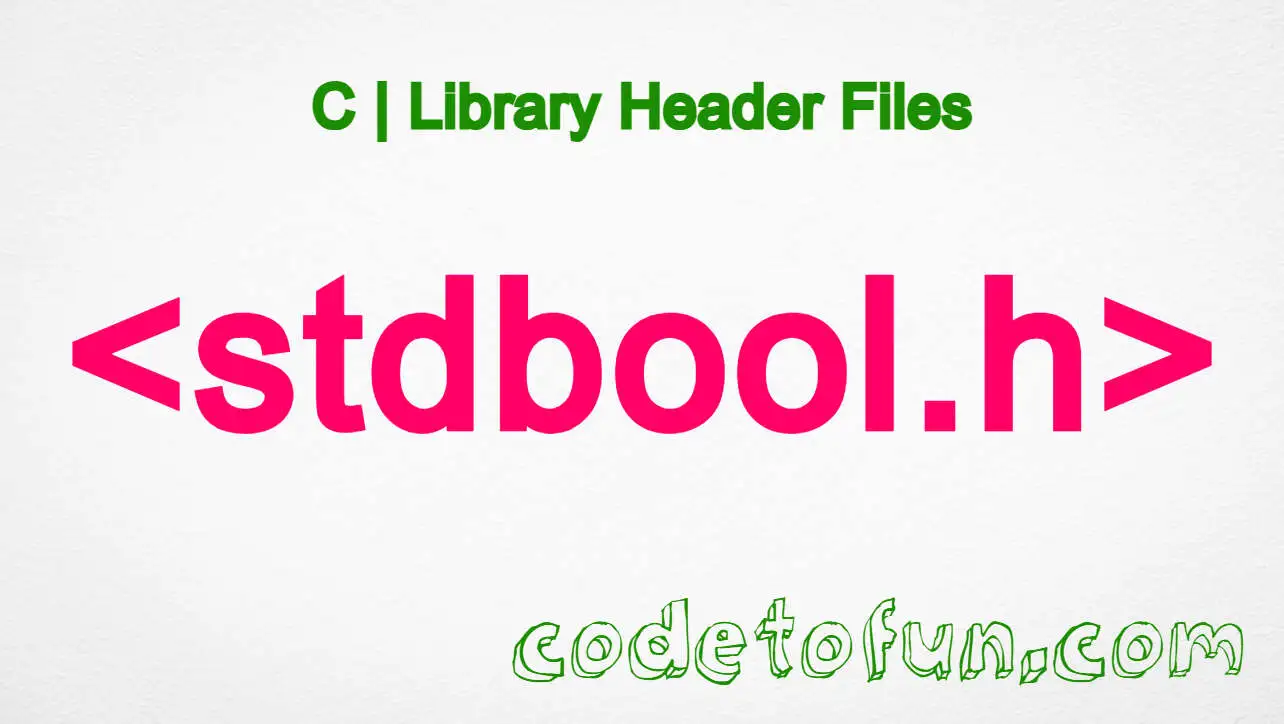
C Header Files
C Library – signal.h
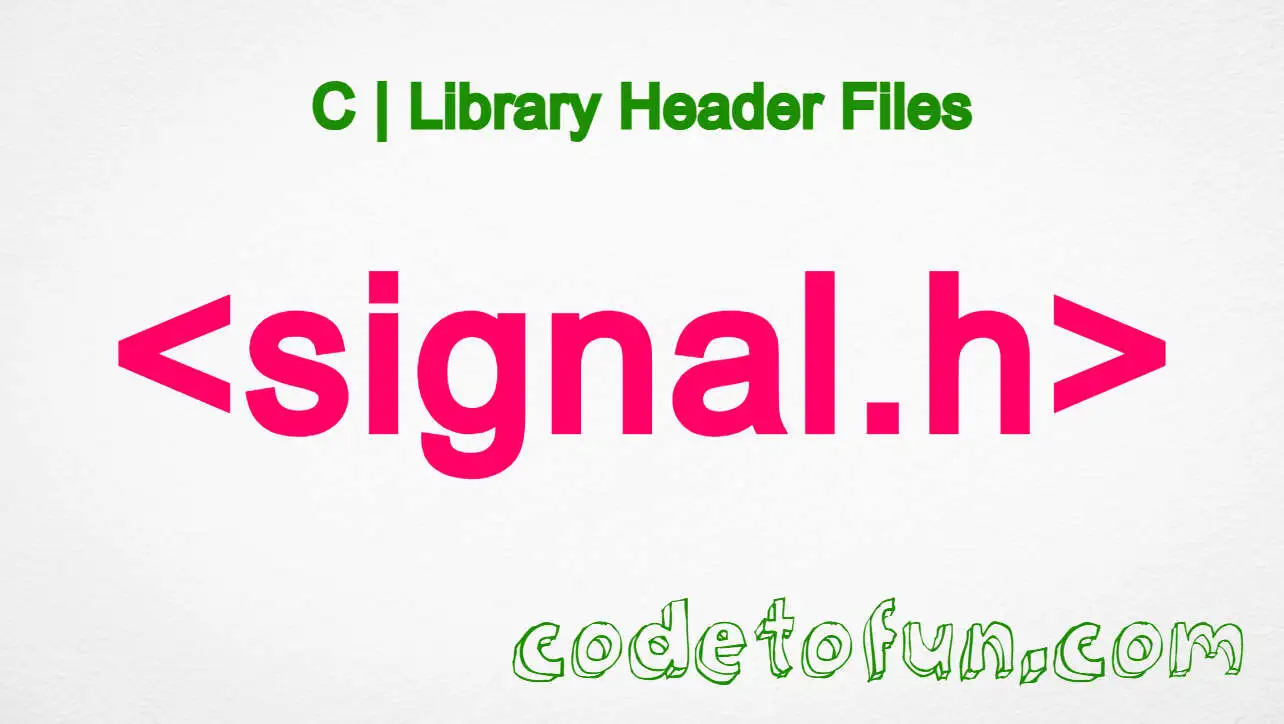
Photo Credit to CodeToFun
🙋 Introduction
The signal.h
header in C defines a set of functions and macros to handle signals. Signals are software interrupts that provide a way to handle asynchronous events such as an attempt to divide by zero, an invalid memory access, or a user request to interrupt the process.
Signal handling allows a program to specify a function, called a signal handler, to be executed when a particular signal is received.
💡 Syntax
To use the signal.h
library, you need to include it in your program:
#include <signal.h>
Functions and Macros Defined in signal.h
The signal.h
header defines several functions and macros to manage signals:
- signal: Sets a function to handle a specific signal.
- raise: Sends a signal to the calling process.
- kill: Sends a signal to a specified process.
- sigaction: Examines and changes a signal action.
- sigemptyset: Initializes a signal set to exclude all signals.
- sigfillset: Initializes a signal set to include all signals.
- sigaddset: Adds a signal to a signal set.
- sigdelset: Deletes a signal from a signal set.
- sigismember: Checks whether a signal is a member of a signal set.
- sigprocmask: Examines and changes blocked signals.
Common Signals
Some of the common signals defined in signal.h
include:
- SIGABRT: Abnormal termination, such as by calling abort.
- SIGFPE: Floating-point exception, such as division by zero.
- SIGILL: Illegal instruction.
- SIGINT: Interrupt signal from the keyboard (e.g., Ctrl+C).
- SIGSEGV: Invalid memory access (segmentation fault).
- SIGTERM: Termination request.
📄 Example
Below is a simple example demonstrating how to use the signal.h
macros to set up a signal handler for the SIGINT signal (typically generated by pressing Ctrl+C).
#include <stdio.h>
#include <signal.h>
#include <unistd.h>
// Signal handler function
void handle_sigint(int sig) {
printf("Caught signal %d\n", sig);
}
int main() {
// Set up the signal handler for SIGINT
signal(SIGINT, handle_sigint);
printf("Press Ctrl+C to trigger SIGINT\n");
// Infinite loop to keep the program running
while (1) {
sleep(1);
}
return 0;
}
💻 Output
Press Ctrl+C to trigger SIGINT Caught signal 2
🧠 How the Program Works
- Signal Handler Function: The handle_sigint function is defined to handle the SIGINT signal. It takes an integer parameter sig which represents the signal number.
- Setting the Signal Handler: The signal function is used to set handle_sigint as the handler for the SIGINT signal.
- Infinite Loop: The while (1) loop keeps the program running so that it can receive signals. The sleep(1) function is used to pause the program for one second in each iteration to reduce CPU usage.
🚧 Common Pitfalls
- Reentrant Functions: Signal handlers should only call reentrant functions (those that can be interrupted at any time and safely called again) since the signal might interrupt the normal flow of execution.
- Signal Interruption: Signals can interrupt functions and cause inconsistent states if not handled correctly.
- Blocking Signals: Ensure critical sections of code are protected by blocking signals to prevent inconsistent states.
✅ Best Practices
- Minimal Signal Handlers: Keep signal handlers as short and simple as possible. Avoid complex logic inside a signal handler.
- Use sigaction: Prefer sigaction over signal for setting up signal handlers, as it provides more control and is more portable.
- Blocking and Unblocking Signals: Use sigprocmask to block signals during critical code sections to avoid race conditions and inconsistent states.
- Reentrant Functions: Use only async-signal-safe functions within signal handlers.
🎉 Conclusion
The signal.h
header in C provides essential tools for handling asynchronous events through signals. By using the functions and macros defined in this header, you can set up signal handlers to manage various signals and ensure your program responds appropriately to different events.
However, handling signals requires careful consideration of common pitfalls and best practices to ensure the program remains robust and reliable. Proper signal management can significantly enhance the flexibility and resilience of your applications.
👨💻 Join our Community:
Author
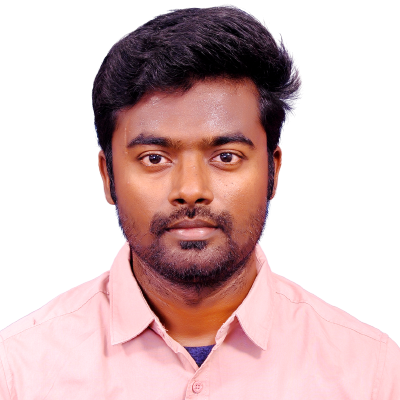
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C Library – signal.h), please comment here. I will help you immediately.