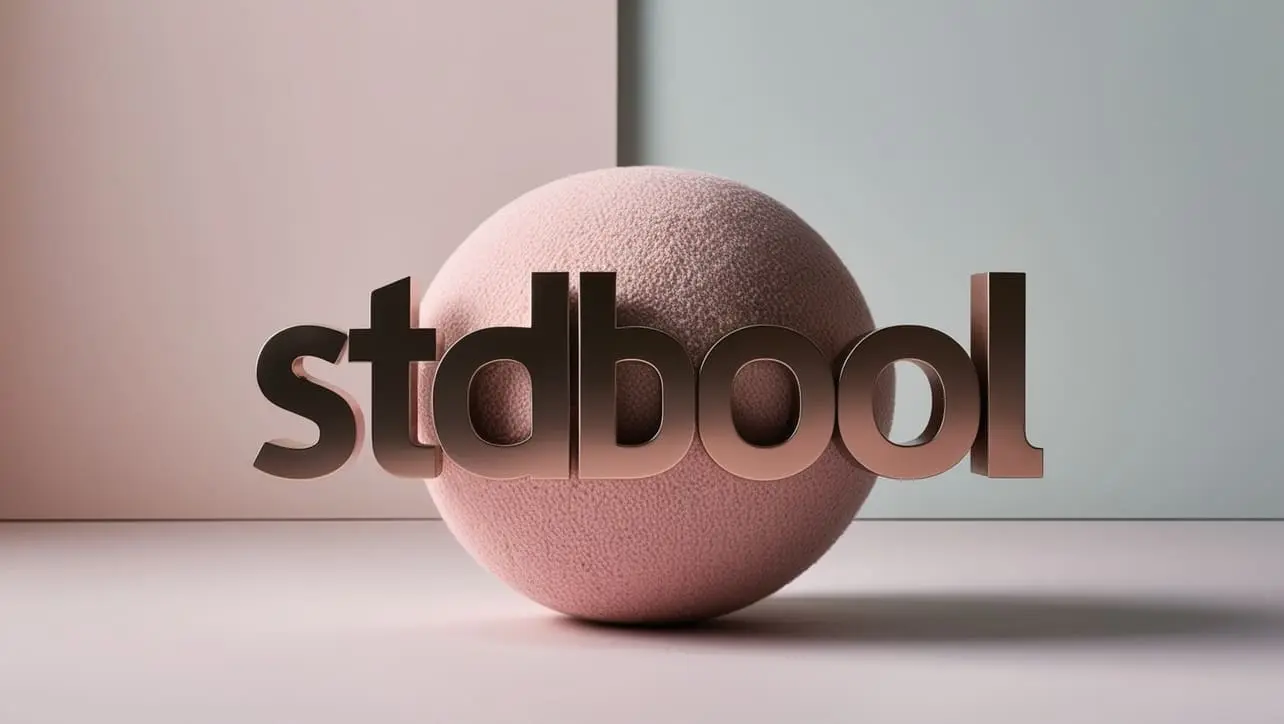
C for Loop
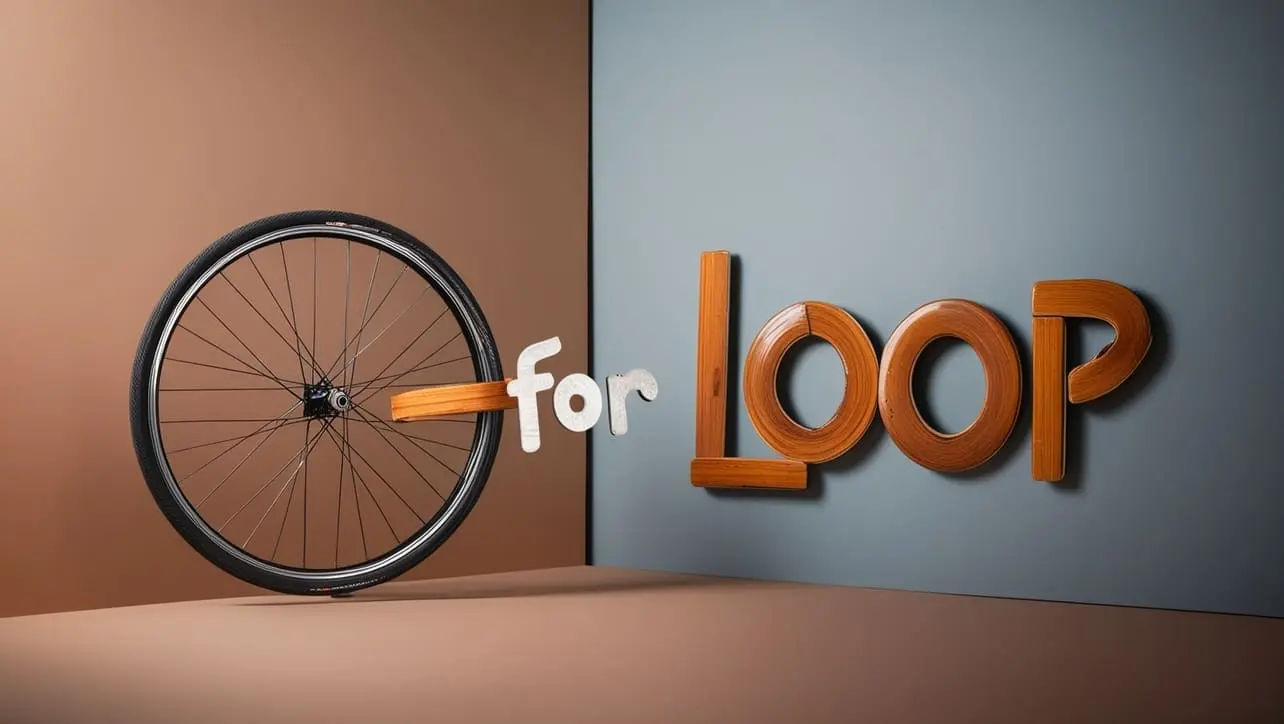
Photo Credit to CodeToFun
🙋 Introduction
The for
loop in C is a powerful control statement that allows you to repeat a block of code a specified number of times.
This guide will provide an in-depth look at how the for
loop works, its syntax, and practical examples of its usage.
🤔 What is a for Loop?
In C programming, the for
loop is used to iterate over a block of code multiple times. It is typically used when the number of iterations is known before entering the loop. The for
loop provides a compact way to initialize, test, and increment a loop variable.
🔑 Key Features
- Control Flow: Iterates a block of code multiple times.
- Compact Syntax: Combines initialization, condition-checking, and increment/decrement in one line.
- Versatility: Can be used for various iteration tasks, including counting and iterating over arrays.
💡 Syntax
The syntax for the for loop is:
for (initialization; condition; increment) {
// Code to be executed for each iteration
}
Components of the for Loop
- Initialization: Sets the starting value of the loop control variable.
- Condition: Tests the loop control variable. If true, the loop body executes. If false, the loop terminates.
- Increment: Updates the loop control variable after each iteration.
📄 Basic Example
Here's a simple example of a for
loop that prints numbers from 0 to 4:
#include <stdio.h>
int main() {
for (int i = 0; i < 5; i++) {
printf("%d\n", i);
}
return 0;
}
💻 Output
0 1 2 3 4
🧠 Explanation
- Initialization: int i = 0 initializes the loop variable i to 0.
- Condition: i < 5 tests whether i is less than 5.
- Increment: i++ increments i by 1 after each iteration.
🔝 Advanced Examples
Iterating Over an Array
The for
loop is commonly used to iterate over arrays:
#include <stdio.h>
int main() {
int arr[] = {10, 20, 30, 40, 50};
int n = sizeof(arr) / sizeof(arr[0]);
for (int i = 0; i < n; i++) {
printf("%d\n", arr[i]);
}
return 0;
}
This example prints each element of the array arr.
Nested for Loops
for
loops can be nested to iterate over multi-dimensional arrays or perform complex iteration tasks:
#include <stdio.h>
int main() {
for (int i = 1; i <= 3; i++) {
for (int j = 1; j <= 3; j++) {
printf("i = %d, j = %d\n", i, j);
}
}
return 0;
}
💻 Output
i = 1, j = 1 i = 1, j = 2 i = 1, j = 3 i = 2, j = 1 i = 2, j = 2 i = 2, j = 3 i = 3, j = 1 i = 3, j = 2 i = 3, j = 3
Common Pitfalls and Best Practices
Infinite Loops:
An infinite loop occurs when the loop condition always evaluates to true. Ensure your loop has a valid terminating condition:
example.cCopiedfor (int i = 0; i < 5; ) { // Missing increment; will cause an infinite loop printf("%d\n", i); }
Off-by-One Errors:
Off-by-one errors occur when the loop iterates one time too many or too few. Double-check your loop conditions:
example.cCopiedfor (int i = 0; i <= 5; i++) { // Will print 6 numbers, from 0 to 5 printf("%d\n", i); }
Proper Initialization:
Ensure the loop variable is correctly initialized. Incorrect initialization can lead to unexpected results:
example.cCopiedfor (int i = 1; i < 5; i++) { // Correct initialization to avoid skipping the first iteration printf("%d\n", i); }
🎉 Conclusion
The for
loop is an essential control structure in C programming that enables repeated execution of a block of code. Its compact syntax and flexibility make it suitable for various tasks, from simple counting to complex iteration over arrays and nested structures.
By understanding its components and best practices, you can harness the full power of the for
loop in your C programs.
👨💻 Join our Community:
Author
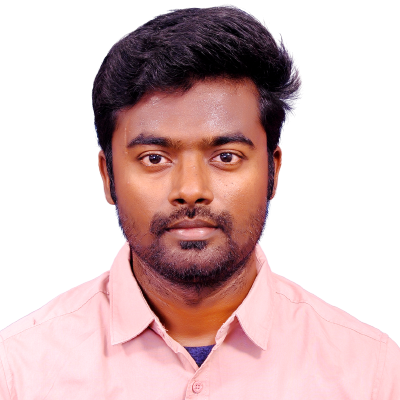
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C for Loop), please comment here. I will help you immediately.