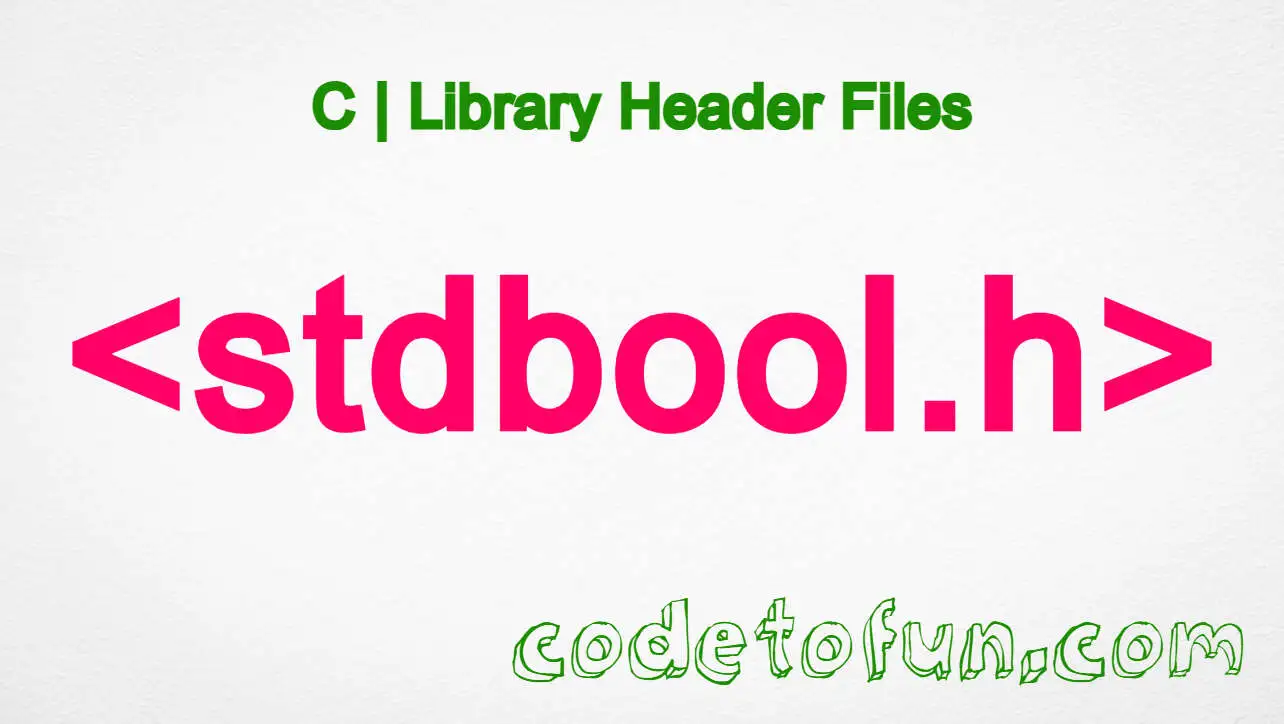
C Header Files
C Library – stddef.h
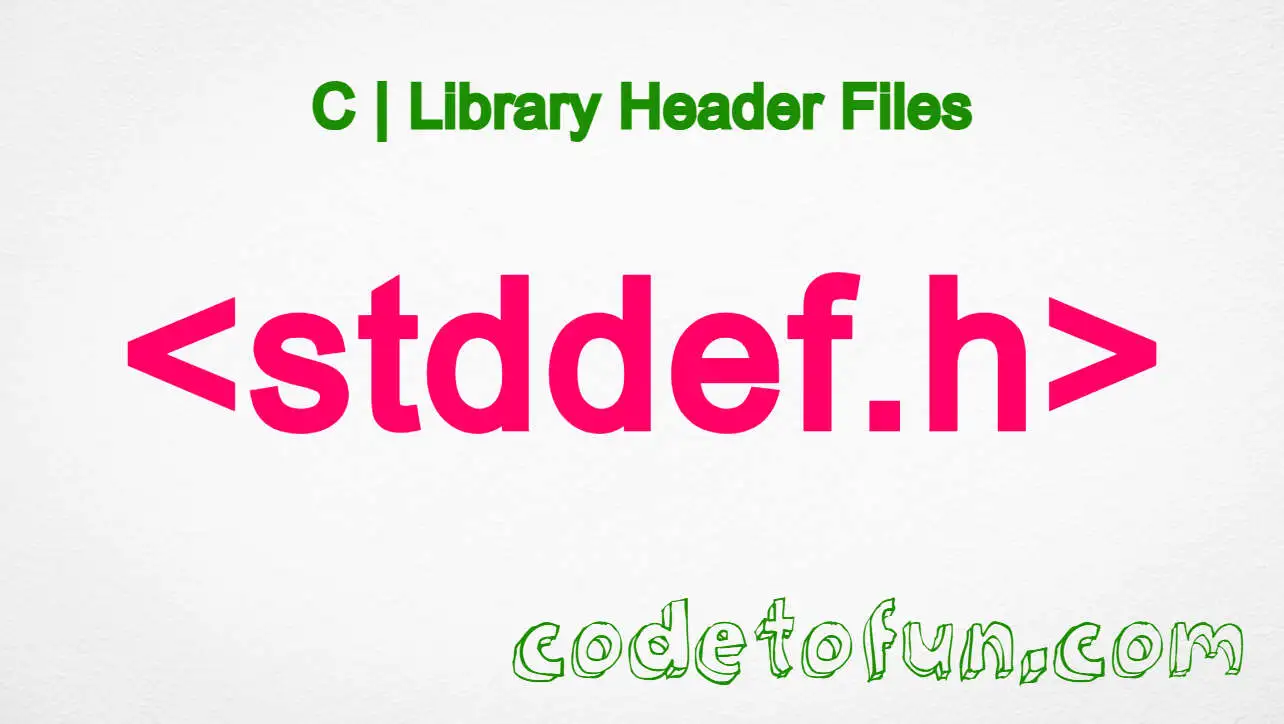
Photo Credit to CodeToFun
🙋 Introduction
The stddef.h
header in C defines several important types and macros that are used for performing various operations, including working with pointer arithmetic, memory management, and type definitions.
It is one of the standard headers in the C library and is included by default in many other standard library headers.
💡 Syntax
To use the stddef.h
library, you need to include it in your program:
#include <stddef.h>
Types and Macros Defined in stddef.h
The stddef.h
header defines several types and macros that facilitate various common tasks in C programming:
- ptrdiff_t: A signed integer type which is the result of subtracting two pointers.
- size_t: An unsigned integer type which is the result of the sizeof operator.
- wchar_t: An integer type used for wide characters.
- NULL: A macro representing a null pointer constant.
- offsetof: A macro which computes the offset of a member within a structure.
Syntax of the Types and Macros
ptrdiff_t:
example.cCopiedtypedef /* implementation-defined */ ptrdiff_t;
size_t:
example.cCopiedtypedef /* implementation-defined */ size_t;
wchar_t:
example.cCopiedtypedef /* implementation-defined */ wchar_t;
NULL:
example.cCopied#define NULL ((void *)0)
offsetof:
example.cCopied#define offsetof(type, member) /* implementation-defined */
📄 Example
Below is a simple example demonstrating how to use some of the types and macros defined in the stddef.h
header.
#include <stdio.h>
#include <stddef.h>
// Define a structure
typedef struct {
int id;
char name[50];
double salary;
} Employee;
int main() {
Employee emp = {1, "John Doe", 75000.0};
// Use size_t to print size of the structure
size_t size = sizeof(Employee);
printf("Size of Employee structure: %zu bytes\n", size);
// Use offsetof to find the offset of the 'salary' member
size_t offset = offsetof(Employee, salary);
printf("Offset of 'salary' in Employee structure: %zu bytes\n", offset);
return 0;
}
💻 Output
Size of Employee structure: 64 bytes Offset of 'salary' in Employee structure: 56 bytes
🧠 How the Program Works
- Structure Definition: The Employee structure is defined with three members: id, name, and salary.
- Using size_t: The sizeof operator returns the size of the Employee structure in bytes, which is stored in a variable of type size_t.
- Using offsetof: The offsetof macro calculates the byte offset of the salary member within the Employee structure.
🚧 Common Pitfalls
- Misusing `NULL`: Assigning NULL to types other than pointers can lead to undefined behavior.
- Pointer Arithmetic: Incorrect use of ptrdiff_t and pointer arithmetic can result in bugs and undefined behavior.
- Structure Alignment: Misalignment of structure members can lead to incorrect calculations with offsetof.
✅ Best Practices
- Consistent Use of Types: Use size_t for array indexing and loop counters to avoid issues with signed vs. unsigned types.
- Pointer Validity: Always ensure pointers are valid before performing arithmetic or dereferencing them.
- Code Readability: Use offsetof to make code involving structure member offsets more readable and maintainable.
- Avoid Magic Numbers: Use the types and macros provided by
stddef.h
instead of hardcoding values, improving code portability and readability.
🎉 Conclusion
The stddef.h
header is an essential part of the C standard library, providing fundamental types and macros that facilitate various programming tasks. By using these types and macros, you can write more robust, readable, and maintainable code.
Understanding and leveraging stddef.h
is crucial for effective C programming, especially when dealing with memory management and pointer arithmetic.
👨💻 Join our Community:
Author
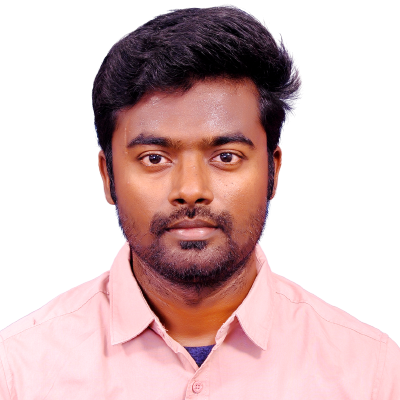
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C Library – stddef.h), please comment here. I will help you immediately.