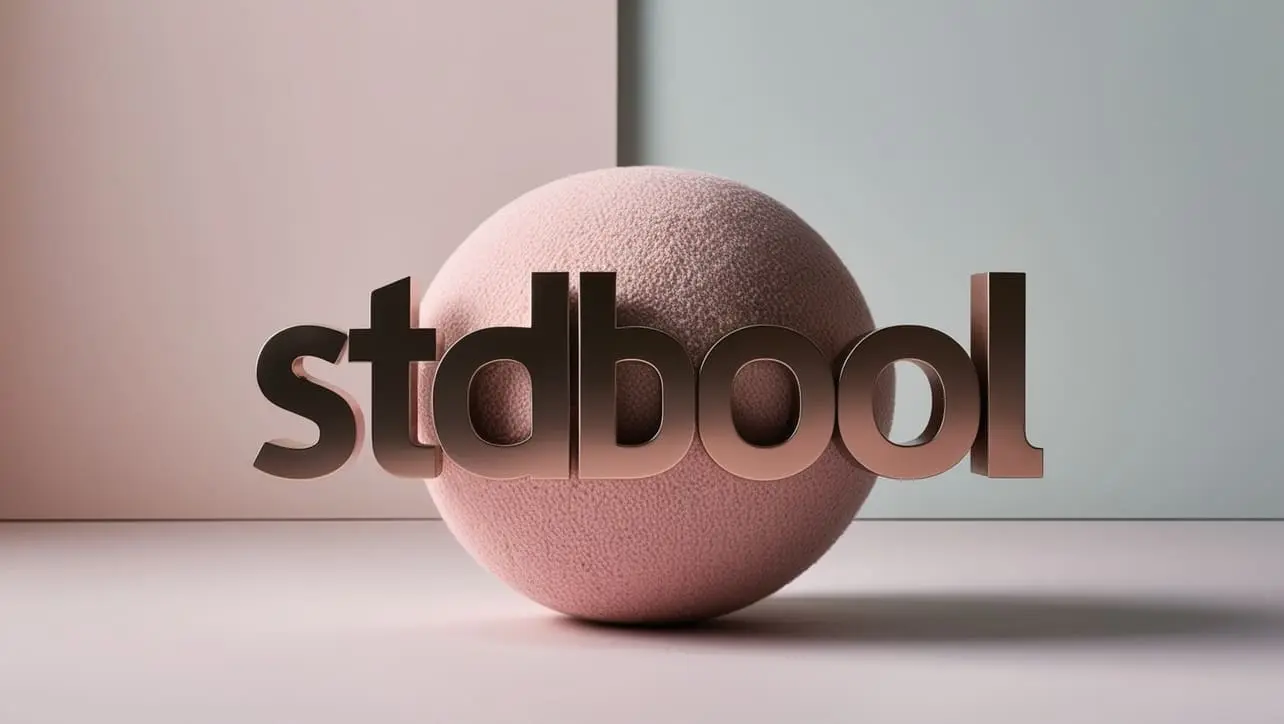
C Topics
- C Intro
- C Control Statement
- C Control Loops
- C String Functions
- C Math Functions
- C Header Files
- C Interview Programs
- Abundant Number
- Amicable Number
- Armstrong Number
- Average of N Numbers
- Automorphic Number
- Biggest of three numbers
- Binary to Decimal
- Common Divisors
- Composite Number
- Condense a Number
- Cube Number
- Decimal to Binary
- Decimal to Octal
- Disarium Number
- Even Number
- Evil Number
- Factorial of a Number
- Fibonacci Series
- GCD
- Happy Number
- Harshad Number
- LCM
- Leap Year
- Magic Number
- Matrix Addition
- Matrix Division
- Matrix Multiplication
- Matrix Subtraction
- Matrix Transpose
- Maximum Value of an Array
- Minimum Value of an Array
- Multiplication Table
- Natural Number
- Number Combination
- Odd Number
- Palindrome Number
- Pascalβs Triangle
- Power of 2
- Power of 3
- Pronic Number
- Perfect Number
- Perfect Square
- Prime Factor
- Prime Number
- Smith Number
- Strong Number
- Sum of Array
- Sum of Digits
- Swap Two Numbers
- Triangular Number
- C Star Pattern
- C Number Pattern
- C Alphabet Pattern
C Program to perform Matrix Transpose
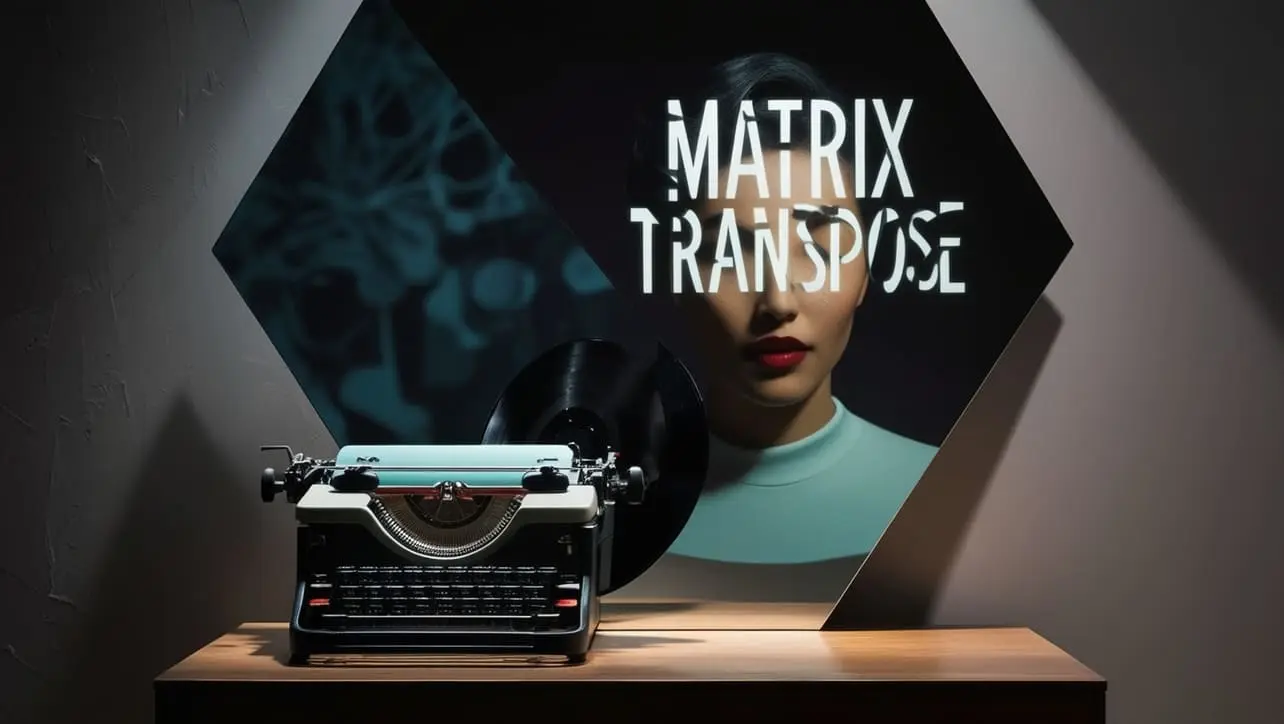
Photo Credit to CodeToFun
Introduction
Matrix operations are fundamental in various fields of computer science and mathematics. One common operation is the transpose of a matrix.
Transposing a matrix involves swapping its rows with columns, resulting in a new matrix where the rows of the original matrix become the columns, and vice versa.
In this tutorial, we'll explore a simple yet effective C program that performs the transpose operation on a given matrix.
Understanding and implementing matrix operations is essential for various applications, including graphics, scientific computing, and data manipulation.
Example
Now, let's look at the C code that performs matrix transpose.
#include <stdio.h>
// Function to perform matrix transpose
void transposeMatrix(int matrix[10][10], int rows, int cols) {
int transposedMatrix[10][10];
// Performing the transpose operation
for (int i = 0; i < cols; ++i) {
for (int j = 0; j < rows; ++j) {
transposedMatrix[i][j] = matrix[j][i];
}
}
// Displaying the transposed matrix
printf("Transposed Matrix:\n");
for (int i = 0; i < cols; ++i) {
for (int j = 0; j < rows; ++j) {
printf("%d\t", transposedMatrix[i][j]);
}
printf("\n");
}
}
// Driver program
int main() {
// Replace these values with your matrix dimensions and elements
int matrix[10][10] = {
{1, 2, 3},
{4, 5, 6}
};
int rows = 2;
int cols = 3;
// Call the function to perform matrix transpose
transposeMatrix(matrix, rows, cols);
return 0;
}
Testing the Program
To test the program with a different matrix, replace the values of matrix, rows, and cols in the main function.
Transposed Matrix: 1 4 2 5 3 6
Compile and run the program to see the transposed matrix.
How the Program Works
- The program defines a function transposeMatrix that takes a matrix, number of rows, and number of columns as input and computes the transpose of the matrix.
- Inside the function, it uses nested loops to swap the rows with columns and stores the result in a new matrix transposedMatrix.
- The transposed matrix is then displayed.
Understanding the Concept of Matrix Transpose
Matrix transpose is a fundamental operation where the rows and columns of a matrix are swapped. If a matrix has dimensions mΓn, its transpose will have dimensions nΓm. The element at position (i, j) in the original matrix becomes the element at position (j,i) in the transposed matrix.
Optimizing the Program
While the provided program is effective, consider exploring and implementing optimizations based on the specific requirements of your application.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
Join our Community:
Author
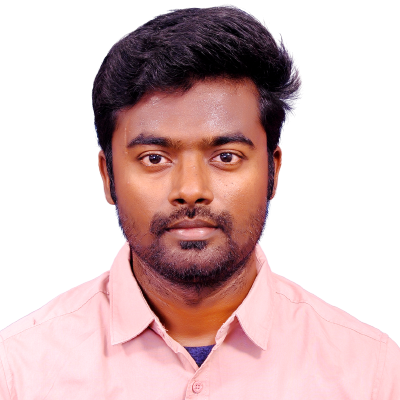
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C Program to perform Matrix Transpose), please comment here. I will help you immediately.