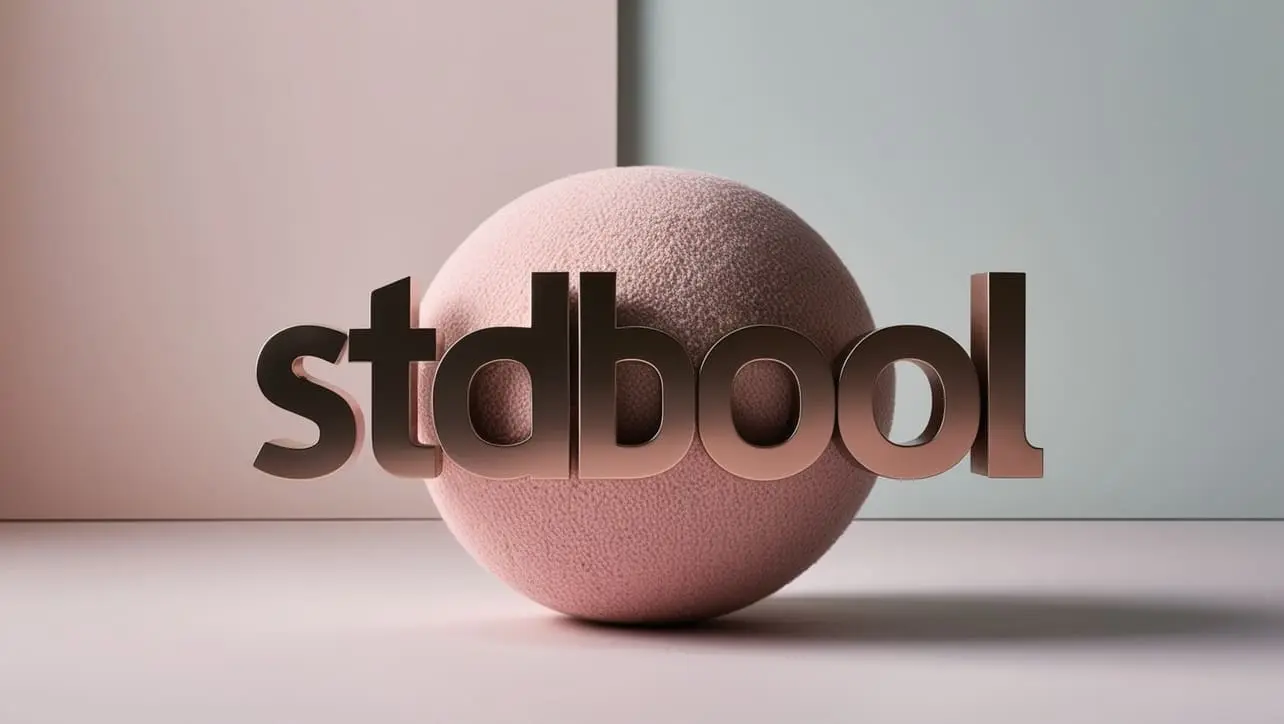
C Header Files
C Library – stdbool.h
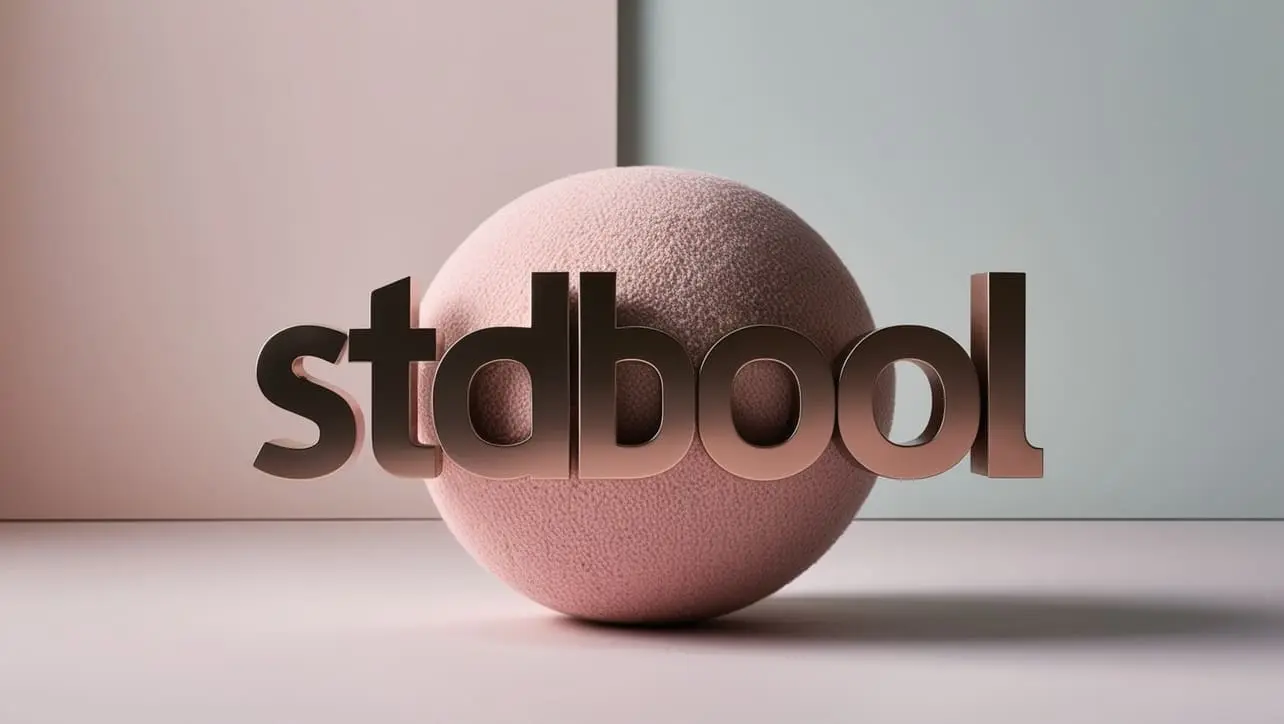
Photo Credit to CodeToFun
🙋 Introduction
The stdbool.h
header in C provides a way to work with boolean data types, which represent true and false values. This header defines the bool type and the constants true
and false
, making C programs more readable and easier to understand by introducing a standard way to handle boolean values.
💡 Syntax
To use the stdbool.h
library, you need to include it in your program:
#include <stdbool.h>
Definitions in stdbool.h
The stdbool.h
header defines the following:
- bool: A macro that expands to _Bool, the built-in boolean type in C.
- true: A macro that expands to the integer constant 1, representing a true value.
- false: A macro that expands to the integer constant 0, representing a false value.
Syntax of the Definitions
bool:
example.cCopiedbool variable_name;
`true` and `false`:
example.cCopiedbool flag = true; bool flag = false;
📄 Example
Below is a simple example demonstrating how to use the stdbool.h
definitions to handle boolean values in a C program.
#include <stdio.h>
#include <stdbool.h>
// Function to check if a number is even
bool is_even(int number) {
return (number % 2) == 0;
}
int main() {
int num = 4;
if (is_even(num)) {
printf("%d is even.\n", num);
} else {
printf("%d is odd.\n", num);
}
return 0;
}
💻 Output
4 is even.
🧠 How the Program Works
- Function Definition: The is_even function takes an integer and returns a boolean value (
true
if the number is even,false
otherwise). - Using bool: The return type of the function is bool, and it uses the
true
andfalse
macros for returning boolean values. - Conditional Statement: In the main function, the is_even function is called, and its return value is used in an if statement to print whether the number is even or odd.
🚧 Common Pitfalls
- Including the Header: Forgetting to include the
stdbool.h
header can lead to compilation errors because the bool,true
, andfalse
definitions will be missing. - Compatibility: Using
stdbool.h
in very old C code may cause compatibility issues, as it was introduced in C99. - Implicit Conversions: C automatically converts any non-zero value to
true
and zero tofalse
, which can sometimes lead to unintended behavior if not carefully handled.
✅ Best Practices
- Consistent Usage: Always use bool,
true
, andfalse
fromstdbool.h
instead of defining custom boolean types or constants. - Initialization: Always initialize boolean variables to
true
orfalse
to avoid undefined behavior. - Explicit Comparison: For clarity, use explicit comparisons in conditions, such as if (flag == true) instead of if (flag).
🎉 Conclusion
The stdbool.h
header is a useful addition to the C programming language, providing a standardized way to work with boolean values. By using the bool type and the true
and false
constants, you can make your code more readable and maintainable.
However, it is important to be aware of common pitfalls and adhere to best practices to ensure your code remains robust and clear.
👨💻 Join our Community:
Author
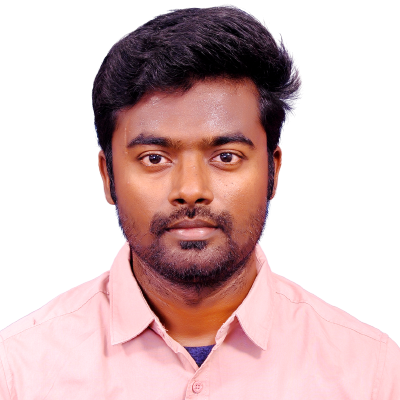
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C Library – stdbool.h), please comment here. I will help you immediately.