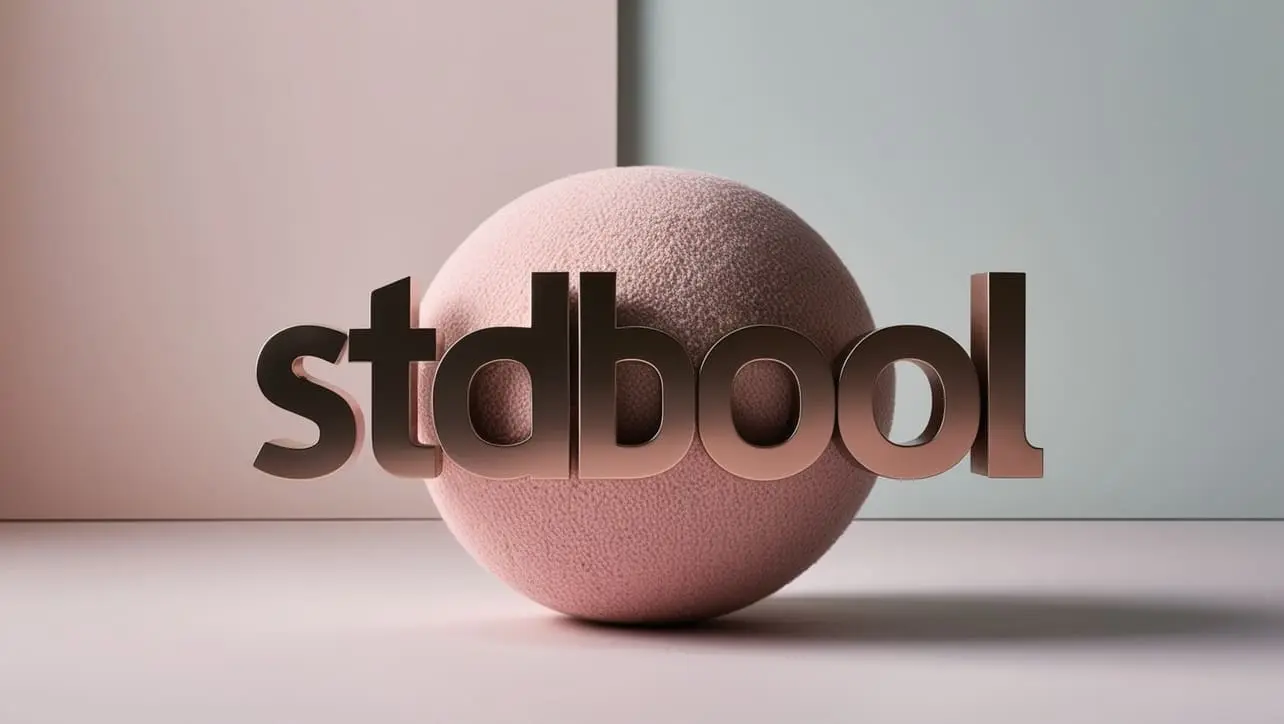
C log10() Function
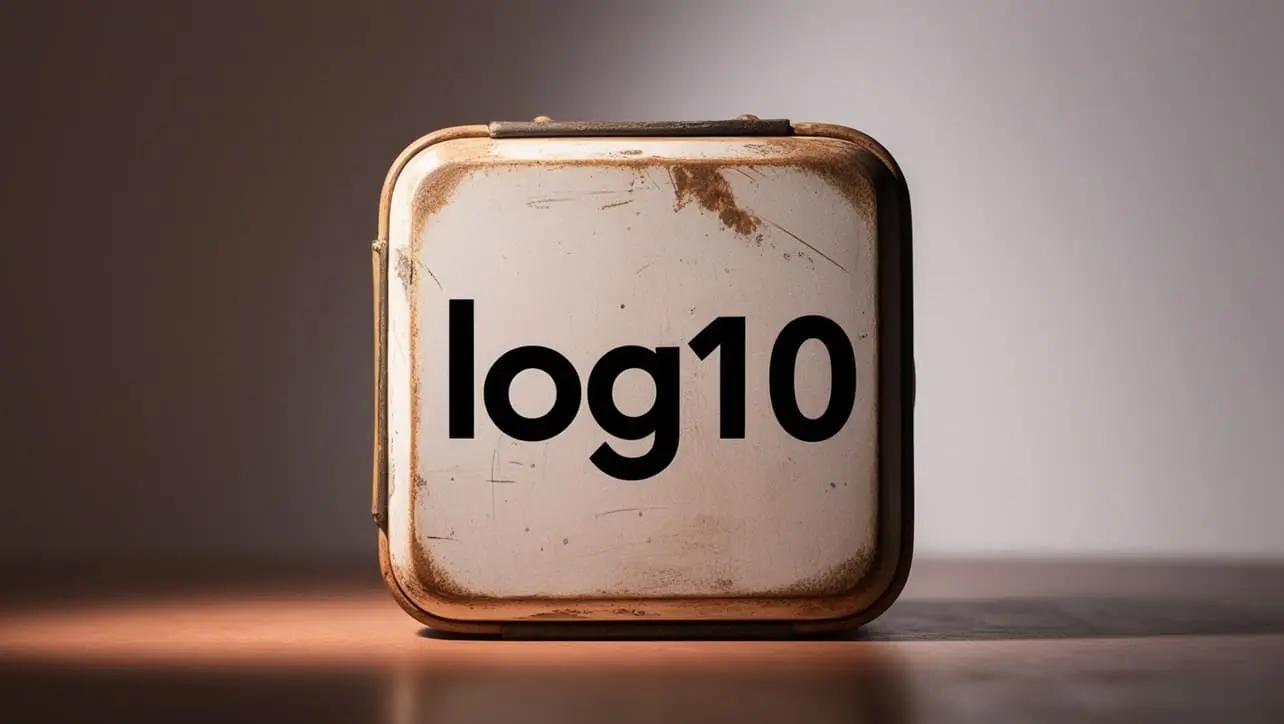
Photo Credit to CodeToFun
đ Introduction
In C programming, mathematical functions play a crucial role in various scientific and engineering applications.
The log10()
function is part of the <math.h> library and is used to calculate the logarithm base 10 of a given number.
In this tutorial, we'll explore the usage and functionality of the log10()
function in C.
đĄ Syntax
The syntax for the log10()
function is as follows:
#include <math.h>
double log10(double x);
- x: The number for which the base-10 logarithm is calculated.
đ Example
Let's dive into an example to illustrate how the log10()
function works.
#include <stdio.h>
#include <math.h>
int main() {
double number = 1000;
// Calculate the base-10 logarithm
double result = log10(number);
// Output the result
printf("Log base 10 of %.2f = %.2f\n", number, result);
return 0;
}
đģ Output
Log base 10 of 1000.00 = 3.00
đ§ How the Program Works
In this example, the log10()
function is used to calculate the base-10 logarithm of the number 1000 and then prints the result.
âŠī¸ Return Value
The log10()
function returns the base-10 logarithm of the given number x as a double value.
đ Common Use Cases
The log10()
function is useful in scenarios where you need to determine the exponent to which the base (10) must be raised to obtain a specific number. It's commonly used in logarithmic scales, signal processing, and scientific calculations.
đ Notes
- The argument x must be greater than 0; otherwise, the behavior of the function is undefined.
- The result may vary slightly due to the finite precision of floating-point arithmetic.
đĸ Optimization
The log10()
function is usually optimized for performance. However, if you are dealing with a large number of logarithmic calculations, consider optimizing the overall algorithm rather than focusing solely on the log10()
function.
đ Conclusion
The log10()
function in C is a valuable tool for calculating the base-10 logarithm of a given number. It is a fundamental function in mathematical computations and provides a way to work with logarithmic scales.
Feel free to experiment with different numbers and explore the behavior of the log10()
function in various scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
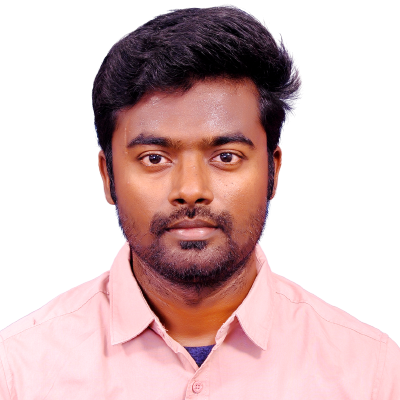
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C log10() Function) please comment here. I will help you immediately.