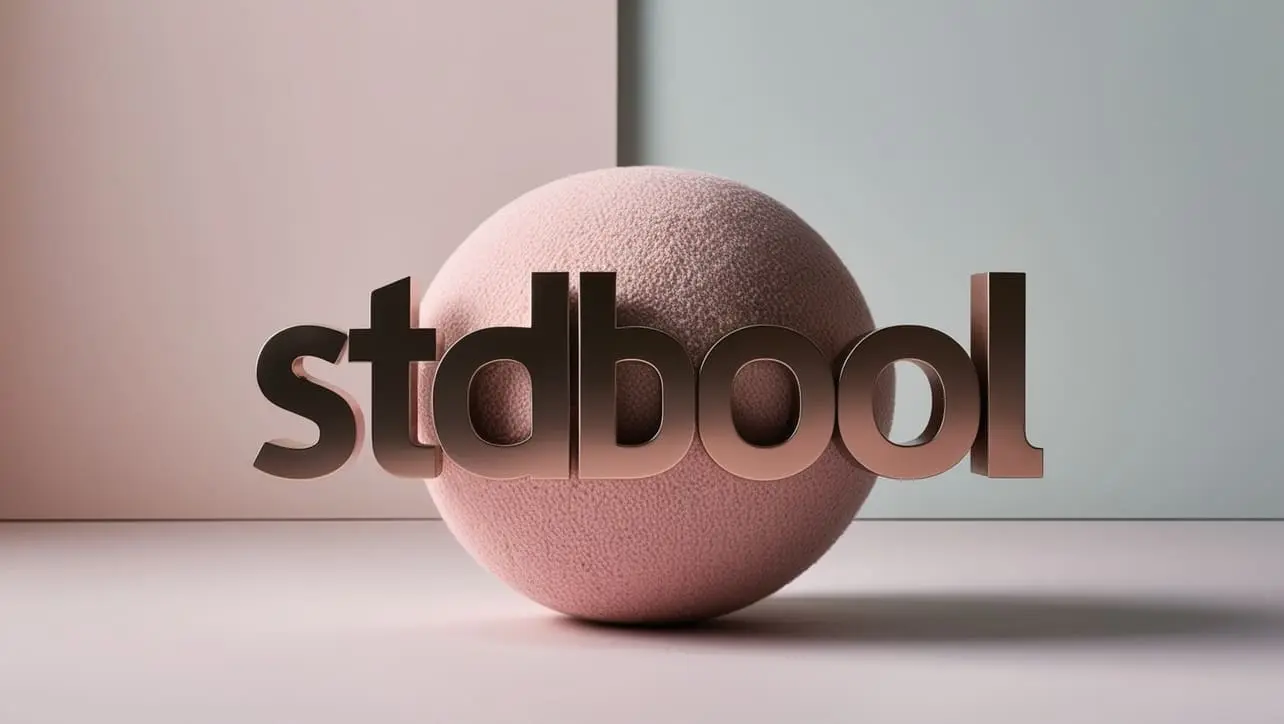
C Library โ errno.h
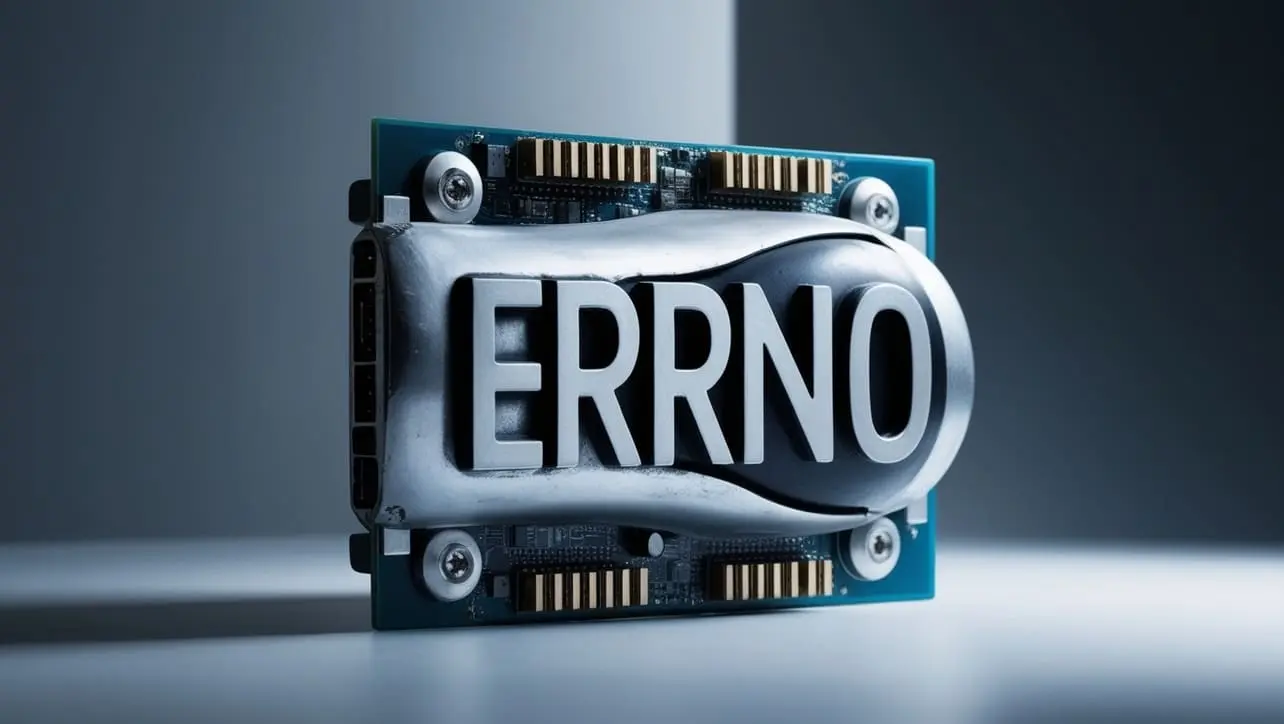
Photo Credit to CodeToFun
Introduction
The errno.h
header in C defines the integer variable errno, which is set by system calls and some library functions in the event of an error to indicate what went wrong. The errno variable is set to zero at program startup and is never reset by any library function. Therefore, it should be explicitly reset to zero before calling a function if the program needs to check for errors.
Syntax
To use the errno.h
library, you need to include it in your program:
#include <errno.h>
Important Macros and Variables
The errno.h
header defines a variable and several macros that represent different error codes. Some common error codes include:
- EDOM: Domain error, typically used when a function like sqrt receives a negative argument.
- ERANGE: Range error, typically used when a result is too large to be represented.
- EINTR: Interrupted function call.
- EINVAL: Invalid argument.
Syntax for Using errno
Declaring errno:
example.cCopiedextern int errno;
- Checking errno after a function call:
example.cCopied
#include <errno.h> #include <stdio.h> #include <math.h> int main() { errno = 0; // Reset errno before function call double result = sqrt(-1); if (errno != 0) { perror("Error occurred"); } return 0; }
Example
Below is a simple example demonstrating how to use the errno variable and perror function to handle errors. This example attempts to open a file that does not exist and checks the errno value if the fopen function fails.
#include <stdio.h>
#include <errno.h>
#include <string.h>
int main() {
FILE *file = fopen("nonexistent.txt", "r");
if (file == NULL) {
printf("Error opening file: %s\n", strerror(errno));
} else {
fclose(file);
}
return 0;
}
Output
Error opening file: No such file or directory
How the Program Works
- Function Call: The fopen function attempts to open a file named "nonexistent.txt" for reading.
- Error Handling: If the file does not exist, fopen returns NULL, and errno is set to indicate the error.
- Printing the Error: The strerror function converts the errno value to a human-readable string, which is printed to the console.
Common Pitfalls
- Not Resetting `errno`: Forgetting to reset errno before a function call can lead to incorrect error handling, as errno may still hold a value from a previous error.
- Ignoring `errno`: Not checking errno after a function that sets it can cause missed error conditions and lead to undefined behavior.
- Misinterpreting `errno` Values: Misunderstanding the meaning of specific errno values can lead to improper error handling.
Best Practices
- Always Reset `errno`: Set errno to zero before a function call if you intend to check it for errors.
- Check `errno` Immediately: Check errno immediately after the function call to ensure that no other operations have altered it.
- Use `perror` or `strerror`: Utilize perror or strerror to print meaningful error messages instead of just printing the errno value.
- Document Error Handling: Clearly document which functions set errno and what the expected error codes are, to ensure proper error handling.
Conclusion
The errno.h
header is an essential tool for error handling in C, allowing programs to detect and respond to errors effectively.
By understanding how to use errno and related macros, programmers can create more robust and reliable code. However, it is crucial to follow best practices and be aware of common pitfalls to avoid potential issues in error handling.
Join our Community:
Author
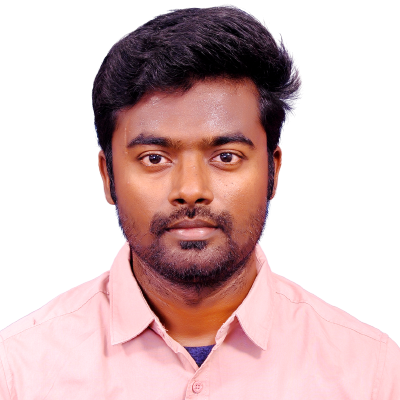
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C Library โ errno.h), please comment here. I will help you immediately.