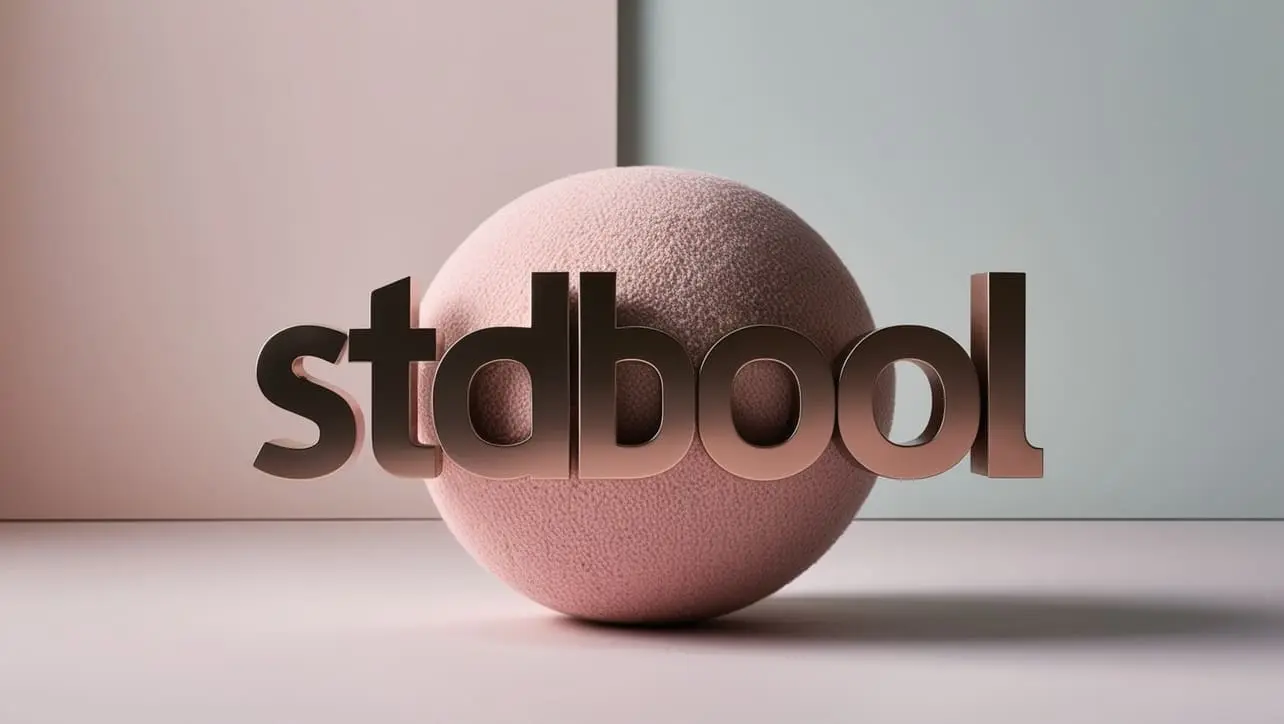
C Library – ctype.h
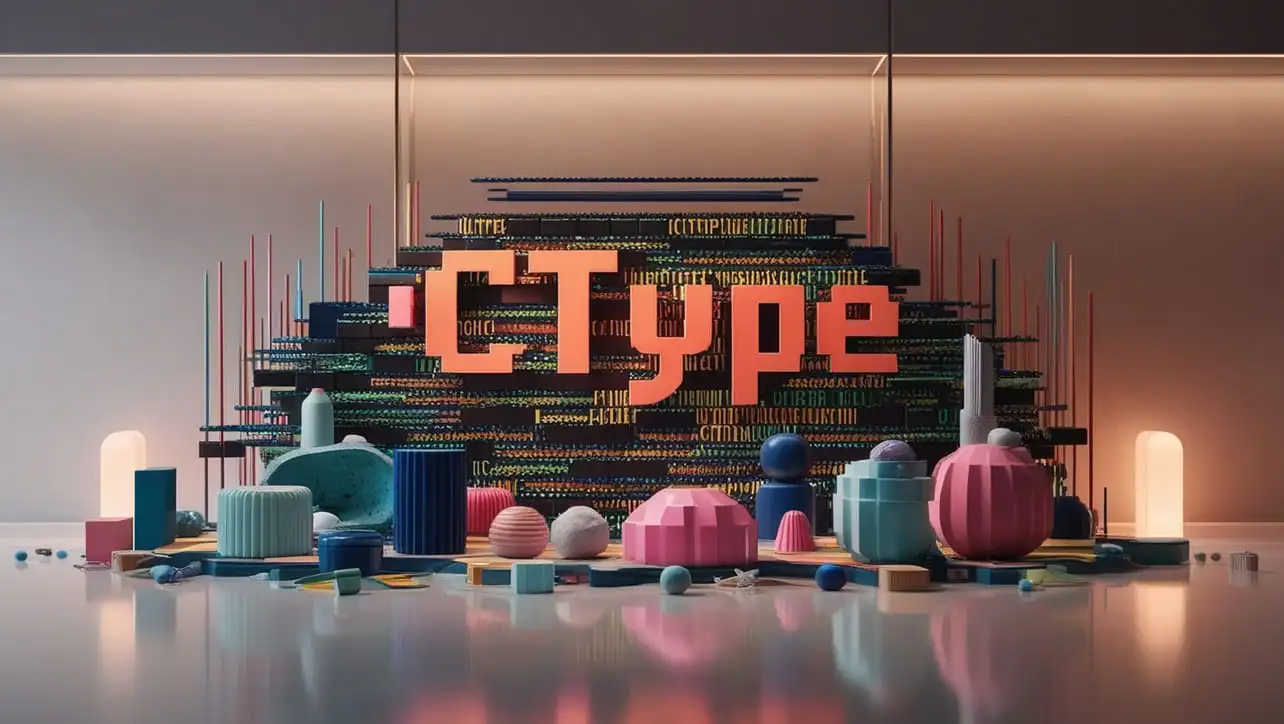
Photo Credit to CodeToFun
Introduction
The ctype.h
header in C provides a set of functions to test and map characters. These functions can determine the type of a character (e.g., alphabetic, digit, etc.) and perform transformations on characters (e.g., converting to uppercase or lowercase).
This library is particularly useful for handling character data in a more readable and portable manner.
Syntax
To use the ctype.h
library, you need to include it in your program:
#include <ctype.h>
Functions Defined in ctype.h
The ctype.h
header defines several functions to test and map characters. Here are some of the most commonly used functions:
Character Testing Functions
- int isalnum(int c): Checks if the character is alphanumeric.
- int isalpha(int c): Checks if the character is alphabetic.
- int isdigit(int c): Checks if the character is a digit.
- int islower(int c): Checks if the character is lowercase.
- int isupper(int c): Checks if the character is uppercase.
- int isspace(int c): Checks if the character is a whitespace character.
Character Mapping Functions
- int tolower(int c): Converts the character to lowercase if it is uppercase.
- int toupper(int c): Converts the character to uppercase if it is lowercase.
Syntax of the Functions
int isalnum(int c);
int isalpha(int c);
int isdigit(int c);
int islower(int c);
int isupper(int c);
int isspace(int c);
int tolower(int c);
int toupper(int c);
Example
Below is a simple example demonstrating how to use some of the ctype.h
functions to test and map characters.
#include <stdio.h>
#include <ctype.h>
void analyze_character(char c) {
if (isalpha(c)) {
printf("%c is an alphabetic character.\n", c);
if (islower(c)) {
printf("%c is lowercase. Its uppercase form is %c.\n", c, toupper(c));
} else {
printf("%c is uppercase. Its lowercase form is %c.\n", c, tolower(c));
}
} else if (isdigit(c)) {
printf("%c is a digit.\n", c);
} else if (isspace(c)) {
printf("%c is a whitespace character.\n", c);
} else {
printf("%c is a special character.\n", c);
}
}
int main() {
char characters[] = {'a', 'Z', '5', ' ', '@'};
for (int i = 0; i < sizeof(characters) / sizeof(char); i++) {
analyze_character(characters[i]);
}
return 0;
}
Output
5 is a digit. is a whitespace character. @ is a special character.
How the Program Works
- Function Definition: The analyze_character function takes a character and analyzes its type using functions from
ctype.h
. - Character Testing: The function uses isalpha, islower, toupper, isdigit, and isspace to determine and manipulate the character.
- Main Function: The main function demonstrates the analyze_character function with an array of different characters.
Common Pitfalls
- Character Encoding: Ensure that the input characters are in the correct encoding (usually ASCII) as
ctype.h
functions are typically designed for standard character sets. - Undefined Behavior: Passing values outside the range of unsigned char (typically -1 to 255) to these functions can result in undefined behavior.
- Locale Dependency: Some functions may behave differently depending on the current locale setting, which can affect character classification and transformation.
Best Practices
- Correct Data Types: Always use int for character testing functions, as these functions expect an int and not a char.
- Use Proper Libraries: Include
ctype.h
to ensure that the functions are properly declared. - Locale Management: Be aware of locale settings and how they may affect the behavior of
ctype.h
functions. Use setlocale if necessary to ensure consistent behavior.
Conclusion
The ctype.h
header in C provides a robust set of functions for testing and mapping characters, making it easier to handle character data in your programs.
By using these functions, you can write cleaner and more portable code. However, it is essential to be mindful of common pitfalls such as character encoding issues and undefined behavior, and to follow best practices to ensure reliable and consistent results.
Join our Community:
Author
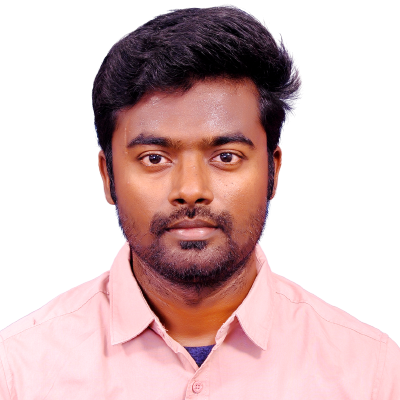
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C Library – ctype.h), please comment here. I will help you immediately.