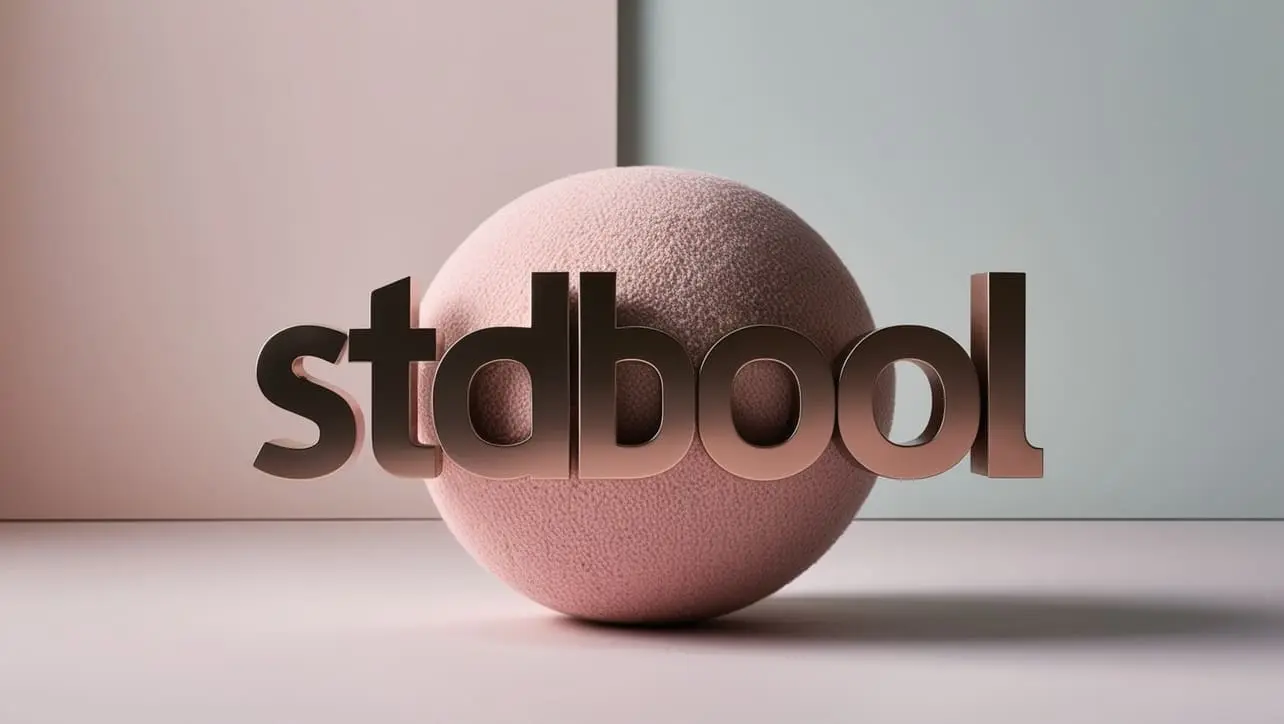
C goto Statement
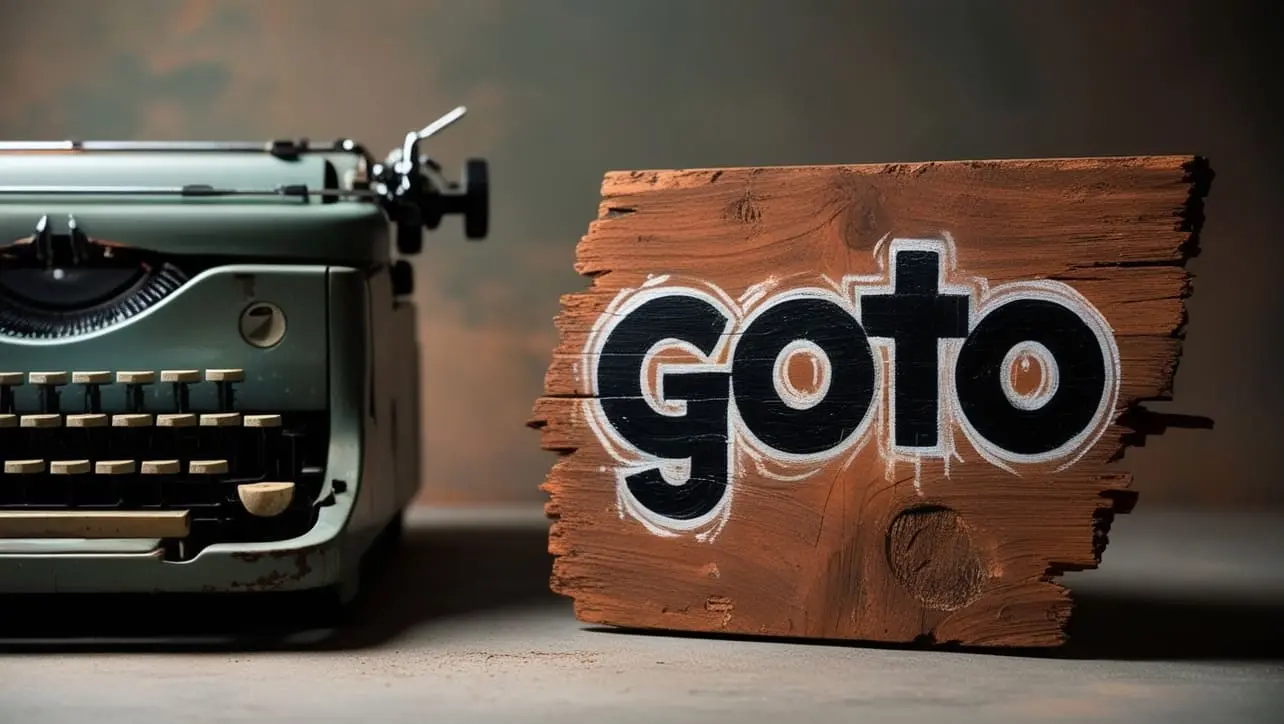
Photo Credit to CodeToFun
Introduction
The goto
statement in C is a control statement that provides an unconditional jump from the goto
statement to a labeled statement within the same function. While often discouraged due to potential readability and maintainability issues, goto
can be useful in certain scenarios, such as breaking out of deeply nested loops or handling errors in a clean way.
What is the goto Statement?
In C programming, the goto
statement allows you to transfer control to a labeled statement elsewhere in the function. This can be useful for simplifying complex control flows, but it should be used sparingly to avoid "spaghetti code," which is difficult to understand and maintain.
Key Features
- Unconditional Jump: Transfers control to a specific labeled statement.
- Flexible Control Flow: Can be used to exit from multiple loops or perform complex jumps within a function.
- Error Handling: Can simplify error handling in certain cases by jumping to a cleanup section of code.
Syntax
The syntax for the goto
statement consists of the goto
keyword followed by a label name. The label is an identifier followed by a colon:
goto label;
...
label:
statement;
Example: Using goto to Exit Nested Loops
Here is an example demonstrating how goto
can be used to break out of nested loops:
#include <stdio.h>
int main() {
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
if (i == 1 && j == 1) {
goto exit_loops; // Exit both loops
}
printf("i = %d, j = %d\n", i, j);
}
}
exit_loops:
printf("Exited the loops\n");
return 0;
}
Output
In this example, the goto
statement exits both the inner and outer loops when i == 1 and j == 1.
i = 0, j = 0 i = 0, j = 1 i = 0, j = 2 i = 1, j = 0 Exited the loops
Common Uses
Error Handling:
The
goto
statement can be useful for error handling, allowing you to jump to a cleanup section at the end of a function.example.cCopied#include <stdio.h> #include <stdlib.h> int main() { FILE *file = fopen("example.txt", "r"); if (file == NULL) { goto error; // Jump to error handling section } // Process the file // ... fclose(file); return 0; error: printf("Failed to open the file\n"); // Perform cleanup if necessary return 1; }
Common Pitfalls and Best Practices
Avoid Overuse:
Using
goto
excessively can lead to code that is difficult to read and maintain. It's best to usegoto
only when it simplifies the control flow significantly.Maintain Readability:
When using
goto
, ensure that the labels are well-named and the control flow remains clear. Avoid jumping around large sections of code.Use Alternative Constructs:
In many cases, alternative constructs like loops, functions, and break statements can achieve the same results without the downsides of
goto
.
Conclusion
The goto
statement in C provides a way to perform an unconditional jump to a labeled statement. While it can be useful in specific scenarios such as breaking out of nested loops or handling errors, it should be used sparingly to maintain code readability and maintainability.
By understanding the proper use cases and best practices, you can make informed decisions about when to use goto
in your C programs.
Join our Community:
Author
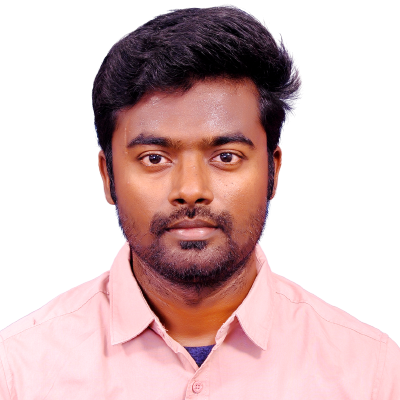
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C goto Statement), please comment here. I will help you immediately.