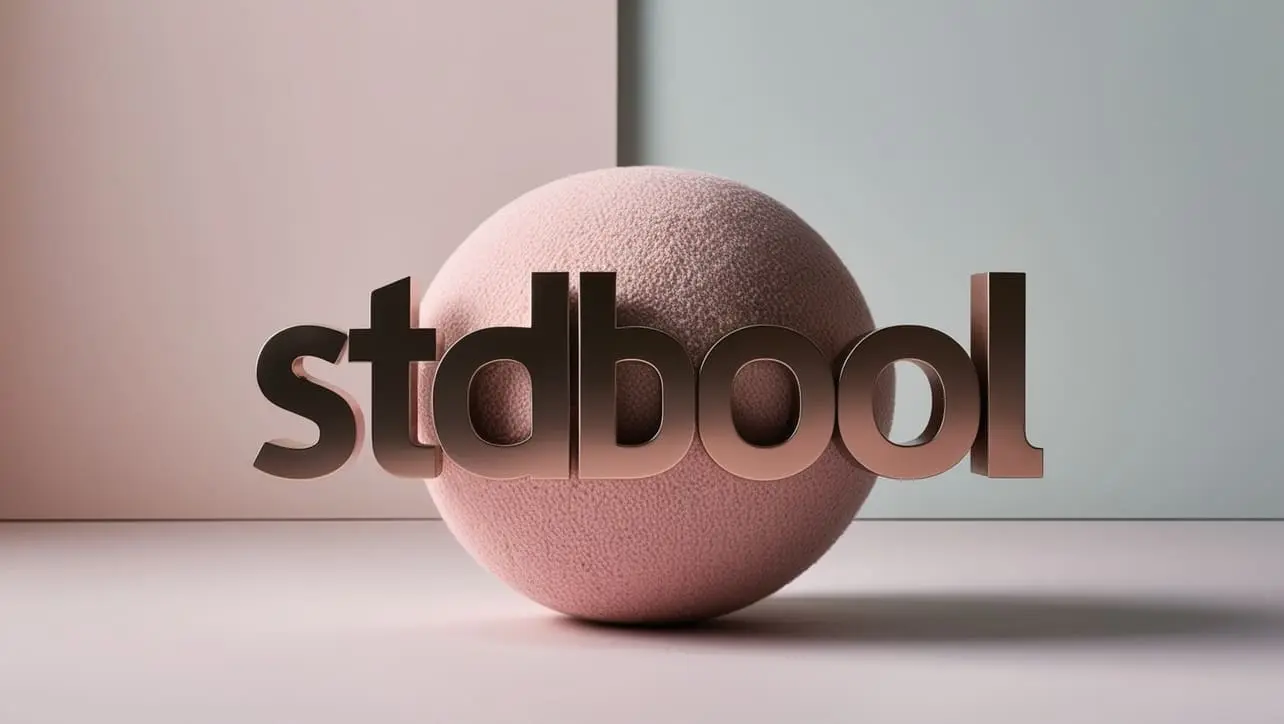
C do while Loop
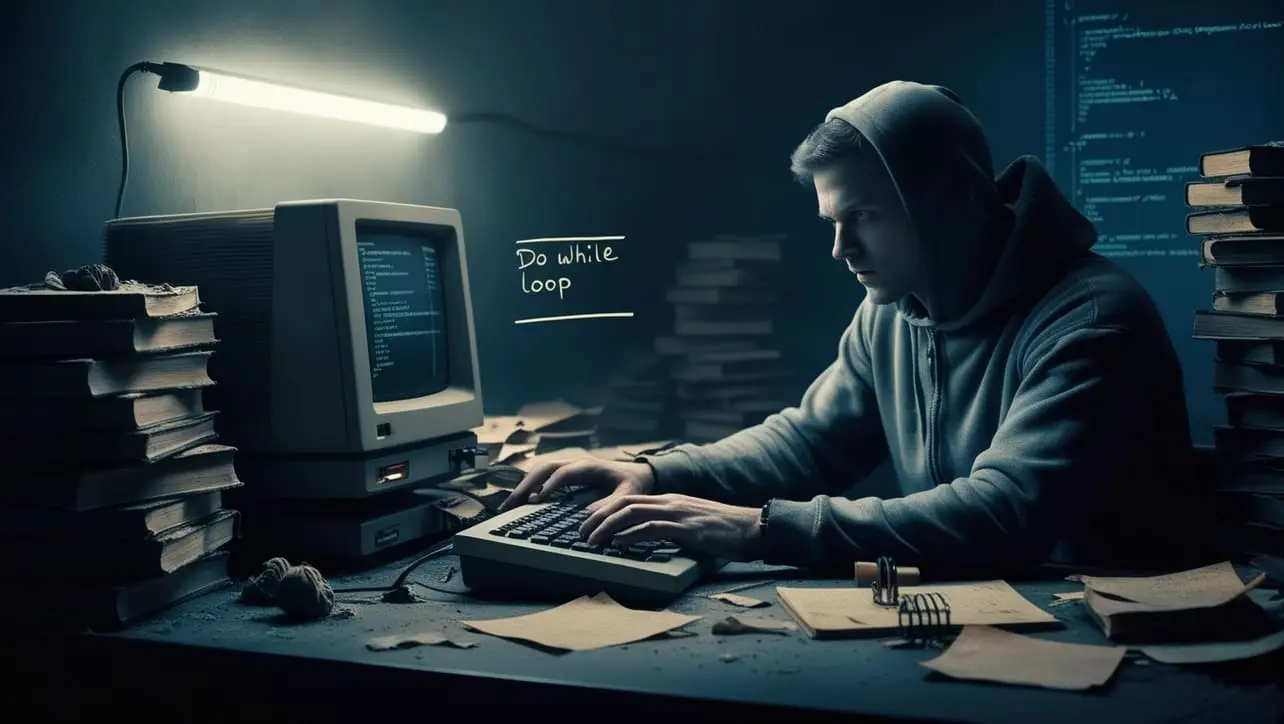
Photo Credit to CodeToFun
π Introduction
The do while
loop in C is a control flow statement that allows code to be executed repeatedly based on a given Boolean condition.
Unlike other loops (for and while), a do while
loop will always execute at least once, even if the condition is false.
π€ What is a do while Loop?
A do while
loop is a post-tested loop, meaning the condition is evaluated after the loop body has been executed. This ensures that the loop executes at least once before any condition check is performed.
π Key Features
- Guaranteed Execution: The loop body executes at least once, regardless of the condition.
- Post-Test Loop: The condition is evaluated after the loop body has been executed.
- Flexible Control Flow: Useful for scenarios where the loop body needs to execute before checking the condition.
π‘ Syntax
The syntax for the do while
loop is as follows:
do {
// Code to be executed
} while (condition);
Explanation
- do: Initiates the loop.
- { }: Contains the code block that will execute.
- while (condition): The condition to be tested after the code block executes. If true, the loop repeats; if false, the loop terminates.
Basic Example
Hereβs a simple example to illustrate a do while
loop:
#include <stdio.h>
int main() {
int i = 0;
do {
printf("%d\n", i);
i++;
} while (i < 5);
return 0;
}
π» Output
In this example, the loop will print the value of i from 0 to 4. The condition i < 5 is evaluated after the loop body executes, ensuring that the body runs at least once.
0 1 2 3 4
Practical Usage
The do while
loop is particularly useful in scenarios where the loop body must execute at least once, such as:
- Input Validation: Prompting user input and validating it in the loop.
- Menus: Displaying a menu at least once before repeating based on user choice.
Example: Input Validation
#include <stdio.h>
int main() {
int num;
do {
printf("Enter a number between 1 and 10: ");
scanf("%d", &num);
} while (num < 1 || num > 10);
printf("You entered: %d\n", num);
return 0;
}
In this example, the user is prompted to enter a number between 1 and 10. The loop ensures the prompt appears at least once and repeats until a valid number is entered.
Example: Menu Display
#include <stdio.h>
int main() {
int choice;
do {
printf("Menu:\n");
printf("1. Option 1\n");
printf("2. Option 2\n");
printf("3. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("Option 1 selected.\n");
break;
case 2:
printf("Option 2 selected.\n");
break;
case 3:
printf("Exiting...\n");
break;
default:
printf("Invalid choice, please try again.\n");
}
} while (choice != 3);
return 0;
}
In this menu example, the menu is displayed at least once and continues to display until the user selects the exit option.
Common Pitfalls and Best Practices
Infinite Loops:
Be cautious of creating infinite loops with do while. Ensure the condition will eventually become false to terminate the loop.
Condition Placement:
Remember that the condition is checked after the loop body executes. Ensure this behavior aligns with your program's logic.
Clear Conditions:
Write clear and concise conditions to maintain readability and prevent logical errors.
π Conclusion
The do while
loop is a valuable control flow statement in C that guarantees the loop body executes at least once.
By understanding its syntax and practical applications, you can effectively use do while
loops in your programs to handle scenarios requiring at least one execution before condition checking.
π¨βπ» Join our Community:
Author
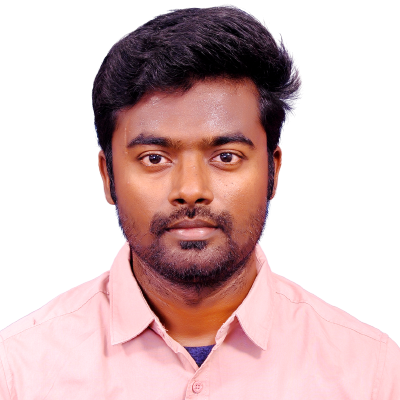
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C do while Loop), please comment here. I will help you immediately.