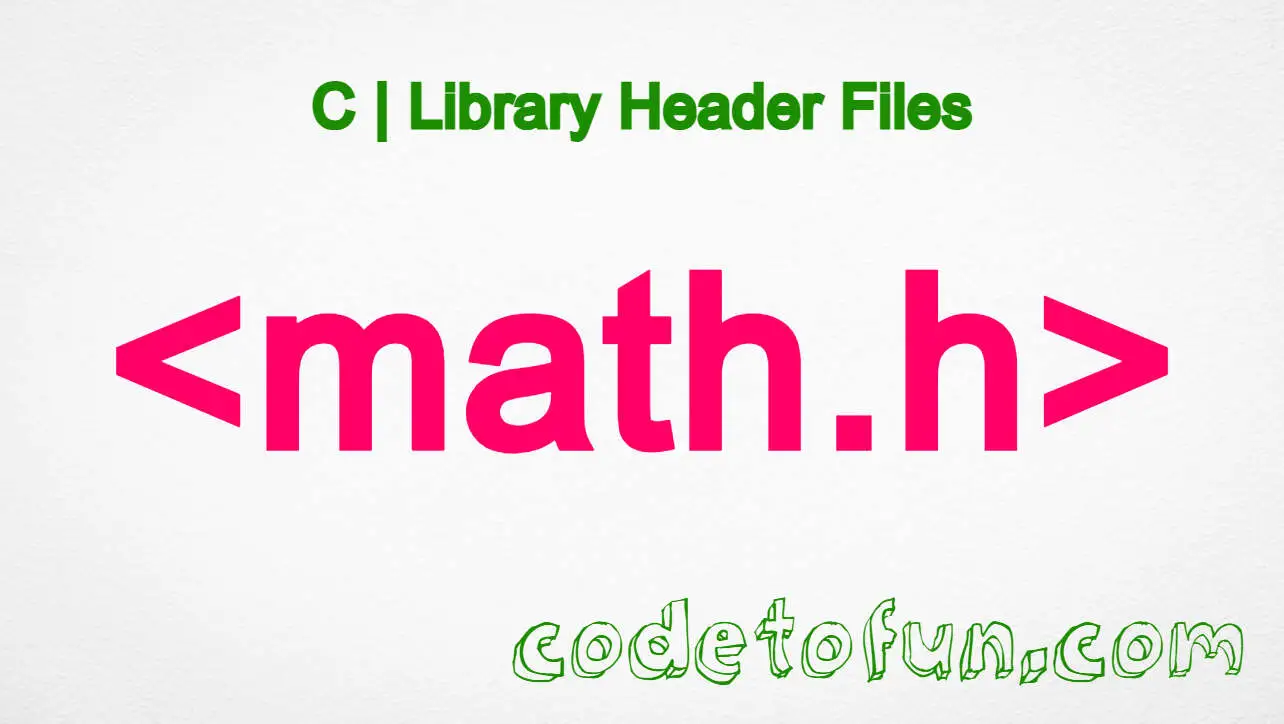
C Header Files
C Library – string.h
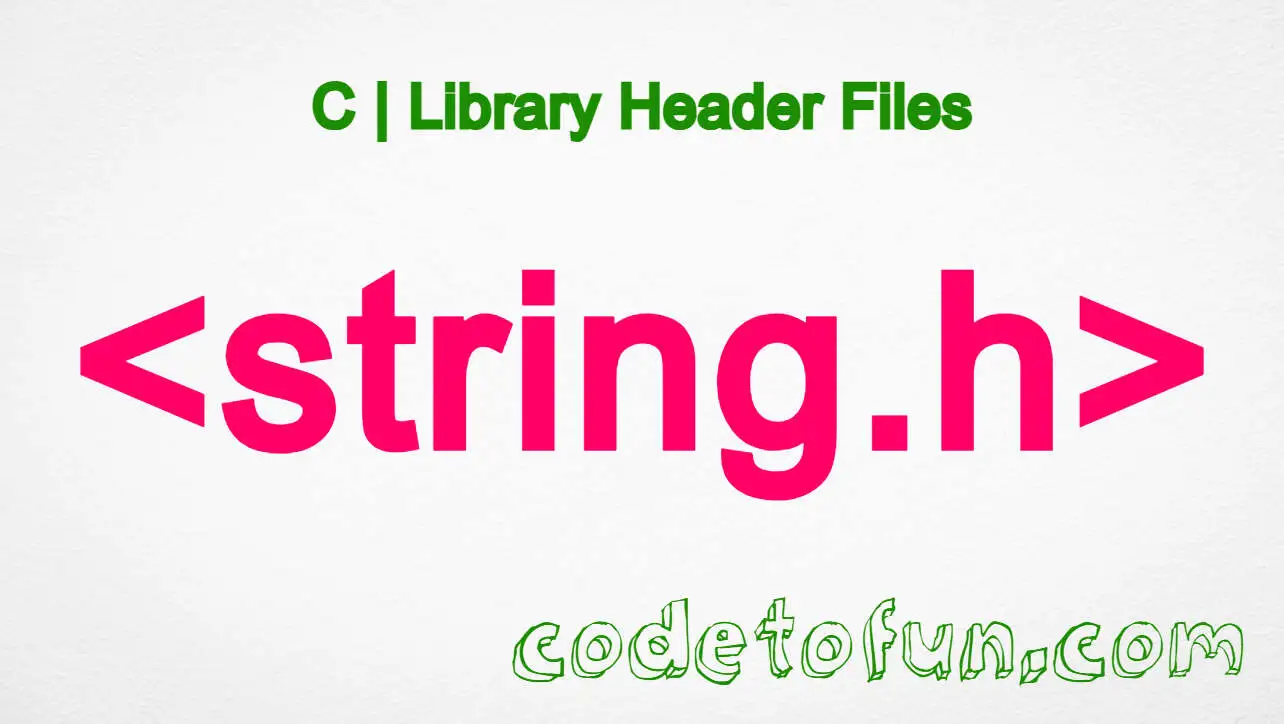
Photo Credit to CodeToFun
🙋 Introduction
The <string.h>
header file in the C programming language provides a collection of functions for manipulating C strings and arrays of characters. These functions enable common operations like copying, concatenation, comparison, and searching within strings. Mastering <string.h>
is essential for efficient string handling in C programs.
💡 Syntax
To use the functions provided by <string.h>
, include the header file at the beginning of your C program:
#include <string.h>
📚 Key Functions and Macros
Here are some of the key functions and macros available in <string.h>
:
strcpy():
Copies a string from a source to a destination.
example.cCopiedchar destination[20]; char source[] = "Hello, World!"; strcpy(destination, source);
strncpy():
Copies up to n characters from a source to a destination, ensuring no overflow.
example.cCopiedchar destination[20]; char source[] = "Hello, World!"; strncpy(destination, source, 5); // Copies "Hello" destination[5] = '\0'; // Null-terminate the string
strcat():
Appends a source string to the end of a destination string.
example.cCopiedchar destination[20] = "Hello, "; char source[] = "World!"; strcat(destination, source);
strncat():
Appends up to n characters from a source string to a destination string.
example.cCopiedchar destination[20] = "Hello, "; char source[] = "World!"; strncat(destination, source, 5); // Appends "World"
strcmp():
Compares two strings lexicographically.
example.cCopiedchar str1[] = "Hello"; char str2[] = "World"; int result = strcmp(str1, str2); // result < 0 because "Hello" is less than "World"
strncmp():
Compares up to n characters of two strings.
example.cCopiedchar str1[] = "Hello"; char str2[] = "Help"; int result = strncmp(str1, str2, 3); // result = 0 because the first 3 characters are equal
strlen():
Returns the length of a string.
example.cCopiedchar str[] = "Hello, World!"; size_t length = strlen(str); // length = 13
strchr():
Finds the first occurrence of a character in a string.
example.cCopiedchar str[] = "Hello, World!"; char *ptr = strchr(str, 'W'); // ptr points to the first occurrence of 'W'
strstr():
Finds the first occurrence of a substring in a string.
example.cCopiedchar str[] = "Hello, World!"; char *ptr = strstr(str, "World"); // ptr points to the first occurrence of "World"
📝 Basic Example:
Here's a complete example demonstrating the use of several <string.h>
functions to manipulate strings:
#include <stdio.h>
#include <string.h>
int main() {
char str1[20] = "Hello, ";
char str2[] = "World!";
char str3[20];
// Copying str2 to str3
strcpy(str3, str2);
printf("str3: %s\n", str3);
// Concatenating str1 and str2
strcat(str1, str2);
printf("str1: %s\n", str1);
// Finding the length of str1
size_t length = strlen(str1);
printf("Length of str1: %zu\n", length);
// Comparing str2 and str3
int result = strcmp(str2, str3);
printf("strcmp result: %d\n", result);
return 0;
}
💻 Output
str1: Hello, World! Length of str1: 13 strcmp result: 0
💰 Benefits
Using the <string.h>
library offers several benefits:
- Standardization: As part of the C standard library,
<string.h>
provides a consistent and portable way to handle string operations across different platforms and compilers. - Ease of Use: The functions in
<string.h>
are straightforward and well-documented, making string manipulation easier even for beginners. - Efficiency: The functions are optimized for performance, ensuring efficient string operations, which is crucial for applications that process large amounts of text.
- Comprehensive Functionality: With a wide range of functions,
<string.h>
covers various aspects of string manipulation, from simple copying to complex searching and comparison. - Interoperability: The standardized functions facilitate interoperability with other libraries and systems that use similar string representations.
🎉 Conclusion
The <string.h>
library in C is an indispensable tool for handling various string operations. By leveraging the functions it provides, you can perform complex string manipulations with ease and efficiency.
Understanding and utilizing <string.h>
is crucial for developing robust and performant C programs.
👨💻 Join our Community:
Author
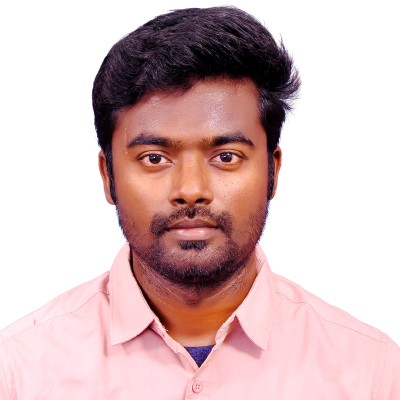
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee