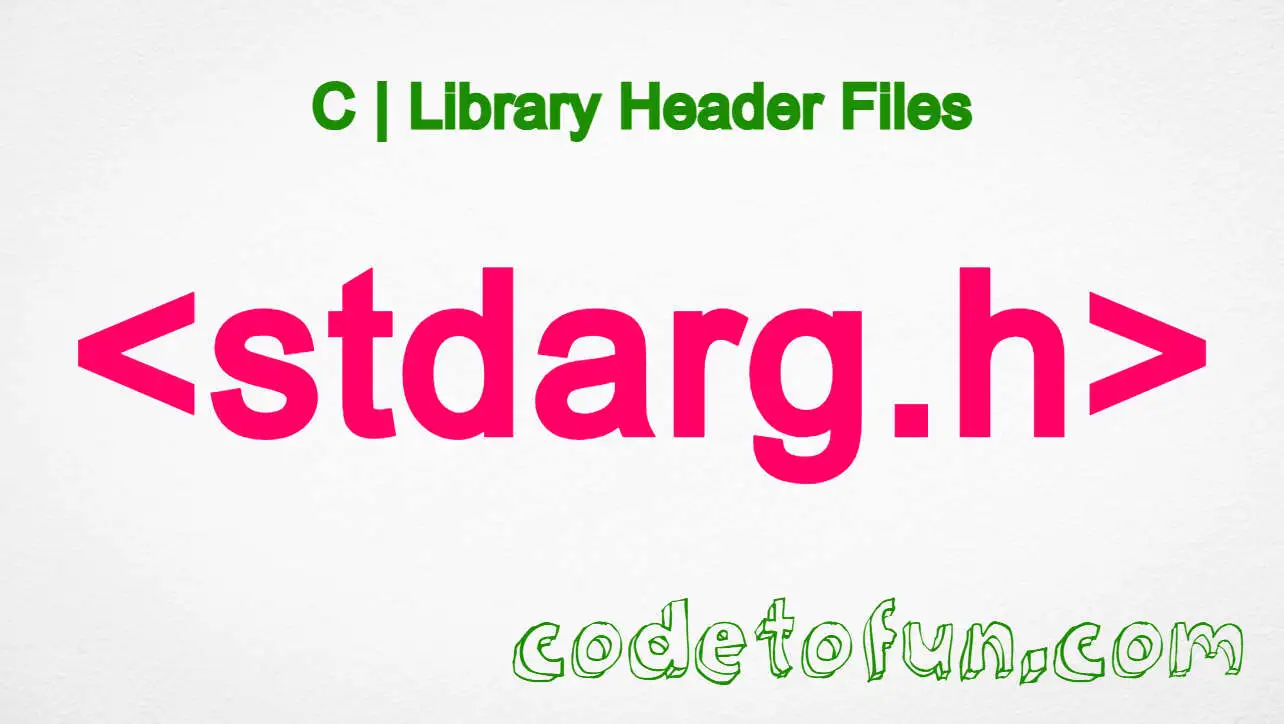
C Header Files
C Library – stdarg.h
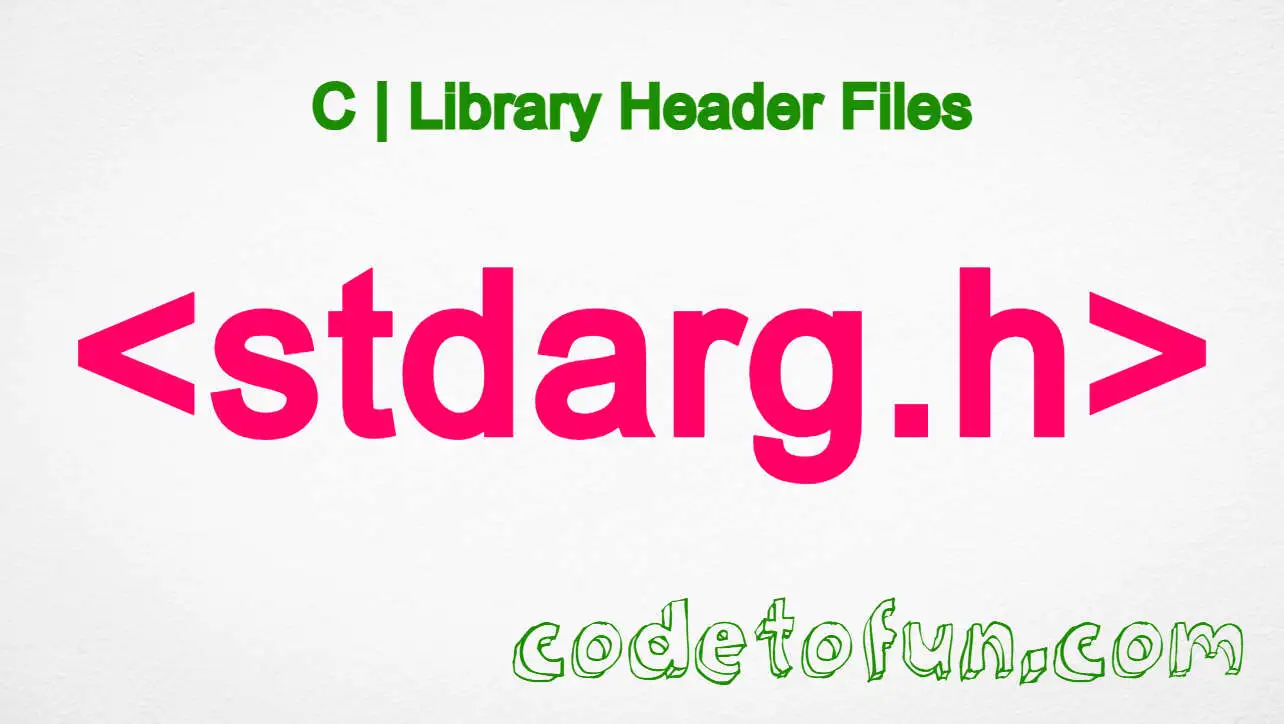
Photo Credit to CodeToFun
🙋 Introduction
The stdarg.h
header in C provides facilities for functions that accept an indefinite number of arguments. It is commonly used for creating functions that can handle a variable number of parameters, such as the printf and scanf family of functions.
This header defines a set of macros that can be used to access the additional arguments of a function.
💡 Syntax
To use the stdarg.h
library, you need to include it in your program:
#include <stdarg.h>
Macros Defined in stdarg.h
The stdarg.h
header defines four macros that facilitate working with variable argument lists:
- va_list: A type suitable for holding information needed by the macros va_start, va_arg, and va_end.
- va_start: Initializes a va_list variable to be used with va_arg and va_end.
- va_arg: Retrieves the next argument in the parameter list of the function.
- va_end: Ends traversal of the variable arguments.
Syntax of the Macros
va_list:
SyntaxCopiedva_list argptr;
va_start:
SyntaxCopiedvoid va_start(va_list ap, last_arg);
- ap: The va_list variable.
- last_arg: The last fixed argument before the variable arguments begin.
va_arg:
SyntaxCopiedtype va_arg(va_list ap, type);
- ap: The va_list variable.
- type: The type of the next argument to be retrieved.
va_end:
SyntaxCopiedvoid va_end(va_list ap);
- ap: The va_list variable.
📄 Example
Below is a simple example demonstrating how to use the stdarg.h
macros to create a function that takes a variable number of arguments. This example defines a function sum that calculates the sum of its integer arguments.
#include <stdio.h>
#include <stdarg.h>
// Function to calculate the sum of an indefinite number of integers
int sum(int count, ...) {
int total = 0;
// Initialize the argument list
va_list args;
va_start(args, count);
// Retrieve each argument and add it to the total
for (int i = 0; i < count; i++) {
total += va_arg(args, int);
}
// Clean up the argument list
va_end(args);
return total;
}
int main() {
printf("Sum of 3, 5, 7: %d\n", sum(3, 3, 5, 7));
printf("Sum of 1, 2, 3, 4, 5: %d\n", sum(5, 1, 2, 3, 4, 5));
return 0;
}
💻 Output
Sum of 3, 5, 7: 15 Sum of 1, 2, 3, 4, 5: 15
🧠 How the Program Works
- Function Definition: The sum function takes an integer count (the number of arguments) followed by an indefinite number of integer arguments.
- Initializing va_list: The va_list variable args is initialized using va_start, with count as the last known argument.
- Accessing Arguments: A loop is used to iterate through the arguments using va_arg, which retrieves each argument as an int.
- Cleaning up: After accessing all arguments, va_end is called to clean up the memory used by va_list.
Common Pitfalls
- Type Safety: The va_arg macro requires the programmer to specify the type of each argument. Mismatching types can lead to undefined behavior.
- Argument Counting: The function must accurately count the number of arguments passed. An incorrect count can result in accessing invalid memory.
- Default Argument Promotion: Arguments of types char and short are promoted to int, and float is promoted to double. This needs to be considered when accessing arguments.
- No Type Checking: Since the variable arguments are not type-checked by the compiler, incorrect usage can cause runtime errors that are hard to debug.
Best Practices
- Consistent Types: Ensure all arguments passed to the function are of the expected type to avoid undefined behavior.
- Sentinel Values: Use sentinel values (like NULL or -1) to mark the end of variable arguments if the count is not passed explicitly.
- Documentation: Clearly document the expected types and order of variable arguments to avoid misuse.
- Validation: Validate the arguments where possible to prevent incorrect usage.
- Use Fixed Arguments: When feasible, prefer fixed arguments for better type safety and compiler checks.
🎉 Conclusion
The stdarg.h
header is a powerful tool for C programmers, enabling the creation of functions with a variable number of arguments. By using the macros provided in this header, you can effectively manage and retrieve these arguments, making your functions more flexible and adaptable. This is particularly useful for functions like printf, which need to handle different numbers and types of parameters seamlessly. However, it is essential to be mindful of common pitfalls and adhere to best practices to avoid potential issues.
👨💻 Join our Community:
Author
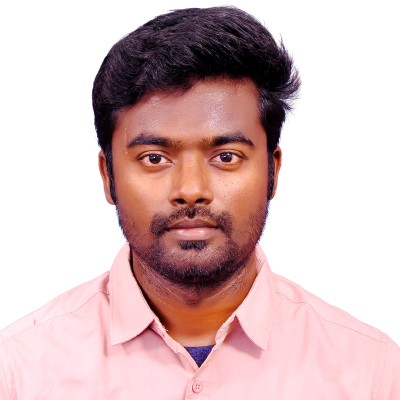
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee