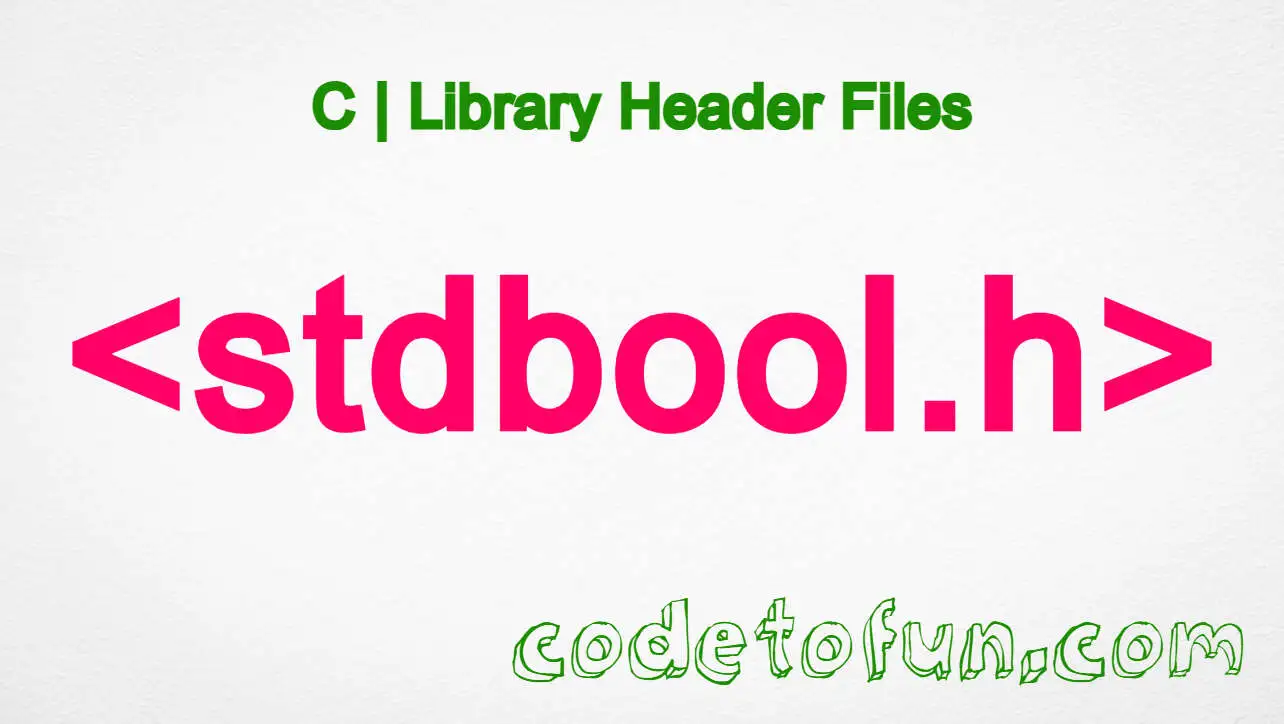
C Header Files
C Library – setjmp.h
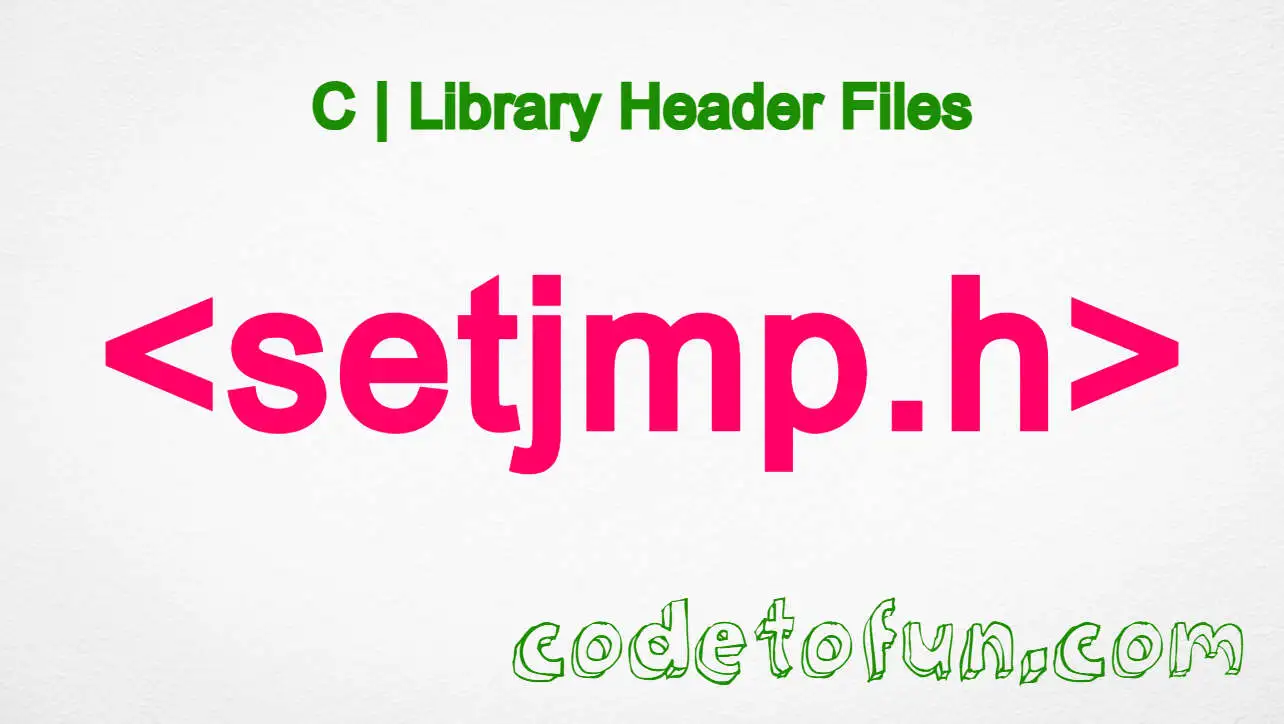
Photo Credit to CodeToFun
🙋 Introduction
The setjmp.h
header in C provides macros for non-local jumps, which can be used to perform error handling and recover from unexpected conditions in your programs.
This header defines the setjmp and longjmp macros, which allow you to save and restore the calling environment.
💡 Syntax
To use the setjmp.h
library, you need to include it in your program:
#include <setjmp.h>
Macros Defined in setjmp.h
The setjmp.h
header defines two key macros:
- setjmp: Saves the current environment (including stack context and registers) for later use by longjmp.
- longjmp: Restores the environment previously saved by setjmp and performs a jump to that point in the program.
Syntax of the Macros
setjmp:
example.cCopiedint setjmp(jmp_buf env);
- env: A buffer of type jmp_buf that stores the environment.
longjmp:
example.cCopiedvoid longjmp(jmp_buf env, int val);
- env: The buffer containing the saved environment.
- val: The value to return to the point where setjmp was called. If val is zero, it will return 1.
📄 Example
Below is an example demonstrating how to use the setjmp.h
macros to handle errors in a function that performs division.
#include <stdio.h>
#include <setjmp.h>
jmp_buf env;
void divide(int a, int b) {
if (b == 0) {
longjmp(env, 1); // Trigger a jump to the saved environment
}
printf("Result: %d\n", a / b);
}
int main() {
if (setjmp(env) == 0) {
// Normal execution path
divide(10, 2);
divide(10, 0); // This will cause a jump
} else {
// Error handling path
printf("Error: Division by zero!\n");
}
return 0;
}
💻 Output
Result: 5 Error: Division by zero!
🧠 How the Program Works
- Environment Buffer: A jmp_buf variable env is declared to store the environment.
- Saving the Environment: setjmp saves the current environment and returns 0 if it's called directly.
- Triggering a Jump: If a division by zero is detected, longjmp is called to restore the saved environment.
- Handling the Jump: When longjmp is called, the program jumps back to the setjmp call, which now returns 1, and the error message is printed.
🚧 Common Pitfalls
- Local Variables: Local variables may have undefined values after a jump if they are not volatile.
- Resource Management: Non-local jumps bypass normal function call/return mechanisms, which can lead to resource leaks (e.g., memory, file handles).
- Code Readability: Excessive use of setjmp and longjmp can make code difficult to read and maintain.
- Control Flow: Misusing setjmp and longjmp can result in unpredictable control flow, making debugging challenging.
✅ Best Practices
- Limit Usage: Use setjmp and longjmp sparingly and only for exceptional error handling.
- Volatile Variables: Declare local variables as volatile if they need to retain their values after a jump.
- Resource Cleanup: Ensure proper resource management by cleaning up resources before performing a jump.
- Clear Documentation: Clearly document the use of setjmp and longjmp to aid understanding and maintenance.
- Consistent Returns: Ensure that longjmp returns a consistent and meaningful value to the calling environment.
🎉 Conclusion
The setjmp.h
header provides a mechanism for performing non-local jumps, which can be useful for error handling in C programs.
By using the setjmp and longjmp macros, you can save the current environment and jump back to it when an error occurs. However, it is essential to use these macros judiciously and follow best practices to avoid common pitfalls and ensure your code remains readable and maintainable.
👨💻 Join our Community:
Author
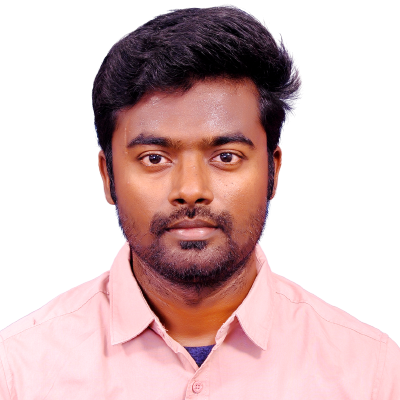
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C Library – setjmp.h), please comment here. I will help you immediately.