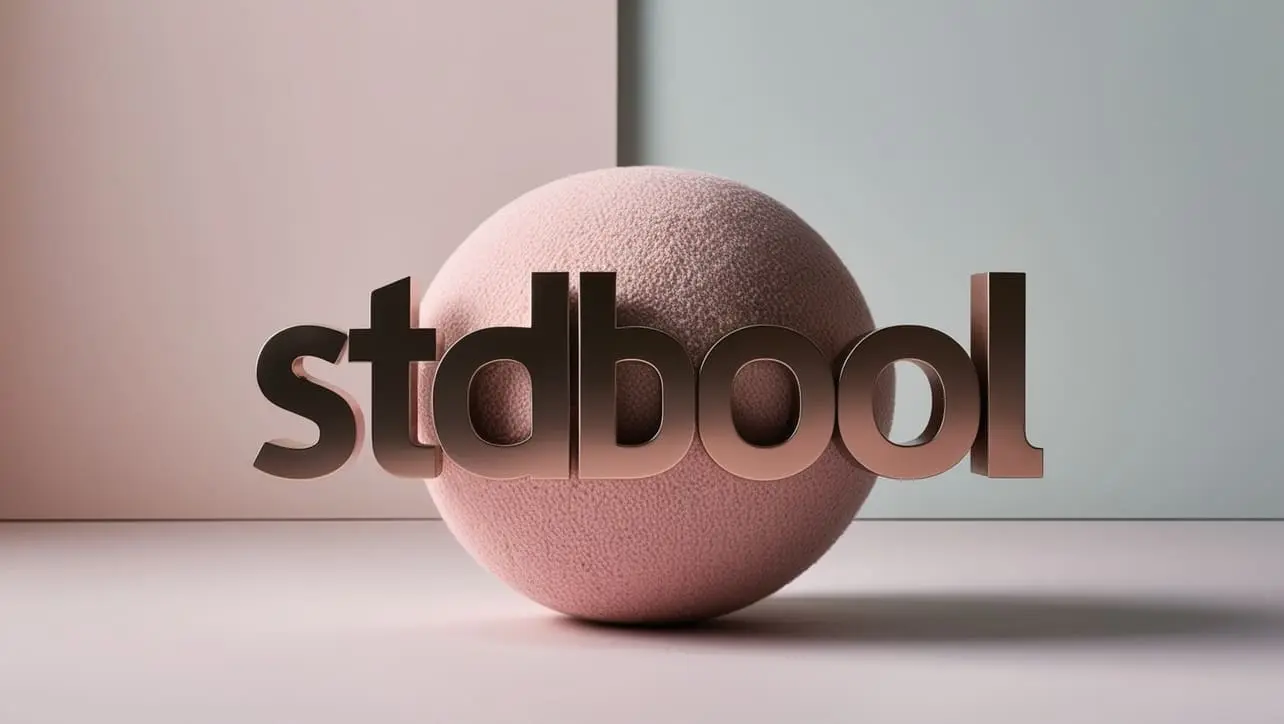
C Library – stdint.h
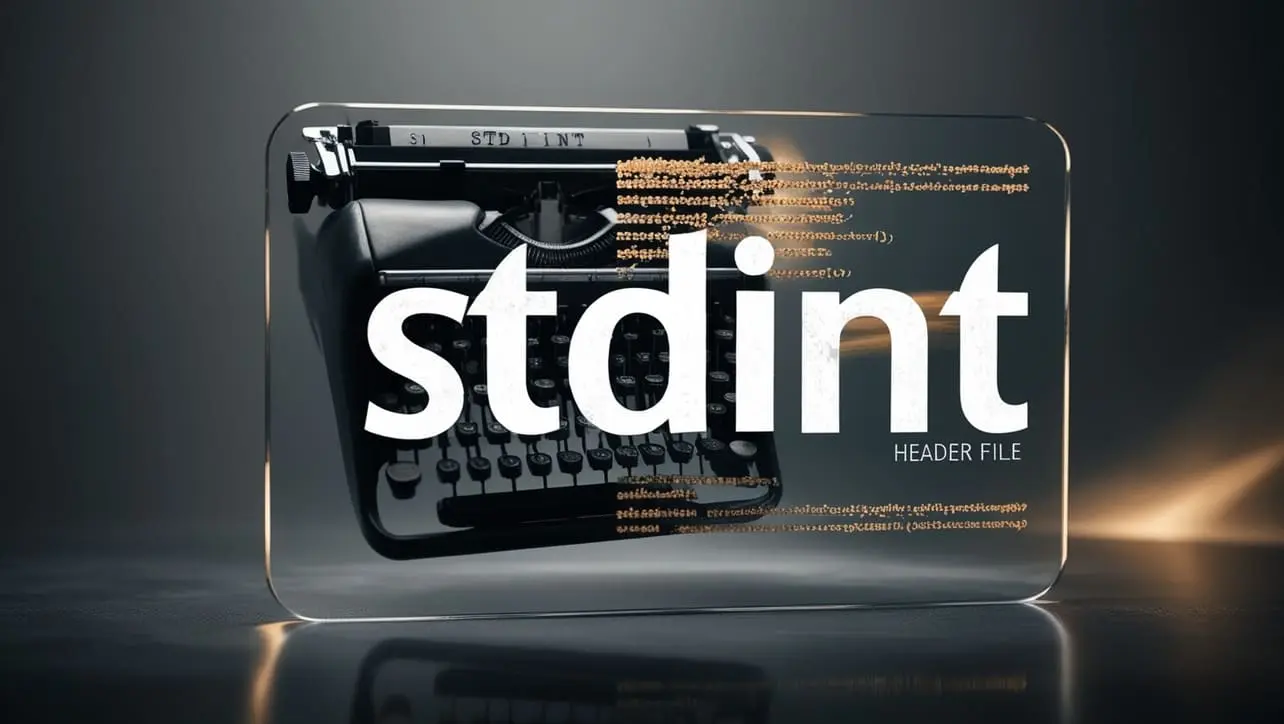
Photo Credit to CodeToFun
🙋 Introduction
The <stdint.h>
header file in C provides a set of typedefs that specify exact-width integer types. These types are particularly useful when you need integers with guaranteed sizes regardless of the underlying platform.
This guide will explore the purpose of <stdint.h>
, its usage, and practical examples.
🎯 Purpose of <stdint.h>
In C programming, the sizes of integer types (such as int, short, long, etc.) can vary depending on the compiler and platform. This variability can lead to portability issues when working with programs that rely on specific integer sizes.
The <stdint.h>
header file addresses this problem by providing integer types with guaranteed sizes, ensuring consistent behavior across different systems.
🔑 Key Features
- Exact-width Integers: Provides integer types with precise widths (in bits).
- Portable Code: Ensures consistent behavior across different platforms.
- Code Clarity: Improves code readability by specifying integer sizes explicitly.
📈 Usage of <stdint.h>
To use the integer types defined in <stdint.h>
, simply include the header file at the beginning of your C program:
#include <stdint.h>
Once included, you can use the defined types in your program.
🔍 Common Integer Types
Here are some of the commonly used integer types provided by <stdint.h>
:
- int8_t: 8-bit signed integer.
- uint8_t: 8-bit unsigned integer.
- int16_t: 16-bit signed integer.
- uint16_t: 16-bit unsigned integer.
- int32_t: 32-bit signed integer.
- uint32_t: 32-bit unsigned integer.
- int64_t: 64-bit signed integer.
- uint64_t: 64-bit unsigned integer.
📝 Example Usage
Here's an example demonstrating the usage of <stdint.h>
types:
#include <stdio.h>
#include <stdint.h>
int main() {
int32_t myInt = 42;
uint16_t myUnsignedInt = 65535;
printf("Signed Integer: %d\n", myInt);
printf("Unsigned Integer: %u\n", myUnsignedInt);
return 0;
}
💻 Output
In this example, int32_t ensures that myInt is a 32-bit signed integer, and uint16_t ensures that myUnsignedInt is a 16-bit unsigned integer.
Signed Integer: 42 Unsigned Integer: 65535
💰 Benefits of <stdint.h>
- Portability: Code using
<stdint.h>
is more portable across different platforms. - Explicitness: The use of exact-width integer types makes code clearer and less error-prone.
- Standardization: Provides a standardized way to define integer types with specific widths.
📚 Common Pitfalls and Best Practices
Avoid Mixing Types:
Avoid mixing
<stdint.h>
types with standard C types (int, short, etc.) to maintain consistency and avoid confusion.Use Appropriate Types:
Choose the appropriate
<stdint.h>
type based on the required size and signedness of the integer.Consider Compatibility:
Be mindful of the target platform's support for
<stdint.h>
types, especially in embedded systems or older compilers.
🎉 Conclusion
The <stdint.h>
header file in C provides a standardized set of integer types with exact widths, ensuring portability and clarity in code.
By using <stdint.h>
types, you can write more robust and maintainable C programs, particularly in situations where integer sizes are critical.
👨💻 Join our Community:
Author
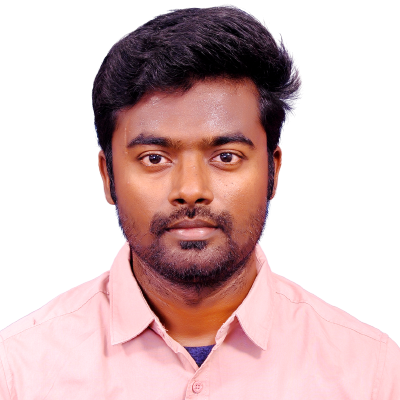
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C Library – stdint.h), please comment here. I will help you immediately.