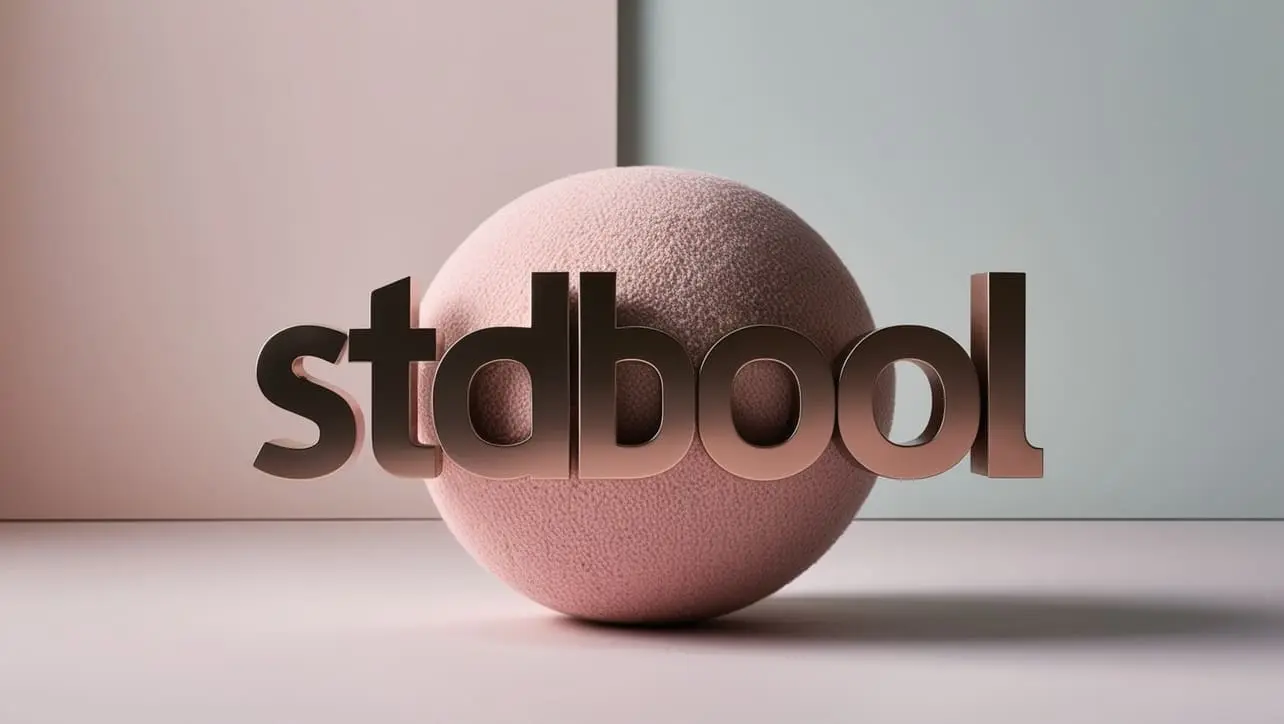
C Topics
- C Intro
- C Control Statement
- C Control Loops
- C String Functions
- C Math Functions
- C Header Files
- C Interview Programs
- Abundant Number
- Amicable Number
- Armstrong Number
- Average of N Numbers
- Automorphic Number
- Biggest of three numbers
- Binary to Decimal
- Common Divisors
- Composite Number
- Condense a Number
- Cube Number
- Decimal to Binary
- Decimal to Octal
- Disarium Number
- Even Number
- Evil Number
- Factorial of a Number
- Fibonacci Series
- GCD
- Happy Number
- Harshad Number
- LCM
- Leap Year
- Magic Number
- Matrix Addition
- Matrix Division
- Matrix Multiplication
- Matrix Subtraction
- Matrix Transpose
- Maximum Value of an Array
- Minimum Value of an Array
- Multiplication Table
- Natural Number
- Number Combination
- Odd Number
- Palindrome Number
- Pascalβs Triangle
- Power of 2
- Power of 3
- Pronic Number
- Perfect Number
- Perfect Square
- Prime Factor
- Prime Number
- Smith Number
- Strong Number
- Sum of Array
- Sum of Digits
- Swap Two Numbers
- Triangular Number
- C Star Pattern
- C Number Pattern
- C Alphabet Pattern
C Program to Check for a Cube Number
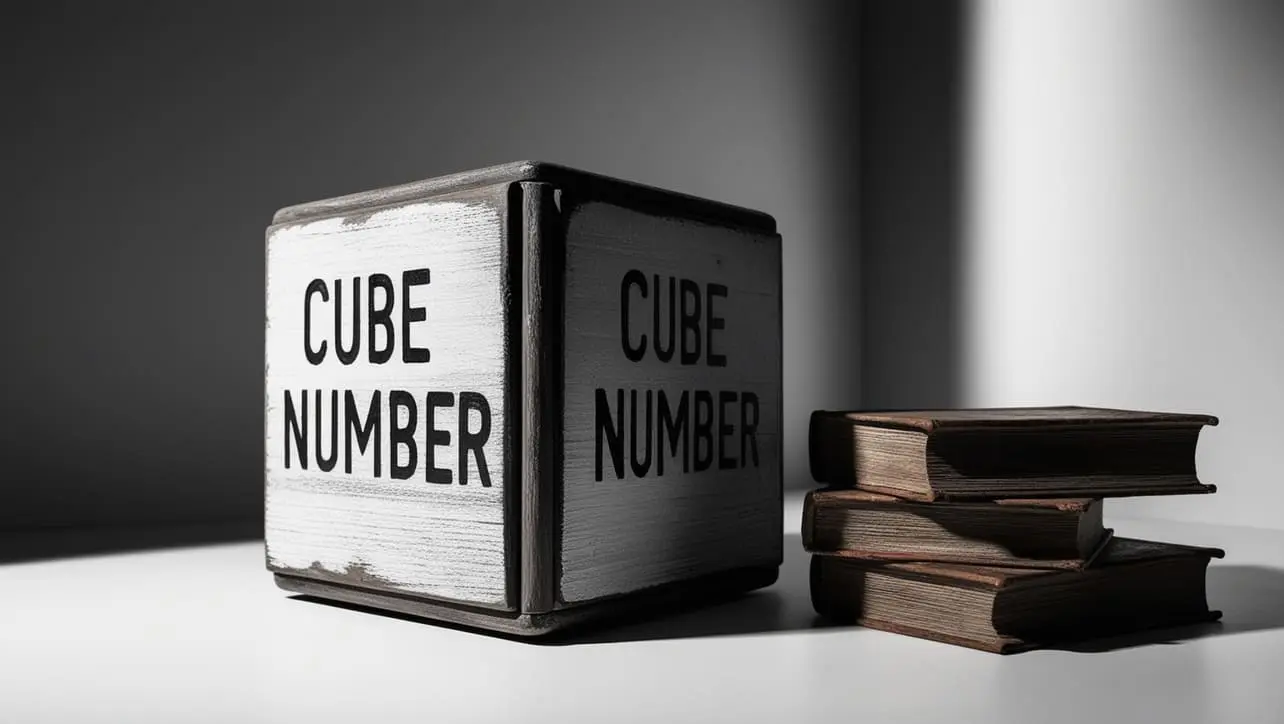
Photo Credit to CodeToFun
π Introduction
In the realm of programming, mathematical properties of numbers often lead to interesting and practical applications. One such property is being a cube number. A cube number is an integer that is the cube of an integer.
In this tutorial, we'll explore a C program that efficiently checks if a given number is a cube number..
π Example
Let's delve into the C code that achieves this functionality.
#include <stdio.h>
#include <math.h>
// Function to check if a number is a cube
int isCube(int number) {
// Calculate the cube root and round to the nearest integer
double cubeRoot = cbrt(number);
int roundedCubeRoot = (int)(cubeRoot + 0.5); // Adding 0.5 for proper rounding
// Check if the cube of the rounded cube root is equal to the input number
return (roundedCubeRoot * roundedCubeRoot * roundedCubeRoot) == number;
}
// Driver program
int main() {
// Replace this value with your desired number
int inputNumber = 27;
// Check if the number is a cube
if (isCube(inputNumber)) {
printf("%d is a cube number.\n", inputNumber);
} else {
printf("%d is not a cube number.\n", inputNumber);
}
return 0;
}
π» Testing the Program
To test the program with different numbers, simply replace the value of inputNumber in the main function.
27 is a cube number.
Compile and run the program to see if the number is a cube.
π§ How the Program Works
- The program defines a function isCube that takes a number as input and calculates its cube root, rounding to the nearest integer.
- It checks if the cube of the rounded cube root is equal to the input number, determining whether it's a cube number.
- The driver program (main function) replaces the value of inputNumber with the desired number and calls the isCube function to check if it's a cube number.
- The program prints whether the input number is a cube number or not based on the result of the isCube function.
π Between the Given Range
Let's dive into the C code that checks whether a number in the range from 1 to 50 is a cube number.
#include <stdio.h>
// Function to check if a number is a cube number
int isCubeNumber(int num) {
int cubeRoot = 1;
// Find the cube root of the number
while (cubeRoot * cubeRoot * cubeRoot < num) {
cubeRoot++;
}
// Check if the cube of the cube root is equal to the number
return cubeRoot * cubeRoot * cubeRoot == num;
}
// Driver program
int main() {
printf("Cube numbers in the range 1 to 50:\n");
// Check and print cube numbers in the range 1 to 50
for (int i = 1; i <= 50; i++) {
if (isCubeNumber(i)) {
printf("%d ", i);
}
}
printf("\n");
return 0;
}
π» Testing the Program
Cube numbers in the range 1 to 50: 1 8 27
Compile and run the program to see the cube numbers within the range of 1 to 50.
π§ How the Program Works
- The program defines a function isCubeNumber that checks if a given number is a cube number.
- Inside the function, it finds the cube root of the number and checks if the cube of the cube root is equal to the original number.
- The main function iterates through the numbers from 1 to 50 and prints those that are cube numbers.
π§ Understanding the Concept of Cube Number
A cube number is the result of raising an integer to the power of 3. For example, 2 * 2 * 2 = 8, so 8 is a cube number.
π’ Optimizing the Program
The provided program is straightforward, but you might consider optimizing it further. For instance, you can explore alternative methods for checking if a number is a cube number without using the cbrt function.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
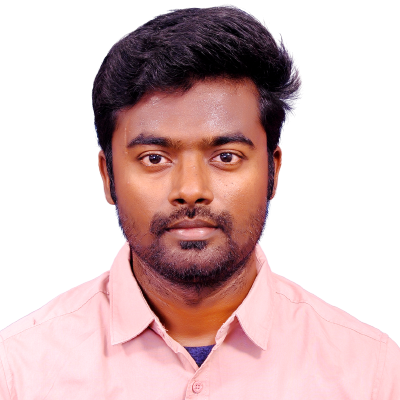
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C Program to Check for a Cube Number), please comment here. I will help you immediately.