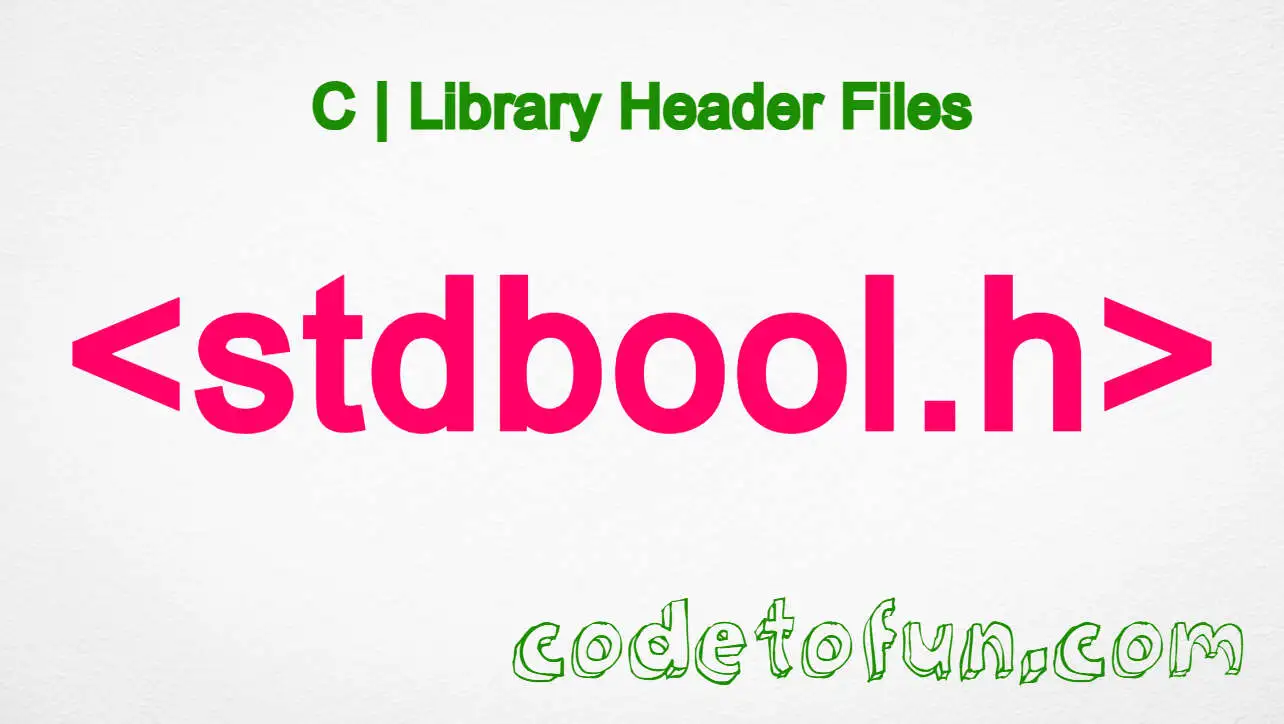
C Header Files
C Library – locale.h
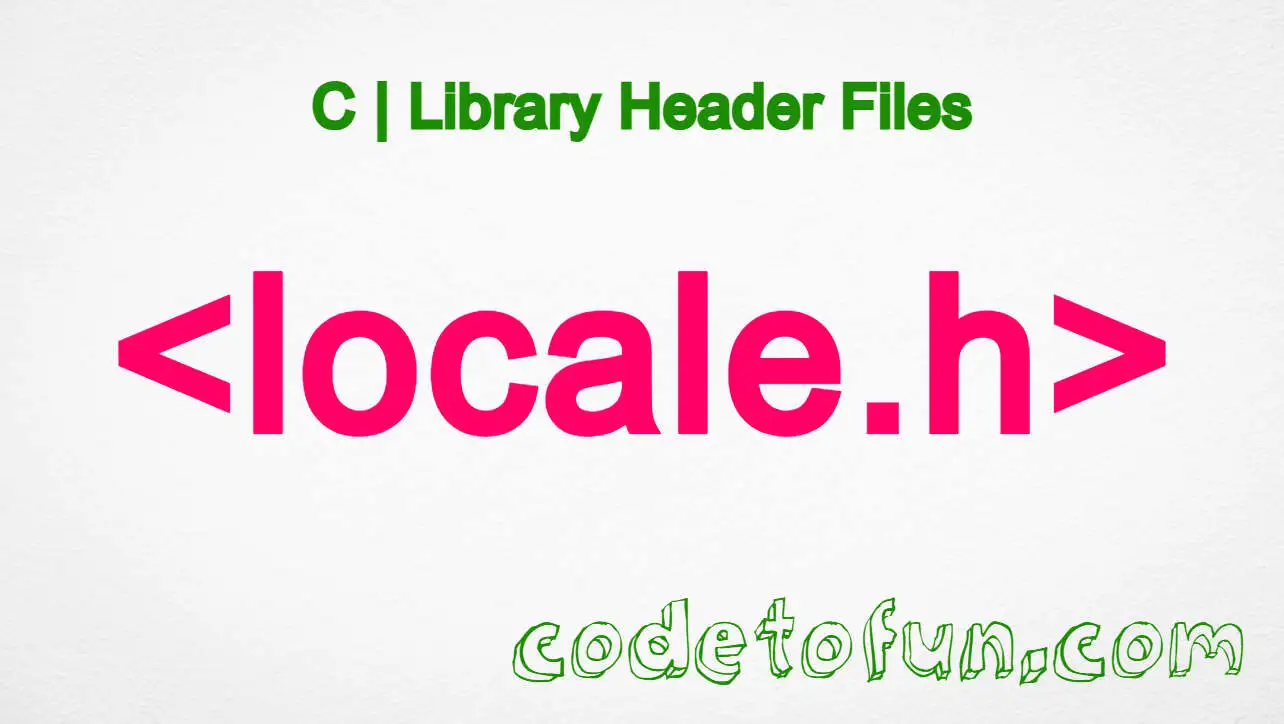
Photo Credit to CodeToFun
๐ Introduction
The locale.h
header in C provides functions and macros for manipulating locale settings. A locale is a set of parameters that defines the user's language, country, and any special variant preferences. It affects various aspects of program behavior, such as the formatting of dates, times, numbers, and monetary values.
The locale.h
library is crucial for developing internationalized programs that need to adapt to different cultural conventions.
๐ก Syntax
To use the locale.h
library, you need to include it in your program:
#include <locale.h>
Macros and Functions Defined in locale.h
The locale.h
header defines several macros and functions to manage and query locale settings.
Macros
- LC_ALL: Selects the entire locale.
- LC_COLLATE: Affects string comparison functions.
- LC_CTYPE: Affects character handling functions.
- LC_MONETARY: Affects monetary formatting.
- LC_NUMERIC: Affects numeric formatting.
- LC_TIME: Affects date and time formatting.
Functions
setlocale: Sets or queries the programโs current locale.
example.cCopiedchar* setlocale(int category, const char* locale);
- category: Specifies which parts of the program's locale should be affected.
- locale: A string specifying a locale name. If NULL, the function queries the current locale.
localeconv: Returns a pointer to a structure containing the numeric and monetary formatting information for the current locale.
example.cCopiedstruct lconv* localeconv(void);
๐ Example
Below is a simple example demonstrating how to use the locale.h
macros and functions to change the programโs locale settings and format a number accordingly.
#include <stdio.h>
#include <locale.h>
#include <stdlib.h>
int main() {
// Set locale to the user's default locale
setlocale(LC_ALL, "");
// Get locale-specific information
struct lconv *lc = localeconv();
// Print the current locale's numeric formatting details
printf("Decimal point character: %s\n", lc->decimal_point);
printf("Thousands separator: %s\n", lc->thousands_sep);
// Format a number according to the current locale
char number[100];
snprintf(number, sizeof(number), "%'f", 1234567.89);
printf("Formatted number: %s\n", number);
return 0;
}
๐ป Output
Decimal point character: . Thousands separator: , Formatted number: 1,234,567.890000
๐ง How the Program Works
- Setting Locale: The setlocale function is used to set the program's locale to the user's default locale.
- Retrieving Locale Information: The localeconv function retrieves the current locale's formatting information, which is stored in a struct lconv.
- Formatting Output: The program prints the current localeโs decimal point character and thousands separator. It then formats a number according to these settings and prints the result.
๐ง Common Pitfalls
- Portability Issues: Not all systems support all locales, and locale names can vary between systems.
- Locale-Dependent Behavior: Be aware that locale settings can affect many standard library functions, which might lead to unexpected behavior if not handled correctly.
- Thread Safety: The setlocale function is not thread-safe. Changing the locale in a multithreaded program can cause data races and inconsistent behavior.
โ Best Practices
- Check for Success: Always check the return value of setlocale to ensure that the locale was set successfully.
- Use Specific Locales: When setting a locale, use specific locale strings (e.g., "en_US.UTF-8") rather than empty strings or "C" to avoid unexpected results.
- Isolate Locale-Sensitive Code: Isolate code that depends on locale settings to avoid side effects and make the program more predictable.
- Restore Original Locale: If you need to temporarily change the locale, store the original locale and restore it afterward to prevent unintended side effects.
๐ Conclusion
The locale.h
header is an essential tool for writing internationalized C programs.
By providing functions and macros to manipulate locale settings, it enables programs to adapt to different cultural conventions. However, developers must be mindful of common pitfalls, such as portability issues and thread safety, and adhere to best practices to ensure their programs behave correctly and predictably across different locales.
๐จโ๐ป Join our Community:
Author
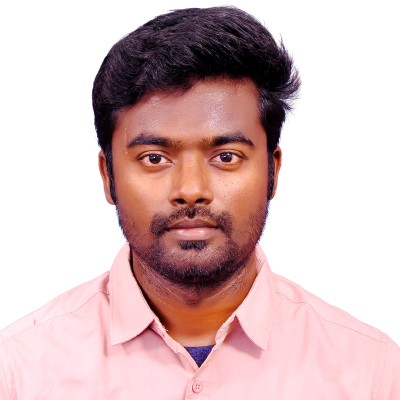
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee