how to use its functions effectively can greatly enhance your ability to perform file handling, manage I/O buffers, and handle errors in your C programs.
π¨βπ» Join our Community:
Author
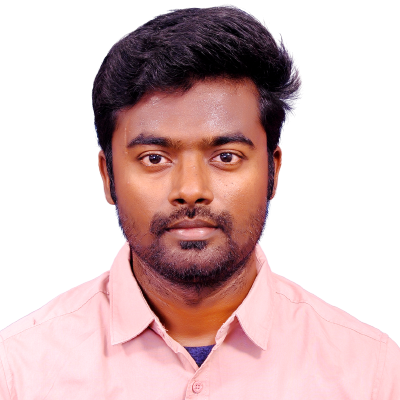
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C Library β stdio.h), please comment here. I will help you immediately.