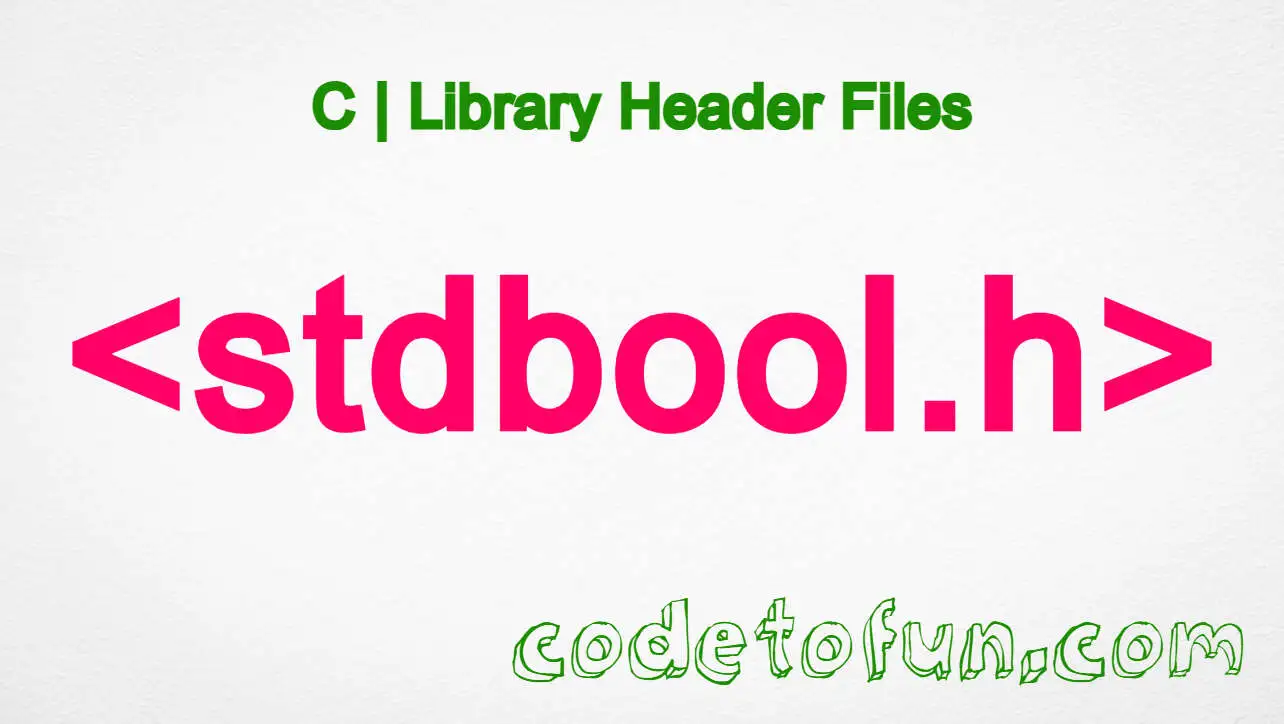
C Header Files
C Library – float.h
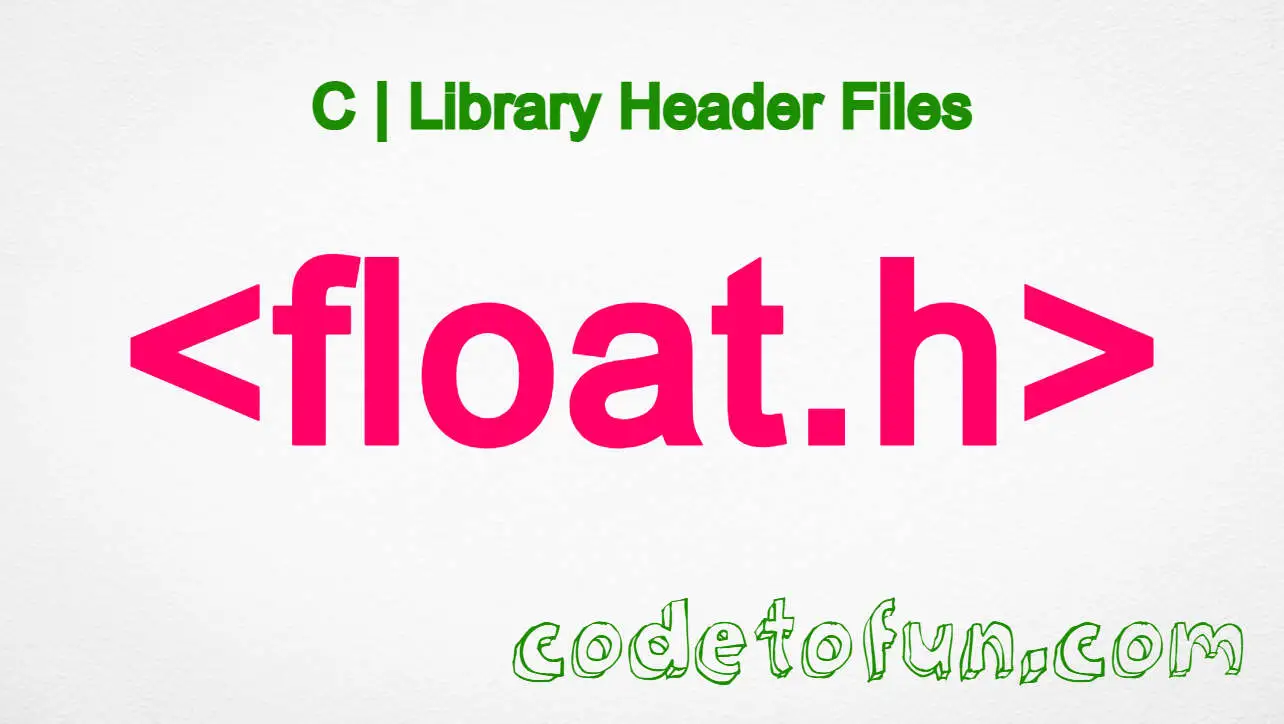
Photo Credit to CodeToFun
🙋 Introduction
The float.h
header in C provides a set of macros that specify the implementation-specific limits and properties of floating-point types. These macros define various aspects of floating-point arithmetic, such as the smallest and largest possible values, the precision, and the smallest difference between two distinct floating-point numbers. This header is essential for writing portable programs that need to handle floating-point numbers consistently across different platforms.
💡 Syntax
To use the float.h
library, you need to include it in your program:
#include <float.h>
Macros Defined in float.h
The float.h
header defines a variety of macros for three floating-point types: float, double, and long double. These macros include:
Float Type Macros
- FLT_MIN: Minimum positive value of type float.
- FLT_MAX: Maximum positive value of type float.
- FLT_EPSILON: Smallest positive float such that 1.0 + FLT_EPSILON != 1.0.
- FLT_DIG: Number of decimal digits of precision for float.
- FLT_MANT_DIG: Number of base-radix digits in the significand of float.
- FLT_MIN_EXP: Minimum negative integer value for the exponent of float.
- FLT_MAX_EXP: Maximum integer value for the exponent of float.
Double Type Macros
- DBL_MIN: Minimum positive value of type double.
- DBL_MAX: Maximum positive value of type double.
- DBL_EPSILON: Smallest positive double such that 1.0 + DBL_EPSILON != 1.0.
- DBL_DIG: Number of decimal digits of precision for double.
- DBL_MANT_DIG: Number of base-radix digits in the significand of double.
- DBL_MIN_EXP: Minimum negative integer value for the exponent of double.
- DBL_MAX_EXP: Maximum integer value for the exponent of double.
Long Double Type Macros
- LDBL_MIN: Minimum positive value of type long double.
- LDBL_MAX: Maximum positive value of type long double.
- LDBL_EPSILON: Smallest positive long double such that 1.0 + LDBL_EPSILON != 1.0.
- LDBL_DIG: Number of decimal digits of precision for long double.
- LDBL_MANT_DIG: Number of base-radix digits in the significand of long double.
- LDBL_MIN_EXP: Minimum negative integer value for the exponent of long double.
- LDBL_MAX_EXP: Maximum integer value for the exponent of long double.
📄 Example
The following example demonstrates how to use some of the macros defined in the float.h
header to display the properties of floating-point types:
#include <stdio.h>
#include <float.h>
int main() {
printf("Float properties:\n");
printf("Minimum positive value: %e\n", FLT_MIN);
printf("Maximum positive value: %e\n", FLT_MAX);
printf("Precision value: %e\n", FLT_EPSILON);
printf("Decimal digits of precision: %d\n", FLT_DIG);
printf("\nDouble properties:\n");
printf("Minimum positive value: %e\n", DBL_MIN);
printf("Maximum positive value: %e\n", DBL_MAX);
printf("Precision value: %e\n", DBL_EPSILON);
printf("Decimal digits of precision: %d\n", DBL_DIG);
printf("\nLong Double properties:\n");
printf("Minimum positive value: %Le\n", LDBL_MIN);
printf("Maximum positive value: %Le\n", LDBL_MAX);
printf("Precision value: %Le\n", LDBL_EPSILON);
printf("Decimal digits of precision: %d\n", LDBL_DIG);
return 0;
}
💻 Output
Maximum positive value: 1.189731e+4932 Precision value: 1.084202e-19 Decimal digits of precision: 18
🧠 How the Program Works
- Including float.h: The program includes the
float.h
header to access the floating-point limits and properties. - Displaying Properties: The program uses printf to display various properties of the float, double, and long double types using the macros defined in
float.h
.
🚧 Common Pitfalls
- Platform Dependency: The actual values of the macros can vary between platforms, so assumptions about specific values should be avoided.
- Precision Errors: Floating-point arithmetic can introduce precision errors, especially when dealing with very small or very large values.
- Type Casting: Be cautious when casting between different floating-point types, as this can lead to loss of precision or range.
✅ Best Practices
- Portable Code: Use the
float.h
macros to write portable code that works consistently across different platforms. - Precision Awareness: Be aware of the precision and range limitations of floating-point types and design algorithms accordingly.
- Documentation: Clearly document any assumptions or dependencies related to floating-point arithmetic in your code.
- Validation: Validate floating-point calculations, especially when dealing with critical applications like financial or scientific computations.
🎉 Conclusion
The float.h
header is a valuable resource for C programmers working with floating-point arithmetic. It provides a standardized way to access the limits and properties of floating-point types, ensuring that programs can handle these types consistently across different platforms.
By understanding and utilizing the macros defined in float.h
, you can write more robust and portable code that effectively manages floating-point calculations.
👨💻 Join our Community:
Author
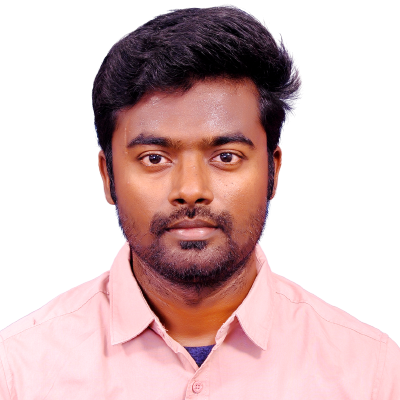
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C Library – float.h), please comment here. I will help you immediately.