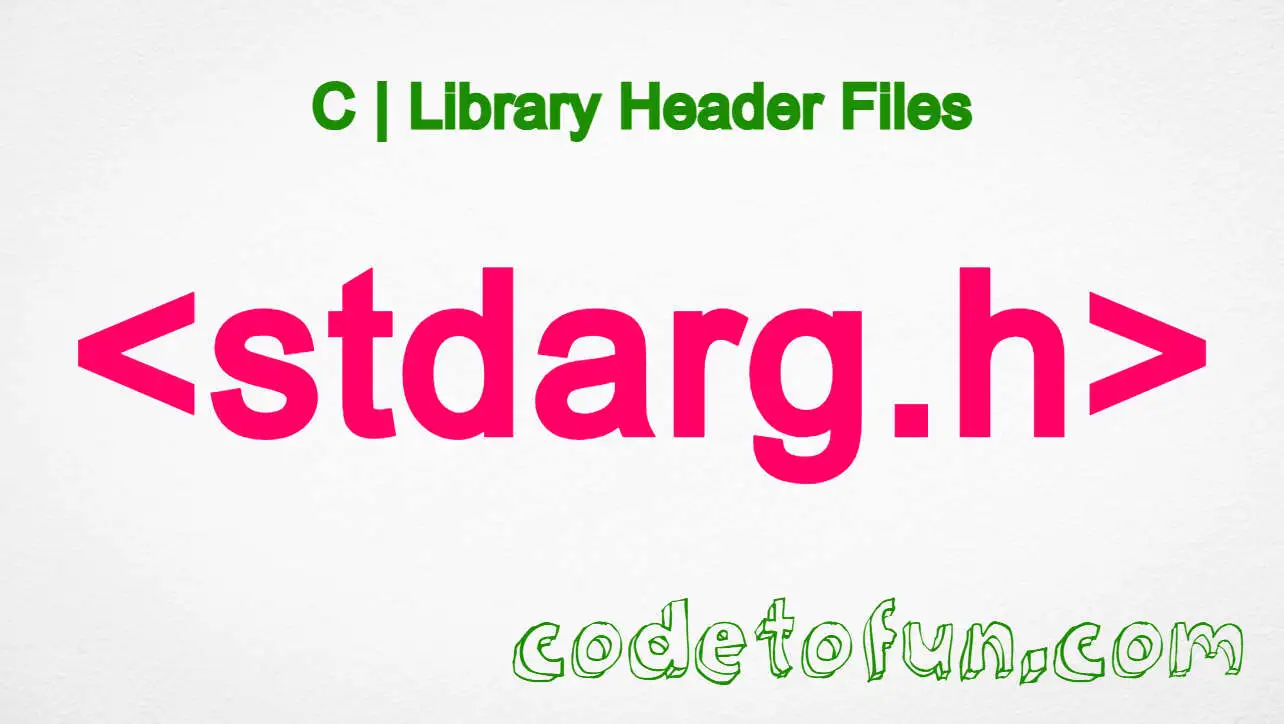
C Basic
C Math Functions
C round() Function
.webp)
Photo Credit to CodeToFun
đ Introduction
In C programming, the round()
function is part of the <math.h> library and is used for rounding floating-point numbers to the nearest integer.
This function is particularly useful when you need to perform arithmetic operations that result in non-integer values, and you want to round the result to the nearest whole number.
In this tutorial, we'll explore the usage and functionality of the round()
function in C.
đĄ Syntax
The syntax for the round()
function is as follows:
double round(double x);
- x: The floating-point number to be rounded.
đ Example
Let's dive into an example to illustrate how the round()
function works.
#include <stdio.h>
#include <math.h>
int main() {
double number = 15.67;
// Round the number
double roundedNumber = round(number);
// Output the result
printf("Original number: %.2f\n", number);
printf("Rounded number: %.0f\n", roundedNumber);
return 0;
}
đģ Output
Original number: 15.67 Rounded number: 16
đ§ How the Program Works
In this example, the round()
function is used to round the floating-point number 15.67 to the nearest integer, and the result is printed.
âŠī¸ Return Value
The round()
function returns a double value representing the rounded integer. The return type is double to accommodate the possibility of rounding to a non-integer value.
đ Common Use Cases
The round()
function is commonly used in scenarios where precise arithmetic operations result in non-integer values, but the application requires an integer result. For example, when dealing with financial calculations or measurements that should be represented as whole numbers.
đ Notes
- The
round()
function rounds towards the nearest integer. If the fractional part is exactly 0.5, the function rounds to the nearest even integer (a method known as "round to even" or "bankers' rounding"). - For rounding towards positive infinity, the ceil() function can be used, and for rounding towards negative infinity, the floor() function is suitable.
đĸ Optimization
The round()
function is generally efficient, but be cautious when using it in performance-critical sections of code. If you are only interested in obtaining the integer part of a floating-point number, consider using type casting ((int)) or the floor() or ceil() functions.
đ Conclusion
The round()
function in C is a valuable tool for rounding floating-point numbers to the nearest integer. It ensures that results are represented in a way that makes sense for the specific application, especially when dealing with calculations that involve non-integer values.
Feel free to experiment with different floating-point numbers and explore the behavior of the round()
function in various scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
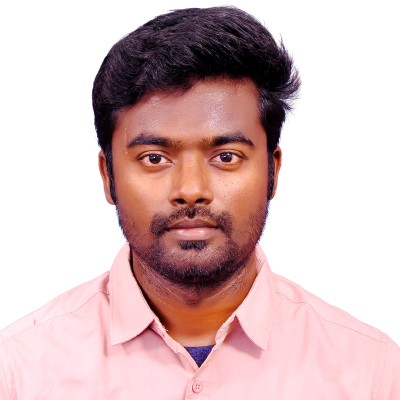
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C round() Function) please comment here. I will help you immediately.